21 How To Round Value In Javascript
19/1/2016 · Rounding numbers in Javascript # Rounding is straight forward. We can round to the nearest integer, round down or round up. JavaScript uses three methods to achieve this: Math.round() - rounds to the nearest integer (if the fraction is 0.5 or greater - rounds up) Math.floor() - rounds down; Math.ceil() - rounds up The initial value of Infinity is Number.POSITIVE_INFINITY. The value Infinity (positive infinity) is greater than any other number. This value behaves slightly differently than mathematical infinity; see Number.POSITIVE_INFINITY for details. As defined by the ECMAScript 5 specification, Infinity is read-only (implemented in JavaScript 1.8.5 ...
Reliable Js Rounding Numbers With Tofixed 2 Of A 3 Decimal
The toFixed () method converts a number into a string, rounding to a specified number of decimals. Note: if the desired number of decimals are higher than the actual number, zeros are added to create the desired decimal length.
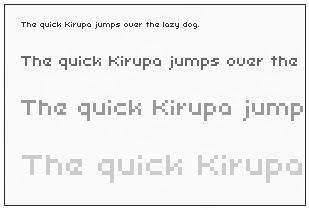
How to round value in javascript. Use the Math.round () Function to Round a Number To2 Decimal Places in JavaScript We take the number and add a very small number, Number.EPSILON, to ensure the number's accurate rounding. We then multiply by number with 100 before rounding to extract only the two digits after the decimal place. JavaScript numbers have the toFixed method that returns a string version of the number rounded to the number of decimal places we passed in as the argument. For instance, we can write: const rounded = Number ((6.6756854).toFixed (1)); console.log (rounded) Then rounded is '6.7' since we passed in 1 to toFixed. The Math.floor() method returns the value of a number rounded downwards to the nearest integer. Example. You can try to run the following code to round down numbers −
The Math.round() method rounds the number to the nearest integer. If decimal part is greater than 0.5, then number is rounded to next integer which has greater absolute value. Absolute value refers to the magnitude value of the number regardless of its sign (for example 12 has greater absolute value than 11, -10 has greater absolute value than -9). JavaScript exercises, practice and solution: Write a JavaScript function to round up an integer value to the next multiple of 5. Now we need to format the above number according to specific decimal places like 123.12 or 123.139.. Using toFixed() method. The toFixed() method formats a number and returns the string representation of a number. Be default the toFixed() method removes the fractional part.. It also accepts the optional argument called digits which means we need to specify the number of digits after the ...
How can I round up a calculated field in adobe acrobat. I have a field in my pdf that calculates FieldNameA/FieldNameB (field name a divided by field name b). How do I round up and remove the decimal places. alana michaels 19/9/2019 · A number can be rounded off to upto 2 decimal places using two different approaches in javascript. Method 1: Using toFixed () method. The Number.toFixed() method takes an integer as an input and returns the the given number as a string in which the fraction is padded to the input integers length. The input integer ranges from 0 to 20. Errors occur when using Math.round and toFixed () because JavaScript's method of storing numbers is not perfect. JavaScript uses a floating-point number format which cannot store certain numbers "exactly". In other words, some numbers are approximations. This applies to all JavaScript calculations, not just rounding numbers.
9/6/2020 · T here is no built-in method to round to a certain number of digits in JavaScript. You might think the Math.round () function would take an argument specifying a desired precision, but it doesn’t.... Math.round() As hinted in the name, Math.round() will return a number rounded up to the nearest integer. const removedDecimal = Math.round(decimal); // returns 5 Using toFixed() The method toFixed() comes in handy when we want to remove only some of the decimal places: Rounding. One of the most used operations when working with numbers is rounding. There are several built-in functions for rounding: Math.floor Rounds down: 3.1 becomes 3, and -1.1 becomes -2. Math.ceil Rounds up: 3.1 becomes 4, and -1.1 becomes -1. Math.round Rounds to the nearest integer: 3.1 becomes 3, 3.6 becomes 4, the middle case: 3.5 ...
In JavaScript, round () is a function that is used to return a number rounded to the nearest integer value. Because the round () function is a static function of the Math object, it must be invoked through the placeholder object called Math. As in the introduction, there are 4 native Javascript Math functions to round off numbers: Math.round () rounds off to the nearest whole number. That is, decimals with less than 0.5 will be rounded down, rounded up if more than or equals to 0.5. Math.ceil () will always round up to the nearest whole number. JavaScript only has the Number type, which can be used as an integer or a double precision float. Because it's a binary representation of a base 10 system, you end up with inaccurate results when you try to do math. 0.1 + 0.2 // returns 0.30000000000000004 ๐ง. Using floats to store monetary values is a bad idea. As you calculate more values ...
JavaScript round() method rounds the number to the nearest integer. Javascript Math Round. Javascript Math.round() is an inbuilt function that returns the value of a number rounded to the nearest integer. If the fractional portion of an argument is more than 0.5, the argument is turned to an integer with the next higher absolute value. The Math.ceil() function in JavaScript is used to round the number passed as parameter to its nearest integer in Upward direction of rounding i.e towards the greater value. Syntax Math.ceil(value) Parameters: This functions accepts a single parameter is the number to be rounded to its nearest integer in upward rounding method. Questions: This question already has an answer here: Round to at most 2 decimal places (only if necessary) 42 answers Answers: NOTE - See Edit 4 if 3 digit precision is important var discount = (price / listprice).toFixed(2); toFixed will round up or down for you depending on the values beyond 2 decimals.
Previous Post Field of view in Javascript using recursive shadow casting Next Post 7 Cool JavaScript Expressions You Should Never Use. One thought on "How to Round Numbers To Arbitrary Values" Kronberg says: Thanks, just want I was looking for.Keep up the good work. I found a lot of nice tips and tricks. The round() method rounds a number to the nearest integer. Note: 2.49 will be rounded down (2), and 2.5 will be rounded up (3). All of the popular programming languages, JavaScript included, provide a built-in rounding function. Strangely, JavaScript's round () function is very no-frills, rounding to the nearest integer only. That doesn't mean that you can't round to the nearest decimal; just that you'll have to put in some work to do so.
26. Little late but, can create a reusable javascript function for this purpose: // Arguments: number to round, number of decimal placesfunction roundNumber(rnum, rlength) { var newnumber = Math.round(rnum * Math.pow(10, rlength)) / Math.pow(10, rlength); return … 14/3/2018 · Rounding Off a number to its nearest integer: To round off a number to its nearest integer, the math.round () function should be implemented in the following way: <script type="text/javascript">. var round =Math.round (5.8); document.write ("Number after rounding : " + round); </script>. It is used to round up the given value to its nearest value. For example, consider a decimal number 4.6 then this number can be rounded up as 5, if the value after the decimal point is less than 5 then it would be round up as 4 and the value more than.5 is round up as 5. JavaScript Number Round Syntax var x= Math.round ("x")
The Math.round () function returns the value of a number rounded to the nearest integer. Minor tweak to this answer: function roundToStep (value, stepParam) { var step = stepParam || 1.0; var inv = 1.0 / step; return Math.round (value * inv) / inv; } roundToStep (2.55, 0.1) = 2.6 roundToStep (2.55, 0.01) = 2.55 roundToStep (2, 0.01) = 2. Share. This method returns the value of a number rounded to the nearest integer. Syntax. Its syntax is as follows −. Math.round( x ) ; Return Value. Returns the value of a number rounded to the nearest integer. Example. Try the following example program.
Angular Format Number 2 Digits Masnurul
Business Intelligence Technology White Paper Custom
How To Round Off In Javascript Up Down To Decimal Places
Solved Acrobat Form Round A Sum Of Two Fields To The Near
Javascript Basic Find The Smallest Round Number That Is Not
Truncate Not Round Off Decimal Numbers In Javascript
Round To 2 Decimal Places Javascript Code Example
How To Round To At Most 2 Decimal Places If Necessary
Javascript Round How To Round The Value In Javascript
Rounding Numbers In Javascript Kirupa Com
How To Round To A Certain Number Of Decimal Places In
Javascript Math Round Precision Benchmark Mswju
Duration Round Off Gantt Asp Net Webforms Syncfusion
Rounding Numbers In Javascript Kirupa Com
2 5 Javascript In Dynaforms Documentation Processmaker
How To Round To A Certain Number Of Decimal Places In
How To Round A Number To 2 Decimal Places In Javascript
How To Format Numbers As Currency Strings Stack Overflow
0 Response to "21 How To Round Value In Javascript"
Post a Comment