24 How To Split String Using Javascript
To split a string in JavaScript, you can use the split () method. The split () method splits a string into an array of substrings and returns the new array. const str = `This is an example string` const tokens = str.split(' ') console.log( tokens) If an empty string ( '') is passed as the separator, the string is split between each character. The split() method splits a string into an array of substrings, and returns the new array. If an empty string ("") is used as the separator, the string is split between each character. The split() method does not change the original string.
Javascript String Split Method Split A String Into An
Dec 02, 2017 - Earn 10 reputation (not counting the association bonus) in order to answer this question. The reputation requirement helps protect this question from spam and non-answer activity. Not the answer you're looking for? Browse other questions tagged javascript string-split or ask your own question.
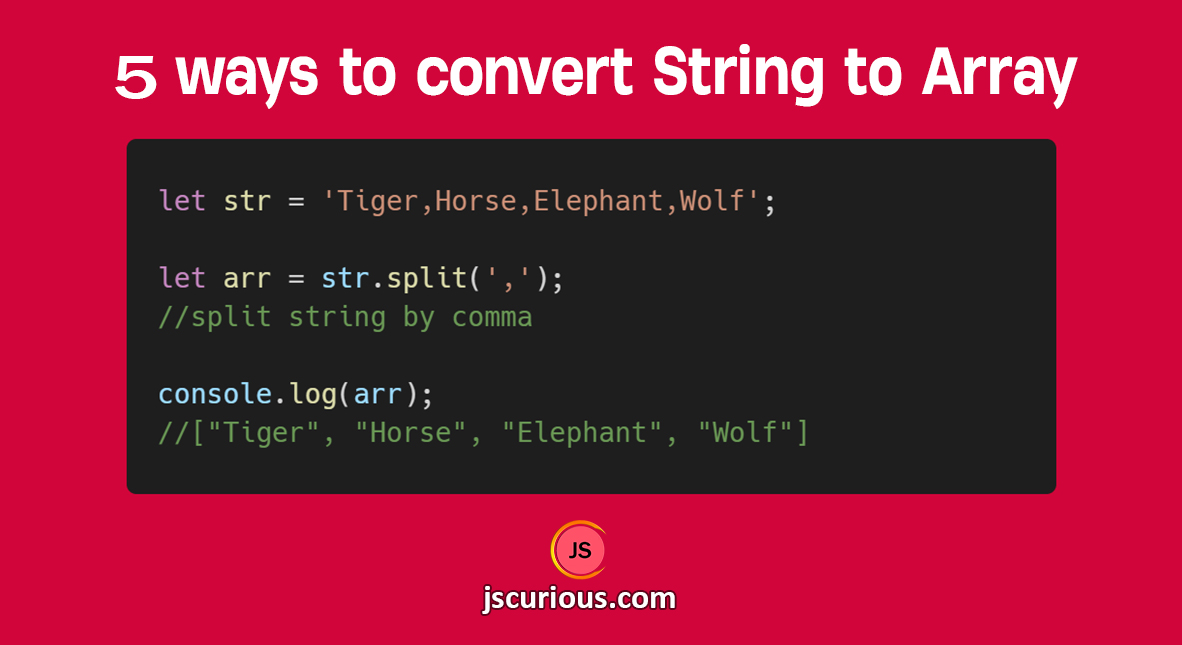
How to split string using javascript. Jul 26, 2021 - Given a statement which contains the string and separators, the task is to split the string into substring. String split() Method: The str.split() function is used to split the given string into array of strings by separating it into substrings using a specified separator provided in the argument. Apr 28, 2021 - That's all you need to know to split() strings with the best of 'em! If this article was helpful, tweet it. Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started The split () Method in JavaScript The split () method splits (divides) a string into two or more substrings depending on a splitter (or divider). The splitter can be a single character, another string, or a regular expression. After splitting the string into multiple substrings, the split () method puts them in an array and returns it.
Using split () When the string is empty, split () returns an array containing one empty string, rather than an empty array. If the string and separator are both empty strings, an empty array is returned. const myString = '' const splits = myString.split() console.log( splits) Copy to Clipboard. The following example defines a function that ... This method splits a String object into an array of strings by separating the string into substrings. ... separator − Specifies the character to use for separating the string. If separator is omitted, the array returned contains one element consisting of the entire string. Properties of a string include the length, prototype and constructor properties. The string object has a number of methods that can be applied to it to allow for manipulation of the string or string object. String methods include the match (), replace (), search (), slice () and split () methods.
Sep 21, 2020 - Javascript string split() is an inbuilt function that splits the String object into an array of strings by separating a string into substrings, using a specified separator string to determine where to make each split. The string split() function is used to split the given string into an array ... The JavaScript split() method is used to split a string using a specific separator string, for example, comma (,), space, etc. However, if the separator is an empty string (""), the string will be converted to an array of characters, as demonstrated in the following example: JavaScript String Split Example Using Split() Method. November 24, 2019 JsTutorials Team javascript. In this tutorial, I am going to explain How To Split String in JavaScript.The JavaScript have a split() method to break the string into the array.We will create different scenario to split string into array using JavaScript.
From this post we shall learn about how to split a string into an array of sub string in JavaScript and will also learn different ways of splitting string. JavaScript's split () Method When the split (delimiter, limit) method is used on a string, it returns an array of substrings, and uses the delimiter argument's value as the delimiter. The split () function has split our string into an array of substrings. Now, we can access each string in the JavaScript array object using an index number. For example: fullNameSplit [1]; This code returns: Surname. The split () method returns an array from which we can retrieve individual values. Each array element corresponds with a word in ...
separator: It is used to specifie the character, or the regular expression, to use for splitting the string. If the separator is unspecified then the entire string becomes one single array element. The same also happens when the separator is not present in the string. There are many ways to split a string in Java. The most common way is using the split () method which is used to split a string into an array of sub-strings and returns the new array. 1. Using String.split () ¶. The string split () method breaks a given string around matches of the given regular expression. There are two variants of split ... In JavaScript, split () is a string method that is used to split a string into an array of strings using a specified delimiter. Because the split () method is a method of the String object, it must be invoked through a particular instance of the String class.
Notice that the spaces stay in the resulting array because they were not used as the separator. ... split() is just one of several methods that help developers work with strings, to learn more see How To Index, Split, and Manipulate Strings in JavaScript Sep 13, 2020 - Notice that the spaces stay in the resulting array because they were not used as the separator. ... split() is just one of several methods that help developers work with strings, to learn more see How To Index, Split, and Manipulate Strings in JavaScript Use RegEx to split a string in Javascript (preserving separators) ✂ Feb 1, 20211 min read Use the Javascript split () function with a Positive Lookbehind Regular Expression to split a string while preserving the separators in the sub-strings: split(/(?<= [<separator characters go here>])/)
From the Mozilla Developer Network for function split (): The split () method returns the new array. When found, separator is removed from the string and the substrings are returned in an array. If separator is not found or is omitted, the array contains one element consisting of the entire string. Split Method in Javascript The Split method is used to split a string into an array of strings and breaking at a specified delimiter string or regular expression. Use the JavaScript String split () to divide a string into an array of substrings by a separator. Use the second parameter (limit) to return a limited number of splits. Was this tutorial helpful ?
In this tutorial, you will learn how to split string by comma, index, slash, newline, hyphen, etc, in javascript. You can use the javascript split () method to split or break a string into parts. Different Ways to Split String In JavaScript You can use split () method to many ways to break/split a string into parts. I have a JavaScript string of arbitrary length, and I need to split it up like cordwood, into equal lengths, and preserve any remainder. With all the great advances in the language of late, it seems like there'd be a String method for that already, doesn't there? We can split a string on a given separator and get back an array of substrings. A string is a data structure that represents a sequence of characters, and an array is a data structure that contains multiple values. And did you know – a string can be broken apart into an array of multiple strings using the split method. Let's see how that works with some examples.
Split function in JavaScript is used to split a string. So it splits the string into the array of substring and after splitting it will give us newel formed array. In other words, we can say that the split function is used to split the string and we must pass a separator into the argument. We have some note and tip to use split method in ... Aug 10, 2017 - Tutorial on JavaScript split string. Learn to use JavaScript string split from code examples of JavaScript split string. Master split javascript now! A split string example. Following is a split string javascript example. In this example we will use a space as separator without limit parameter. The alert shows broken string after using split method. See example by clicking the link below: Experience this example online
The JavaScript split () method splits a string object to an array of strings. This is by breaking up the string into substrings. the split method performs the following. To split a string into an array of substrings. As the name implies, the split () method in JavaScript splits the string into the array of substrings, puts these substrings into an array, and returns the new array. It does not change the original string. When the string is empty, rather than returning an empty array, the split () method returns the array with an empty string. slice () extracts a part of a string and returns the extracted part in a new string. The method takes 2 parameters: the start position, and the end position (end not included). This example slices out a portion of a string from position 7 to position 12 (13-1): Remember: JavaScript counts positions from zero. First position is 0.
If you want to explode or split a string from a certain character or separator you can use the JavaScript split() method. The following example will show you how to split a string at each blank space. The returned value will be an array, containing the splitted values. The split method in JavaScript The JavaScript split method is used to break a given string into pieces by a specified separator like a period (.), comma, space or a word/letter. The method returns an array of split strings. Syntax of split method split () method just creates an array from an input string and a delimiter string. The join () method returns a string containing the values of all the elements in the array glued together using the string parameter passed to join () You can use split () and join () to emulate replace () method, but It will make your code less clear to understand.
Aug 14, 2017 - By splitting strings you can determine how many words are in a sentence, and use the method as a way to determine people’s first names and last names, for example. ... The JavaScript trim() method removes white space from both ends of a string, but not anywhere in between.
Split String And Display Result Accordingly Using Javascript
Split And Join In Javascript Examples And Use In Array
Javascript String Split Tutorial
You Can Include Delimiters In The Result Of Javascript S
How To Split A String In Javascript
Split String Method In Java How To Split String With Example
4 Ways To Convert String To Character Array In Javascript
Javascript Split String Example How To Split A String Into
Javascript String Methods List With Detailed Examples
How To Manipulate A Part Of String Split Trim Substring
Various Ways To Convert String To Array In Javascript Js
How To Split Strings In C Javatpoint
Javascript String Split Tutorial
String Split Method In Javascript Devconquer
3 Different Ways To Split A String In Typescript Codevscolor
Javascript Split String By Comma Pakainfo
How To Split String Without Using Inbuilt Functions Quora
Javascript Split A String And Convert It Into An Array Of
Javascript Fundamental Es6 Syntax Split A Multiline String
Get Url And Url Parts In Javascript Css Tricks
Javascript Split Examples Dot Net Perls
Pentaho Spoon Cannot Split String In Javascript Step Stack
0 Response to "24 How To Split String Using Javascript"
Post a Comment