25 Compare 2 Arrays Javascript
There are a variety of JavaScript and jQuery methods that help you get the difference between two arrays. Let's discuss each of them. You can use a single line of code using the filter method of JavaScript Array, which will output those elements of arr1 that are not found in arr2. It can be done in three ways. 18/4/2019 · In Javascript, to compare two arrays we need to check that the length of both arrays should be same, the objects present in it are of the same type and each item in one array is equal to the counterpart in another array. By doing this we can conclude both arrays are the same or not.
How To Sum Two Arrays Data In Javascript Stack Overflow
In this guide, we will discuss how to compare and get matching values like strings or numbers from two Arrays? The result will be the set of values that are available in both arrays. While working in Javascript may need to compare two arrays to find intersection values that are matching and fetch out the matching values.
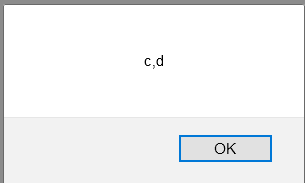
Compare 2 arrays javascript. [1, 2, 1, 2].equals([1, 2, 1, 2]) === true; You may say "But it is much faster to compare strings - no loops..." well, then you should note there ARE loops. First recursive loop that converts Array to string and second, that compares two strings. So this method is faster than use of string. 2 Comparing Arrays in JavaScript. 2.1 Checking if Arrays Contain the Same Values, Regardless of Order. 2.2 Checking if Arrays Contain the Same Values, In The Same Order. 2.3 Finding Values in an Array that Aren't in Another. SHARE: RELATED GUIDES. Feb 26, 2020 - See the Pen JavaScript - Find the difference of two arrays - array-ex- 23 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus · Previous: Write a JavaScript program to compute the union of two arrays. Next: Write a JavaScript function to remove.
If you comparing 2 arrays but values not in same index, then try this. var array1=[1,2,3,4] var array2=[1,4,3,2] var is_equal = array1.length==array2.length && array1.every(function(v,i) { return ($.inArray(v,array2) != -1)}) console.log(is_equal) how to check two array values are equal in javascript; compare two arrays javascript if any elements are equal; check if 2 arrays have same values javascript; react compare two arrays and check if they same elements; javascript compare two arrays and check if they same elements; javascript see if 2 char arrays have the same values // program to compare two arrays function compareArrays(arr1, arr2) { // compare arrays const result = JSON.stringify(arr1) == JSON.stringify(arr2) // if result is true if(result) { console.log('The arrays have the same elements.'); } else { console.log('The arrays have different elements.'); } } const array1 = [1, 3, 5, 8]; const array2 = [1, 3, 5, 8]; compareArrays(array1, array2);
Javascript queries related to "javascript compare two arrays of objects return difference" js array diff between 2 arrays js compare properties two arrays of objects comparing a value between two array objects javascript 27/5/2020 · let arrayDifference = arr1.filter (x => arr2.indexOf (x) === -1); console.log (arrayDifference); Here, arr1 elements are compared in the second array, which are not present in the second array, it’s difference. In this approach, we will compare the elements of the first array with the elements of the second array. Compare two arrays and get those values that did not match JavaScript Javascript Web Development Object Oriented Programming We have two arrays of literals that contain some common values, our job is to write a function that returns an array with all those elements from both arrays that are not common.
Apr 28, 2021 - That’s all about comparing arrays in JavaScript. ... Average rating 5/5. Vote count: 20 Aug 07, 2020 - Arrays are one of the most used data types in any programming language. Learn how to merge two arrays in JavaScript with this short guide. ... Website, name & logo © 2017-2021 30-seconds Individual snippets licensed under CC0-1.0 Powered by Netlify, Next.js & GitHub Jul 25, 2019 - exchange value between 2 items in array javascript
Apr 28, 2021 - This post will discuss how to find the difference between two arrays in JavaScript. The solution should return an array containing all the elements of the first array which are not present in the second array. For example, the difference between arrays [1,2,3,4] and [3,4,5] is [1,2]. Basic approach to compare array and object in javascript. The most basic approach is to convert the whole array or the object to a string then compare if those strings are equal or not. To convert an array or object we will be using JSON.stringify (). In this article, we cover the different methods to compare two arrays and check if they are equal using javascript. In this process we will also understand the pros and cons of various methods used. If you are new to the concept of array equality, each section of the article would be useful.
Apr 03, 2020 - Write a function to return difference between two arrays. ... javascript create variable containing an object that will contain three properties that store the length of each side of the box · convert the following 2 d array into 1 d array in javascript var array3 = array1 === array2 That will compare whether array1 and array2 are the same array object in memory, which is not what you want. In order to do what you want, you'll need to check whether the two arrays have the same length, and that each member in each index is identical. Assuming your array is filled with primitives—numbers and or strings—something like this should do Comparing two arrays in JavaScript using either the loose or strict equality operators ( == or === ) will most often result in . Here's an array, which has number and string values. List.equals method return true if both elements are of same size and both contains same set of elements in exactly same order. To keep an element the test ...
Jan 12, 2021 - New JavaScript and Web Development content every day. Follow to join our +2M monthly readers. Comparing two arrays in JavaScript using either the loose or strict equality operators (== or ===) will most often result in false, even if the two arrays contain the same elements in the same order. Method 1: Brute Force approach Compare each and every item from the first array to each and every item of second array. Loop through array1 and iterate it from beginning to the end. Loop through array2 and iterate it from beginning to the end.
10/5/2020 · The simplest and fastest way to compare two arrays is to convert them to strings by using the JSON.stringify() method and then use the comparison operator to check if both strings are equal: const arr1 = [ ' ' , ' ' , ' ' , ' ' , ' ' ] ; const arr2 = [ ' ' , ' ' , ' ' , ' ' , ' ' ] ; // compare arrays if ( JSON . stringify ( arr1 ) === JSON . stringify ( arr2 ) ) { console . log ( 'Both arrays are equal!' ) ; } else { console . log ( 'Arrays … Mar 14, 2021 - JavaScript: The best way to compare array elements ... JavaScript arrays are a special type of objects, and just like regular objects, comparison of two arrays will return false even when they contain the same elements: JavaScript code to get common elements from two Arrays. By 'get common elements from two arrays', here we mean the mathematical intersection of two arrays. Those elements which are present in both the arrays are referred to as common elements here. There are many ways to get the common elements. Let's see two of them here and their time ...
Array.prototype.equals to Compare Two Arrays in JavaScript JavaScript provides us the capability to add new properties and methods to the existing classes. We can use Array.prototype to add our custom method equals into the Array object. In the below example, we will first check the length of both the arrays and then comparing each item. Thank you for the clarification. It means that you need to perform N * M comparisons, where N and M are lengths of the two arrays. Do it in two nested loops. This is simple is your array keys are consecutive integers and you know the sizes. In Javascript, any array is an associative container with any keys. JavaScript array equality: A smarter way to compare two arrays. Here's how you can find if an array is actually equal or not. Posted on April 28, 2021. Since JavaScript array type is actually a special object type, comparing two arrays directly with === or == operator will always return false: let arrOne = [1, 2, 3]; let arrTwo = [1, 2, 3 ...
To compare two Arrays in JavaScript, you should check that the length of both arrays should be the same, the objects presented in it be the same type, and each item in one array is equivalent to the counterpart in the compared array. This tutorial will show you some ways of comparing two arrays. 1 week ago - The 2 in years[2] is coerced into a string by the JavaScript engine through an implicit toString conversion. As a result, ';2' and '02' would refer to two different slots on the years object, and the following example could be true: ... A JavaScript array's length property and numerical properties ... 4 weeks ago - The every() method tests whether all elements in the array pass the test implemented by the provided function. It returns a Boolean value.
Aug 03, 2020 - let difference = arr1.filter(x => !arr2.includes(x)); Also, we can use the following methods to compare two arrays javascript. Equality comparison: When programming in JavaScript, it is important to understand that arrays and objects are compared by reference rather than value. This means a comparison of two equal-length arrays using the loose equality operator (==) will often result in false ... Dec 31, 2019 - Now we are writing the compare function it helps us to compare above two arrays. ... Step 1: We need to declare the function with two parameters. ... Step 2: We need to Initialize an empty array.
When we sort the two arrays if they contain the same elements their order becomes the same. We can then simply check if both the arrays have the same element present at the same index and return true and false otherwise.When we sort the two arrays if they contain the same elements their order becomes the same. It is possible to compare arrays using the "every" method in javascript. A possible solution is. botType.length === serviceList.length && serviceList.every(item => botType.indexOf(item) > -1) Enter fullscreen mode. Exit fullscreen mode. I started with length arrays comparison, to be sure to have the same items. Arrays are objects in JavaScript, so the triple equals operator === only returns true if the arrays are the same reference.. const a = [1, 2, 3]; const b = [1, 2, 3]; a === a; // true a === b; // false. How do you compare whether two arrays are equal? Equality is a tricky subject: the JavaScript spec defines 4 different ways of checking if two values are "equal", and that doesn't take into ...
Sep 07, 2020 - how to compare elements of two arrays of different objects in javascript then make a new array with the differences · how to compare elements of two arrays in javascript Aug 03, 2020 - how to compare elements of two arrays of different objects in javascript then make a new array with the differences The behavior for performing loose equality using == is as follows:. Loose equality compares two values for equality after converting both values to a common type. After conversions (one or both sides may undergo conversions), the final equality comparison is performed exactly as === performs it.; Loose equality is symmetric: A == B always has identical semantics to B == A for any values of A ...
To properly compare two arrays or objects, we need to check: That they're the same object type (array vs. object). That they have the same number of items. That each item is equal to its counterpart in the other array or object. It parses two arrays a1 and a2 that are to compare. The method returns true if arrays are equal, else returns false. The Arrays class has a list of overloaded equals() method for different primitive types and one for an Object type.. Note: While using the array of objects, don't forget to override the equals() method.
Compute The Set Difference Using Javascript Arrays
Comparing Two Arrays In Javascript Returning Differences
Let S Get Those Javascript Arrays To Work Fast Gamealchemist
How Do I Merge Two Arrays In Javascript 30 Seconds Of Code
Javascript Algorithm Find Difference Between Two Arrays
Javascript Array Find The Unique Elements From Two Arrays
Javascript Array Difference Example Get Difference Between
4 Ways To Convert String To Character Array In Javascript
How To Compare Two Javascript Array Objects Using Jquery
Find Common Values From Two Arrays In Javascript Code Premix
Algorithms 101 Find The Difference Between Two Arrays In
How To Compare Two Arrays In Javascript
How To Remove Duplicates From An Array Of Objects Using
Union Of Two Arrays In Javascript T4tutorials Com
Comparing Two Arrays Get The One Which Is Not Present In
How To Get The Difference Between Two Arrays As A Distinct
Comparison Of Two Arrays Using Javascript By Sai Gowtham
Javascript Merge Arrays 3 Important Ways To Merge Arrays In
How Do I Compare Two Arrays In Javascript 30 Seconds Of Code
How To Get The Difference Between Two Arrays In Javascript
How To Remove Array Duplicates In Es6 By Samantha Ming
Comparison Of Two Arrays Using Javascript By Sai Gowtham
Dynamic Array In Javascript Using An Array Literal And
Compare A Generator To Using Array Map And Filter Egghead Io
0 Response to "25 Compare 2 Arrays Javascript"
Post a Comment