30 Try Catch For Javascript
What is try-catch, throw and finally in JavaScript? These are programming statement which lets you handle the program flow if any error happens. try - We put the test code inside the try statement block. catch - if the test code fails, the catch block helps to handle the error. Apr 12, 2019 - Although there is the ECMAScript standard for JavaScript, it’s important to be aware of your code’s context and the non-standard features it may afford you. A good example is the conditional catch statement: ... This code, which is another way to conditionally trigger a catch statement ...
Javascript Try Catch Amp Error Handling Es6 Tutorial 2019
The try catch statement marks a block of statements to try and specifies a response should an exception be thrown. We can declare try block with a catch or finally and nested try block in JavaScript without any complaints from JavaScript Engine.
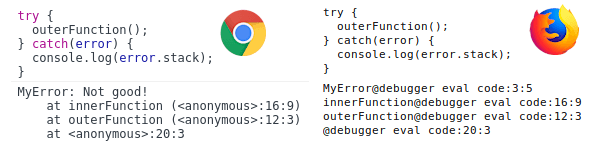
Try catch for javascript. In this tutorial, you will learn ... in JavaScript with the help of examples. The try, catch and finally blocks are used to handle exceptions (a type of an error). Before you learn about them, you need to know about the types of errors in programming. ... Syntax Error: Error in the syntax. For example, if ... Made with ️ ️ in NYC © 2021 Codecademy with ️ ️ in NYC © 2021 Codecademy Dec 18, 2019 - Learn how try catch finally will be executed in JavaScript
React treats error handling ... differently. While try-catch works in a lot of non-vanilla JavaScript applications, it only works for imperative code. Because React components are declarative, try-catch isn't a reliable option. To compensate for this, React implements what are called error ... Learn how to properly handle errors in your JavaScript apps using the try/catch/finally construct as well as the throw statement. JavaScript Errors - Throw and Try to Catch by Shivani Aggarwal March 3, 2021 by Shivani Aggarwal Published: March 3, 2021 Last Updated on March 5, 2021 0 comment 84 views
And we can use a regular try..catch instead of .catch. That's usually (but not always) more convenient. That's usually (but not always) more convenient. But at the top level of the code, when we're outside any async function, we're syntactically unable to use await , so it's a normal practice to add .then/catch to handle the final ... try..catch 는 유효한 코드에서 발생하는 에러만 처리할 수 있습니다. 이런 에러를 '런타임 에러 (runtime error)' 혹은 '예외 (exception)'라고 부릅니다. try..catch 는 동기적으로 동작합니다. setTimeout처럼 '스케줄 된 (scheduled)' 코드에서 발생한 예외는 try..catch 에서 ... A try / catch block is basically used to handle errors in JavaScript. You use this when you don't want an error in your script to break your code. While this might look like something you can easily do with an if statement, try/catch gives you a lot of benefits beyond what an if/else statement can do, some of which you will see below.
The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block. The finally statement lets you execute code, after try and catch, regardless of the result. Jun 07, 2019 - catch: The block that follows this statement will see if the code following the try statement gave an error, and decides what to do with it · With these statements, in JavaScript, you can also add a throw or a finally clause. Let’s see what role they play. Note: Javascript works by reading the code first, then running it. If your code is invalid, for example being syntactically incorrect from having unmatched curly braces somewhere, the Javascript engine won't being able to read it. ... This is a "parsetime error" as the code cannot be parsed properly. try... catch can only catch errors ...
Advanced try/catch/finally in Javascript and Typescript. Take a detailed look at the implementation of a try-catch-finally-block. Tom • Typescript & Javascript, Coding Guides, Learning • 21 05 2021. Error-handling in Javascript & Typescript. A Simple Try Catch. Let's start with the simple try...catch example. This works as expected, ... Learn more about the core concepts around the JavaScript ecosystem. medium . A Developer Introduction To The Cloud. A quick guide on understanding and distinguishing cloud vendors and their numerous products. try-catch in javascript is just as valid and useful as in any other language that implements them. There is one major reason its not used as much in javascript as in other languages. Its the same reason javascript is seen as an ugly scripting language, its the same reason as why people think javascript programmers aren't real programmers:
async function thing() { let x let y try { x = await fooBar() } catch (err) { console.log('handle fooBar error', err) try { y = await otherThing() } catch (e) { console.log('handle otherThing error', e) } } return x } It is possible to have 1 try/catch block, but it becomes increasingly difficult to handle all errors thrown at 1 location. Async/await without try/catch in JavaScript. When async/await was announced it became a game-changer in JavaScript development. It allows writing code in a synchronous way and we don't need to have chained promise handlers: That how this code can be refactored with the async/await syntax: Now it is easier to follow the code. Nov 22, 2017 - Learn how to handle JavaScript Errors with Try, Throw, Catch, & Finally ... Udemy Black Friday Sale — Thousands of Web Development & Software Development courses are on sale for only $10 for a limited time! Full details and course recommendations can be found here.
A catch -block contains statements that specify what to do if an exception is thrown in the try -block. If any statement within the try -block (or in a function called from within the try -block) throws an exception, control is immediately shifted to the catch -block. If no exception is thrown in the try -block, the catch -block is skipped. Jul 20, 2021 - You can nest one or more try statements. If an inner try statement does not have a catch-block, the enclosing try statement's catch-block is used instead. You can also use the try statement to handle JavaScript exceptions. See the JavaScript Guide for more information on JavaScript exceptions. Apr 13, 2012 - There is a provision for try-catch block in javascript. While in java or any other language it is mandatory to have error handling, I don't see anybody using them in javascript for greater extent. ...
Promise.prototype.catch () The catch () method returns a Promise and deals with rejected cases only. It behaves the same as calling Promise.prototype.then (undefined, onRejected) (in fact, calling obj.catch (onRejected) internally calls obj.then (undefined, onRejected)). JavaScript try-catch with javascript tutorial, introduction, javascript oops, application of javascript, loop, variable, data types, operators, javascript if, objects, map, typedarray etc. JavaScript try…catch A try…catch is a commonly used statement in various programming languages. Basically, it is used to handle the error-prone part of the code. It initially tests the code for all possible errors it may contain, then it implements actions to tackle those errors (if occur).
JavaScript try and catch The try statement allows you to define a block of code to be tested for errors while it is being executed. The catch statement allows you to define a block of code to be executed, if an error occurs in the try block. The JavaScript statements try and catch come in pairs: try { {{{{{{{{{{{{ } catch (err) { alert("The engine can't understand this code, it's invalid"); } The JavaScript engine first reads the code, and then runs it. The errors that occur on the reading phase are called "parse-time" errors and are unrecoverable (from inside that code). That's because the engine can't understand the code. Complete tutorial on JavaScript try catch. Find best ways to handle JavaScript error in your code. Learn from JavaScript try catch examples.
Nov 07, 2011 - There are many cases where it makes sense to have no catch block, like in an async function, sometimes. Being forced by the javascript language to create empty catch blocks is clearly pointless. ... A try without a catch clause sends its error to the next higher catch, or the window, if there ... Apr 04, 2020 - This tutorial shows you how to handle exceptions using JavaScript try catch statement. It also introduces you the finally clause for the cleanup code. Sep 07, 2020 - It’s ok not to use .catch at all, if there’s no way to recover from an error. In any case we should have the unhandledrejection event handler (for browsers, and analogs for other environments) to track unhandled errors and inform the user (and probably our server) about them, so that our ...
1 week ago - You can throw exceptions using ... the try...catch statements. ... Just about any object can be thrown in JavaScript. Nevertheless, not all thrown objects are created equal. While it is common to throw numbers or strings as errors, it is frequently more effective to use one of the exception types specifically created for this ... If a script does not contain a try/catch block, then it misses the exception message and the message is thrown up to the next script in the call stack. The call stack is a structure in the JavaScript Engine used to keep track of function calls, i.e., functions calling functions, where each function is considered as a different script. try catch JavaScript Example. To illustrate the try/catch blocks in action, let's use an example. In the example below, we misspelled a JavaScript variable name in our code. let ourVariable = "Test"; console.log(ourVarible); Our code returns: ReferenceError: ourVarible is not defined.
Try and Catch Block: The try statement allows you to check whether a specific block of code contains an error or not. The catch statement allows you to display the error if any are found in the try block. try { Try Block to check for errors. } catch (err) { Catch Block to display errors. javascript try catch Errors are almost inevitable in JavaScript programs. As a JavaScript developer, it is your responsibility to anticipate errors and handle them effectively. This will ultimately help you to create programs which are robust, reliable and efficient. try { (((((((((} catch (e) { console.log ("The engine does not understand this code, it is invalid"); } In the example above, you can see unmatched curly braces. Generally, the engine of JavaScript first reads the code and then runs it. The errors, occurring in the reading phase are known as "parse-time" errors.
How To Avoid Try Catch Statements Nesting Chaining In
Exploring The Exception Error Handling In Javascript Html Page
Don T Use Try Catch In Javascript
Javascript Try Catch How Does Try Catch Work In Javascript
Try Catch In Javascript How To Handle Errors In Js
Try Catch Finally In Javascript Demo
Programminghunk Try Catch Exception Handling
Handling Exceptions In Javascript
Handling Errors In Javascript With Try Catch And Finally
Snowflake Error Handling Procedure And Functions Dwgeek Com
Javascript Multiple Try Catch Code Example
Javascript Errors A Comprehensive Guide To Master Error
Hackerrank Day 3 Try Catch And Finally 10 Days Of
How To Use Try Catch And Settimeout With Async Await
Learn Try And Catch Finally Block Advance Javascript Tutorial Part 55
Javascript Try Catch Block Youtube
Exception Handling In Javascript
Validacion De Campos Try Catch Finally En Javascript Puro
Javascript Try Catch Dev Community
Async Await Not Working With Then And Catch Stack Overflow
Async Await Without Try Catch In Javascript By Dzmitry
Try Catch To Avoid Program Freeze In Javascript
Introduction To Error Handling In Angular 7 Part 1 Angular
The Ultimate Guide To Exception Handling With Javascript Try
Handling Errors In Javascript With Try Catch And Finally
Javascript Try Catch Code Example
Don T Use Try Catch In Javascript
0 Response to "30 Try Catch For Javascript"
Post a Comment