32 Javascript Array Includes Performance
indexOf () was added to the ECMA-262 standard in the 5th edition; as such it may not be present in all browsers. You can work around this by utilizing the following code at the beginning of your scripts. This will allow you to use indexOf () when there is still no native support. This algorithm matches the one specified in ECMA-262, 5th edition ... If you need to find if a value exists in an array, use Array.prototype.includes (). Again, it checks each element for equality with the value instead of using a testing function. If you need to find if any element satisfies the provided testing function, use Array.prototype.some ().
Http Performance Java Jersey Vs Go Vs Node Js Full
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
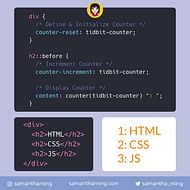
Javascript array includes performance. includes: this is syntactic sugar for the old common way of writing [code]myArray.indexOf('some value') !== -1 [/code]Which can now be written as [code]myArray.includes('some value') [/code]It simply checks to see if some value exists in the array... In an array, the equivalent is using splice () based on an element's index. As in the previous point, depending on indices is slow. Insert an Item: It is faster to add an item to a Set than to add... The Array.push () has a Constant Time Complexity and so is O (1). All it does is add an element and give it an index that's 1 greater than the index of the last element in the array. So it doesn ...
Jan 26, 2020 - Medium served me recently this article by Samantha Ming on various approaches in ES6 to deduplicate an array. I had to solve a similar… Array push / pop / shift is ~approx 20x+ faster than any object equivalent. Surprisingly Array.shift() is fast ~approx 6x slower than an array pop, but is ~approx 100x faster than an object attribute deletion. Amusingly, Array.push( data ); is faster than Array[nextIndex] = data by almost 20 (dynamic array) to 10 (fixed array) times over. Nov 09, 2018 - Everyone who works with JS until now is familiar with Array (don’t tell me you don’t). But what exactly is Array? Well, in general, Array is type of structure representing block of data (numbers…
Jul 18, 2017 - Searching for a sub-string in a string, is one of the most basic operations, that we come across every now and then, and what I usually do is, to look into other places in the code and see what… The array reduce method is really powerful. But people often run into trouble as soon as they step beyond the basic examples. Simple things like addition and multiplication are fine. But as soon as you try it with something more complicated, it breaks. Using it with anything other than numbers starts to get really confusing. I think this is because the examples in most tutorials mask some of ... ECMAScript 2016. The JavaScript naming convention started with ES1, ES2, ES3, ES5 and ES6. But, ECMAScript 2016 and 2017 was not called ES7 and ES8. Since 2016 new versions are named by year (ECMAScript 2016 / 2017 / 2018).
Definition and Usage. The findIndex() method returns the index of the first array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex() returns the index of that array element (and does not check the remaining values) Using for loops is like going backwards, not to mention that forEach is slow because it is modifying/mutating the original array, whereas .map() returns a new array, is much faster, and without the side effect of mutating the original array. There are fewer and fewer cases where a for loop is viable. Array.includes () The includes () method determines whether an array includes a certain value and returns true or false as appropriate. So in the example above, if we want to check if 20 is one of the elements in the array, we can do this:
Apr 24, 2018 - ES2016 Specifications included the includes() method for Array data structur... W rapping up, we've found that JavaScript's built-in.includes () method is the fastest way to check if a JavaScript array contains an item, unless you have an array with a lot of items. In most cases,.includes () is both more readable and faster than for, so definitely use.includes (). Javascript performance test - for vs for each vs (map, reduce, filter, find). ... There are lot of corner cases that javascript function consider like getters, sparse array and checking arguments that are passed is array or not which adds up to overhead.
JavaScript microbenchmarks, JavaScript performance playground. Measure performance accross different browsers. Array.prototype.includes # Community Input. @lolinoid: contains > @prvnbist That's a method DOM Nodes, most known example for it would be getting a list of classnames which will be a node list then you can use contain method to see if it has a classname. Or you can convert it to an array and then use includes method Note: Compared to the array's includes function, Object's hasOwnProperty and Set/Map's has functions seem to perform close to O(1) in different tests, clearly more efficient in terms of larger data sets.
Sep 22, 2020 - The some() method tests whether at least one element in the array passes the test implemented by the provided function. It returns true if, in the array, it finds an element for which the provided function returns true; otherwise it returns false. It doesn't modify the array. Duplicating an Array. While this sounds like a less interesting scenario, this is the pillar of immutable functions, which doesn't modify the input when generating an output. Performance testing findings here again show the same interesting trend — when duplicating 10k arrays of 10k random items, it is faster to use the old school solutions. Updated 04/29/2020. As the commenter rightly pointed out it would seem V8 was optimizing out the array includes calls. An updated version that assigns to a var and uses it produces more expected results. In that case Object address is fastest, followed by Set has and in a distant third is Array includes (on my system / browser).
Javascript array includes performance The JavaScript array is a powerful data structure in web technologies. Methods such as .map(), .filter(), .includes(), and .reduce() help to overcome the issues ahead of the day (Checkout my previous article on these 3 methods). However, there are two similar but different ways that are part of your arsenal. Nov 18, 2019 - Noob v. Algorithms #21: Intersection of Two Arrays JS .includes () vs .some () JavaScript array is a powerful data structure in web technologies. Methods such as .map (), .filter (), .includes (), and .reduce () helps a lot to overcome issues facing day to day (Checkout my previous article on these 3 methods). However, there are two similar but different methods that are part of your arsenal.
This is a short response I wrote to a question on /r/javascript.The user who asked it was curious whether there would be any performance difference between using a JavaScript string's indexOf vs includes performance when trying to find a substring within a larger string.. Let's take a look at the ECMAScript specification to see what it says.. In the case of indexOf vs includes, you'll ... Mar 15, 2019 - JavaScript Arrays are great when you only have a few items, but when you have a large amount of data or want to do complex transformations with lots of map, filter, and reduce method calls, you’ll see a significant slowdown in performance using Array.prototype methods. JavaScript microbenchmarks, JavaScript performance playground. Measure performance accross different browsers.
Big O Performance of Arrays and Objects in JavaScript. The efficiency of the program would be directly relying on Time Complexity when we deal with a huge amount of data to perform operations like searching, sorting, access, insertion and removal of an element. We could selectively use objects and arrays based on the need to enhance the ... What you need is processing relative big data in a low memory, low performance environment. The general solution to this is using streams. In these streams you put only a single or a few chunks in the memory, process it, and free the memory. So you won't need a lot of memory and processing power to do the job. So yes, I am right that with a small quantity of data, Array.includes and Set.has perform roughly the same, but we can see how quickly performance degrades, and the change is so small that it's hard to justify not making it, even for small data samples. Should the size of the data increase, especially the size of the valuesToKeep array, the code is future proof.
Aug 23, 2017 - includes also is of no use if you want to know where the string is in the array. Concerning performances: according to this article on the subject there are no noticeable difference although includes may be a very little bit slower. 2. Object.keys. The Object.keys() method returns an array of Object keys. This creates an array that contains the properties of the object. You can then loop through the array to get the keys and ... The array has a length of 10, and the loop iterates for 1,000 times. So each execution of this code represents 10,000 operations. In the following sections, you'll find the different library imports and JavaScript for each method, the results of the tests appear at the end of this blog article.
Includes () vs indexOf () in JavaScript. Abuja, Nigeria. ES2016 Specifications included the includes () method for Array data structure. The includes () method check if an array includes a certain element, returning true or false as appropriate. But in ES5 we are used to performing operations like this with indexOf () method. .includes() returns a boolean true or false if the item sought is in the array. .indexOf() returns the index if found, -1 if not. If you are searching for NaN (not a number) in an array, use .includes(). indexOf() won't find it because it uses a strict equality operator === under the hood, and NaN === NaN evaluates to false . Using Sets in ES6 to produce lists of unique objects is faster than using arrays, and less error prone than using objects. In this lesson, we explore the pitfalls of the object approach and the speed implications of the array approach. We will then instrument the array approach and the set ...
array.includes vs _.includes. Run results for: array includes - MeasureThat . ... JavaScript microbenchmarks, JavaScript performance playground. Measure performance accross different browsers. measurethat . Now, there is a better way to filter the elements using the following snippet. Method 1: Brute Force approach. Compare each and every item from the first array to each and every item of second array. Loop through array1 and iterate it from beginning to the end. Loop through array2 and iterate it from beginning to the end. Compare each and every item from array1 to array2 and if it finds any common item then return true ... We're sorry but JSBEN.CH doesn't work properly without JavaScript enabled. Please enable it to continue
Aug 25, 2018 - by pacdiv Here’s how you can make better use of JavaScript arraysPhoto by Ben White on UnsplashQuick read, I promise. Over the last few months, I noticed that the exact same four mistakes kept coming back through the pull requests I checked. I’m also posting this article because The includes() method determines whether an array includes a certain value among its entries, returning true or false as appropriate. Syntax includes ( searchElement ) includes ( searchElement , fromIndex )
Exploration And Practice Of Frontend Memory Optimization
Beginner S Guide To Javascript Arrays Daniel Puiatti
How To Check If A Javascript Array Is Empty Or Not With Length
Javascript Array Performance To Add New Element By Different
Should You Use Includes Or Filter To Check If An Array
Increaseing Performance Of React Applications Via Array
Performance Tools Built Into Crm Powerobjects Powerobjects
Multikey Indexes Mongodb Manual
Performance Marketing In 2021 Everything You Need To Know
Lib Prefer Using Array Includes Instead Of Array Indexof
Measuring The Performance Of Javascript Functions
Simulated Performance Of The Antenna Array With Without The
Javascript Performance For Vs Foreach
Performance Of Javascript Array Ops By Miguel Albrecht Medium
Javascript Arrays From O N 2 To O N Code Examples
How To Check If Array Includes A Value In Javascript
High Performance Quasi 2d Perovskite Light Emitting Diodes
Javascript Array Contains How To Use Array Includes Function
Improve Performance With The Object Pool Design Pattern In
Remove Duplicate Values From Javascript Array How To
Benchmark Array Includes Measurethat Net
Should You Use Includes Or Filter To Check If An Array
How Performant Are Array Methods And Object Methods In
Performance Of Javascript Foreach Map And Reduce Vs For
11 Ways To Check For Palindromes In Javascript By Simon
Javascript Typedarray Performance Newbedev
10 Javascript Array Methods To Boost Your Code Performance
Performance Of Javascript Foreach Map And Reduce Vs For
The Fastest Way To Find Minimum And Maximum Values In An
Seven Different Ways To Check Presence Of An Element In An
0 Response to "32 Javascript Array Includes Performance"
Post a Comment