23 Javascript String Match Function
Learn how to create Google Sheets Match function alternative using JavaScript in Apps Script. String match () Method Another way to check if a string contains a substring is to use the match () method. It accepts a regular expression as a parameter and searches the string for a match. If it finds the matches, it returns an object, and null if no match is found.
To get a string contains only letters (both uppercase or lowercase) we use a regular expression (/^ [A-Za-z]+$/) which allows only letters. Next the match () method of string object is used to match the said regular expression against the input value. Here is the complete web document.
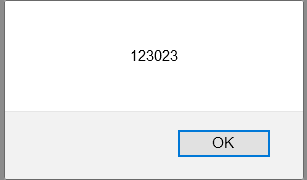
Javascript string match function. Definition and Usage. The match () method searches a string for a match against a regular expression, and returns the matches, as an Array object. Read more about regular expressions in our RegExp Tutorial and our RegExp Object Reference. Note: If the regular expression does not include the g modifier (to perform a global search), the match () ... 9/7/2018 · The string.match() is an inbuilt function in JavaScript used to search a string for a match against any regular expression. If the match is found, then this will return the match as an array. Syntax: string.match(regExp) Parameters: Here the parameter is “regExp” (i.e. regular expression) which will compare with the given string. In this case, we .match() an array with the first match along with the index of the match in the original string, the original string itself, and any matching groups that were used. But say you want to see how many times the word "are" occurs in a string. To do that, just add the global search flag to your regular expression:
Introduction to the JavaScript String search () function. The search () method accepts a regular expression and returns the index of the first match in a string: let index = str.search (regexp); Code language: JavaScript (javascript) In this syntax, the regexp is a regular expression. If you pass a non-RegExp into the method, it will convert ... 4.Using string.match () method: To check if a string contains substring or not, use the Javascript match method. Javascript match method accepts a regular expression as a parameter and if the string contains the given substring it will return an object with details of index, substring, input, and groups. The number from a string in javascript can be extracted into an array of numbers by using the match method. This function takes a regular expression as an argument and extracts the number from the string. Regular expression for extracting a number is (/ (\d+)/). Example 1: This example uses match () function to extract number from string.
Description This method is used to retrieve the matches when matching a string against a regular expression. To get a string contains only letters and numbers (i.e. a-z, A-Z or 0-9) we use a regular expression /^ [0-9a-zA-Z]+$/ which allows only letters and numbers. Next the match () method of string object is used to match the said regular expression against the input value. Here is the complete web document.+ We use String.prototype.match function. The match() method retrieves the matches when matching a string against a regular expression. So we need to create a regular expression which will match all our words but whitespace characters. JavaScript regular expression has a particular part \S which matches a single non-whitespace character.
8/6/2019 · Although the match function doesn't accept string literals as regex patterns, you can use the constructor of the RegExp object and pass that to the String.match function: var re = new RegExp(yyy, 'g'); xxx.match(re); Any flags you need (such as /g) can go into the second parameter. ; str1. match ("number"); // "number" is a string. returns ["number"] str1. match (NaN); // the type of NaN is the number. returns ["NaN"] str1. match (Infinity); // the type of Infinity is the number. returns ["Infinity"] str1. match (+ Infinity); // returns ["Infinity"] str1. match (-Infinity); // returns ["-Infinity"] str2. match (65); // returns ["65"] str2. match (+ 65); // A number with a positive sign. returns ["65"] str3. match (null); // … The JavaScript Match function is one of the String Functions. This JavaScript string match function is used to search for a specified string and return the array of matched substrings. The syntax of the string match function in JavaScript is
In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): Both String#includes() and String#indexOf() are case sensitive. Neither function supports regular expressions. To do case insensitive search, you can use regular expressions and the String#match() function, or you can convert both the string and substring to lower case using the String#toLowerCase() function. The JavaScript string match() method is used to match the string against a regular expression. We can use global search modifier with match() method to get all the match elements otherwise the method return only first match.
In JavaScript, match () is a string method that is used to find matches based on regular expression matching. Because the match () method is a method of the String object, it must be invoked through a particular instance of the String class. Returns -1 if not found. localeCompare (string,position) Compares two strings in the current locale. match (RegExp) Search a string for a match using specified regular expression. Returns a matching array. replace (searchValue, replaceValue) Search specified string value and replace with specified replace Value string and return new string. 19/11/2020 · The Javascript match() method is used for strings only. What it does is that it compares a given string with a regular expression supplied to it to identify matches between them. Once found it returns the matches as an object of type Array or may return a null response if not match is found.
string.replace () description When you want to do a search and replace on a string in JavaScript, the replace () function is the one you want to make use of. This function searches the provided haystack, or string, for one or several substrings that match a given pattern or regular expression. It then replaces those matches with a replacement. JavaScript String match () method Let's take some examples of using the match () method. 1) Using the match () method with the expression that has the global flag The following example shows how to use the match () method with the global flag. In this article. Tests for a match or extracts portions of a text string based on a pattern. Description. The IsMatch function tests whether a text string matches a pattern that can comprise ordinary characters, predefined patterns, or a regular expression.The Match and MatchAll functions return what was matched, including sub-matches.. Use IsMatch to validate what a user has typed in a Text ...
While it does the job well, you must note that the function is actually intended to return the index at which a given substring is found, and -1 when there's no match. Return Value: If match is found, position of the first occurrence in the string is returned. If match is NOT found, -1 is returned. Syntax: str.indexOf(searchStr); Also, JavaScript is really flexible and versatile. Here we've only scratched the surface of all the string functions JavaScript can do and it is constantly evolving. Recommended Articles. This has been a guide to JavaScript String Functions. Here we discussed how to use string function in JavaScript programming with the help of examples. Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec () and test () methods of RegExp, and with the match (), matchAll (), replace (), replaceAll (), search (), and split () methods of String.
search () Searches a string for a specified value, or regular expression, and returns the position of the match. slice () Extracts a part of a string and returns a new string. split () Splits a string into an array of substrings. startsWith () Checks whether a string begins with specified characters. The method str.match returns capturing groups only without flag g. The method str.matchAll always returns capturing groups. If the parentheses have no name, then their contents is available in the match array by its number. Named parentheses are also available in the property groups.
Excel If Function Contains Text Partial Match Computergaga
How Javascript Works Regular Expressions Regexp By
Excel Formula Extract All Partial Matches Exceljet
Excel If Function With Partial Text Match If With Wildcards
Javascript Regex Match Example How To Use Js Replace On A
Beginning To Use Regex In Javascript By Cristina Murillo
Excel If Function With Partial Text Match If With Wildcards
Excel If Function Contains Text Partial Match Computergaga
Javascript Extract Number From String Regex Amp Replace
20 Javascript String Functions String Methods With
Javascript Split How To Split A String Into An Array In Js
How Javascript Works Regular Expressions Regexp By
Practical Javascript Programming Session 6 8
Python Regex Re Match Re Search Re Findall With Example
Everything You Need To Know About Regular Expressions By
Ms Excel How To Use The Match Function Ws
11 Ways To Check For Palindromes In Javascript By Simon
Apache Jmeter User S Manual Functions And Variables
Introduction To Pattern Matching In Ruby Toptal
Vlookup And Index Match Examples In Excel
0 Response to "23 Javascript String Match Function"
Post a Comment