21 Array Javascript With Key
Now we need to merge the two array of objects into a single array by using id property because id is the same in both array objects. Note: Both arrays should be the same length to get a correct answer. First way. Here we are the using map method and Object.assign method to merge the array of objects by using id. The array.keys() method is used to return a new array iterator which contains the keys for each index in the given input array. Syntax: array.keys() Parameters: This method does not accept any parameters. Return Values: It returns a new array iterator.
How To Merge Two Arrays As Key Value Pair In Javascript
Object.keys() returns an array whose elements are strings corresponding to the enumerable properties found directly upon object. The ordering of the properties is the same as that given by looping over the properties of the object manually.
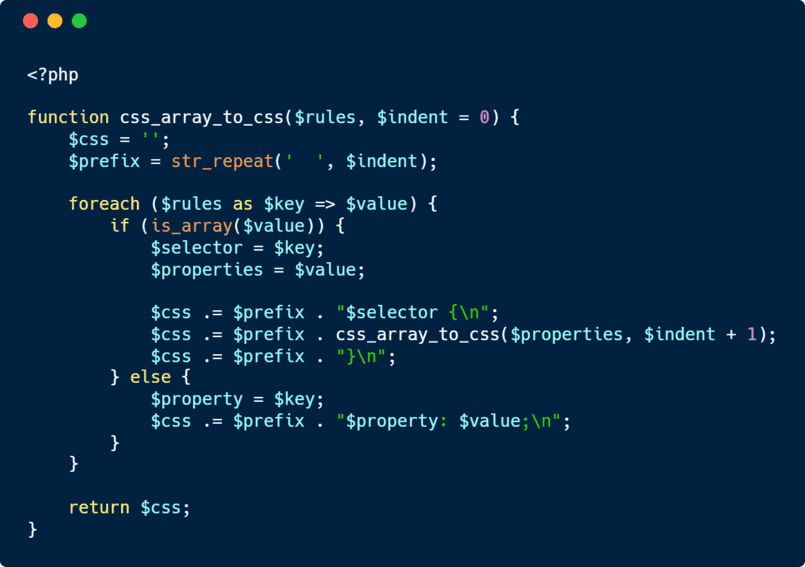
Array javascript with key. An associative array is an array with string keys rather than numeric keys. Does JavaScript support associative arrays? Let's explore the subject through examples and see. In JavaScript, you can't use array literal syntax or the array constructor to initialize an array with elements having ... An associative array can contain string based keys instead of zero or one-based numeric keys in a regular array. If we had the following array defined in Javascript: we could loop through the array and display the index and then the value like so: This would display the following: We were unable to load Disqus. Array javascript with key. Gtmtips Search Datalayer For A Key Value Pair Simo Ahava S 4 Ways To Convert String To Character Array In Javascript Displaying All Elements Of Javascript Array By Looping 5 Ways To Convert Array Of Objects To Object In Javascript How To Remove Duplicates From An Array Of Objects Using ...
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: It works because when you pass the array [1,2,3] as the hash (map/associative-array) key, is being converted to the string '1,2,3' before performing the hash lookup. It should suit your needs as long as you don't need two different arrays of the same value to map to different hash values. var c = [1,2,3] // a [c] evaluates to 'hello' even ... In a language like php this would be considered a multidimensional array with key value pairs, or an array within an array. I'm assuming because you asked about how to loop through a key value array you would want to know how to get an object (key=>value array) like the person object above to have, let's say, more than one person.
Array; Plain objects also support similar methods, but the syntax is a bit different. Object.keys, values, entries. For plain objects, the following methods are available: Object.keys(obj) - returns an array of keys. Object.values(obj) - returns an array of values. Object.entries(obj) - returns an array of [key, value] pairs. Type of Array in JavaScript with Example. There are two types of string array like integer array or float array. 1. Traditional Array. This is a normal array. In this, we declare an array in such a way the indexing will start from 0 itself. 0 will be followed by 1, 2, 3, ….n. Array.prototype.keys () The keys () method returns a new Array Iterator object that contains the keys for each index in the array.
The JavaScript Array keys() method returns a new Array Iterator object that contains the keys for each index in the array. In this article, you will learn about the keys() method of Array with the help of examples. Sep 21, 2020 - JavaScript array keys() method returns an Array Iterator object with the keys of an array. ... The syntax for the Javascript Array keys() method is the following. Jul 27, 2021 - Use Object.entries(obj) to get an array of key/value pairs from obj. Use array methods on that array, e.g. map, to transform these key/value pairs.
Implementing keys () method with holes in between the array. var arr = ['Mon','Tue','Wed',,'Fri']; //A hole is present in-between the elements. Note: It is clear from the above example that Array keys () method does not ignore the holes present as an array element in the given array. It assigns a key value to that empty hole too. We can store key => value array in JavaScript Object using methods discussed below: Method 1: In this method we will use Object to store key => value in JavaScript. Objects, in JavaScript, is the most important data-type and forms the building blocks for modern JavaScript. These objects are quite different from JavaScript's primitive data ... Better readability could be achieved using array.filter, since it creates the array for you and all you have to do is return true or false.No need to create an array, do the comparison and push yourself. In the same way when checking for the values, Object.keys can be used with array.every to iterate through your constraints and see if each of the current item's keys match the constraint.
Working of JavaScript Arrays. In JavaScript, an array is an object. And, the indices of arrays are objects keys. Since arrays are objects, the array elements are stored by reference. Hence, when an array value is copied, any change in the copied array will also reflect in the original array. For example, It takes the object that you want to iterate over as an argument and returns an array containing all properties names (or keys). You can then use any of the array looping methods, such as forEach(), to iterate through the array and retrieve the value of each property. Here is an example: The JavaScript forEach method is one of the several ways to loop through arrays. Each method has different features, and it is up to you, depending on what you're doing, to decide which one to use. In this post, we are going to take a closer look at the JavaScript forEach method. Considering that we have the following array below:
A common problem faced by programers is looping over an enumerable dataset. This data can come in the form of arrays, lists, maps or other objects. In this article we will deal with this problem and learn 4 ways to loop through objects using javascript to retrieve multiple key-value pairs. What are a key and value in an array? Different methods to obtain key-value pairs; Browser Support; Other Related Concepts; What are a key and value in an array? Keys are indexes and values are elements of an associative array. Associative arrays are basically objects in JavaScript where indexes are replaced by user-defined keys. JavaScript Array keys() ... Definition and Usage. The keys() method returns an Array Iterator object with the keys of an array. keys() does not change the original array. Browser Support. The numbers in the table specify the first browser version that fully supports the method: Chrome 38: Edge 12:
Group Array of JavaScript Objects by Key or Property Value Raw group-objects-by-property.md Group array of JavaScript objects by keys. This fork of JamieMason's implementation changes the key parameter to be an array of keys instead of just a single key. This makes it possible to group by multiple properties instead of just one. The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value. Arrays in javascript are not like arrays in other programming language. They are just objects with some extra features that make them feel like an array. It is advised that if we have to store the data in numeric sequence then use array else use objects where ever possible. And to create an associative array with a key value pair it is feasible ...
May 07, 2020 - var myArray = {id1: 100, id2: 200, "tag with spaces": 300}; myArray.id3 = 400; myArray["id4"] = 500; for (var key in myArray) { console.log("key " + key + " has value " + myArray[key]); } Introduction to Associative Array in Javascript. An Associative array is a set of key-value pairs and dynamic objects which the user modifies as needed. When user assigns values to keys with datatype Array, it transforms into an object and loses the attributes and methods of previous data type. Associative array uses string instead of a number ... Create an object from an array of key/value pairs in JavaScript. How to Create a JavaScript Object From Array of Key/Value Pairs? Create an object from an array of key/value pairs in JavaScript. Daniyal Hamid ; 08 May, 2021 ; 3 Topics; 2 min read; Let's suppose you have an array of key/value pairs like the following: ...
How to sort the array of objects by key in JavaScript. javascript1min read. JavaScript has an inbuilt sort method which helps us to sort the array of the objects easily; we don't need to write our own custom sort methods. consider we have an array of objects with name and price properties. To give examples, we will be creating an array of students. We will push some student details in it using javascript array push. We will verify these changes by looping over the array again and printing the result. Basically we will use javascript array get key value pair method. 1. Using an empty JavaScript key value array. var students = []; Jul 23, 2020 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
The call to new Array(number) creates an array with the given length, but without elements. The length property is the array length or, to be precise, its last numeric index plus one. It is auto-adjusted by array methods. If we shorten length manually, the array is truncated. We can use an array as a deque with the following operations: The Object.values() method returns an array of a given object's own enumerable property values, in the same order as that provided by a for...in loop. (The only difference is that a for...in loop enumerates properties in the prototype chain as well.) JavaScript provides many functions that can solve your problem without actually implementing the logic in a general cycle. Let's take a look. Find an object in an array by its values - Array.find. Let's say we want to find a car that is red. We can use the function Array.find. let car = cars.find(car => car.color === "red");
Sum from array values with similar key in JavaScript. Let's say, here is an array that contains some data about the stocks sold and purchased by some company over a period of time. We want to write a function that takes in this data and returns an object of arrays with key as stock name (like 'AAPL', 'MCD') and value as array of two ...
Javascript Take A Flat Array And Create A New Array With
How To Find Unique Objects In An Array In Javascript By
Useful Javascript Array And Object Methods By Robert Cooper
Javascript Es6 Learn Array Keys Array Values And Array
Javascript Fundamental Es6 Syntax Filter An Array Of
Javascript First Key From Associative Array Yin Yang Source
Javascript Sort Array Of Objects Code Example
How To Convert A Nested Php Array To A Css Sass Less Rule
Javascript How To Check If An Array Has Duplicate Values
Vue Js Create New Key Value In A Dynamic Builded Array
4 Ways To Convert String To Character Array In Javascript
D3 Array Input Line Graph Example Stack Overflow
Remove Element From Array Javascript First Last Value
Javascript Merge Array Of Objects By Key Es6 Reactgo
Object Keys Function In Javascript The Complete Guide
Javascript Object Keys Method Get Array Of Keys From
Javascript Pull One Key From Array Of Objects Code Example
5 Ways To Convert Array Of Objects To Object In Javascript
Javascript Array Of Objects Determine If Key Value Exists
Hacks For Creating Javascript Arrays
0 Response to "21 Array Javascript With Key"
Post a Comment