30 Javascript Json Search By Value
Printing a JSON value to the page. Let's say you wanted to print part of the JSON (the wind speed data) to the page, not just the console. (By "print," I mean make the value appear on the page, not send it to a printer.) Printing the value involves a little bit of JavaScript (or jQuery to make it easier). To access the JSON object in JavaScript, parse it with JSON.parse(), and access it via "." or "[]". JavaScript ... I need to parse JSON response in key value pair. Please note channel list- contain hashmap of channel name and its description
Json Data Binding Navigation And Search Functionality In
5 days ago - To serialize circular references ... which will require finding and replacing (or removing) the cyclic references by serializable values. Issue with plain JSON.stringify for use as JavaScript...
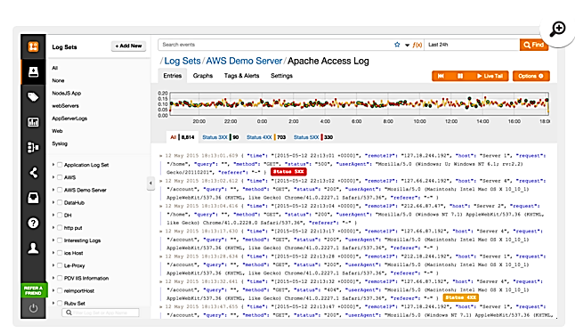
Javascript json search by value. The find () method is used to return the value of the first element that satisfies the testing function. If the value matches, then this condition is satisfied and the respective key is returned. This is the key to the value of the object. Note: This method was added in the ES6 specification and may not be supported on older browser versions. Encoding Data as JSON in JavaScript. Sometimes JavaScript object or value from your code need to be transferred to the server during an Ajax communication. JavaScript provides JSON.stringify() method for this purpose which converts a JavaScript value to a JSON string, as shown below: Stringify a JavaScript Object In JSON, the data are in key/value pairs separated by a comma,. JSON was derived from JavaScript. So, the JSON syntax resembles JavaScript object literal syntax. However, the JSON format can be accessed and be created by other programming languages too.
Any JSON file contains the key-value pair separated by the comma operator. JavaScript objects are an integral part of the React app, so they need to get accessed from JSON files/data to be uses in components. This guide will demonstrate how to get a JavaScript object from a JSON file or access it using a fetch () HTTP request. Dec 24, 2015 - Code Review Stack Exchange works best with JavaScript enabled ... By clicking “Accept all cookies”, you agree Stack Exchange can store cookies on your device and disclose information in accordance with our Cookie Policy. Given a JSON Object, the task is to check whether a key exists in Object or not using JavaScript. We're going to discuss few methods. hasOwnProperty () This method returns a boolean denoting whether the object has the defined property as its own property (as opposed to inheriting it). Syntax:
A JavaScript library to make manipulation and extraction of data from a JSON very easy and fast. JSON-FIND. The goal of the module is provide easy access to JSON or JSON-compatible* values. This is not intended for complex JSON queries, but rather for retrieving specifc values without the need to constantly reference a file's structure. In other words, if you are treating JSON as a database and making multiple queries on the same file ... Search through a JSON object using JavaScript ... Jan 31, 2019. Jan 31, 2019 ・1 min read. As my opening contribution, i leave here a method i developed to search inside a JSON object for a specific value (could be or not the name of the object property). ... {Object} obj object to be searched * @param {any} searchValue the value/key to search ...
Feb 19, 2019 - JSON object consist of curly braces ( { } ) at the either ends and have key-value pairs inside the braces. Each key-value pair inside braces are separated by comma (, ). JSON object looks something like this : ... JSON text/object can be converted into Javascript object using the function ... We are required to write a JavaScript function that takes in one such object as the first argument, a key string as the second and a value string as the third argument. The function should then check for the given key value pair in the JSON object. If there exists any object then the function should return an array of all such objects. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. JSON is a string format. The data is only JSON when it is in a string format. When it is converted to a JavaScript variable, it becomes a JavaScript object.
JSON data is stored as key-value pairs similar to JavaScript object properties, separated by commas, curly braces, and square brackets. A key-value pair consists of a key also called name (in double quotes), followed by a colon ( : ), followed by value (in double-quotes): 25/5/2020 · Now, add a view to the Index and do the following steps in your view. Now, add JavaScript. Now, your JSON file has been created. For retrieving whole data from JSON file, write the code, given below:. showData.text ('Loading the JSON file.'); For searching the users from JSON file, add a TextBox in your Index view. How to check for 'undefined' or 'null' in a JavaScript array and display only non-null values? Remove '0','undefined' and empty values from an array in JavaScript; How to get all unique values in a JavaScript array? Update 'a' record with 'b' and 'b' with 'a' in a MySQL column (swap) with only 'a' and 'b' values?
In this article, we've given you a simple guide to using JSON in your programs, including how to create and parse JSON, and how to access data locked inside it. In the next article, we'll begin looking at object-oriented JavaScript. JSON Object Literals. JSON object literals are surrounded by curly braces {}. JSON object literals contains key/value pairs. Keys and values are separated by a colon. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
JSONPath is a lightweight library to find and extract sections from a JSON data. In this page you will learn how to work with JSONPath and JavaScript. ... An array comprising either values or normalized path expressions which match the path expression supplied as input. ... JavaScript Code to find and extract various parts of the above JSON ... 7/10/2013 · dict = {} json.forEach(function(x) { dict[x.code] = x.name }) and then simply. countryName = dict[countryCode] For a list of countries this doesn't matter much, but for larger lists this method guarantees the instant lookup, while the naive searching will depend on the list size. But here, I will describe how to convert string type data into JSON data.The normally client received data into string format, that need to convert string into JSON data, JSON help to process data easily.The JSON.parse() method help to parse json data from string.The JavaScript JSON.parse() is synchronous type method that means it execution ...
JavaScript Object Notation also knew as JSON. Between server and client relationship JSON is used which is an extremely lightweight data-interchange format for data exchange and easy to parse and quick to generate. ... JavaScript provides a method that converts a JavaScript value to a JSON String by using JSON. stringify ( ) as shown in the ... JSON.parse() Function Syntax. parse() function is provided by JSON library in order to parse JSON data format. There are two parameters where one is optional. JSON.parse(text[, reviver]) text is the data the function will parse which is in JSON format.; reviver is optional parameters where If a function, this prescribes how the value originally produced by parsing is transformed, before being ... Mar 01, 2021 - Searches a JSON document or JSON compatible object for a given key.
The JSON.parse () method parses a JSON string, constructing the JavaScript value or object described by the string. An optional reviver function can be provided to perform a transformation on the resulting object before it is returned. value A value to encode. replacer Array of properties to encode or a mapping function function(key, value). space Amount of space to use for formatting. Most of the time, JSON.stringify is used with the first argument only. But if we need to fine-tune the replacement process, like to filter out circular references, we can use the second argument of JSON.stringify. We can use Object.entries () to convert a JSON array to an iterable array of keys and values. Object.entries (obj) will return an iterable multidimensional array. [ ["key1", "val1"], ["key2", "val2"], ["key3", "val3"], ] We can use this output to find our keys and values in a bunch of different ways.
4 weeks ago - A protip by steveniseki about jquery and javascript. This will find the element with the id myData. This is vanilla JavaScript and this is how we did front-end development in the "old" days :). Step 2 - Loop through every object in our JSON object. Next step is to create a simple loop. We can then get every object in our list of JSON object and append it into our main div. 8var index = students.findIndex(std=> std.id === 200); But this function is not supported by even not so old versions of a few browser as well as in IE (EDGE supports it). So below is a workaround using javascript: You can use either Array.forEach or Array.find or Array.filter. 1var students = [.
But I also find myself sometimes generating different lookup dictionaries from the same set of data, and I would still only need to iterate the data once. As for the .sort() bonus question, I would say that in terms of readability and code maintenance, yes, I would consider it one of the best ways of sorting a json collection. JSON data is written as name/value pairs, just like JavaScript object properties. A name/value pair consists of a field name (in double quotes), followed by a colon, followed by a value: "firstName": "John". JSON names require double quotes. JavaScript names do not. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Implements the IJsonValue interface which represents a JSON value. A JsonValue object can represent the three primitive JSON value types of Boolean, Number and String, and can also represent the complex value types of Array and Object by providing ways to access them. 30/7/2020 · Hi, Can you change the order of below 2 lines of code. This is just an idea and I have not tried by myself. FROM - formContext.getAttribute("msevtmgt_eventid").setValue(null); Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. Easy JavaScript - find value in nested JSON - JSFiddle - Code Playground Close
The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf (). JSON_VALUE function. JSON_MODIFY function. It returns a scalar value from JSON. We get an object or an array from the JSON. Output data type - Nvarhcar(4000) Output data type - Nvarchar(max) It returns a NULL Value if we try to return an array or object. The JSON object contains methods for parsing JavaScript Object Notation (JSON) and converting values to JSON. It can't be called or constructed, and aside from its two method properties, it has no interesting functionality of its own.
JSON parsing is the process of converting a JSON object in text format to a Javascript object that can be used inside a program. In Javascript, the standard way to do this is by using the method JSON.parse(), as the Javascript standard specifies.. Using JSON.parse() Javascript programs can read JSON objects from a variety of sources, but the most common sources are databases or REST APIs. you can get value for that JSON value as. response.data.data [0] ["username"] If you want to get all the usernames from the array, then you can do something like this: var arr = response.data.map (function (a, b) { return a.username; }); arr will contain all the username details. Muhammed Neswine. JSON Format JSON's format is derived from JavaScript object syntax, but it is entirely text-based. It is a key-value data format that is typically rendered in curly braces. When you're working with JSON, you'll likely see JSON objects in a.json file, but they can also exist as a JSON object or string within the context of a program.
How To Fetch And Display Json Data In Html Using Javascript
Extract Scalar Values From Json Data Using Json Value
Inspect The Json From The Response Payload Documenting Apis
Working With Json Data In Python Python Guides
Js Find Value In Object Code Example
Postman Extract Value From The Json Object Array By Knoldus
Composing Cts Query Expressions Search Developer S Guide
How To Create Javascript List Filter And Search
Want To Analyse Your Tweets How To Import Twitter Json Data
Indexing Querying And Full Text Search Of Json Documents
Extract Scalar Values From Json Data Using Json Value
Never Confuse Json And Javascript Object Ever Again By
Live Search Json Data Using Ajax Jquery Webslesson
How To Read Local Json File In React Js By Rajdeep Singh
What Is A Json File Example Javascript Code
Jsoniq The Json Query Language
Filtering Json Array In Javascript Code Example
Removing Parameters Outputted In Json Response How To Remove
Extract Scalar Values From Json Data Using Json Value
An Introduction To Json Digitalocean
How To Parse Json Data With Python Pandas By Ankit Goel
Github Josdejong Jsoneditor A Web Based Tool To View Edit
How To Swap Key And Value Of Json Element Using Javascript
Convert Json Data Dynamically To Html Table Using Javascript
How To Store Query And Create Json Documents In Oracle Database
Load Data From Json To Redshift 2 Easy Methods
What Is Json Amazon Web Services
0 Response to "30 Javascript Json Search By Value"
Post a Comment