26 Javascript Table Object Properties
Sets or returns which inner-borders (between the cells) that should be displayed in a table: summary: Not supported in HTML5. Sets or returns a description of the data in a table: tFoot: Returns a reference to the <tfoot> element of a table: tHead: Returns a reference to the <thead> element of a table: width: Not supported in HTML5. Use style.width instead. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Traversing An Html Table With Javascript And Dom Interfaces
JavaScript Properties Properties are the values associated with a JavaScript object. A JavaScript object is a collection of unordered properties. Properties can usually be changed, added, and deleted, but some are read only.
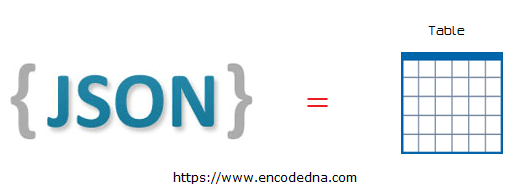
Javascript table object properties. Window object properties. Each object has its own properties, the top-level objectWindowIs the parent of all other child objects, which can appear on each page and can be used multiple times in a single JS application. The following table lists someWindowObject common properties: 3/11/2016 · The Object.assign() method only copies enumerable and own properties from a source object to a target object." So, in your first attempt: el.style = { height: el.offsetHeight + 500 + 'px' } You are attempting to replace the entire object, which fails, but Object.assign just copies properties… To detect all the property values of object without knowing the key can be done in a number of ways depending on browsers. The majority of browsers support ECMAScript 5 (ES5). Let’s see what methods can be used for getting the property value based on different specifications.
The HTMLTableElement interface provides special properties and methods (beyond the regular HTMLElement object interface it also has available to it by inheritance) for manipulating the layout and presentation of tables in an HTML document. JavaScript object is a collection of properties, and a property is an association between a name (or key) and a value. And we as developers use it excessively. In the initial days of my programming career, I found it difficult to work with the object manipulation. JavaScript | Object Properties. Object properties are defined as a simple association between name and value. All properties have a name and value is one of the attributes linked with the property, which defines the access granted to the property. Properties refer to the collection of values which are associated with the JavaScript object.
Table Object Properties Created: February 17th, 2009 The Table object of the DOM supports a handful of unique properties and methods to help you easily perform tasks like dynamically generate a table or certain rows/columns. This is on top of the standard DOM Element properties/ methods the Table object has access to. Following are the methods of table object −. Method. Explanation. createCaption () It generates an empty <caption> element and adds it to the table. createTFoot () It generates an empty <tfoot> element and adds it to the table. createTHead () It generates an empty <thead> element and adds it to the table. Summary. JavaScript provides several ways to check if a property exists in an object. You can choose one of the following methods to check the presence of a property: hasOwnProperty () method. in operator. Comparison with undefined.
Whereas the original Excel.Table object is an API object, the toJSON method returns a plain JavaScript object (typed as Excel.Interfaces.TableData) that contains shallow copies of any loaded child properties from the original object. The Object.getOwnPropertyNames () method returns an array of all properties (including non-enumerable properties except for those which use Symbol) found directly in a given object. One can think of an object as an associative array (a.k.a. map, dictionary, hash, lookup table). The keys in this array are the names of the object's properties. I found this on MDN . https://developer.mozilla /en-US/docs/Web/JavaScript/Reference/Operators/Property_accessors. In JS arrays are associative arrays and objects are also the same.
31/1/2019 · Last Updated : 31 Jan, 2019. The Table object is used for representing an HTML <table> element. It can be used to create and access a table. Syntax: To access table element.: document.getElementById ("id"); To create a table object: document.createElement ("TABLE"); Below program illustrates the Table Object : @David Caunt: Thanks :-) Unfortunately, the accepted answer would still fall foul of the DontEnum bug and you never know what JSON object might have a string like "valueOf" or "constructor" as one of its keys. Welcome to a quick tutorial and examples on how to add properties to a Javascript object. Have you tried using the "usual" array push and concat on objects in Javascript? Just to find out that they don't work? Well, arrays and objects are 2 different stories in Javascript.
Some common solutions to display JavaScript objects are: Displaying the Object Properties by name Displaying the Object Properties in a Loop Displaying the Object using Object.values () JavaScript provides a bunch of good ways to access object properties. The dot property accessor syntax object.property works nicely when you know the variable ahead of time. When the property name is dynamic or is not a valid identifier, a better alternative is square brackets property accessor: object [propertyName]. Object.keys returns enumerable properties. For iterating over simple objects, this is usually sufficient. If you have something with non-enumerable properties that you need to work with, you may use Object.getOwnPropertyNames in place of Object.keys. ECMAScript 2015+ (A.K.A. ES6) Arrays are easier to iterate with ECMAScript 2015.
The attributes collection is iterable and has all the attributes of the element (standard and non-standard) as objects with name and value properties. Property-attribute synchronization. When a standard attribute changes, the corresponding property is auto-updated, and (with some exceptions) vice versa. Since the objects in JavaScript can inherit properties from their prototypes, the fo...in statement will loop through those properties as well. To avoid iterating over prototype properties while looping an object, you need to explicitly check if the property belongs to the object by using the hasOwnProperty () method: Here's a very common task: iterating over an object properties, in JavaScript Published Nov 02, 2019 , Last Updated Apr 05, 2020 If you have an object, you can't just iterate it using map() , forEach() or a for..of loop.
The this Keyword. In a function definition, this refers to the "owner" of the function. In the example above, this is the person object that "owns" the fullName function. In other words, this.firstName means the firstName property of this object. Read more about the this keyword at JS this Keyword. JavaScript object is a non-primitive data-type that allows you to store multiple collections of data. Note: If you are familiar with other programming languages, JavaScript objects are a bit different. You do not need to create classes in order to create objects. Here is an example of a JavaScript object. function generate_table {// get the reference for the body var body = document. getElementsByTagName ("body") [0]; // creates a <table> element and a <tbody> element var tbl = document. createElement ("table"); var tblBody = document. createElement ("tbody"); // creating all cells for (var i = 0; i < 2; i ++) {// creates a table row var row = document. createElement ("tr"); for (var j = 0; j < 2; j ++) {// Create a …
Objects in JavaScript, similar to other programming languages, can be compared to real-life objects. In JavaScript, an object is a standalone entity with properties and methods. Consider the example of a cup of tea, where a cup is an object with properties. We can clearly say that the cup has a color, design, weight, material, etc. I have a javascript object, and I want to recursively search it to find any properties that contain a specific value. ... To get an array of keys to objects where property col3 equals to "C": Object.keys(table).filter(function(row) { return table[row].col3==='C'; }); This would return ['row1', 'row3']. To get a new object of rows where property ... The hasOwnProperty() method returns a boolean value that indicates if the object has the specified property as its own property or not. If the object contains the "key" property, a function is created. This would return true if it could find no keys in the loop, meaning the object is empty. If any key is found, the loop breaks returning false.
The method Object.getOwnPropertySymbols () returns an array of Symbols and lets you find Symbol properties on a given object. Note that every object is initialized with no own Symbol properties, so that this array will be empty unless you've set Symbol properties on the object. The static method Object.defineProperty () defines a new property directly on an object, or modifies an existing property on an object, and returns the object.
20 Useful Javascript Data Table Libraries Bashooka
How To Get All The Td Values Of A Table In A Javascript
Programmers Sample Guide Dynamically Generate Html Table
Pdf Working With Getelementsbytagname In Javascript
Traversing An Html Table With Javascript And Dom Interfaces
Using The Javascript Tool Ringcentral Engage Voice
Better Visualization Of Console Data With Tables
Table 6 1 From Pro Node Js For Developers Semantic Scholar
Javascript Hash Table Associative Array Hashing In Js
How To Use Object Destructuring In Javascript
Data Binding Revolutions With Object Observe Html5 Rocks
How Can I Display A Javascript Object Stack Overflow
Back To The Basics How To Generate A Table With Javascript
Html Table Basics Learn Web Development Mdn
Better Visualization Of Console Data With Tables
Build A Dynamic Table With Sorting Html Css Amp Javascript Frontend Mini Projects Dylan Israel
Jquery Plugin To Render Tables From Json Or Js Objects
Javascript How To Add A Row To An Html Table In Js With Source Code
The Document Object Model Eloquent Javascript
Back To The Basics How To Generate A Table With Javascript
Es6 Map Vs Object What And When By Maya Shavin
Add Or Remove Table Rows Dynamically In Angularjs
Build A Realtime Table With Datatables
Dynamically Add Remove Rows In Html Table Using Javascript
0 Response to "26 Javascript Table Object Properties"
Post a Comment