32 Javascript Declare New Object
Mar 09, 2018 - In this article we will learn multiple ways to create objects in JavaScript like Object Literal, Constructor Function, Object.Create method… JavaScript allows multiple white spaces and line breaks in a variable declaration. Multiple variables can be defined in a single line separated by a comma. JavaScript is a loosely-typed language, so a variable can store any type value. Variable names are case-sensitive. Variable names can contain letters, digits, or the symbols $ and _.
Making Sense Of Es6 Class Confusion Toptal
Again, you can use the new objects exactly the same way as in 1. and 1.1. 2. Using object literals. Literals are shorter way to define objects and arrays in JavaScript. To create an empty object using you can do: var o = {}; instead of the "normal" way: var o = new Object(); For arrays you can do: var a = []; instead of: var a = new Array();
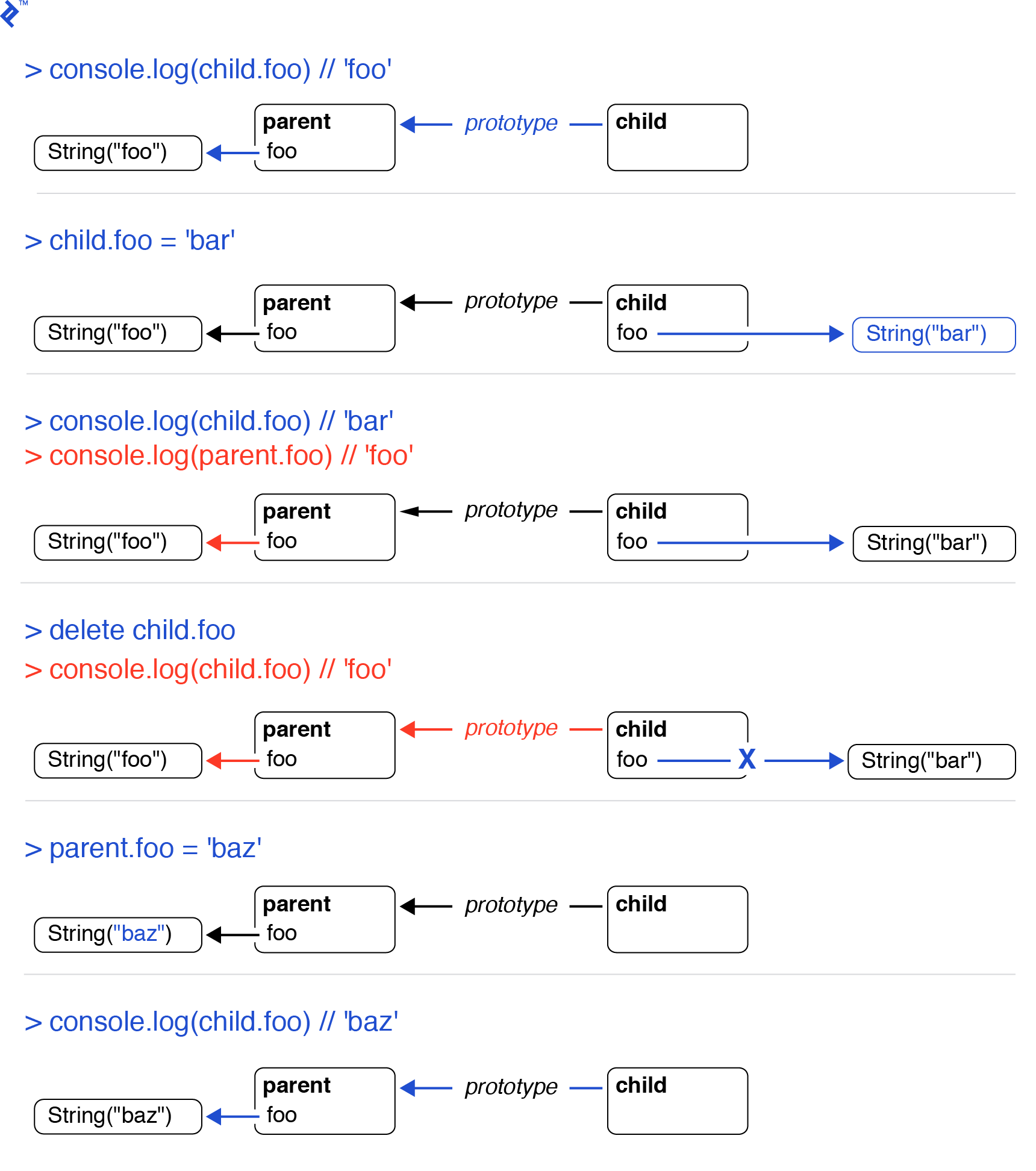
Javascript declare new object. It binds property or function which is declared with this keyword to the new object. It returns newly created object unless the constructor function returns a non-primitive value (custom JavaScript object). If constructor function does not include return statement then compiler will insert ... Aug 20, 2020 - The value of the carbs property is a new object. Here is how we can access the carbs property. ... Consider the case where we have the values of our properties stored in variables. const name = 'apple'; const category = 'fruits'; const price = 1.99; const product = { name: name, category: category, price: price } JavaScript ... When learning JavaScript one of the basics is to understand how to use variables. Variables are containers for values of all possible types, e.g. number, string or array (see data types ).
Dec 11, 2020 - The Rest/Spread Properties for ECMAScript proposal (stage 4) adds spread properties to object literals. It copies own enumerable properties from a provided object onto a new object. Mar 24, 2020 - by Kaashan Hussain We all deal with objects in one way or another while writing code in a programming language. In JavaScript, objects provide a way for us to store, manipulate, and send data over the network. There are many ways in which objects in JavaScript differ from objects in Objects are integral to javascript development and understanding how objects are generated and used is essential.
Understanding Objects in JavaScript ... This is a JavaScript formatting practice in which the final item in a series when declaring a collection of data has a comma at the end. Though this formatting choice can be used for cleaner diffs and easier code manipulation, whether to use it or not is a matter of preference. ... 'bar' } const map = new ... Try it Yourself » Do Not Declare Strings, Numbers, and Booleans as Objects! When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object First, declare the object by using an object function; Lastly, instantiate the newly created object by using the "new" keyword; Lets take this one step at a time. We will now proceed to create an object called "userobject", which, at this stage, does nothing: Step 1: declare the object by using an object function
Object initializer. Objects can be initialized using new Object(), Object.create(), or using the literalnotation (initializernotation). An object initializer is a comma-delimited list of zero or more pairs of property names and associated values of an object, enclosed in curly braces ({}). Syntax. The built-in primitive types in JavaScript are functions only e.g. Object, Boolean, String, Number is built-in JavaScript functions. If you write Object in browser's console window and press Enter then you will see the output "function Object ()". In the above example, we have created an object of MyFunc using new keyword. How should i declare it. and wat namespaces or assembly should i declare. Posted 27-Feb-14 20:27pm. ... Creation of new object: var JSONQury = new Object (); Example which shows creation of an object in javascript using JSON: XML < ...
Creating a JavaScript Object. With JavaScript, you can define and create your own objects. There are different ways to create new objects: Create a single object, using an object literal. Create a single object, with the keyword new. Define an object constructor, and then create objects of the constructed type. Create an object using Object.create(). TypeScript Type Template. Let's say you created an object literal in JavaScript as −. var person = { firstname:"Tom", lastname:"Hanks" }; In case you want to add some value to an object, JavaScript allows you to make the necessary modification. Suppose we need to add a function to the person object later this is the way you can do this. Among the Object constructor methods, there is a method Object.assign () which is used to copy the values and properties from one or more source objects to a target object. It invokes getters and setters since it uses both [ [Get]] on the source and [ [Set]] on the target. It returns the target object which has properties and values copied from ...
Apr 22, 2021 - A protip by fr0gs about javascript, programming, and learning. Objects can be declared same as variables using var or let keywords. The p1 object is created using the object literal syntax (a short form of creating objects) with a property named name. The p2 object is created by calling the Object() constructor function with the new keyword. In Javascript, the declaration of a new property within any object, is very simple and there's even 2 ways to do it: // Declare programatically window.MyProperty = function () { alert ("Hello World"); }; // Declare with Brackets window ["MyProperty"] = function () { alert ("Hello World"); }; The Window variable, is an object, therefore to ...
On this path your first option is to create an empty object: var modal = <IModal>{}; Secondly to fully realise the compulsory part of your interface. It can be useful whether you are calling 3rd party JavaScript libraries, but I think you should create a class instead, like before: var modal: IModal = { content: '', form: '', //... One of them is an object literal, and the other one is a constructor, two different ways of creating an object in javascript. var objectA = {} //This is an object literal var objectB = new Object() //This is the object constructor 1 week ago - JavaScript is designed on a simple object-based paradigm. An object is a collection of properties, and a property is an association between a name (or key) and a value. A property's value can be a function, in which case the property is known as a method. In addition to objects that are predefined ...
In this article, I'll show you the basics of working with object arrays in JavaScript. If you ever worked with a JSON structure, you've worked with JavaScript objects. Quite literally. JSON stands for JavaScript Object Notation. Creating an object is as simple as this: Jul 23, 2020 - One of the easiest way to instantiate an object in JavaScript. Constructor is nothing but a function and with help of new keyword, constructor function allows to create multiple objects of same flavor as shown below: Output: Explanation: A class in OOPs have two major components, certain parameters and few member functions. In this method we declare ... The Object.create () method creates a new object, using an existing object as the prototype of the newly created object. To understand the Object.create method, just remember that it takes two parameters. The first parameter is a mandatory object that serves as the prototype of the new object to be created.
The generator function in JavaScript returns a Generator object. Its syntax is similar to function expression, function declaration or method declaration, just that it requires a star character *. The generator function can be declared in the following forms: Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. May 03, 2017 - Quality Weekly Reads About Technology Infiltrating Everything
Do Not Declare Strings, Numbers, and Booleans as Objects! When a JavaScript variable is declared with the keyword "new", the variable is created as an object: 2/7/2019 · Create Javascript objects using the “new” keyword with the Object() constructor. Now, let’s look at how declaring objects with the “new” keyword and the Object() constructor. It’s as simple as specifying the “new” keyword followed by the Object() constructor. This would create a new, but empty (undefined) object for you to play with. 4 weeks ago - The new operator lets developers create an instance of a user-defined object type or of one of the built-in object types that has a constructor function.
Declare a number variable in JavaScript JavaScript has a single Number object to store numeric values; behind the scenes, it only uses floating points (" floats "), even if it seems to use integers (it simply omits the ".0 " after it). The Array () constructor creates Array objects. You can declare an array with the "new" keyword to instantiate the array in memory. Here's how you can declare new Array () constructor: let x = new Array (); - an empty array JSON objects can be created with JavaScript. Let us see the various ways of creating JSON objects using JavaScript −. Creation of an empty Object −. var JSONObj = {}; Creation of a new Object −. var JSONObj = new Object (); Creation of an object with attribute bookname with value in string, attribute price with numeric value.
Object references and copying. One of the fundamental differences of objects versus primitives is that objects are stored and copied "by reference", whereas primitive values: strings, numbers, booleans, etc - are always copied "as a whole value". That's easy to understand if we look a bit under the hood of what happens when we copy ... In JavaScript, you can make objects in a number of different ways. In this guide, you'll learn step by step how you can create new JavaScript objects. What Is a JavaScript Object? A JavaScript object is a variable that can hold many different values. It acts as the container of a set of related values.
Object Create In Javascript The Object Create Method Is One
Javascript Es5 Interview Questions Amp Answers
Object Oriented Javascript For Beginners Learn Web
The Most Common Patterns To Create Objects In Javascript Es5
Addyosmani Com Essential Javascript Namespacing Patterns
N00b Javascript Creating An Object From Multiple Objects
How To Inspect A Javascript Object
Everything About Javascript Objects By Deepak Gupta
3 Ways To Clone Objects In Javascript Samanthaming Com
Javascript Create Object How To Define Objects In Js
5 Different Ways To Create Objects In Java Dzone Java
Prototypes In Javascript In This Post We Will Discuss What
Chapter 17 Objects And Inheritance
Javascript Object Initializer Geeksforgeeks
The Secret Life Of Objects Eloquent Javascript
Javascript Es6 Classes Objects In Programming Languages
Data Binding Revolutions With Object Observe Html5 Rocks
Understanding The Difference Between Object Create And New
How To Check If Object Is Empty In Javascript Samanthaming Com
5 Different Ways To Create Objects In Java Dzone Java
Javascript Objects Explore The Different Methods Used To
M150 Data Computing And Information Ppt Download
4 Ways To Create Powershell Objects Ridicurious Com
Creating Objects The Java Tutorials Gt Learning The Java
How To Add An Object To An Array In Javascript Geeksforgeeks
Is Creating Js Object With Object Create Null The Same As
Javascript Date And Time In Detail By Javascript Jeep
Object Create In Javascript The Object Create Method Is One
0 Response to "32 Javascript Declare New Object"
Post a Comment