29 Javascript Remove From Object
Deleting is the only way to actually remove a property from an object. Setting the property to undefined or null only changes the value of the property. It does not remove property from the object. JavaScript object operations - How to add / remove item from a JavaScript object Getting into JavaScript frameworks like vue.js and react.js, now it's time to review some of the JavaScript fundamentals.
Remove Item From An Array Of Objects By Obj Property By
Mar 27, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
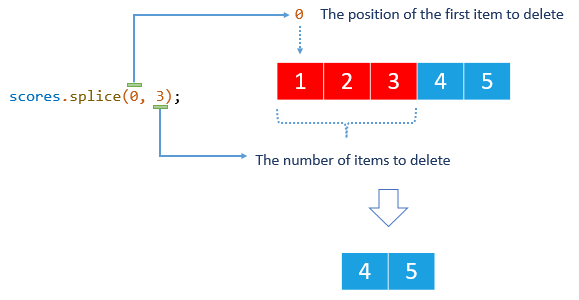
Javascript remove from object. That is actually a JavaScript object, associative arrays do not exist in JavaScript. - alex Aug 11 '10 at 5:05 2 Yeah just some confusion with terminology I think, ie it's Javascript not Jquery, and it's an object not array (OP may come from other languages with associative arrays). - thomasrutter Aug 11 '10 at 5:12 This will remove all objects with an id of 0.. It also creates a copy of the array and does not modify the original array. This is why we need to reassign the output. If you'd like to modify the array in place, then you might have to resort to good ol' for loops. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Javascript delete is an inbuilt operator that removes the property from the object. The delete operator removes both the value of the property and the property itself. If there are no more references to the same property, then it is eventually released automatically. The delete operator also has the return value. Feb 11, 2021 - The only way to fully remove the properties of an object in JavaScript is by using delete operator. If the property which you’re trying to delete doesn’t exist, delete won’t have any effect and can return true. ... The delete operator removes a property from an object. how to remove a property from an object in javascript
javascript has various methods like, new Set (), forEach () method, for loop, reduct (), filter () with findIndex () to remove duplicate objects from javascript array. In the below, we will demonstrate to you javascript methods with examples for removing duplicate objects from the array. 6 days ago - In this tutorial, we'll go over how to remove a property from a JavaScript object. We'll cover the delete operator, as well as the {...rest} syntax and reduce() method. Method 2: Converting the array to a Set to remove the duplicates: A Set object holds only unique values of any type. This property can be used to store only the objects that are unique in the array. Each object of the array is first converted into a JSON encoded string using JSON.stringify method. The JSON encoded string is then mapped to an ...
Using Delete Operator This is the oldest and most used way to delete property from an object in javascript. You can simply use the delete operator to remove property from an object. If you want to delete multiple properties, you have to use the delete operator multiple times in the same function. May 20, 2020 - javascript how-do-i-remove-a-property-from-a-javascript-object Before destructuring, we would typically use the delete keyword to remove properties from an object. The issue with delete is that it's a mutable operation, physically changing the object and potentially causing unwanted side-effects due to the way JavaScript handles objects references.
Jul 19, 2019 - how to remove a property from an object in javascript Use the splice () Method to Remove an Object From an Array in JavaScript The method splice () might be the best method out there that we can use to remove the object from an array. It changes the content of an array by removing or replacing existing elements or adding new elements in place. The syntax for the splice () method is shown below. Objects in JavaScript can be thought of as maps between keys and values. The deleteoperator is used to remove these keys, more commonly known as object properties, one at a time. var obj = { myProperty: 1
Aug 16, 2020 - Learn how to delete object properties in JavaScript Delete property from the JavaScript object using ES6 feature destructuring . Destructuring is a JavaScript ECMAScript 6 version new features, it is an easy way of extracting or removing values from data stored such as objects and Arrays. we will learn about Destructuring feature in the ES6 Destructuring chapter. One traditional way of removing an item from JavaScript array is by using the built-in splice method. And that's what we did here. Last argument is set to 1 to make sure we only delete 1 item. You can specify any number here, but in this case we only need to delete 1.
Use the delete Operator to Remove a Property From an Object in JavaScript. One method to remove any of the properties of any object is the delete operator. This operator removes the property from the object. For example, we have an object myObject with the properties as id, subject, and grade, and we need to remove the grade property. JavaScript. Jul 20, 2021 - The JavaScript delete operator removes a property from an object; if no more references to the same property are held, it is eventually released automatically. The delete operator is designed to remove properties from JavaScript objects, which arrays are objects. The reason the element is not actually removed from the array is the delete operator is more about freeing memory than deleting an element. The memory is freed when there are no more references to the value. Clear or Reset a JavaScript Array
The delete () method removes a specified value from a Set object, if it is in the set. The pop() and shift() methods change the length of the array.. You can use unshift() method to add a new element to an array.. splice()¶ The Array.prototype.splice() method is used to change the contents of an array by removing or replacing the existing items and/or adding new ones in place. The first argument defines the location at which to begin adding or removing elements. another thing i want, There will be separate buttons for every object in array. if i want to delete that particular object in the array button clicked. how to do it . i have used angular js ng-repeat to generate items. can you help me - Thilak Raj Jan 21 '16 at 4:32
Jan 13, 2021 - To remove an object key from a JavaScript object, you can’t use the assignment operator (=). Instead, you need to learn the rules for the… Jun 24, 2021 - This tutorial covers multiple ways of removing property from an object in javascript with examples ES6 delete key in object examples - using delete … how to remove a property from an object in javascript
Apr 28, 2021 - This post will discuss how to remove a property from an object in JavaScript... The delete operator removes a given property from an object, returning true or false as appropriate on success and failure. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Get code examples like "remove object from object javascript" instantly right from your google search results with the Grepper Chrome Extension.
Deleting a property of an object with ES5 JavaScript The delete functionality of properties is also given in ES5 JavaScript. The keyword to use is delete and can be used similar to what the adding of a property looks like. 1 In JavaScript, there seems to be an easy way to pretty much anything (disagree with me in the comments :manshrugging:). One of the things that _isn't so easy is removing duplicate objects from a JavaScript array of objects. In this quick tip, I'll provide you with a simple function that will return an array of JavaScript objects with duplicates ... The delete operator is designed to be used on object properties. It has no effect on variables or functions. Note: The delete operator should not be used on predefined JavaScript object properties. It can crash your application.
You can only delete a Property or Method of an object using the Delete Operator. It will completely remove the Property from the collection. Delete will not delete the regular variables or functions You can only delete the property owned by the object. There are various ways to remove a property from a JavaScript object. Find out the alternatives and the suggested solution. Published May 22, 2018. The semantically correct way to remove a property from an object is to use the delete keyword. Given the object. const car = { color: 'blue', brand: 'Ford'} JavaScript array splice() Javascript array splice() is an inbuilt method that changes the items of an array by removing or replacing the existing elements and/or adding new items. To remove the first object from the array or last object from the array, then use the splice() method.
Try the demo. delete employee[name] removes the property which name is contained inside name variable. 2. Object destructuring with rest syntax. Another approach to removing properties, but in an immutable manner without altering the original object, is to use the object destructuring and rest syntax.. The idea is simple: destructure the object to the property you want to remove, and the ...
Solved Remove All Object Styles Paragraph Styles Charac
Javascript Remove Object From Array If Value Exists In Other
Prevent Duplicate Objects In Array Javascript Code Example
How To Remove Object From Array Of Objects Using Javascript
Javascript Remove Object From Array By Value
Drag And Remove An Object From A Group Using Javascript
Javascript Remove Object Property Anjan Dutta
Remove A Property Of An Object Immutably In Redux Javascript
Remove Duplicates From An Array In Javascript Without Using
How To Remove A Property From A Javascript Object
Restrict An Object In Javascript Maxim Orlov
Github Jojonki Js Remove Property Remove Specific
Javascript Array Splice Delete Insert And Replace
How To Remove A Key From An Object In Javascript Latest
Javascript Remove Object From Array Pakainfo
How To Remove A Property Of Javascript Object Rustcode
Affinity Photo Remove An Object From A Photo
How To Remove A Property From An Object In Javascript
How To Remove Object From Array Of Objects Using Javascript
How To Remove Property From Javascript Object Tecadmin
How To Remove A Property From A Javascript Object
Top 4 Methods On How To Remove Objects In Photoshop
Help On Remove Duplicate Object Within For Loop Javascript
Remove Duplicate Array Object Javascript Code Example
Remove Elements From A Javascript Array Geeksforgeeks
How To Immutably Remove Property From Javascript Object
Remove Object From An Array Of Objects In Javascript
Python Object Tutorial How To Create Delete Amp Initialize
0 Response to "29 Javascript Remove From Object"
Post a Comment