30 Clone An Object In Javascript
How to Clone a JavaScript Object Cloning an object in JavaScript is a common task for any project: from simple objects to complicated ones. As a rule, the assignment operator doesn't generate a copy of an object. It is capable of assigning a reference to it. Aug 16, 2018 - A quick and easy guide on how to deep copy an object in JavaScript.
How To Clone Javascript Object Tecadmin
I have a super class that is the parent (Entity) for many subclass (Customer, Product, ProductCategory…)I'm looking to clone dynamically an object that contains different sub objects in Typescript. In example : a Customer that has different Product who has a ProductCategory
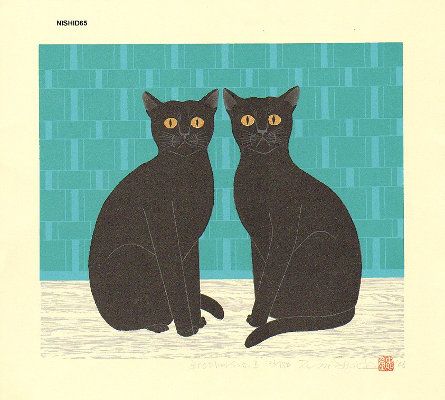
Clone an object in javascript. heroClone is a clone object of hero, meaning that it contains all the properties of hero.. hero === heroClone evalutes to false — hero and heroClone are, nevertheless, difference object instances.. 1.1 Object spread bonus: add or update cloned props. An immediate benefit of using object spread is that you can update or add new properties to the cloned object in place if you need it. JSON.parse turns a string into an object. Combining them can turn an object into a string, and then reverse the process to create a brand new data structure. Note: This one safely copies deeply nested objects/arrays! Nov 01, 2020 - Note: Copying objects derived from built-in JavaScript objects will result in extra, unwanted properties. ... The HTML standard includes an internal structured cloning/serialization algorithm that can create deep clones of objects. Although still limited to certain built-in types, it can preserve ...
JavaScript offers many ways to create shallow and deep clones of objects. You can use the spread operator (...) and Object.assign () method to quickly create a shallow object duplicate. For the deep cloning of objects, you can either write your own custom function or use a 3rd-party library like Lodash. Edit existing answers to improve this post. It is not currently accepting new answers or interactions. What is the most efficient way to clone a JavaScript object? I've seen obj = eval(uneval(o)); being used, but that's non-standard and only supported by Firefox. Deep Copy Objects. All methods above create shallow copies of objects. If you have non primitive data types as properties, and you try to make a copy, the object will be copied, BUT the underlying objects will be passed by reference to the new object. This means a shallow copy instead of a deep copy has been created.
Javascript object is the collection of properties, and the property is an association between the key-value pair. Javascript objects are reference values, you can't simply just copy using the = operator. Clone Object In Javascript JavaScript offers many ways to copy an object, but not all provide a deep copy. 1. Shallow copy. To shallow copy, an object means to simply create a new object with the exact same set of properties. We call the copy shallow because the properties in the target object can still hold references to those in the source object.. Before we get going with the implementation, however, let's first write some tests, so that later we can check if everything is working as expected. Nov 25, 2020. "Cloning" an object in JavaScript means creating a new object with the same properties as the original object. Objects in JavaScript are stored by reference, which means that two variables can point to the same object in memory. Modifying one object variable can impact other variables. const obj1 = { a: true, b: true }; const obj2 ...
Nov 20, 2018 - Read programming tutorials, share your knowledge, and become better developers together. ... In this post we will discuss the different methods to copy the javascript object and discuss the outcome of each method. Cloning in javascript is nothing but copying an object properties to another object so as to avoid creation of an object that already exists. There are a few ways to clone a javascript object. 1) Iterating through each property and copy them to a new object. 2) Using JSON method. Nov 23, 2017 - Objects are the fundamental blocks of JavaScript. An object is a collection of properties, and a property is an association between a key (or name) and a value. Almost all objects in JavaScript are instances of Object which sits on the top of the prototype chain.
The Object.assign () method is used to copy all enumerable values from a source object to the target object. When a JavaScript variable is declared with the keyword " new ", the variable is created as an object: x = new String (); // Declares x as a String object. y = new Number (); // Declares y as a Number object. z = new Boolean (); // Declares z as a Boolean object. Avoid String, Number, and Boolean objects. They complicate your code and slow down ... Cloning using object spread. This one is the simplest way to clone a plain JavaScipt object, const clone = {. ...sampleObject. }; Where sampleObject is the object which we want to copy, and clone is the shallow copy of object. Let's take an example of creating a shallow copy of the "person" object, const person = {.
Definition and Usage The cloneNode () method creates a copy of a node, and returns the clone. The cloneNode () method clones all attributes and their values. Tip: Use the appendChild () or insertBefore () method to insert the cloned node to the document. When we copy an object or array and all the level properties are copied without any reference, then it is Deep cloning. Objects should always be deeply cloned. When we copy an object or array and all the level properties are copied without any reference, then it is Deep cloning. Objects should always be deeply cloned. May 05, 2018 - Searching how to deep clone an object in JavaScript on the internet, you’ll find lots of answers but the answers are not always correct. ... My suggestion to perform deep copy is to rely on a library that’s well tested, very popular and carefully maintained: Lodash. Lodash offers the very convenient clone ...
Jul 02, 2019 - Cloning an object in JavaScript a task that is almost always used in any project, to clone everything from simple objects to the most complicated ones. As it may seem simple for not seasoned… It's important to understand how to clone an object in JavaScript correctly. It is possible to create a shallow copy and a deep copy of an object. A shallow copy of an object references the original. So any changes made to the original object will be reflected in the copy. A JavaScript object is a complex data type that can contain various data types. For example, const person = { name: 'John', age: 21, } Here, person is an object. Now, you can't clone an object by doing something like this. const copy = person; console.log(copy); // {name: "John", age: 21}
Aug 04, 2016 - To do this for any object in JavaScript will not be simple or straightforward. You will run into the problem of erroneously picking up attributes from the object's prototype that should be left in the prototype and not copied to the new instance. If, for instance, you are adding a clone method ... Mar 27, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. const cloneFood = Object.assign({}, food); {} is the object that is modified. The target object is not referenced by any variable at that point, but because Object.assign returns the target object, we are able to store the resulting assigned object into the cloneFood variable. We could switch our example up and use the following:
Nov 22, 2017 - So I'm always looking for a way to use vanilla JS whenever possible these days, and I discovered th... Object.create () creates a new object and Object.getPrototypeOf () gets the prototype chain of the original instance and adds them to the newly created object Object.assign () does as we've seen earlier, which is to (shallow) copy the instance variables over to the newly created object We can clone the object as one of the main tasks in JavaScript because it is most widely used; it takes some time consumption because the entire object property and attributes are also copied the same in the destination or target object in the script. By using this cloning concept, it will be achieved in three different ways.
1 week ago - The Object.assign() method copies all enumerable own properties from one or more source objects to a target object. It returns the modified target object. Since cloning objects is not trivial (complex types, circular references, function etc.), most major libraries provide function to clone objects. Don't reinvent the wheel- if you're already using a library, check if it has an object cloning function. One way to clone a JavaScript object is to use the Object.assign method. For instance, we can write: const obj = {. a: 1. }; const copy = Object.assign ( {}, obj); console.log (copy); We call Object.assign with an empty object and obj to copy the properties of obj onto the empty object and return it. Then copy has the same content as obj .
Cloning objects in javascript is very common when it comes to functional programming where you work with immutable objects a lot. For example, if you are working with a state management library in... Code language: CSS (css) The reason is that the address is reference value while the first name is a primitive value. Both person and copiedPerson references different objects but these objects reference the same address objects.. Deep copy example. The following snippet replaces the Object.assign() method by the JSON methods to carry a deep copy the person object: Jul 22, 2019 - Because objects in #JavaScript are references values, you can't simply just copy using the =. But no worries, here are 3 ways for you to clone an object 👍
When we perform actions with the object, e.g. take a property user.name, the JavaScript engine looks at what's at that address and performs the operation on the actual object. Now here's why it's important. When an object variable is copied, the reference is copied, but the object itself is not duplicated. Sep 21, 2020 - Objects are the fundamental blocks of JavaScript. An object is a collection of properties, and a property is an association between a key (or name) and a value. Almost all objects in JavaScript are instances of Object which sits on the top of the prototype chain.
How To Clone Objects In Javascript Weekly Webtips
How To Deep And Shallow Clone An Object In Javascript
Different Ways To Duplicate Objects In Javascript By
Cloning In Javascript Object Ta Digital Labs
What Causes A Type 021 Error While Using Object Cloning And
Understanding Deep And Shallow Copy In Javascript Manjula Dube
Javascript Fundamental Es6 Syntax Create A Deep Clone Of
6 Different Ways To Clone Objects In Javascript By Iliyas
Dev Diaries Javascript Cloning
Be Aware When Cloning Objects In Javascript Dev
Ways To Clone An Object In Javascript By Tran Son Hoang
What Is The Most Efficient Way To Deep Clone An Object In
Showing Objects In The Javascript Console Without Going Mad
How To Clone A Javascript Object
3 Ways To Clone An Object In Javascript Dev Community
How To Deep Clone In Javascript Geeksforgeeks
Javascript Clone Objects And Deep Copy Without Reference
Clone Javascript Object With Json Stringify By Mayank Gupta
3 Ways To Clone Objects In Javascript Samanthaming Com
How To Deep Clone An Array In Javascript Dev Community
Deep Copy Array Of Object Javascript
Different Ways To Duplicate Objects In Javascript By
Deep Clone An Object And Preserve Its Type With Typescript
What Is The Most Efficient Way To Deep Clone An Object In
Understanding Object Cloning In Javascript Part I
Eternus Solutions Want To Know How To Deep Clone A
Remove Duplicate Array Of Objects Javascript Code Example
How Do I Correctly Clone A Javascript Object Stack Overflow
0 Response to "30 Clone An Object In Javascript"
Post a Comment