21 How To Draw With Javascript
For w=1000, h=1000, stepSize=20. Your function would draw 2500 lines where only 100 lines would suffice. For w=800, h=400, stepSize=20. Your function would draw 800 lines where only 60 lines would suffice. In addition the canvas resize you should look to put that outside of that function and do it only once at the beginning of the program! This is a simple video to show you how to utilize the javascript class found at (http://www.c-point /javascript_vector_draw.htm) to draw 2D shapes, lines,...
Javascript Draw On Html5 Canvas Element Video Packt
You're on the right track with beginShape () / curveVertex () / endShape (), it should (in theory) be just a matter of iterating through each landmark position and passing the x,y coordinates to draw. Currently you seem to pass the landmark directly: curveVertex (landmarkPositions); while curveVertex () requires x, y coordinates.
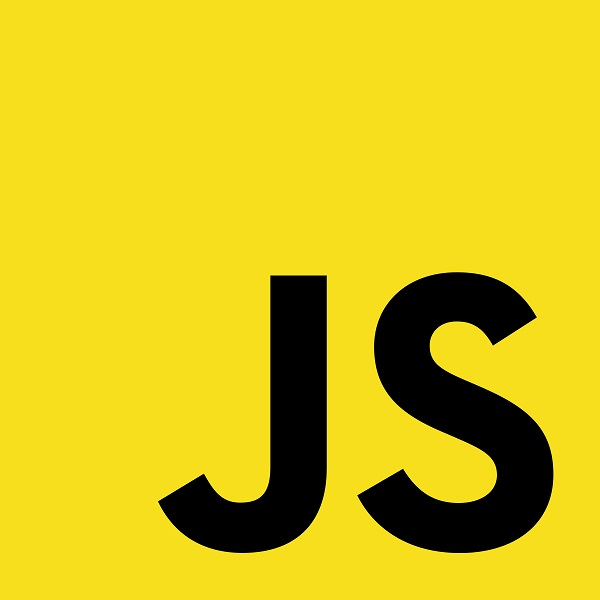
How to draw with javascript. 1 week ago - Note: Angles in the arc function are measured in radians, not degrees. To convert degrees to radians you can use the following JavaScript expression: radians = (Math.PI/180)*degrees. The following example is a little more complex than the ones we've seen above. It draws 12 different arcs all with ... Canvas allows drawing and pixel manipulation using JavaScript API. This drawing has to be performed on canvas' context. Think of context as a page to paint and API as available tools (or brushes). The context can either be "2d" or "webgl"(3d). For the purpose of this introduction we will always assume "2d" context. In this article, we looked at how to draw on an image with JavaScript. Implementing a brush to draw on images by using the <canvas> HTML element is not complex. However, it inevitably involves boilerplate code. Specifically, when implementing all the minor options such a feature should offer to the end-users.
To calculate the coordinate of where to draw the new circle, where you can use some trigonometry to calculate the coordinate for the center of the next circle. To use JavaScript's Math.cos and Math.sin functions though, you need to first convert degrees to radians. The following two functions do both these tasks for you. Drawing an image in canvas using in JavaScript. Javascript Web Development Object Oriented Programming. Following is the code for drawing an image in canvas using JavaScript −. Steps for drawing a line in JavaScript. To draw a line on a canvas, you use the following steps: First, create a new line by calling the beginPath() method. Second, move the drawing cursor to the point (x,y) without drawing a line by calling the moveTo(x, y). Finally, draw a line from the previous point to the point (x,y) by calling the lineTo(x,y) method.
In this energetic video, we create a drawing app similar to MS PAINT… We utilize the power of HTML5 & JavaScript's canvas to allow the user to draw to the ca... Apr 26, 2021 - You'll also see that we are chaining ... in JavaScript, and it is a good technique if you want to make multiple variables all equal to the same value. We wanted to make the canvas width and height easily accessible in the width/height variables, as they are useful values to have available for later (for example, if you want to draw something ... Blank paper. First, we need something to draw on. Let's start with a web-page with some text, and an area beside it for sketching. We can use the HTML 5 canvas tag to create our sketchpad area. Edit the HTML for your page, add the canvas tag with an id name of your choice, and change the dimensions to fit your layout.
Drawing with JavaScript and Coding with Chrome illustrates how you can draw colorful, animated, abstract pictures using a bit of JavaScript and the Coding wi... Feb 26, 2020 - JavaScript exercises, practice and solution: Write a JavaScript program to draw a circle. JavaScript libraries for drawing graphs. Low-level libraries offering graph-oriented modeling primitives. In fact, some of them have been used to build the JavaScript modeling libraries listed above. D3. D3.js is a JavaScript library for manipulating documents based on data. Right now, I would say is the most popular library of its kind.
p5.js | draw () Function. The draw () function is called after setup () function. The draw () function is used to executes the code inside the block until the program is stopped or noLoop () is called. If the program does not contain noLoop () function within setup () function then draw () function will still be executed once before stopping it. By default, JavaScript tries to use the least amount of memory possible. To achieve that, it doesn't copy the objects that are passed as functions arguments or even stored into arrays. For our ... The “draw” helper method. We will use the bracket syntax for a helper method below called draw. The “draw” helper method. This method takes three parameters: radius, or how far the pen is from the center. the angle relative to the star’s three o’clock position. an action, which is either moveTo or lineTo.
There are also effects, such as ... an image with JavaScript (blurring or distorting it), that can be realistically handled only by a pixel-based approach. In some cases, you may want to combine several of these techniques. For example, you might draw a graph with SVG ... 1 week ago - The examples provided should give you some clear ideas about what you can do with canvas, and will provide code snippets that may get you started in building your own content. <canvas> is an HTML element which can be used to draw graphics via scripting (usually JavaScript). 6/4/2014 · You can start with this: <!DOCTYPE html> <html> <head> <title>EaselJS example</title> <script type="text/javascript" src="http://cdnjs.cloudflare /ajax/libs/EaselJS/0.7.1/easeljs.min.js"></script> <script> var canvas, stage; var drawingCanvas; var oldPt; var oldMidPt; var color; var stroke; var index; function init() { if …
HTML5 Drawing Board is possible to create with a Javascript Library named Literally Canvas. Like deviantArt, you can add a custom hand drawing field. Practically, this is the project, which is used in deviantArt Muro, but we are giving you a very easy example with Literally Canvas for creation of your own HTML5 Drawing Board. Check out demos Read introduction Download version 4.6.0 Learn how to use the JavaScript language and the ProcessingJS library to create fun drawings and animations. Our mission is to provide a free, world-class education to anyone, anywhere. Khan Academy is a 501(c)(3) nonprofit organization.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. You can create responsive charts with JSCharting through a couple simple steps: Define a <div> tag in the HTML file with a unique id. Provide this id, data, and any other options when calling JSC.Chart () in the JavaScript file. Even if you draw the content of an SVG inside a canvas you will still have issue with zomming - Temani Afif Aug 14 '19 at 21:27 from the link above : SVG does not depend on the resolution, means it is resolution independent.
This post shows how to implement simple JavaScript drawing. After page is loaded, JavaScript will generate HTML table and attach onMouseDown and onMouseOver event handlers to the each table cell. When user clicks the left mouse button and move mouse pointer over table cell, table cell will change background-color property to the selected color. May 25, 2016 - A super short version, here, without position:absolute in vanilla JavaScript. The main idea is to move the canvas' context to the right coordinates and draw a line. Uncomment click handler and comment mousedown & mousemove handlers below to get a feel for how it is working. 26/2/2020 · JavaScript drawing [6 exercises with solution] [An editor is available at the bottom of the page to write and execute the scripts.] 1. Write a JavaScript program to draw the following rectangular shape. Go to the editor Expected Output: Click me to see the solution. 2. Write a JavaScript program to draw a circle. Go to the editor Expected Output:
It turns out it's actually fairly simple and easy to implement with JavaScript! Live code example on Codepen permalink. We'll be building this cool drawing app. Try it out and have a peek at the code. See the Pen JavaScript mouse drawing on the canvas 👨🎨 by Chris Bongers (@rebelchris) on CodePen. HTML Structure permalink Step 3: Draw on the Canvas. Finally, you can draw on the canvas. Set the fill style of the drawing object to the color red: ctx.fillStyle = "#FF0000"; The fillStyle property can be a CSS color, a gradient, or a pattern. The default fillStyle is black. The fillRect ( x,y,width,height) method draws a rectangle, filled with the fill style, on the ... 4. Flotr JavaScript Plotting Library. Flotr enables you to draw appealing graphs in most modern browsers with an easy to learn syntax. It comes with great features like legend support, negative ...
Nov 30, 2017 - Since my company has given me a requirement of drawing in a browser programmatically, simply shown as Figure 1.1 above, I would like to share some points with you about drawing in JavaScript… Sep 01, 2017 - In this article, I'm going to explain how to create an HTML5 canvas that enables a user to draw on the screen. This is a relatively simple Javascript snippet that enables some awesome functionality! Let's dive in... Nov 14, 2019 - Introduced with HTML version 5 to draw graphics using JavaScript · Graphics can be 2D or 3D and it’s able to use hardware acceleration · Used often today for creating games and visualizations (data or artistic) ... When working with a canvas there are five steps to get started.
A few primitive shapes can be drawn directly onto the graphics context in JavaScript. The most common shapes for HTML5 and CSS3 programming that most web developers use are rectangles and text. Rectangle functions You can draw three different types of rectangles: clearRect(x, y, w, h): Erases a rectangle with the upper-left corner (x,y) and […] Update the redraw function to draw the outline image using the canvas context's drawImage method. Its parameters are the image object we loaded, the position we want to draw the image, and the dimensions of the image. function redraw(){ ... context.drawImage(outlineImage, drawingAreaX, drawingAreaY, drawingAreaWidth, drawingAreaHeight);} Demo Outline The basic syntax of the rect() method can be given with: ... The following JavaScript code will draw a rectangle shape centered on the canvas.
See the Pen javascript-drawing-exercise-1 by w3resource (@w3resource) on CodePen. Contribute your code and comments through Disqus. Previous: javascript Drawing Exercises. Next: Write a JavaScript program to draw a circle.
Draw Text On Html5 Canvas Using Javascript Syncbite Com
How To Draw Animated Circles In Html5 Canvas Tutorials24x7
Draw Points Circles On A Canvas With Javascript Html5 Our
How To Draw On Scroll Using Javascript Dev Community
How To Draw Geometrical Shapes In Html Canvas Via Javascript
Drawing Scalable Vector Graphics With D3 Javascript Vegibit
10 Javascript Libraries To Draw Your Own Diagrams 2020 Edition
Draw With Code Introducing Kids To Coding Using Simple Type
Drawing Custom Shapes In Html5 Authorcode
Mindfusion Javascript Diagram Library V2 6 Mindfusion
Draw Shapes In Javascript Learn To Code Geometrical Shapes
Random Lucky Draw Application Using Javascript Programming
Draw Floor Plan On Canvas Using Javascript Stack Overflow
Draw Path Using Google Maps Javascript Api Phppot
How To Draw Stars With Javascript And Html5 Canvas By Nevin
Draw A Square In Javascript Using Canvas Stack Overflow
Creating A Beautiful Clock Using Html5 Canvas By Ganesh
Drawing Geometric Figures A Picture Speaks A Thousand Words
0 Response to "21 How To Draw With Javascript"
Post a Comment