31 How To Assign Value In Javascript
JavaScript Variable: Declare, Assign a Value with Example Variables are used to store values (name = "John") or expressions (sum = x + y). Declare Variables in JavaScript Before using a variable, you first need to declare it. I am trying to assign an ajax response to a variable. How would I go about doing this? @Swoodend is right. You don't want to do this. It's a horrible idea, and you're having a hard time getting it to work because it's the worst way to work with AJAX requests. It'll be easiest for you to use promises, which jQuery has made very convenient.
Different Types Of Javascript Variables And Their Lifecycle
Assign values to variables and add them together: let x = 5; // assign the value 5 to x. let y = 2; // assign the value 2 to y. let z = x + y; // assign the value 7 to z (5 + 2) Try it Yourself ». The assignment operator ( =) assigns a value to a variable.
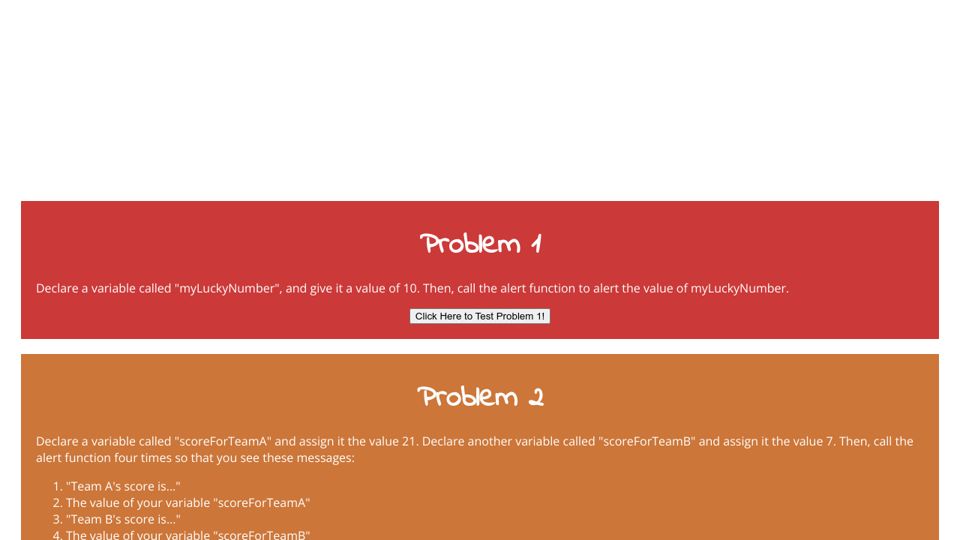
How to assign value in javascript. Note: this is not the recommended way anymore. It's way better to ES6's default parameters. Because quite often, you might not want the default to kick in for ALL falsy values -- I'll explain falsy values in the next section. Most likely, we only want the default value to be set if no value or undefined is passed as the argument. JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... Assignment operators assign values to JavaScript variables. Jul 04, 2017 - JavaScript allocates a memory spot for it. A variable firstName is created and is given a copy of name's value. firstName has its own memory spot and is independent of name . At this moment in the code, firstName also has a value of "Carlos". We then change the value of name to "Carla".
The Object.assign () method was introduced in ES6 that copies all enumerable own properties from one or more source objects to a target object, and returns the target object. The Object.assign () method invokes the getters on the source objects and setters on the target object. It assigns properties only, not copying or defining new properties. In this article, you will learn how JavaScript interacts with HTML. Azure Summit 2021 - Attend Largest Azure Conference Ever x CFP is Open Now: C# Corner Software Architecture Virtual Conference JavaScript includes variables which hold the data value and it can be changed anytime. JavaScript uses reserved keyword var to declare a variable. You can assign a value to a variable using equal to (=) operator when you declare it or before using it. Where does JavaScript store variables?
Object.assign takes two or more parameters. The first is always the target object you want to assign values to. In this instance, it's an empty object. The second parameter is our object defined above. This is the source material we are going to take from to assign to the target object (our first parameter). We cannot assign multiple literal values to a variable i. To overcome this problem, JavaScript provides an array. An array is a special type of variable, which can store multiple values using special syntax. Every value is associated with numeric index starting with 0. For instance, you might create a variable named money and assign the value 2000.50 to it later. For another variable, you can assign a value at the time of initialization as follows. <script type = "text/javascript"> <!-- var name = "Ali"; var money; money = 2000.50; //--> </script>
Sep 16, 2016 - Also note when using ... will update the wrong item value. Safest option as I've mentioned would be to place an id on the input itself then target that. ... Not the answer you're looking for? Browse other questions tagged javascript html function or ask your own ... How to assign value to textbox control present in iframe using javascript TextBox value not assigning to variable in asp textbox value empty check without page reload in javascript. Oct 09, 2017 - Notice we are not assigning a value to these variables just yet. We are only saying they should exist. If you were to look at the value of each variable here, it would be undefined. ... ES2015 is the updated version of JavaScript (also known as ECMAScript). The more lax term for ES2015 is ES6. In ...
May 23, 2017 - The function appears to work based on values observed via alerts, however, I cannot figure out how to assign the value from var newTopic to var smSearchPhrase inside the script. I experimented by placing script inside the function, but that didn't work either. Any assistance is appreciated. JavaScript allows multiple white spaces and line breaks in a variable declaration. Multiple variables can be defined in a single line separated by a comma. JavaScript is a loosely-typed language, so a variable can store any type value. Variable names are case-sensitive. Variable names can contain letters, digits, or the symbols $ and _. 26/6/2020 · The javaScript assignment operator is assigning a value to left hand side operand after executing the right hand side operand operation. This assignment operator is used to reduce the arithmetic logic by simplifying the logic. The rule for assignment operator is the first operand must be any variable followed by equal (=) operator.
Once you declare a variable and assign it a value, your program can manipulate 0:11. what's stored in the variable as it runs, like the score in a game for example. 0:15. You can use variables to do a variety of different things 0:20. with the value stored in or assigned to them. 0:23. 14/1/2019 · To assign your input element's value to a variable, just reverse the above assignment like this: var newValue = newValue; document.getElementById('userVal').value = newValue; Check the following Code Snippet for a practical example on how to assign a variable to your input element's value attribute: After the EXLskills Javascript Fundamentals Course, you'll have all you need to program applications for the browser, servers, and even desktop interfaces! ... Yes, this a 100% free course that you can contribute to on GitHub here! ... Feel free to reach out to us via live chat here! ... Assigning values ...
Jul 20, 2021 - The simple assignment operator (=) is used to assign a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables Javascript has zero indexed integer arrays and also associative arrays. They work in a similar manner but there is a useful way to be able to initialise an associative array with both keys and values in one call. This post will look at the way a zero based integer based array can be initialised and then the ways it can be done with associative arrays in Javascript. Assigning Default Values - Traditional Approach. Traditionally the default value of unpassed parameters were set in the body of the function. The specific parameters were checked for an undefined value, and if the condition satisfied, they were initialized with a default value.
I have searched around for how to Pass javascript variable's value to C# code behind, but have not got no good result. The key is how to assign an object ID to the variable and then its value can be passed to C# code behind. Appreciate if you can share your experience. How to assign javascript value to out-system local variable May 22, 2017 - Javascript will be interpreted in Client's browser and you can not assign it to PHP variable which is interpreted on SERVER . Feasible Solution : You can submit the Javascript value via ajax or through form submit.
Setting the value of the text input element through JavaScript In order to set the value of a text input field element in a form, we can use the following code: oFormObject.elements ["element_name"].value = 'Some Value'; Let us look at an example to illustrate how to set the value of the text input element through javascript. JavaScript JavaScript Reference ... hash host hostname href origin pathname port protocol search assign() reload() replace() DOM Navigator. ... The value property sets or returns the value of the value attribute of a text field. The value property contains the default value OR the value a user types in (or a value set by a script). ... here text1 is javascript value. submitAction_%FormName ( document.%FormName,'#ICSetFieldMOD_TEST_FLUID_PG.RPLAN.' + text1); Assigned this value to Page field MOD_TEST_FLUID_PG.RPLAN using PeopleSoft specific submitAction with action ICSetField. now able to access this from record field change peoplecode.
This page explains how to use the assignment operator to store values in JavaScript variables. May 08, 2019 - In JavaScript, you can reassign values to variables you declared with `let` or `var`. I used to reassign values a lot. But as I got better with JavaScript, I realized you shouldn't reassign values if you can. This is because: 1. You may change external state by accident when you reassign values 2. 13/5/2019 · This method adds the specified attribute to an element, and set it’s specified value. If the attribute already present, then it’s value is set/changed. Syntax: element.setAttribute (attributeName, attributeValue) Parameters: attributeName: This parameter is required. It specifies the name of the attribute to add.
In JavaScript, the Object data type is used to store key value pairs, and like the Array data type, contain many useful methods. These are some useful methods you'll use while working with objects. Object Assign Method. The Object.assign() method is used to . add properties and values to an existing object; make a new copy of an existing object, or I need to assign a -1 value to the Drop and a +1 value to the Add. The result is then multiplied by the value in a Fees field to produce a SubTotal, that is then added into the form's Total field. I suspect I need a Javascript in the SubTotal's Calculation area: var (Drop/Add) = this.get (Drop/Add).value. =IF (Drop/Add = Drop, -1, 0) There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let's show you each of them separately and point the differences. The first method uses document.getElementById ('textboxId').value to get the value of the box:
In JavaScript, the equal sign (=) is an "assignment" operator, not an "equal to" operator. This is different from algebra. The following does not make sense in algebra: ... In JavaScript, however, it makes perfect sense: it assigns the value of x + 5 to x. An array in JavaScript is a type of global object used to store data. Arrays can store multiple values in a single variable, which can condense and organize our code. JavaScript provides many built-in methods to work with arrays, including mutator, accessor, and iteration methods. Dec 24, 2020 - The var statement declares a ... initializing it to a value. ... Variable name. It can be any legal identifier. ... Initial value of the variable. It can be any legal expression. Default value is undefined. Alternatively, the Destructuring Assignment syntax can also be ...
Properties in the target object are overwritten by properties in the sources if they have the same key. Later sources' properties overwrite earlier ones. The Object.assign () method only copies enumerable and own properties from a source object to a target object. Object.assign () Method Among the Object constructor methods, there is a method Object.assign () which is used to copy the values and properties from one or more source objects to a target object. It invokes getters and setters since it uses both [ [Get]] on the source and [ [Set]] on the target. JavaScript Object.assign () Method The Object.assign () method is used to copy the values of all enumerable own properties from one or more source objects to a target object. Objects are assigned and copied by reference. It will return the target object.
1)im calling a javascript fn and passing the actionname as a parameter to the controller actionmethod...in return if the action method runs fine im storing a sucess value in tempdata like tempdata["issucess"]="true" now when the redirect happens i need to get the tempdata value in my javascript fn to perform some other action. Aug 17, 2017 - When we assign and use a reference-type variable, what we write and see is: ... Notice that the value, the address, contained by the variable arr is static. The array in memory is what changes. When we use arr to do something, such as pushing a value, the Javascript engine goes to the location ... Aug 15, 2018 - How to assign outsystem local variable with javascript
4 0 0. Hello, Unfortunately, you cannot assign JS variables to Liquid variables. The reason is Liquid is processed at serverside. What you can do is, create an element with JS and push that JS variable content to that element if you need to show the JS content on a page. 0 Likes. However, you can assign a value dynamically in JavaScript. You can do this by using either innerHMTL or innerText properties. Note: Please do not leave the article "mid way", because I have explained the difference between properties innerHTML and innerText at the end of this article. Using JavaScript innerHTML Property
4 Ways To Swap Variables In Javascript
Quick Tip How To Declare Variables In Javascript Sitepoint
Javascript S Memory Model As Programmers Declaring
Assign Value To Multiple Variables At Once In Javascript
Cannot Assign New Values To Object In Javascript Stack Overflow
Javascript Const Geeksforgeeks
How To Declare A Variable In Javascript With Pictures Wikihow
What Is Ng Change In Angular Js And How Do Assign Value To It
Passing Html Vlaues To Javascript Function Code Example
3 Ways To Set Default Value In Javascript Samanthaming Com
Assign Value To A Two Dimensional Array In Javascript
How To Change A Variable Value In Javascript Code Example
Javascript Strings Properties And Methods
Assign Value To Properties Of Object Without Property Name In
How To Create Or Assign Values To Variables So That A
Javascript Fetch Api How To Save Output To Variable As An
Quick Tip How To Declare Variables In Javascript Sitepoint
How To Get A Grip On Reference Vs Value In Javascript
Assigning The Value Of One Variable To Another Basic Javascript Free Code Camp
Javascript Variables And Assignment Operators
How To Prompt The User For Input In Javascript Dummies
Subtract And Assign In Javascript Tech Funda
Javascript S Memory Model As Programmers Declaring
How To Check If A Variable Is An Array In Javascript
4 Ways To Convert String To Character Array In Javascript
How To Declare A Variable In Javascript With Pictures Wikihow
0 Response to "31 How To Assign Value In Javascript"
Post a Comment