26 How To Use Document In Javascript
26 rows · document.createElement(element) Create an HTML element: document.removeChild(element) Remove an HTML element: document.appendChild(element) Add an HTML element: document.replaceChild(new, old) Replace an HTML element: document.write(text) Write into the HTML output stream JavaScript can access all the elements in a webpage making use of Document Object Model (DOM). In fact, the web browser creates a DOM of the webpage when the page is loaded. The DOM model is created
Ios Pdf Viewer With Javascript Pspdfkit Sdk
document is a variable that comes with JavaScript (at least in the browser context). Instead, try the following for the relevant line. $ (document).ready (function () { You'll also want to take the onLoad attribute off of the body tag, else it will run twice.
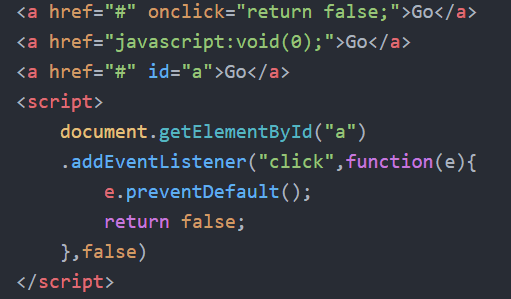
How to use document in javascript. Code included inside $ (document).ready () will only run once the page Document Object Model (DOM) is ready for JavaScript code to execute. Code included inside $ (window).on ("load", function () {... }) will run once the entire page (images or iframes), not just the DOM, is ready. 1 2 The way a document content is accessed and modified is called the Document Object Model, or DOM. The Objects are organized in a hierarchy. This hierarchical structure applies to the organization of objects in a Web document. Window object − Top of the hierarchy. It is the outmost element of the object hierarchy. Use DOM methods such as getDocumentById (), querySelector () to select a <form> element. The document.forms [index] also returns the form element by a numerical index. Use form.elements to access form elements. The submit event fires when users click the submit button on the form.
Display JavaScript variable using document.write () method The document.write () method allows you to replace the entire content of HTML <body> tag with HTML and JavaScript expressions that you want to be displayed inside the <body> tag. Suppose you have the following HTML element: <body> <h1>Hello World</h1> <p>Greetings</p> </body> Definition and Usage The write () method writes HTML expressions or JavaScript code to a document. The write () method is mostly used for testing: If it is used after an HTML document is fully loaded, it will delete all existing HTML. 1/8/2019 · JSDoc is the most popular Javascript documentation generator. All you need to do is to simply run jsdoc command with a filename as an argument. That is it. It will generate HTML file with documentation that is ready to use. The website is https://github /jsdoc/jsdoc. Docusaurus
A web page is essentially a document represented by the DOM as nodes and objects. It allows programs to manipulate the document's content, structure, and styles. In this tutorial, we will learn how to use JavaScript to access different nodes (HTML elements) in the DOM. Let us start with the first method: getting element by ID. Get Element By ID Introduction to JavaScript querySelector() and querySelectorAll() methods. The querySelector() is a method of the Element interface. The querySelector() allows you to find the first element that matches one or more CSS selectors. You can call the querySelector() method on the document or any HTML element. Using it along with other measures, such as xsrf tokens will add an extra layer to your protection. Cookie Functions¶ There is a small but convenient set of functions for working with cookies. It is handier than a manual modification of the document.cookie. The shortest way to access cookies is by using regular expressions. getCookie(name)¶
The more common "angular way" of hiding/showing DOM elements is to use the ngHide and/or ngShow directives -- "declare" them in your HTML (hence this statement on the Overview page: . Angular is built around the belief that declarative code is better than imperative when it comes to building UIs and wiring software components together In this JavaScript tutorial you will learn how to change CSS using JavaScript as well as creating CSS. CHECK OUT THESE AWESOME PEOPLE!Daniel SimionescuMeet ... Welcome to a short tutorial on how to use the Javascript document fragment. Congratulations code ninja, you have found one of the underrated top-secrets in Javascript. The document fragment is a lightweight container for holding HTML elements, an alternative to directly inserting elements into the DOM.
If you save the above HTML file, and load it into the browser of your choice, you should see a blank page with the title of the page as Today's Date. You can then open up the Console and begin working with JavaScript to modify the page. We'll begin by using JavaScript to insert a heading into the HTML. let d = new Date (); 17/11/2020 · How to use document.write() in JavaScript. The document.write() function is commonly used when testing simple web applications or when learning JavaScript. document.write() will print the contents of the parameter passed to it to the current node at execution time. Here is a simple example: <script>document.write("Some Text")</script> JavaScript - Document Object Model or DOM. Every web page resides inside a browser window which can be considered as an object. A Document object represents the HTML document that is displayed in that window. The Document object has various properties that refer to other objects which allow access to and modification of document content ...
28/5/2019 · All we will need to create a blank word document is the Document and Packer features from the docx module and the saveAs feature from file-saver module. import { Document, Packer } from "docx" import { saveAs } from "file-saver" Next add an event listener that is listening for clicks on the button we created in Step 3. Using the document.execCommand function in Javascript to style, modify and insert content within a content editable container. Learn how to use Javascript's document.execCommand function which allows you to style, modify and insert content of a content editable container Go to your test site and create a new folder named scripts. Within the scripts folder, create a new file called main.js, and save it. In your index.html file, enter this code on a new line, just before the closing </body> tag:
JavaScript appendChild(): Examples. 1) Simple example // Create a new paragraph element, and append it to the end of the document body let p = document.createElement("p"); document.body.appendChild(p); 2) How to use the appendChild() method There is a client-side option for exporting HTML to a word document using JavaScript. Client-side export to Doc features makes the web application easy to use. The user can export a particular portion of the material of the web page without refreshing the site. In this tutorial, we'll explain to you how to export HTML to a JavaScript doc. Moreover, the document object provides various properties and methods to access and manipulate the web elements loaded on the page. To identify and access the DOM elements, JavaScript uses three ways: First, Accessing elements By ID. Second, Accessing elements By TagName. Third, Accessing elements By className.
How to document your code # There's a standard approach to JS documentation known as JSDoc. It follows a standard format. /** * [someFunction description] * @param { [type]} arg1 [description] * @param { [type]} arg2 [description] * @return { [type]} [description] */ var someFunction = function (arg1, arg2) { // Do something... The Document.write () method writes a string of text to a document stream opened by document.open (). Note: Because document.write () writes to the document stream, calling document.write () on a closed (loaded) document automatically calls document.open (), which will clear the document. JavaScript is especially useful when you want to take user information and process it without sending the data back to the server. JavaScript is much faster than sending everything to the server to process, but you must be able to read user input and use the right syntax to work with that input.
Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement () to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the document: complete — the document and all sub-resources have finished loading, and the load event is about to fire. Tip: Use document.readyState === "complete" to detect when the page is fully loaded i.e stylesheets, images, frames etc. Read more about onreadystatechange on MDN. 3. Using JavaScript Timer The document.getElementById () method returns the element of specified id. In the previous page, we have used document.form1.name.value to get the value of the input value. Instead of this, we can use document.getElementById () method to get value of the input text. But we need to define id for the input field.
Document writeln () Method It is different from write () method, writeln () method writes text with the newline after each statement.
Controlling Active Technologies With Custom Javascript Code
Code Documentation For Javascript With Jsdoc An Introduction
1 Writing Your First Javascript Program Javascript
Javascript Document Object Studytonight
How To Use The Document Viewer In Javascript With React
Developing Acrobat Applications Using Javascript Js Developer
Github Devexpress Examples Reporting How To Use The Web
Writing Documentation For Your Javascript Project Flatlogic
Handling Common Javascript Problems Learn Web Development Mdn
How Javascript Interacts With Your Browser By Apuck003 Medium
Javascript Dom Tutorial With Example
How To Use Javascript Document Fragment A Simple Example
Javascript And The Document Object Model
Intervening Against Document Write Web Google Developers
Document Object Model Wikipedia
Enter Full Screen Mode With Javascript By Aiman Rahmat
Should I Use Or Javascript Void 0 On The Href
Creating A Dynamic Html Table With Javascript By Daniel
What Is Javascript Learn Web Development Mdn
Pdf Working With Getelementsbytagname In Javascript
Vanilla Js Addeventlistener Queryselector And Closest By
How To Code Adobe Javascript How To Code Pdf Javascript
0 Response to "26 How To Use Document In Javascript"
Post a Comment