25 How To Create An Object Array In Javascript
Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Oct 05, 2020 - // With that said enjoy the notes ...ted-objects-in-javascript-f02f1bd6387f ... Array objects. ... create a new array that the elements are the length of the elements in array accepted as argument js ... Because you can’t use the dot notation with numbers and usually want to ...
Javascript Array Distinct Ever Wanted To Get Distinct
Jul 20, 2017 - Not the answer you're looking for? Browse other questions tagged javascript arrays object-literal or ask your own question.
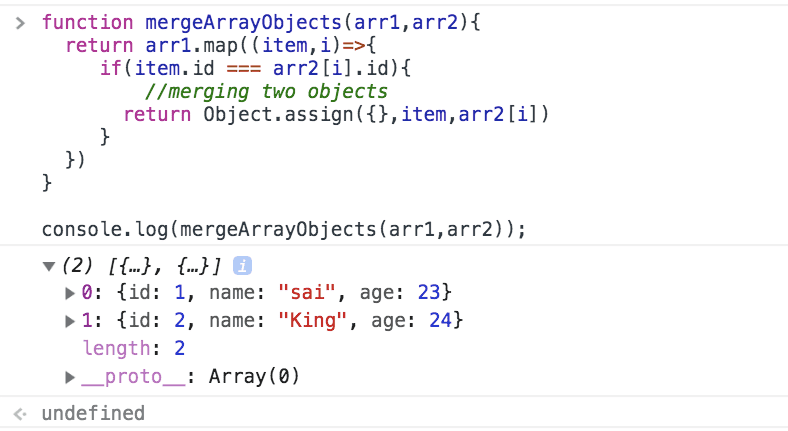
How to create an object array in javascript. JavaScript Array. JavaScript array is an object that represents a collection of similar type of elements. There are 3 ways to construct array in JavaScript. By array literal; By creating instance of Array directly (using new keyword) By using an Array constructor (using new keyword) 1) JavaScript array literal One of the most crucial advantages of an array is that it can contain multiple elements on the contrary with other variables. An element inside an array can be of a different type, such as string, boolean, object, and even other arrays. So, it means that you can create an array having a string in the first position, a number in the second, and ... JavaScript is more than just strings and numbers. JavaScript lets you create objects and arrays. Objects are similar to classes. The objects are given a name, and then you define the object's properties and property values. An array is an object also, except arrays work with a specific number ...
Mar 23, 2017 - Browse other questions tagged javascript arrays ecmascript-6 javascript-objects or ask your own question. ... The full data set for the 2021 Developer Survey now available! Podcast 371: Exploring the magic of instant python refactoring with Sourcery ... Is there any class+subclass combination ... Creating Arrays: The Array Constructor The most popular method for creating arrays is using the array literal syntax, which is very straightforward. However, when you want to dynamically create arrays, the array literal syntax may not always be the best method. An alternative method is using the Array constructor. In this tutorial, you will learn how to create a table from an array of objects in javascript. Having a table with fixed values for heading, rows, and columns only requires use of HTML table, th, tr, and td tags. But what if you want to generate table dynamically when you don't have fixed set of values for heading, rows, and columns? Such thing only happen when you want to pull data from server.
I'm learning javascript coming from a .NET background. I have a question about how to deal with arrays of objects, creating and manipulating doesn't seem as easy/obvious as .NET. Often in .NET code I use structs (c#) or structures (VB.NET) for managing simple value constructs, for example (in VB.NET): The Array object has many properties and methods which help developers to handle arrays easily and efficiently. You can get the value of a property by specifying arrayname.property and the output of a method by specifying arrayname.method(). JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array ();
Summary: in this tutorial, you will learn how to convert an object to an array using Object's methods.. To convert an object to an array you use one of three methods: Object.keys(), Object.values(), and Object.entries().. Note that the Object.keys() method has been available since ECMAScript 2015 or ES6, and the Object.values() and Object.entries() have been available since ECMAScript 2017. The target object is the first argument and is also used as the return value. The following example demonstrates how you can use the Object.assign () method to convert an array to an object: const names = ['Alex', 'Bob', 'Johny', 'Atta']; const obj = Object.assign({}, names); console.log( obj); Take a look at this guide to learn more about the ... Convert object to array in Javascript. Javascript, being a broad and varied-natured programming language consists of an ample amount of features to convert an object into an array. These methods are predefined in the language itself. Let's look at the different ways of converting objects to arrays in javascript.
The literal notation array makes it simple to create arrays in JavaScript. It comprises of two square brackets that wrap optional, comma-separated array elements. Number, string, boolean, null, undefined, object, function, regular expression, and other structures can be any type of array element. Apr 01, 2013 - I have a variable which is an array and I want every element of the array to act as an object by default. To achieve this, I can do something like this in my code. var sample = new Array(); sample... 1 week ago - This can be useful to reveal "hidden" ... because another property has the same name earlier in the prototype chain). Listing accessible properties only can easily be done by removing duplicates in the array. ... JavaScript has a number of predefined objects. In addition, you can create your own ...
1 week ago - Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. Neither the length of a JavaScript array nor the types of its elements are fixed. Since an array's length can change at any time, and data can be stored at non-contiguous locations in the ... Finally with ES2017, it's official now! We have 3 variations to convert an Object to an Array in JavaScript Given two arrays the task is to create an object from them where the first array contains the keys of the object and the second array contains the values of the object. Return null if the array lengths are not the same or if the arrays are empty.
In this tutorial, we will review how to create an object, what object properties and methods are, and how to access, add, delete, modify, and loop through object properties. Creating an Object. An object is a JavaScript data type, just as a number or a string is also a data type. As a data type, an object can be contained in a variable. Example values in the paramVal[i] array: ["Jon", "15", "[email protected]"] I need to create a JavaScript object that places all of the items in the array in the same object. For example {newParamArr[0]:paramVal[0], newParamArr[1]:paramVal[1], ...} The lengths of the two arrays are always the same, but the length of arrays can increase or decrease. Create HTML List From Javascript Array/Object - Simple Examples By W.S. Toh / Tips & Tutorials - HTML & CSS , Tips & Tutorials - Javascript / April 21, 2021 April 21, 2021 Welcome to a tutorial on how to create an HTML list from a Javascript array or object.
Working of JavaScript Arrays. In JavaScript, an array is an object. And, the indices of arrays are objects keys. Since arrays are objects, the array elements are stored by reference. Hence, when an array value is copied, any change in the copied array will also reflect in the original array. For example, May 14, 2020 - JSON stands for JavaScript Object Notation. ... This object represents a car. There can be many types and colors of cars, each object then represents a specific car. Now, most of the time you get data like this from an external service. But sometimes you need to create objects and their arrays ... CodinGame is a challenge-based training platform for programmers where you can play with the hottest programming topics. Solve games, code AI bots, learn from your peers, have fun.
Aug 09, 2019 - image from undraw.co Originally posted on afewminutesofcode To convert an array into an object... Tagged with javascript, beginners, tutorial, webdev. How to Create an Object in JavaScript? In order to create objects, javascript provides a few options using which one can create objects as per one's need. 1. Making Use of Object Initializer Syntax. Object initializer syntax is a list of property names (keys) along with their respective values, enclosed within curly braces ({…}). Creating an array of objects based on another array of objects JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have an array of objects containing data about likes of some users like this − ...
Walk through the solution to the third project in this JS practice session and see how to create a JavaScript array of object literals. ... In the video, the practice file shown says the object must have 4 properties. You only need the 3 properties: name, inventory, and unit_price for each ... The Object.fromEntries() method takes a list of key-value pairs and returns a new object whose properties are given by those entries. The iterable argument is expected to be an object that implements an @@iterator method, that returns an iterator object, that produces a two element array-like object, whose first element is a value that will be used as a property key, and whose second element ... You can also use this same method to merge two arrays into one object, even if one of the arrays doesnt contain objects but only primitive values - however if you do this you need to make sure that at least one of the arrays hold only objects as a primitive will default to its index as the key, so you will get errors if there is a duplicate key.
JavaScript - The Arrays Object. The Array object lets you store multiple values in a single variable. It stores a fixed-size sequential collection of elements of the same type. An array is used to store a collection of data, but it is often more useful to think of an array as a collection of variables of the same type. JSON looks similar to JavaScript’s way of writing arrays and objects, with a few restrictions. All property names have to be surrounded by double quotes, and only simple data expressions are allowed—no function calls, bindings, or anything that involves actual computation. Using Object.fromEntries() In ES2019+, you can achieve that by using the Object.fromEntries() method in the following way: // ES2019+ const obj = Object.fromEntries(pairs); console.log(obj); // {foo: 'bar', baz: 'qux'} Using a for Loop. To create an object from an array of key/value pairs, you can simply use a for loop in the following way:
In this tutorial, we will learn how to create arrays; how they are indexed; how to add, modify, remove, or access items in an array; and how to loop through arrays. Creating an Array. There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword. One of the easiest way to instantiate an object in JavaScript. Constructor is nothing but a function and with help of new keyword, constructor function allows to create multiple objects of same flavor as shown below: Introduction to JavaScript Array Array.from () method In ES5, to create an array from an array-like object, you iterate over all elements and add each of them to an intermediate array as shown in the following example:
When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ... Jul 20, 2021 - The Array() constructor is used to create Array objects. In JavaScript, you can use four different techniques to create new objects. You can create an object literal (with other words a standalone object) by either defining its properties and methods or using the Object.create () method. These objects are the instances of the global Object () object type.
Arrays In Javascript How To Create Arrays In Javascript
Javascript Array Distinct Ever Wanted To Get Distinct
Useful Javascript Array And Object Methods By Robert Cooper
Javascript Array A Complete Guide For Beginners Dataflair
How To Remove A Property From A Javascript Object
How To Create An Object With Dynamic Keys In Javascript
How To Add An Object To An Array In Javascript Geeksforgeeks
Gtmtips Create String From Multiple Object Properties Simo
5 Ways To Convert Array Of Objects To Object In Javascript
Javascript Group An Array Of Objects By Key By Edison
Chapter 17 Objects And Inheritance
5 Ways To Convert Array Of Objects To Object In Javascript
Javascript Take A Flat Array And Create A New Array With
How To Remove Duplicates From An Array Of Objects Using
Let S Get Those Javascript Arrays To Work Fast Gamealchemist
How To Display An Array Of Objects Within An Object Ionic
Converting Object To An Array Samanthaming Com
Data Structures Objects And Arrays Eloquent Javascript
Javascript Lesson 26 Nested Array Object In Javascript
Javascript Array A Complete Guide For Beginners Dataflair
Javascript Array Distinct Ever Wanted To Get Distinct
Create Object With Key Value In Javascript Code Example
Javascript Merge Array Of Objects By Key Es6 Reactgo
0 Response to "25 How To Create An Object Array In Javascript"
Post a Comment