22 How To Concatenate In Javascript
Code language: CSS (css) In this example, the job and location has the same property country.When we merged these objects, the result object (remoteJob) has the country property with the value from the second object (location).Merge objects using Object.assign() method. The Object.assign() method allows you to copy all enumerable own properties from one or more source objects to a target ... The + operator will concatenate two strings, ie. "Hello" + " World" //> "Hello World". Using += is a short cut for assigning and concatenating a variable with its self.
Best Way To Solve This Simple Concatenate Challenge
When used on strings, the + operator is called the concatenation operator. Adding Strings and Numbers. Adding two numbers, will return the sum, but adding a number and a string will return a string: ... Because of this, in JavaScript, ~ 5 will not return 10. It will return -6.
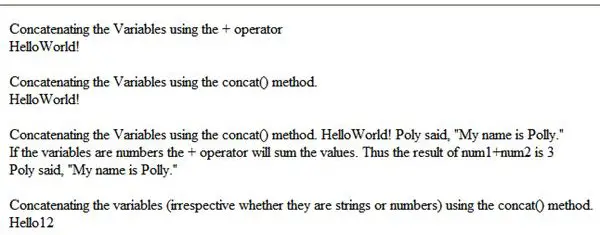
How to concatenate in javascript. JavaScript has a built-in method for merging strings or arrays together called concat().It allows you to take an array, combine it with one or many different arrays and make a new array containing the elements from all the arrays - the same principle applies to strings. how to concatenate strings javascript . javascript by Misty Mandrill on Jan 05 2020 Comment . 7. how to concatenate strings and variables in javascript . javascript by TechWhizKid on Apr 06 2020 Comment . 2. concantene number in js . javascript by Joyous Jaguar on Jun 08 2020 ... In vanilla JavaScript, there are multiple ways available to combine properties of two objects to create a new object. You can use ES6 methods like Object.assign () and spread operator (...) to perform a shallow merge of two objects. For a deeper merge, you can either write a custom function or use Lodash's merge () method.
To merge two or more arrays in JavaScript, the concat () function is used. The syntax for concatenating multiple arrays using the concat method is: array_name. concat(first_array, second_array,..., N_array) The concat () function takes multiple arrays as a parameter and joins them together as one array. Concatenate text and variables in javascript alert box with a new line character Feb 17, 2011 10:24 PM | johnzee | LINK Hi, I need to add text string and variables in a javascript alert box in code behind, for example code could give a message like this with a new line in between: JavaScript String concat() Method. The JavaScript concat() method combines two or more strings. concat() accepts as many parameters as you want, each one representing a string to merge. The concat() method is an alternative to the concatenation operator. Here's the syntax for the JavaScript concat() string function:
Plan A for string concatenation: using the + operator. string1 + string2. If you ask a Javascript programmer how to concatenate a string, this is likely the method he'll respond with. It simply uses the + operator to concatenate the right operand with the left operand. This method used to be fairly slow, but has recently been optimized enough ... "Concatenation" is the process of joining things together. In JavaScript, concatenation is most commonly used to join variable values together, or strings with other strings, to form longer constructions.. Somewhat confusingly, the most common JavaScript concatenation operator is +, the same as the addition operator.A few examples: Basic Concatenation String concatenation "+" operator In Javascript, you can do that in a multitude of ways, and one of them is by using the String concatenation operator, or the "+" operator. Now, this "+" operator, if used with numbers, like this: var a = 1 + 2;
Check out my courses and become more creative!https://developedbyed Javascript String Concatenation | Javascript Tutorial For Beginners | Javascript ES6In... The concat method creates a new array consisting of the elements in the object on which it is called, followed in order by, for each argument, the elements of that argument (if the argument is an array) or the argument itself (if the argument is not an array). It does not recurse into nested array arguments. Fundamentals of String Concatenation in JavaScript. Concatenation is the operation that forms the basis of joining two strings. Merging strings is an inevitable aspect of programming. Before getting into "String Concatenation in JavaScript", we need to clear out the basics first. When an interpreter executes the operation, a new string is ...
# 4 Ways to Combine Strings in JavaScript. Here are 4 ways to combine strings in JavaScript. My favorite way is using Template Strings. Why? Because it's more readable, no backslash to escape quotes, no awkward empty space separator, and no more messy plus operators 👏 In the previous post, I have already published the article "How to Loop Through JavaScript Array ". This article tells the ways to loop any Array by using different types of looping statements in JavaScript. Along with accessing an element of an array using looping statements, you can also concatenate, join and sort an array in JavaScript. Concatenate and Interpolate Strings This is probably one of the most widespread and popularized uses of backticks since it will allow us to concatenate and interpolate strings so our code is much cleaner. We are going to look at a few examples comparing the old syntax with the one provided by the backticks. const name = 'Gerardo';
In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string. To concatenate multiple string variables, use the JavaScript concatenation operator. Definition and Usage The concat () method joins two or more strings. concat () does not change the existing strings, but returns a new string.
JavaScript String object has a built-in concat () method. As the name suggests, this method joins or merges two or more strings. The concat () method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings. Appending with the concat () Method I know that I've had to look this up in the past because every language is a little different with its built-in functions. concat () is basically an included function with JavaScript strings that takes in as many arguments as you want and appends — or concatenates — them. Dec 11, 2019 · 2 min read In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. To concatenate a string, you add a plus sign+ between...
Other ways to concatenate string values in JavaScript include: Using the + operator. Using the ES6 template literal. According to the performance test between the assignment operators and the string concat () method, it is strongly recommended that the assignment operators (+, +=) are used instead of the concat () method. Conclusion. The concat ... Code language: JavaScript (javascript) The concat () accepts a varied number of string arguments and returns a new string containing the combined string arguments. If you pass non-string arguments into the concat (), it will convert these arguments to a string before concatenating. There are 3 ways to concatenate strings in JavaScript. In this tutorial, you'll the different ways and the tradeoffs between them. The + Operator. The same + operator you use for adding two numbers can be used to concatenate two strings.. const str = 'Hello' + ' ' + 'World'; str; // 'Hello World'. You can also use +=, where a += b is a shorthand for a = a + b. ...
The concat() function concatenates the string arguments to the calling string and returns a new string. Changes to the original string or the returned string don't affect the other. If the arguments are not of the type string, they are converted to string values before concatenating. Fourth way : concat () method. The concat method accepts the string as an argument and returns the new string by concatenating existing string with an argument. Note: The concat ( ) method doesn't modify or change the existing string. We can also concatenate more than one string by passing multiple arguments to the concat method. JavaScript String concat () Method The JavaScript string concat () method combines two or more strings and returns a new string. This method doesn't make any change in the original string.
Concatenation String And Class In Vuejs Pakainfo
Javascript Merge Array Of Objects By Key Es6 Reactgo
Javascript Array Push Is 945x Faster Than Array Concat
How To Work With Strings In Javascript Digitalocean
How To Work With Strings In Jmeter Blazemeter
How To Work With Strings In Jmeter Blazemeter
How To Concatenate Javascript Files With Codekit Tom Mcfarlin
How To Concatenate Strings Javascript Code Example
Why Does Javascript Handle The Plus And Minus Operators
Sql Server Concatenate Operations With Sql Plus And Sql
How To Concatenate Variables In Javascript The Two Methods
Jquery Html Javascript String Concatenation Onclick Event In
Concatenate String And Field And Update Another Field
How Can We Concatenate 2 Or More Strings In Javascript By
Setting Up The Grunt Configuration To Concatenate Our Css
Javascript Basics String Concatenation With Variables And
Mysql Concat Function W3resource
4 Different Ways To Concat String In Javascipt Tutorials
Javascript Concatenate Strings Es6 String Concat Method
Basic C Program To Concatenate Strings Using Pointer C
0 Response to "22 How To Concatenate In Javascript"
Post a Comment