33 Command Design Pattern Javascript
24/2/2017 · The command pattern is a behavioral design pattern in which an object is used to encapsulate all information needed to perform an action or trigger an event at a later time. This information includes the method name, the object that owns the … JavaScript Command Design Pattern with Example Code.
The Command Design Pattern What Is It And How Can We Apply
The Command Pattern is a strange beast in the context of object-oriented programming. Unlike most objects, a command object represents a verb, rather than a noun. This is a little less odd in a language like JavaScript where functions are actually a type of object, but the classical sense of the Command pattern is still different than a function.
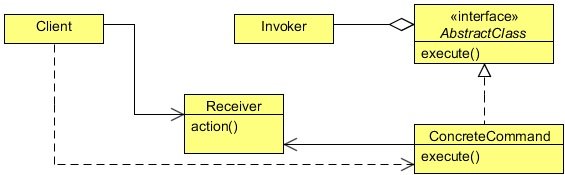
Command design pattern javascript. Jun 04, 2017 - By the way whole Redux architecture is actually Command pattern where: The Store = The Receiver The Action = The Command Dispatch = Executor · P.S. I found it useful to read Addy's book along GoF book. ... Not the answer you're looking for? Browse other questions tagged javascript design-patterns ... May 23, 2019 - In this article, I am going to describe the how the **Command Pattern; and** how and when it should be applied. Tagged with javascript, typescript, patterndesign, cleancode. Prototype Design Pattern. The prototype design pattern lets us create clones of the existing objects. This is similar to the prototypal inheritance in JavaScript. All of the properties and methods of an object can be made available on any other object by leveraging the power of the __proto__ property.
Command Pattern is a behavioral JS design pattern that aims to encapsulate actions or operations as objects. This pattern is useful in scenarios where we want to decouple or split the objects executing the commands from objects issuing the commands. Design Patterns in Javascript v.ES6. ... Design Patterns in ES6. A software design pattern is a general reusable solution to a commonly occurring problem within a given context. Adapter. The Adapter Pattern converts the interface of a class into another interface the clients expect. ... Command. The Command Pattern encapsulates a request as an ... Command Game Programming Patterns Design Patterns Revisited. Command is one of my favorite patterns. Most large programs I write, games or otherwise, end up using it somewhere. When I've used it in the right place, it's neatly untangled some really gnarly code. For such a swell pattern, the Gang of Four has a predictably abstruse description:
The Command Pattern The Command pattern aims to encapsulate method invocation, requests, or operations into a single object and gives us the ability to both parameterize and pass method calls … - Selection from Learning JavaScript Design Patterns [Book] Factory Pattern. Like a factory producing multiple copies of the same unit or product — thus the name. We have a class with common properties and functions and we create objects from this class. Follow the example below. OUTPUT: Here, the factory is "peopleFactory", the common traits are "name", "age", "hobby" and ... :books: Educational list of Javascript Design Patterns - KleoPetroff/javascript-design-patterns
All design patterns have been implemented in ES6, the new version of javascript. Since javascript does not have any implementation of interfaces, the examples here use implied interfaces, which means that as long as a class has attributes and methods that a particular interface is supposed to have, it is considered to implement that interface. Introducing our new series: #TechTips ! In these videos, our in-house developer team at Hackages share with you the tech tips you need to become a better de... In object-oriented programming, the command pattern is a behavioral design pattern in which an object is used to encapsulate all information needed to perform an action or trigger an event at a later time. This information includes the method name, the object that owns the method and values for the method parameters — Wikipedia
Behavioral Design Patterns: Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method and Visitor Who Is the Course For? This course is for JavaScript developers who want to see not just textbook examples of design patterns, but also the different variations and tricks that can be ... #YauhenK #webDev #JS #JSPatterns #ityoutubersruะัะตั ะฟัะธะฒะตัััะฒัั ะฒ ะบัััะต «JavaScript Design Patterns».ะ ะดะฐะฝะฝะพะผ ะฒะธะดะตะพ-ะบัััะต ะผั ั ะฒะฐะผะธ ... Jul 17, 2019 - We will discuss the following patterns in detail: Chain of Responsibility Pattern, Command Pattern, Iterator Pattern, Mediator Pattern, Observer Pattern, State Pattern, Strategy Pattern, and Template Pattern. ... This is a class-based creational design pattern.
21/12/2019 · What is the command design pattern? Design patterns are usually categorized between three different types of categories , and in this case the command pattern falls into the behavioral one. The reason why is because its purpose is to encapsulate objects that have the double responsibility of deciding which methods to call and what happens inside. The command design pattern encapsulates actions as objects. Therefore, it is helpful if you wish to decompile or execute objects. The command, client, receiver, invoker are participants in this design pattern. The example below implements the command design pattern: JavaScript Design Patterns Gang of Four. For full access to all 16 lessons, including source files, subscribe with Elements. Overview; Transcript; 6.1 The Command Pattern. Commands encapsulate method invocations in an object. This has several benefits, as you'll see in this lesson. 1.
I've never used this pattern thus I am really interested in hearing what could be good implementations of this pattern using javascript es6 syntax. After reading about this pattern on Google I've written my own implementation using a small example with only 2 types of command (update and delete) and a mockup db for simplicity. An open-source book on JavaScript Design Patterns. Learning JavaScript Design Patterns A book by Addy Osmani ... The general idea behind the Command pattern is that it provides us a means to separate the responsibilities of issuing commands from anything executing commands, delegating this responsibility to different objects instead. ... The command pattern decouples the objects executing the commands from objects issuing the commands. The command pattern encapsulates actions as objects. It maintains a stack of commands whenever a command is executed, and pushed to stack. ... This has been a brief introduction to the design patterns in modern JavaScript (ES6). This subject is ...
Jan 16, 2018 - If your team is used to functional programming, then know that design patterns like the Command pattern can be used in a functional way as well. In software engineering, a design pattern is a general, reusable solution to a common problem or situation, such as building a JavaScript web application. A design pattern is like a blueprint that provides a reusable template for solving these problems. Using a design pattern has many advantages for your JS code: They are widely applicable. Morioh is the place to create a Great Personal Brand, connect with Developers around the World and Grow your Career!
Command pattern is a data driven design pattern and falls under behavioral pattern category. A request is wrapped under an object as command and passed to invoker object. Invoker object looks for the appropriate object which can handle this command and passes the command to the corresponding object which executes the command. All the 23 (GoF) design patterns implemented in Javascript - GitHub - fbeline/design-patterns-JS: All the 23 (GoF) design patterns implemented in Javascript Feb 21, 2017 - With the command pattern we can easily improve the design. ... So we added this function. ... Basically, what this function does is to serve as the command center of the Calculator object. Here we are sending the Calculator object the type of command we would like to invoke and the parameters ...
To wrap up, I would say the command design pattern ends my 7 best sums of JavaScript design patterns. The command design pattern encapsulates method invocation, operations or requests into a single object so that we can pass method calls at our discretion. Dec 05, 2019 - The code is easier to use, understand and test since the commands simplify the code. ... I will now show you how you can implement this pattern using JavaScript/TypeScript. In our case, I have made up a problem in which there is a class named Agent which defines the attributes: stockTrade; ... Definition: The command pattern encapsulates a request as an object, thereby letting us parameterize other objects with different requests, queue or log requests, and support undoable operations. The definition is a bit confusing at first but let's step through it.
According to Wikipedia In object-oriented programming, the command pattern is a behavioral design pattern in which an object is used to encapsulate all information needed to perform an action or... JavaScript Command. The Command pattern encapsulates actions as objects. Command objects allow for loosely coupled systems by separating the objects that issue a request from the objects that actually process the request. These requests are called events and the code that processes the requests are called event handlers. Oct 24, 2019 - Command pattern is a kind of behavior pattern in JavaScript Design pattern. Definition: To send requests to some objects, but we don't know what the requested operation is, so we hope to design the program in a loose coupling way, so that the coupling relationship between the sender and the ...
Behavioral Design Patterns: Chain of Responsibility, Command, Interpreter, Iterator, Mediator, Memento, Observer, State, Strategy, Template Method and Visitor Who Is the Course For? This course is for JavaScript developers who want to see not just textbook examples of design patterns, but also the different variations and tricks that can be ... Chain of Responsibility Structure. There are three parts to the Chain of Responsibility pattern: sender, receiver, and request. The sender makes the request. The receiver is a chain of 1 or more objects that choose whether to handle the request or pass it on. The request itself can be an object that encapsulates all the appropriate data. The command design pattern includes method invocations, operations, or applications in a single object so that we can run method calls using our discretion. The command design pattern lets us issue commands from anything that executes commands and instead delegate responsibility to various objects.
Nov 18, 2011 - I’ve been looking for a JavaScript Command Pattern implementation for a while now. If you google that term (or click that link) you’ll find a handful or more, that I simply don’t like. Granted, the implementations that I’ve found do work, but there’s always something about them that ... Welcome to my Command Design Pattern Tutorial! The Command design pattern allows you to store a list of commands for later use. With it you can store multiple commands in a class to use over and over. I cover the basic pattern in numerous ways including descriptions, diagrams and code. 28/4/2020 · The command design pattern encapsulates method invocation, operations, or requests into a single object so that we can pass method calls at our discretion. The command design pattern gives us an opportunity to issue commands from anything executing commands and delegates responsibility to different objects instead.
Command Design Pattern In C Dot Net Tutorials
Factory Designpattern With Composition In Javascript By
The Command Design Pattern In Javascript Jsmanifest
Discussion Of The Command Design Pattern In Javascript Dev
Design Patterns Command Pattern Codeproject
Learning The Command Pattern In Javascript Discoversdk Blog
Design Patterns Explained Dependency Injection With Code
Patterns For Javascript Frontend Applications By Richard Ng
The 3 Types Of Design Patterns All Developers Should Know
Practical Design Patterns In Javascript
The Command Pattern Learning Javascript Design Patterns Book
Command Design Pattern Explained With C Examples
Encapsulating Logic Using The Command Pattern Blog
Javascript Design Patterns The Ultimate Guide To The Most
Command Design Pattern Explained With C Examples
Command Design Pattern In Javascript
Understanding Design Patterns Command Pattern Using
The Command Design Pattern In Javascript Jsmanifest
Javascript Design Pattern Command Pattern
Command Pattern In Java And Python En Proft Me
Javascript Common Design Patterns
Command Design Pattern In Javascript
Command Design Pattern Example Java Code Geeks 2021
Javascript Design Patterns Javatpoint
Command Design Pattern In Javascript
0 Response to "33 Command Design Pattern Javascript"
Post a Comment