25 How To Remove Duplicate Values From Array In Javascript
Remove duplicates using a hash-map. In our brute force solution, we loop through the array twice, what if we were to loop through just once? We can do this if we use an object to keep track of key value pairs, i.e. our "hash-map". The "key" is the element in the array, and the "value" is either true or falsy (undefined). Alternatively, you can use the newly introduced ES6 for-of loop instead of for loop to perform this filtration very easily, as demonstrated in the following example:
Remove Duplicate Values From Array Javascript Simple Code
In the above program, Set is used to remove duplicate items from an array. A Set is a collection of unique values.
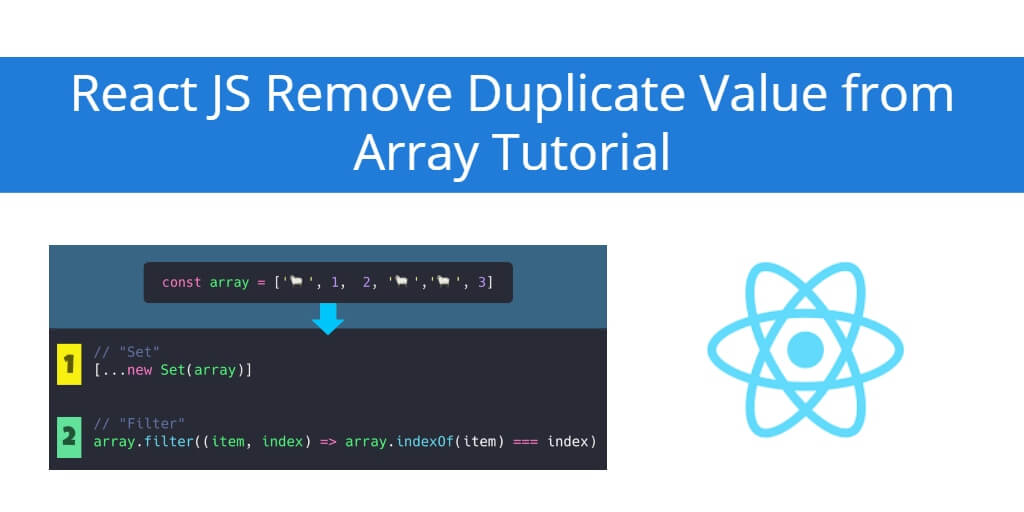
How to remove duplicate values from array in javascript. Basic Javascript: Removing Duplicates from an Array # beginners # programming # ... For this week, I wanted to come up with multiple ways to remove duplicates within an array. 1. filter() function removeDuplicates (array) {return ... using the reduce function to produce a total based on an array of values, we could start the reduce loop ... Jan 20, 2019 - Here are three ways to filter out duplicates from an array and return only the unique values. My favorite is using Set cause it’s the shortest and simplest 😁 It is quite common to use Set to remove duplicate items from an array. This can be achieved by wrapping an existing array into Set constructor and then transforming it back into array: const arr = [1, 2, 'a', 'b', 2, 'a', 3, 4]; const uniqueArr = [...new Set(arr)]; console.log(uniqueArr); This works great for arrays of primitive values, however ...
Using.filter () and.indexOf () removes the duplicate array values in a succinct and readable manner, allowing us to get all of the unique values in the array without much overhead. Finding Duplicated Values in an Array In some cases, we may want to find all of the values in an array that occur more than once. Test your JavaScript, CSS, HTML or CoffeeScript online with JSFiddle code editor. In such a case you can use the JavaScript code to remove duplicates from array of objects. The remove Duplicates from Array of Items method takes a standard object as input. You will be required to enter the dimensions and types of each item into the text box. The code then converts the dimension and type of the item into String data.
Using indexOf () method JavaScript offers the indexOf () method, by which we can find the index of element and then remove the duplicate values from the given array. The indexOf () method searches for the specific element within the array and returns its value. This method returns the index of the first occurrence of an element within the array. Another thing worth nothing is that we can use the same method to return only the duplicate values by inverting the comparison: let duplicateArray = originalArray.filter((item, index, array) => { return array.indexOf(item) !== index }) Using Array.prototype.reduce () & Array.prototype.includes () The below examples takes an input array of objects or primitive types in an array, delete the duplicate items from an array, return the new array without duplicates. if an array contains primitive types, it is very easy to filter Duplicates if an array contains objects, We need to filter the duplicates based on key and value pair equal validation.
May 25, 2015 - Just remove the uneval (it creates ... an Array from the Set. (i.imgur /GuwtZnT.png). It will take a lot of time before we can use it every day, but it's nice to see this in Javascript. (well, it's still a bad language.) \$\endgroup\$ ... Here's an approach that utilises the fact that an object can also be used as a hashmap. The value of a is not ... Read: How to delete values from an array in Javascript To remove duplicate elements, we use the function Array.indexOf which returns the first index of the array where a given element is found. let data = [11,3,4,7,3,11,45,7]; let result = data.filter ((item,index)=> { // filter javascript array I needed to ensure the reducer was not adding duplicate data to state. Normally, I would utilize a Set to remove any duplicate values, but the data returned from my API was an array of JavaScript objects. Set can remove any duplicates of the same object within an array but cannot detect that different objects have identical keys and values.
In vanilla JavaScript, there are multiple ways available to remove duplicate elements from an array. You can either use the filter () method or the Set object to remove all repeated items from an array. Let us say that we have the following array that contains duplicate elements: const numbers = [1, 2, 3, 2, 4, 4, 5, 6]; Removing duplicates from an array in JavaScript can be done in a variety of ways, such as using Array.prototype.reduce(), Array.prototype.filter() or even a simple for loop. But there's an easier alternative. JavaScript's built-in Set object is described as a collection of values, where each value may occur only once. Javascript has a built-in Object Set which is the best approach to remove duplicates from javascript array because it only keeps unique values. Set object available in ES6. But there are also other approaches to get a unique or distinct array in js. which we are going to implement in this post. You can also check :
Anyone of the object properties can be used as a key. The key is extracted from the object and used as the index of the new temporary array. The object is then assigned to this index. This approach will remove the duplicate objects as only one of each object of the original array will get assigned to the same index. Instead of a delete method, the JavaScript array has a variety of ways you can clean array values. You can remove elements from the end of an array using pop, from the beginning using shift, or from the middle using splice. The JavaScript Array filter method to create a new array with desired items, a more advanced way to remove unwanted elements. Jul 07, 2020 - It’s a common use case in many projects to need to remove duplicates from an array in JavaScript. For interviews, it’s a common coding challenge to practice for everyone. Here are seven ways to filter out duplicates from an array and return only the unique values.
To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Then, convert the set back to an array. Jul 01, 2019 - Last week, we learned how to merge two or more arrays together with vanilla JS. One potential challenge with this approach is that it can result in duplicates in your array. Today, let’s learn how to remove duplicates from an array. The technique This one is actually really simple, thanks ... Here are 3 ways to filter out duplicates from an array and return only the unique values...
How to find and remove duplicates in a JavaScript array Published Nov 16, 2020 If you want to remove the duplicates, there is a very simple way, making use of the Set data structure provided by JavaScript. The purpose of this article is to share with you the best ways to remove duplicate objects from JavaScript Array based on specific property/key. We will also analyze their performance in terms of execution time for different lengths of the array. If you want to learn how to remove duplicate PRIMITIVE values from JavaScript array check this article. How to remove duplicate property values in array - JavaScript? Let's say the following is our array −. We need to remove the duplicate property value i.e. 87 is repeating above. We need to remove it. For this, use the concept of map ().
May 31, 2016 - We work on arrays all the time and one of the most frequent operations that we perform on arrays is removing duplicates. ... In this post, we will learn how to remove duplicate values from a JavaScript array. We will be discussing various ways to get unique values out of Arrays using the latest ES6 TypeScript method. JavaScript has a number of methods to operate on Arrays, further, we will discuss the best combination of these different types of JavaScript functions ... Let's look at two ways to remove them. Using the Array.filter() method. The Array.filter() method creates a new array with only elements that pass a test you include as a callback function.. We can use it to remove the duplicates. On each iteration, we'll use Array.indexOf() to see if our item already exists. If the returned index is smaller than the current index, that means an instance ...
Mar 12, 2020 - By initializing a Set with a ... we pass values and Set automatically removes the duplicates. Then we convert it to an array by wrapping it into square brackets []. This method works with anything that’s not an object: numbers, strings, booleans, symbols. Download my free JavaScript Beginner's ... Remove duplicate values from Javascript array - How to & Performance comparison Posted by Viktor Borisov The purpose of this article is to share with you the best ways to remove duplicate PRIMITIVE(strings, numbers and booleans) values from an array in Javascript and compare their performance in terms of execution time. Vanilla JS: Remove duplicates by tracking already seen values (order-safe) Or, for an order-safe version, use an object to store all previously seen values, and check values against it before before adding to an array.
Assuming that you have a JavaScript array with duplicates, you can remove the duplicates using uniq method as shown: var arr = ['a','b','c','a','b'] console.log('unique arra is ',_.uniq(arr)) The uniq method returns an array after removing duplicate elements from the passed in array. First Method - Remove duplicate objects from array in JavaScript Using new Set () You can use the new set () method to remove the duplicate objects from an array in javascript. We have objects of the array in javascript. And it has contains duplicate objects. Feb 01, 2018 - Learn multiple ways to remove duplicates from an array in JavaScript.
Nov 21, 2010 - JS: How to remove duplicate items from an Array? I guess there will be a simple way ... There are various different methods for finding the duplicates in the array. We will discuss two ways for finding the duplicates in the array. Using Set: The Set object lets you store unique values of any type, whether primitive values or object references. This is the easiest method to remove the duplicate element and get unique elements from ... In this tutorial, you will see how to remove duplicate values from a JavaScript array with simple and quick examples of JS code. First of all, I would like to let you know that, there are multiple ways are there to do this task. Here you are going to see two different ways to remove duplicates from an array.
7 Ways To Find And Remove Duplicate Values In Microsoft Excel
Program To Remove Duplicate Elements In An Array C Programs
7 Ways To Remove Duplicates From An Array In Javascript By
How To Remove Duplicate Values In An Array In Javascript
Remove Duplicate Values From Array In Javascript
Algodaily Remove Duplicates From Array Description
Learn Javascript Remove Duplicate Elements From An Array Facebook
How To Remove Duplicates From An Array In Javascript Hello
Remove Duplicates In A Javascript Array Using Es6 Set And
Remove Duplicate Values From An Array In Javascript Clue
Remove Duplicates From Array In Javascript
Java Program To Remove Duplicate Elements In An Array In
Javascript Remove Duplicates From Sorted Array In Place By
Remove Duplicate Elements From An Array Using Angularjs
Java Exercises Remove The Duplicate Elements Of A Given
React Js Remove Duplicate Value From Array Tutorial Tuts Make
C Program To Remove Duplicates From Array In C Qa With
How To Remove Array Duplicates In Es6 By Samantha Ming
Removing Duplicate Arrays From An Array Of Arrays
How To Remove Duplicates In Excel
6 Ways To Remove Duplicates From An Array In Javascript Es6
Youvcode Knowledge Shared 2 X Knowledgehow To
How To Remove Duplicate Objects From An Array In Javascript
Remove Elements From A Javascript Array Geeksforgeeks
0 Response to "25 How To Remove Duplicate Values From Array In Javascript"
Post a Comment