28 Javascript Array Each Jquery
var arr = ['one','two','three','four','five']; $.each(arr, function(index, value){ console.log('The value at arr[' + index + '] is: ' + value); }); #1 Use jQuery each () function to iterate over an Array. Let's have an array object with some values in it. As you see below myArray is an array variable that holds values as some fruits name. Now we want to display all fruit names one by one i.e., using jQuery each function we can iterate over this array variable, and display all the item values.
Objects Arrays Json Using Jquery
Description: Retrieve all the elements contained in the jQuery set, as an array. version added: 1.4.toArray() This method does not accept any arguments..toArray() returns all of the elements in the jQuery set: 1. alert( $( "li").toArray() );
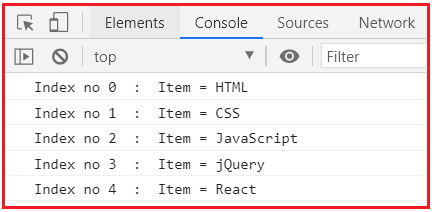
Javascript array each jquery. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. 3 weeks ago - The forEach() method executes a provided function once for each array element. Dec 17, 2015 - In the case of an array, the callback is passed an array index and a corresponding array value each time. (The value can also be accessed through the this keyword, but Javascript will always wrap the this value as an Object even if it is a simple string or number value.)
The JavaScript method toString () converts an array to a string of (comma separated) array values. In JavaScript For Loop, the iteration is done from 0 to the 'one less than the length of the array'. That is the loop will execute until the condition is true. 1. 2. 3. for (var i = 0; i < numbers.length; i++) {. alert (numbers [i]); } The above code will show us each of these 5 numbers in an alert box. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The "JQuery..each()" function however works differently from the $(selector).each() function that works on the DOM element using the selector. But both iterate over a jQuery object. Callback method In the "JQuery..each()" method we're able to pass in an arbitrary array or object in which for each item will have the callback function executed. Aug 04, 2016 - Learn how to loop through elements, arrays and objects with jQuery using the $.each() function, jQuery’s foreach equivalent. jQuery’s foreach equivalent can be very useful for many situations. These… Differences between jQuery and Javascript Both $ ('.text-input') and.getElementsByClassName ('text-input') select all the HTML elements with the given class, and both return array-like objects, but...
14/10/2010 · jQuery.each() jQuery.each() jQuery.each(array, callback) array iteration. jQuery.each(array, function(Integer index, Object value){}); object iteration. jQuery.each(object, function(string propertyName, object propertyValue){}); example: jQuery.each() or $.each() Function This is the simplest way of looping around an array or a JSON array in JavaScript or jQuery. Of course, you can use the for loop but that is so old school.. Here is the syntax of jQuery.each() function:. jQuery.each(array, callback) // or for objects it can be used as jQuery.each(object, callback) Definition and Usage. The every() method returns true if all elements in an array pass a test (provided as a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a false value, every() returns false (and does not check the remaining values); If no false occur, every() returns true
What do you want to do with such an array? jQuery methods are performed on arrays that contain DOM elements, not strings. - Å ime Vidas Oct 13 '11 at 11:18 I looking to use string as a selector to show/hide element with THE SAME classes in different div. Filtering - sort of. Definition and Usage The forEach () method calls a function once for each element in an array, in order. forEach () is not executed for array elements without values. For example, the jQuery factory function $ () returns a jQuery object that has many of the properties of an array (a length, the [] array access operator, etc.), but is not exactly the same as an array and lacks some of an array's built-in methods (such as.pop () and.reverse ()).
In JavaScript for loop iterates through each and every item in an array. Arrays in JavaScript are zero-based, that means array's first item's index number will be 0 and so on as mentioned below in the screenshot. Let's understand for loop The below block of code sets up a loop which contains 3 conditions within. Earlier developers (including me) were using the jQuery Each method -.each (), for doing the process of looping over the array elements. But now all these works can be simply done by the JavaScript's.forEach () method. So I present you with the 7 ways to avoid jQuery Each method with an equivalent JavaScript.forEach () method on your website. Jul 04, 2021 - The jQuery each() method iterates over the arrays, array like objects, objects and DOM elements. Here I provides it's 7 most used ways in development so that you can master this function.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. The.each () is used for looping in jQuery. It is similar to foreach loop in the PHP which loops until it gets data. It works with an Array, Object, and jQuery selector, and you don't have the need to know how many data are available it performs iteration continuously until it getting data that makes it differ from other looping methods. The jQuery each method has two parameters, an array and a callback. The callback method has two values passed to it, the current index and the item value, which is the opposite of the array and Lodash forEach methods. The callback method is executed against each item in the array. $.each (myArray, function (index, value) {
PHP Array Functions PHP String Functions PHP File System Functions PHP Date/Time Functions PHP Calendar Functions PHP MySQLi Functions PHP Filters PHP Error Levels ... The jQuery.each() or $.each() can be used to seamlessly iterate over any collection, whether it is an object or an array. jQuery.each ( array, callback ) Returns: Object. Description: A generic iterator function, which can be used to seamlessly iterate over both objects and arrays. Arrays and array-like objects with a length property (such as a function's arguments object) are iterated by numeric index, from 0 to length-1. Other objects are iterated via their named ... jQuery Arrays: Mapping an Array . The jQuery method $.map() method lets you use a function to project the contents of an array or a map object into a new array, using a function to determine how each item is represented in the result. Example: Mapping an Array using jQuery
For Each JavaScript example files and code download. The below image shows the file structure of the JavaScript For Each example code. It has the JavaScript asset iteration.js to define forEach callbacks to parse types of array collections. On a landing page, I show the code for each type of array iteration using forEach. Download. Conclusion The jQuery.each () method is a static method of the jQuery object. It is a more generalized method that can be used to iterate over any object, array or array-like object. So the syntax is quite simple: jQuery.each (OBJECT,CALLBACK); 1. jQuery.each(OBJECT,CALLBACK); The OBJECT argument is any object, array or array-like object. Purpose of forEach in JavaScript. The forEach method is generally used to loop through the array elements in JavaScript / jQuery and other programming languages. You may use other loops like for loop to iterate through array elements by using length property of the array, however, for each makes it quite easier to iterate and perform some ...
jQuery each is an iteration function. Through this function, we can loop through both the arrays and objects. Arrays will be looped through from 0 to array.length - 1. Objects will be looped through the object's name property. Aug 25, 2019 - Description: Iterate over a jQuery object, executing a function for each matched element · The .each() method is designed to make DOM looping constructs concise and less error-prone. When called it iterates over the DOM elements that are part of the jQuery object. JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array ();
14/10/2020 · javascript array for-each loop October 14, 2020 April 11, 2021 AskAvy javascript forEach() method calls a function once for each element in an array , callback function is not executed for array elements without values. Mar 02, 2020 - In this post, we’ve demonstrated how to use the jQuery.each() function to iterate over DOM elements, arrays and objects. It’s a powerful and time-saving little function that developers should have in their toolkits. And if jQuery isn’t your thing, you might want to look at using JavaScript’... Note To loop over a object array in JSON formatted string, you need to converts it to JavaScript object (with JSON.parse() or $.parseJSON()) before parse it with jQuery $.each(). See this example - JQuery Loop Over JSON String […]
var arr = ['one','two','three','four','five']; $.each(arr, function(index, value){ console.log('The value at arr[' + index + '] is: ' + value); }); Luckily, the $.each() jQuery utility method does exactly what you need it to. In the following demo, we are gonna take a Javascript array and, without any need to convert it to a jQuery array, loop over its values and add them to the document object model (DOM): The.serializeArray () method creates a JavaScript array of objects, ready to be encoded as a JSON string. It operates on a jQuery collection of form s and/or form controls. The controls can be of several types: 1
jQuery provides an object iterator utility called $.each() as well as a jQuery collection iterator: .each().These are not interchangeable. In addition, there are a couple of helpful methods called $.map() and .map() that can shortcut one of our common iteration use cases.. link $.each() $.each() is a generic iterator function for looping over object, arrays, and array-like objects. Read this tutorial and learn several JavaScript and jQuery methods that help you get the difference between two arrays easily. Choose the best one for you. ... This method calls a provided callback function once for each element in the array and constructs a new array containing all the values for which callback returns a value is true. Array ...
Looping Through And Array Object And Creating An Other Object
Jquery Object To Array Working Of Jquery Object To Array
What Is Jquery An Intro For Beginners Course Report
Jquery If Statement Learn The Examples Of Jquery If Statement
Arrow Function Foreach Each Each By Tg Medium
Vue Js Vs Jquery Use Cases And Comparison With Examples
Arrays From Javascript Jquery Interactive Front End Web
Jquery Push Value To Array Code Example
Jquery Each Method With Examples Dot Net Tutorials
In Depth Jquery Each Loop Function Usage With Example 5
Work With Javascript For Loop Foreach And Jquery Each
How To Select All Lt Div Gt Elements On A Page Using Javascript
Looping Over Javascript Array Of Arrays With Data For Loop
How To Convert Comma Separated String Into An Array In Javascript
Create A Select With Jquery Receiving Array From Ajax It Qna
Jquery Vs Javascript Know Their Differences And Similarities
In Depth Jquery Each Loop Function Usage With Example 5
Check If Value Exists In Array Jquery And Javascript
Looping Javascript Arrays Using For Foreach Amp More
Using Jquery Each Function To Loop Through Arrays Objects
How To Show All Data In Array Using Jquery Magento 2
Jquery Map Function Javatpoint
5 Jquery Each Function Examples Sitepoint
Check If Value Exists Jquery In Array Jquery Inarray
Cis 133 Mashup Javascript Jquery And Xml Chapter 3 Building
0 Response to "28 Javascript Array Each Jquery"
Post a Comment