23 Javascript Function Declaration Syntax
May 02, 2020 - The syntax that we used before is called a Function Declaration: ... There is another syntax for creating a function that is called a Function Expression. Jun 21, 2020 - The JavaScript development scope for writing blocks of codes brings a lot of confusion for newbie JS developers, including some intermediate devs regarding the use of function declaration…
Javascript Functions Concept To Ease Your Web Development
A parameter is the variable listed inside the parentheses in the function declaration (it's a declaration time term) An argument is the value that is passed to the function when it is called (it's a call time term). We declare functions listing their parameters, then call them passing arguments.
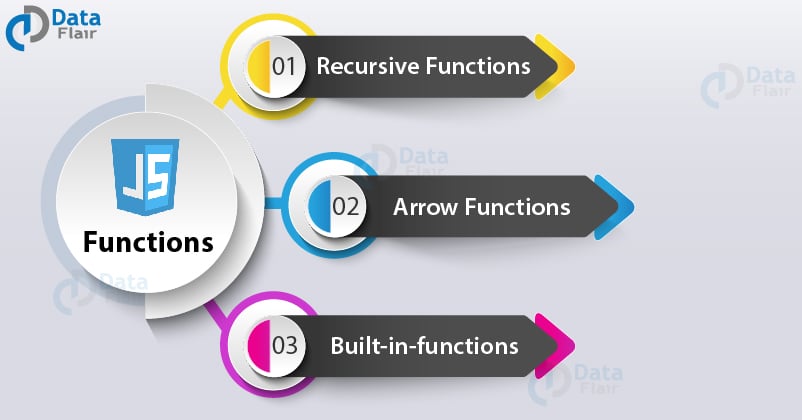
Javascript function declaration syntax. 9/3/2020 · JavaScript functions Syntax is a really important matter. Syntax: function functionName(x, y, z,………….)//function definition {//code or logic} functionName(1,2,3,…….);//function calling. Explanation: The function is declared by using the function keyword. Function name is functionName(x,y,z) and called function definition. In this tutorial, we will learn several ways to define a function, call a function, and use function parameters in JavaScript. Defining a Function. Functions are defined, or declared, with the function keyword. Below is the syntax for a function in JavaScript. function nameOfFunction() { // Code to be executed } The declaration begins with the ... A JavaScript function is a procedure or a subprogram, i.e., a block of code that performs a certain task. It is a group of reusable code that you can use anywhere in the code. It helps you to divide a large program into small and manageable functions. With the help of functions, you don't need to write the same block of code repeatedly.
JavaScript Function Syntax A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. This is a valid declaration of a function in JavaScript, rather a powerful one (I think). It is not only declaring the function, but also storing the declaration in the variable named add. This is ...
Nov 10, 2017 - A declared function is “saved for later use”, and will be executed later, when it is invoked (called). Just as Variable Declarations must start with “var”, Function Declarations must begin with… Feb 01, 2020 - There are three common ways of ... two are types of “Arrow Functions”, which have the shortest syntax for a function. The syntactical differences between them are like so: Function Declaration is declared as a separate statement, in the main code flow.... The declaration of a function start with the function keyword, followed by the name of the function you want to create, followed by parentheses i.e. () and finally place your function's code between curly brackets {}. Here's the basic syntax for declaring a function: function functionName () {
JavaScript | Function expression. Function Expression allows us to create an anonymous function which doesn't have any function name which is the main difference between Function Expression and Function Declaration. A function expression can be used as an IIFE (Immediately Invoked Function Expression) which runs as soon as it is defined. The function expression is the working horse in JavaScript. Usually, you deal with this type of function declaration, alongside the arrow function (if you prefer short syntax and lexical context). 2.1 Named function expression A function is anonymous when it does not have a name (name property is an empty string ''): Function declarations in JavaScript are hoisted to the top of the enclosing function or global scope. You can use the function before you declared it: hoisted ( ) ; // logs "foo" function hoisted ( …
Summary In short, use function declarations when you want to create a function on the global scope and make it available throughout your code. Use function expressions to limit where the function is available, keep your global scope light, and maintain clean syntax. The form a function expression takes is function () {}. To invoke the expression when its declared you must change the format to (function () {} ()) The Function Declaration (1) creates the function putting it into the variable named welcome. Line (2) copies the variable welcome into the variable anotherFunc. It is possible to call the function as both welcome () and anotherFunc (). If we have used a Function Expression to declare welcome, in the first line nothing would change:
In JavaScript, if no return value is specified, the function will return undefined. Functions are objects. Define a Function. There are a few different ways to define a function in JavaScript: A Function Declaration defines a named function. To create a function declaration you use the function keyword followed by the name of the function. When ... Learn JavaScript - Functions as a variable. Example. A normal function declaration looks like this: function foo(){ } A function defined like this is accessible from anywhere within its context by its name. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The syntax of JavaScript is the set of rules that define a correctly structured JavaScript program. The examples below make use of the log function of the console object present in most browsers for standard text output. The JavaScript standard library lacks an official standard text output function (with the exception of document.write). Since JavaScript function declaration is not an executable statement, it is not common to end it with a semicolon. Function expression stands for a function which is stored in a variable: Example. var a = function (b, c) { return b * c} Try it Live. Aug 15, 2016 - Dan Prince looks at factory functions in JavaScript, examining the different types, their use cases & how they allow us to separate data from computations.
JavaScript variables can hold numbers like 100 and text values like "John Doe". In programming, text values are called text strings. JavaScript can handle many types of data, but for now, just think of numbers and strings. Strings are written inside double or single quotes. Numbers are written without quotes. Feb 20, 2018 - Almost three years after arrow functions were added to JavaScript, they remain to be one of the most asked about features of the language. In this article we’ll explore the shorter syntax of arrow… A JavaScript class is a blueprint for creating objects. A class encapsulates data and functions that manipulate data. Unlike other programming languages such as Java and C#, JavaScript classes are syntactic sugar over the prototypal inheritance. In other words, ES6 classes are just special functions.
Declaring a Function The syntax to declare a function is: function nameOfFunction () { // function body } A function is declared using the function keyword. 1 week ago - A factory function is any function which is not a class or constructor that returns a (presumably new) object. In JavaScript, any function can return an object. When it does so without the new… A javascript function is a fundamental building block of code that is designed to define once and called multiple times to perform a different-different task. Also, there are different ways to declare the function in javascript. A javascript function is executed when "something" invokes it (calls it) using the invoke method.
Function declaration and arrow functions are different in their essence, but in the scope of your question, it's basically a code style preference. I personally prefer Function declaration as I find it easier to spot the meaning of that line of code. What class User {...} construct really does is:. Creates a function named User, that becomes the result of the class declaration.The function code is taken from the constructor method (assumed empty if we don't write such method).; Stores class methods, such as sayHi, in User.prototype.; After new User object is created, when we call its method, it's taken from the prototype, just as ... A function expression syntax returns a function rather than declaring a function that's why we need to it inside a variable or constant. Functions are first-class objects in JavaScript, which means...
1 week ago - Prior to ES6, there was a lot of confusion about the differences between a factory function and a constructor function in JavaScript. Since ES6 has the `class` keyword, a lot of people seem to think… They are pretty similar with some ... the normal way to create a function in JavaScript(Anonymous function Declaration), both has usage, cons and pros: ... A Function Expression defines a function as a part of a larger expression syntax (typically a variable assignment ). Functions ... See how TypeScript improves day to day working with JavaScript with minimal additional syntax. ... Explore how TypeScript extends JavaScript to add more safety and tooling.
A function definition (also called a function declaration, or function statement) consists of the function keyword, followed by: The name of the function. A list of parameters to the function, enclosed in parentheses and separated by commas. The JavaScript statements that define the function, enclosed in curly brackets, {...}. Jun 11, 2012 - However as kangax pointed out, ... syntax to be used anywhere a statement is allowed. It’s as yet non standard so not recommended for production development ... To quote Ben Cherry’s excellent article: “Function declarations and function variables are always moved (‘hoisted’) to the top of their JavaScript scope by the ... The first and most obvious way to declare a function in JavaScript is to use a function declaration. A function named multiply (), which takes two parameters x and y, multiplies them, and returns ...
The basic syntax to create a function in JavaScript is shown below. Syntax: function functionName (Parameter1, Parameter2, ..) { // Function body } To create a function in JavaScript, we have to first use the keyword function, separated by name of function and parameters within parenthesis. The part of function inside the curly braces {} is the ... Use the function keyword to declare a function. Use the functionName () to call or invoke a function to execute it. A function implicitly returns undefined, use the return statement to explicitly specify the return value for the function. The arguments is an object that is accessible only inside the function body.
Javascript Fixing Function Is Not Defined Error
What Is The Difference Between Function Declaration And
Creating Custom Javascript Syntax With Babel Tan Li Hau
Javascript What The Heck Is An Immediately Invoked Function
Javascript Functions Concept To Ease Your Web Development
Typescript Function Parameter Javatpoint
Javascript Function Declaration Syntax Var Fn Function
Apache Jmeter User S Manual Functions And Variables
Var Functionname Function Vs Function Functionname
Extract Functions Arguments Using Destructure In Javascript
Javascript Functions Studytonight
A Complete Guide To Css Functions Css Tricks
Exercise 1 Surface Area Of A Cylinder A Look Up The Chegg Com
Function In Javascript Function Declaration And Calling
Important Javascript Functions You Need To Know About Edureka
Javascript Function Declaration What Is A Function By
0 Response to "23 Javascript Function Declaration Syntax"
Post a Comment