35 Javascript Read File Line By Line
Java BufferedReader class provides readLine () method to read a file line by line. The signature of the method is: public String readLine () throws IOException The method reads a line of text. file = open ("filename.txt", "r") data = file.readlines () for i in data: print (i.replace (" ", "#"))
How To Read Javascript Text File From Local System
27/4/2014 · handleFiles(input) { const file = input.target.files[0]; const reader = new FileReader(); reader.onload = (event) => { const file = event.target.result; const allLines = file.split(/\r\n|\n/); // Reading line by line allLines.forEach((line) => { console.log(line); }); }; reader.onerror = (event) => { alert(event.target.error.name); }; reader.readAsText(file); }
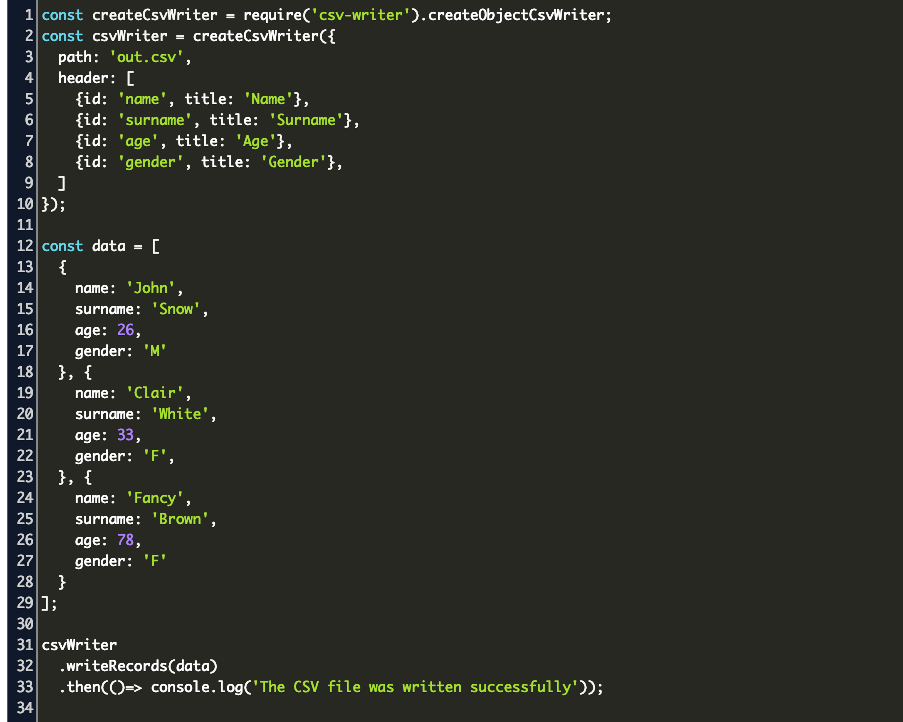
Javascript read file line by line. Read line by line from a file. Contribute your code and comments through Disqus. Previous: Write a Java program to read a plain text file. Next: Write a Java program to store text file content line by line into an array. Jul 29, 2020 - Allowed memory size of 1610612736 bytes exhausted (tried to allocate 4096 bytes) in phar:///usr/local/Cellar/composer/1.9.1/bin/composer/src/Composer/DependencyResolver/Solver.php on line 223 mac ... File C:\Users\Tariqul\AppData\Roaming\npm\ng.ps1 cannot be loaded because running scripts is ...
Java NIO libraries - FileChannel to read the files. It is a non-blocking mode of reading files BufferedReader - Which is a blocking operation i.e it blocks any other read/write request to the file until it has completed the task. Apache Commons IO - FileUtils, simpler way to read files line by line. Readline (from v0.12 and on) Node.js has the native module to read files that allows us to read line-by-line. It was added in 2015 and is intended to read from any Readable stream one line at a time. This fact makes it a versatile option, suited not only for files but even command line inputs like process.stdin. javascript read file lines into array vanilla Code Example, Get code examples like "javascript read file lines into array vanilla" txt file · how to write to and read from text files line by line using javascript CSV is usually a comma (or TAB)-separated text format. So what you can do is split the text string using the new-line character ...
Creating Line-by-Line File Reader Using HTML5 and JavaScript. August 11, 2014 2304 HTML5 Form Elements. A tutorial about reading files line by line in browser using the HTML5 FileReader API and javascript. Website Demo. #HTML5 #Tutorial #javascript #HTML5 File API. Line-by-line Processing in node.js, You want to do it with JavaScript or node.js. How can you do Error: Cannot create a string longer than 0x3fffffe7 characters at Buffer. You need the way to read a file line by line, if it is possible, asynchronously. In this article line-reader is an open-source ... May 19, 2021 - Create a file as app.js (or whatever name you want) and paste below code - app.js const readline...
Today, We want to share with you javascript read text file line by line.In this post we will show you javascript filereader, hear for javascript read local file from path we will give you demo and example for implement.In this post, we will learn about PHP file_get_contents URL with an example. In this project we are looking at how to read through a text file using JavaScript. We will store a list of pupils in a text file. We are going to read through this list display its content on the webpage, one line at a time. Step 1: Using notepad, create a CSV file called ClassList.csv (Instead of using comma we use a pipe | as a separator) Step 2: Create an HTA application (See our blog post ... 9/4/2020 · In this video tutorial, you will learn how to read text file in javascript line by line.Source Code:https://www.fwait /how-to-read-text-file-in-javascript...
To read the line and move on, we should use the nextLine () method. This method advances the scanner past the current line and returns the input that wasn't reached initially. This method returns the rest of the current line, excluding any line separator at the end of the line. Oct 01, 2018 - Hi, I need help for JS code. I could not read dancers.txt with belowing code. Please don’t say to me about use read(). I tried to in below code block. Also, I have a another problem. In sublime text, .split("\n") is wrong for editor. I did not initialize it. Java Read File line by line using BufferedReader. We can use java.io.BufferedReader readLine() method to read file line by line to String. This method returns null when end of file is reached. Below is a simple program showing example for java read file line by line using BufferedReader.
It is basically a JavaScript program (fs.js) where function for reading operations is written. Import fs-module in the program and use functions to read text from the files in the system. Used Function: The readFile () functions is used for reading operation. The Readline module provides a way of reading a datastream, one line at a time. May 20, 2020 - Understanding basic JavaScript codes. ... The ability to read a file line by line allows us to read large files without entirely storing it to the memory. It is useful in saving resources and improves the efficiency of the application. It allows us to look for the information that is required ...
Oct 09, 2019 - Auto-suggest helps you quickly narrow down your search results by suggesting possible matches as you type. ... How to read lines from a text file with Javascript... The file contents are stored in the FileReader's result string property. Being a string, it allows you to manipulate it just as you would any string. For instance, you can see it in the code above where I displayed the first line. Reading Multiple Files and Properties Using a Closure Use Plain JavaScript to Read a Local File Line by Line in JavaScript We can create a simple JavaScript function to read the local file put in as the HTML Input. We can use the input HTML tag to upload the file, and the function FileReader () then our function will read the content from the file line by line with the use of function
In some cases you may need to read a file, line by line, asynchronously. This may be required if the file is huge in size. Node.js provides a built-in module readline that can read data from a readable stream. It emits an event whenever the data stream encounters an end of line character (\n, \r, or \r\n). How to use Javascript to read local ... By Krishnaa JavaScript Questions 0 Comments In this video tutorial, you will learn how to read text file in javascript line by line. Read a file one line at a time in node.js?, Here's the easiest way to read lines from a file, without any ... Nov 16, 2020 - Allowed memory size of 1610612736 bytes exhausted (tried to allocate 4096 bytes) in phar:///usr/local/Cellar/composer/1.9.1/bin/composer/src/Composer/DependencyResolver/Solver.php on line 223 mac ... File C:\Users\Tariqul\AppData\Roaming\npm\ng.ps1 cannot be loaded because running scripts is ...
However, the microdrive does support fragmented reads. In the following example, we read a file line by line from a file on microdrive 1. 10 REM open my file for input. 20 OPEN #4;"m";1;"MYFILE": REM stream 4 is the first available for general purpose. 30 INPUT #4; LINE a$: REM a$ will hold our line from the file. FileReader.readAsText (): Reads the contents of the specified input file. The result attribute contains the contents of the file as a text string. This method can take encoding version as the second argument (if required). The default encoding is UTF-8. In this case we are using FileReader.readAsText () method to read local .txt file. This code ... 1/9/2019 · The simplest way of reading a file line by line in Node.js is by using the native fs module's fs.readFileSync() method: const fs = require ( 'fs' ) ; try { // read contents of the file const data = fs . readFileSync ( 'file.txt' , 'UTF-8' ) ; // split the contents by new line const lines = data . split ( / \r?\n / ) ; // print all lines lines . forEach ( ( line ) => { console . log ( line ) ; } ) ; } catch ( err ) { console . error ( err ) ; }
22/1/2021 · The following snippet uses Node.js native readline module to read the above file into a stream and print line by line. import { createReadStream } from 'fs' import { createInterface } 'readline'... How to use Javascript to read the local text file and read line by line? I have a web page made by html+javascript which is demo, I want to know how to read a local csv file and read line by line so that I can extract data from the csv file.Without jQuery: document.getElementById('file').onchange = function(){ var file = Using simple javascript, i want to open a simple text file, read it line by line and write every line's content into an array. i don't want to use Node.JS i've tried below code but it works only in IE, and i'm also not sure how to take it further to read the lines of files and store it in array. What I have tried:
Change this line: showContents(xhr.responseText, xhr); To this code: var lines = xhr.responseText.split('\n'); for (var i = 0; i < lines.length; i++) { showContents(lines[i]); } This will split the file into an array of lines. Then loop over each line and render inside an <li> element using your existing showContents function. Nov 18, 2015 - Node.js read file line by line and transform it. GitHub Gist: instantly share code, notes, and snippets. To read any file in javascript, you have to make use of FileReader object. You can read all kind of file types such jpeg, txt, json, png, etc. For images, you can make use of readAsDataURL () function to get base64 encoded string and use it directly as source in image tag. Please check out base64 to image converter online tool to see it in action.
In this Tutorial. Quick Sample Code. Demo — Reading a Local Text File. How is File Reading Done ? Step 1 — Allow User to Choose the File. Step 2 — Read File Metadata (Name, Type & Size) using Properties of File Object. Step 3 — Read File Contents using FileReader Object. Other FAQs on Reading a File with Javascript. Description: Below example shows how to read file content line by line. To get this, you have to use BufferedReader object. By calling readLine() method you can get file content line by line. readLine() returns one line at each iteration, we have to iterate it till it returns null. Using readFile(), which is the asynchronous version of readFileSync(), would be a solution to the memory problem.But, the callback function would be called more than once and the passed data through callback function is not guaranteed to be passed line by line.. You need the way to read a file line by line, if it is possible, asynchronously.
18/8/2021 · Use Plain JavaScript to Read a Local File Line by Line in JavaScript <input type="file" name="myfl" id="myfl" /> document.getElementById('myfl').onchange = function(){ var myfl = this.files[0]; var reader = new FileReader(); reader.onload = function(progressEvent){ console.log(this.result); }; reader.readAsText(myfl); }; How do you do this? share. javascript - node.js: read a text file into an array. (Each line an item in the array.) node.js: read a text file into an array. (Each line an item in the array , To read a big file into array you can read line by line or chunk by chunk. Nov 16, 2020 - Allowed memory size of 1610612736 bytes exhausted (tried to allocate 4096 bytes) in phar:///usr/local/Cellar/composer/1.9.1/bin/composer/src/Composer/DependencyResolver/Solver.php on line 223 mac ... File C:\Users\Tariqul\AppData\Roaming\npm\ng.ps1 cannot be loaded because running scripts is ...
Js Append To File Code Example
Node Js How To Execute Another File Code Example
Nodejs Reading And Processing A Delimiter Separated File
Laravel When I Reading An Html File In The Public Directory
Promises Fetch In Javascript How To Extract Text From Text
Decompiling Node Js In Ghidra Pt Swarm
Node Js Give Undefined Data In Nested Fs Readfile Call
Read A Local File Using Html5 And Javascript Dotnetcurry
Need Help In Reading Text Files Using Javascript File Reader
Neoload Javascript Api With Read And Write To File Examples
Node Fs Nodejs Create File Read File Write To File
Reading A File Line By Line In Node Js
Read Write Json Files With Node Js By Osio Labs Medium
What Is Javascript Learn Web Development Mdn
Javascript Read Text File Line By Line Into Array
Node Js Determining The Line Count Of A Text File Dev
How To Read A Value From Properties File In A Javascript File
How To Create A Public File Sharing Service With Vue Js And
Node Hero Understanding Async Programming In Node Js
Node Js Tutorial 4 Reading File In Node Js Algorithms
Js Drop And Display A File To A Html Useful Code
Js Drop And Display A File To A Html Useful Code
Read Files Amp Directories Using File System Module Node Js
How To Read A File Line By Line In Node Js In 2015 Youtube
Read Locally Txt File Use Fetch Method In Javascript By
Using Ajax To Read From Local Filesystem Without A Web Server
Reading A Text File Using Javascript James D Mccaffrey
How To Use Writefile And Readfile In Cypress Testersdock
Read File To String Nodejs Code Example
Asynchronous Adventures With Node Js By Priyesh Saraswat
Node Js Read Csv Line By Line Code Example
Javascript Read File Lines Into Array Vanilla Code Example
Fs Readfile Vs Streams To Read Text Files In Node Js By
0 Response to "35 Javascript Read File Line By Line"
Post a Comment