24 Javascript Print Json Object To String
I've got a javascript object which has been JSON parsed using JSON.parse I now want to print the object so I can debug it (something is going wrong with the function). ... when combined with a string like this: console.log("object: " + obj) it does not display the object, but instead will output "object: ... Today I am sharing an example here, which shows how to convert a JSON string data to a JSON object in JavaScript.
How To Use Gson Gt Fromjson To Convert The Specified Json
The JSON.stringify() method accepts up to three parameters: the JSON object, a replacer, and space. Only the JSON object is required. The remaining two parameters are optional. If you skip the optional parameters when calling JSON.stringify(), the output JSON string will not include any spaces or line breaks. This makes it hard to read the ...
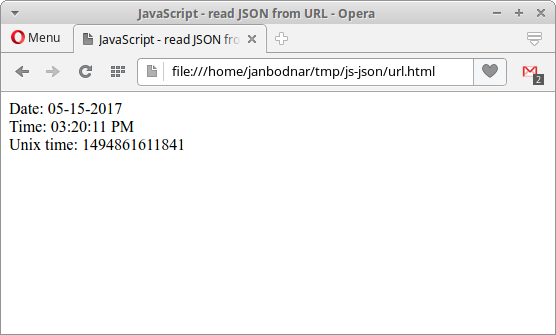
Javascript print json object to string. JSON.stringify() converts a value to JSON notation representing it: If the value has a toJSON() method, it's responsible to define what data will be serialized.; Boolean, Number, and String objects are converted to the corresponding primitive values during stringification, in accord with the traditional conversion semantics.; undefined, Functions, and Symbols are not valid JSON values. It's a standard text-based format which shows structured data based on JavaScript object syntax. It is commonly used for transmitting data in web applications. JSON is highly recommended to transmit data between a server and web application. To convert a Java object into JSON, the following methods can be used: 1 week ago - The JSON.parse() method parses a JSON string, constructing the JavaScript value or object described by the string. An optional reviver function can be provided to perform a transformation on the resulting object before it is returned.
Use the JavaScript function JSON.stringify () to convert it into a string. const myJSON = JSON.stringify(obj); The result will be a string following the JSON notation. myJSON is now a string, and ready to be sent to a server: Example. const obj = {name: "John", age: 30, city: "New York"}; const myJSON = JSON.stringify(obj); JavaScript Object Notation (JSON). It is a lightweight data transferring format. It is very easy to understand by human as well as machine. It is commonly used to send data from or to server. Nowadays it is widely used in API integration because of its advantages and simplicity. The main difference between a JSON object and a regular JavaScript object - also called an object literal - comes down to the quotation marks. All the keys and string type values in a JSON object have to be wrapped in double quotation marks ("). JavaScript object literals are a bit more flexible.
The JSON.stringify() method is used to print the JavaScript object. JSON.stringify() Method: The JSON.stringify() method is used to allow to take a JavaScript object or Array and create a JSON string out of it. While developing an application using JavaScript many times it is needed to serialize the data to strings for storing the data into a ... Full disclosure I am the author of this package but another way to output JSON or JavaScript objects in a readable way complete with being able skip parts, ...12 answers · Top answer: Please use a <pre> tag demo : http://jsfiddle /K83cK/ var data = { "data": { "x": "1", ... The JSON.stringify() method converts an object or value to a JSON string. JSON.stringify skips some JavaScript-specific objects, such as properties storing undefined, symbolic properties, and function properties. The toString( ) method is called without arguments and should return a string. The string you return should be based on the value of ...
If you want to create a JSON string representation of your JavaScript object, make use of the JSON.stringify() function. JavaScript Object Notation (JSON) is a lightweight data interchange format. It is commonly used to exchange data with a webserver. When data is sent to a server it must be sent as a string. JSON.stringify() method converts JavaScript data to a JSON-formatted string. It is usually applied to JS objects to produce a ready-made JSON string to be ... In this tutorial I will show you how you can pretty print a JSON string. It is a one liner, but it makes all the difference. Normally when we use JSON.stringify, we only want to serialise a JSON object, but sometimes after we serialise it, we might want to do debugging, or maybe we want to store the JSON with indentation.. JSON.stringify can take 3 arguments.
I wouldn't normally do it this way but its for a practice exercise to convert an object without using JSON.stringify(). The object to convert is below: obj = { num: 0, string: "string", func: 13 Feb 2018 · 9 answersconsole.log(JSON.stringify(data)) will do what you need. ... The value from input_data Object will be typeof "1" : String , but you can ... “print json data in javascript” Code Answer's. turn object into string javascript. javascript by Vbbab on Mar 19 2020 Comment.
Every object has a toString() method that is automatically called when the object is to be represented as a text value or when an object is referred to in a manner in which a string is expected. By default, the toString() method is inherited by every object descended from Object.If this method is not overridden in a custom object, toString() returns "[object type]", where type is the object type. Given a JavaScript object and the task is to print the JSON object in pretty ( easy to read) format using JavaScript. Use <pre> element to display the object in pretty format. Approach: Declare a JSON object and store it into variable. Use JSON.stringify(obj) method to convert JavaScript objects into strings and display it. How to convert a string to JavaScript object? ... How to convert a JavaScript date object to a string? Javascript Map vs Object — What and when? HTML DOM Map Object; How to convert a JSON string into a JavaScript object? Convert an object to another type using map() method with Lambda in Java? Previous Page Print Page. Next Page ...
JSON StringifyThe JSON.stringify() method converts a JSON-safe JavaScript value to a JSON compliant string. What are JSON-safe values one may ask! Let's make a list of all JSON-unsafe values and anything that isn't on the list can be considered JSON-safe. JSON-unsafe values:undefinedfunction(){}(ES6+) SymbolAn object with This is an old post but I'm chiming in because (as Narem briefly mentioned) a few of the printf-like features are available with the console.log formatters.In the case of the question, you can benefit from the string, number or json formatters for your data. In the above example, the JSON.stringify() method is used to convert an object to a string. The typeof operator gives the data type of the result variable. Example 2: Convert Object to String Using String()
The JSON.parse () method parses a string and returns a JavaScript object. The string has to be written in JSON format. The JSON.parse () method can optionally transform the result with a function. Sep 19, 2019 - JSON means JavaScript Object Notation. This is one of the reasons why pretty-printing is implemented natively in JSON.stringify(). The third argument in it pretty prints and sets the spacing to use − 5 Ways to Convert a Value to String in JavaScript. ... // ⚠️ JSON.stringify(string); ... (foo) but I don't like this method (it will create an object of String, in contrast to String ...
console object has log function which prints the an object or any javascript variable. console.log ("employee Object :"+obj); This will prints the object metadata as in the form of an string. However, This is not useful to debug and know the object content values. output : employee Object :[object Object] if you add comma (,) to log function ... Feb 24, 2020 - If you have a string, you need to JSON.parse(...) first – Vihung Aug 5 '14 at 12:40 ... User Pumbaa80's answer is great if you have an object you want pretty printed. If you're starting from a valid JSON string that you want to pretty printed, you need to convert it to an object first: Let's check out the different ways of converting a value to a string in JavaScript. The preferred way from Airbnb's style guide is String()...
A String or Number object that's used to insert white space into the output JSON string for readability purposes. Start with an object. const data = { color: 'red', wheels: 4, engine: { cylinders: 4, size: 2.2 } } To convert it to JSON and pretty print. JSON.stringify(data, null, 2) Dec 15, 2020 - The JSON.stringify() method converts a JavaScript object or value to a JSON string, optionally replacing values if a replacer function is specified or optionally including only the specified properties if a replacer array is specified. Sep 19, 2020 - A quick reference for the parse and stringify methods of the JSON object.
Feb 19, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. To print the JSON output beautifully (aka pretty-print), you can use the JSON.stringify() method and pass a spacing value (or indentation) as the third argument to the function in JavaScript. TL;DR /* Pretty print JSON output ️ */ // Define a white-space value // as the third argument in the function JSON.stringify(obj, null, 4); May 14, 2018 - Convert String to JSON Object using Javascript is an essential task if you are working heavily on JavaScript-based applications. Developer faces many issues when they begin working with JSON and JavaScript in the beginning stage and this kind of solution is very handy.
In JavaScript, we have a JSON.stringify () method which is used to convert an object into a string. Consider we have a JavaScript object like: var user = { firstName : 'Ramesh' , lastName : 'Fadatare' , emailId : 'ramesh@gmail ' , age : 29 } Now we need to convert the above object into a string by using JSON.stringy () method. var obj = {a: "a", b: "b" /*...*/}; var string = JSON.stringify(obj); // OUTPUT: // "{'a':'a', 'b':'b'}" Nov 19, 2020 - JSON.stringify() takes a JavaScript object and then transforms it into a JSON string.
Aug 18, 2020 - There are so many programming languages, and every language has its own features. But all of them have one thing in common: they process data. From a simple calculator to supercomputers, they all work on data. It's the same with humans: there are so many countries, so many cultures, and JS Examples JS HTML DOM JS HTML Input JS HTML Objects JS HTML Events JS Browser JS Editor JS Exercises JS Quiz JS Certificate ... A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse(), and the data becomes a JavaScript ... Nov 15, 2020 - The JSON (JavaScript Object Notation) ... and objects. It is described as in RFC 4627 standard. Initially it was made for JavaScript, but many other languages have libraries to handle it as well. So it’s easy to use JSON for data exchange when the client uses JavaScript and the server is written on Ruby/PHP/Java/Whatever. ... JSON.stringify to convert ...
JSON string and JS object. ... JSON. parse(), ... Convert this JS object into a JSON object. What does JavaScript’s JSON object do to JSON data and what does it convert a JavaScript object into? You can use the JSON.stringify() method to easily convert a JavaScript object a JSON string. The JSON data contains the key-value pair as string values; thus, sometimes the data may in a string format and need to send over API call. The data should be formatted to send the request data from the client to the server, and the response will be mostly in the JSON format, but you can convert the string to JSON using the function stringify().The basic syntax of the function stringify() is ...
Converting JSON objects into strings can be particularly useful for transporting data in a quick manner. We've gone over the general format of JSON and how you may expect to see it as a .json file, or within JavaScript as an object or a string. Comparison to JavaScript Object This will encode the object to a string in both the web browser and Node.js. In order to get a valid string of JSON, you need to pass the JavaScript object to the JSON.stringify method. As you can ... If an object being stringified has a property named toJSON whose value is a function, then the toJSON() method customizes JSON stringification behavior: instead of the object being serialized, the value returned by the toJSON() method when called will be serialized. For example:
Modifying Json Data Using Json Modify In Sql Server
How To Work With Json In Javascript Digitalocean
How To Read And Write A Json Object To A File In Node Js
Parse Json And Store Json Data Using Node Js Codez Up
How To Convert Html Form Field Values To A Json Object
Json Handling With Php How To Encode Write Parse Decode
How To Print Json Object Or Convert It To An Array Ionic V3
Working With Json Data In Python
Python Json Dump And Dumps For Json Encoding
Python Json Encode Dumps Decode Loads Amp Read Json File
How To Pretty Print Beautify A Json String Our Code World
Python Pretty Print Json Journaldev
Clone Javascript Object With Json Stringify By Mayank Gupta
How Can I Pretty Print Json In A Shell Script Stack Overflow
Javascript Reading Json From Url With Fetch Api Jquery
How To Convert Json String To Array Of Json Objects Using
Convert Json Data Dynamically To Html Table Using Javascript
Inspect The Json From The Response Payload Documenting Apis
How To Fetch And Display Json Data In Html Using Javascript
Never Confuse Json And Javascript Object Ever Again By
Esp32 Arduinojson Printing Prettified Json String
0 Response to "24 Javascript Print Json Object To String"
Post a Comment