34 Build Tree Array From Flat Array In Javascript
I'm an introvert who loves to program, build stuff, and solve problems. Works @ Neo4j . I can be reached at @oskarhane , ohane and blog@oskarhane . 1,2,3,4,5,6,7. The arr.flat () method was introduced in ES2019. It is used to flatten an array, to reduce the nesting of an array. The flat () method is heavily used in functional programming paradigm of JavaScript. Before flat () method was introduced in JavaScript, various libraries such as underscore.js were primarily used.
Build A Collapsible Tree Menu With Vue Js Recursive
Home » Javascript » Build tree array from flat array in javascript. Build tree array from flat array in javascript . Posted by: admin December 24, 2017 Leave a comment. Questions: I have a complex json file that I have to handle with javascript to make it hierarchical, in order to later build a tree.
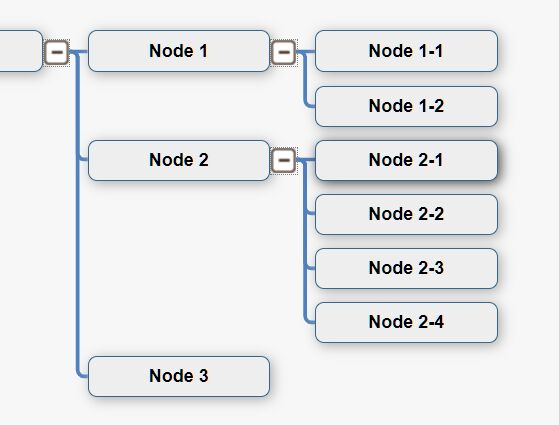
Build tree array from flat array in javascript. 18/9/2015 · var nested = []; for (var i=0; i < flat.length; i++) { // split components up var routes = flat[i].path.split("."); // get parent key var key = routes[0]; // check if we've already added the key var index = -1; for (var j=0; j < nested.length; j++) { if (nested[j].path == key) { index = j; break; } } if (index===-1) { // if we have a new parent add it nested.push(flat[i]) } else { // create subpaths property on new object if … Array.prototype.flat () The flat () method creates a new array with all sub-array elements concatenated into it recursively up to the specified depth. Flat array of objects into deep nested object (tree) with dynamic structure [closed] Asked 2020-12-01 06:40:30. Active 2020-12-01 08:08:56. Viewed 87 times. ... javascript arrays object tree. 1 answers. Oldest Votes #1 votes:3 Accepted. Here's some code that works with recursion. It first finds all the options for the first query, and then ...
Building a hierarchical tree from a flat list: an easy-to-understand solution & visualisation. I came across "how to build a hierarchical tree from a flat list" and viewed some solutions ... Functional JavaScript: Traversing Trees with a Recursive Reduce, JavaScript lets us create tree-like structures easily enough. We can stick objects inside arrays, and arrays inside objects. But there's no real @cubearth: With recursion, each node n is responsible of building its own subtree, yes. Jan 30, 2020 - Javascript function to flatten a nested Associative Array (tree) to a List - flatten.js
How could I build a tree array from this flat array in Javascript by their's parentId and Id. ... How could I build a tree array from this flat array in Javascript by their's parentId and Id. (Example Tree Structure) As mentioned, the data we receive to build this tree example is a flattened array in the following format. Each element represents one node of the tree and can be the child of only one parent node. Node 8 has no parent, so we can see in the array below that the object for id 8 has a parentId equal to null. Build a tree array (3 dimensional) from a flat array in Typescript/Javascript Tags: arrays , javascript , reactjs , tree , typescript I have incoming data in this format:
Build tree array from flat array in JavaScript. We have a complex json file that we have to handle with JavaScript to make it hierarchical, in order to later build a tree. parentId − the id of the parent node (which is 0 if the node is a root of the tree) The JSON data is already "ordered", means that an entry will have above itself a parent ... Jun 03, 2020 - This article explains an effcient array to tree algorithm. We can use a single pass to transform a flat array into a nested tree. Building a hierarchical tree from a flat list — Explained in detail. ... where we build a hierarchical tree; given an array that contains id and parent id, create a hierarchical tree that will parse the data and spit out a formatted object. ... Online JavaScript benchmark tool / playground. Find the best performance and speed up your code!
Aug 01, 2019 - I have a list of "page" objects with a parent field. This parent field references another object in the list. I would like to create a tree hierarchy from this list based on this field. Here is wh... 1/8/2013 · You can use npm package array-to-tree https://github /alferov/array-to-tree. It's convert a plain array of nodes (with pointers to parent nodes) to a nested data structure. Solves a problem with conversion of retrieved from a database sets of data to a nested data structure (i.e. navigation tree… I have a complex json file that I have to handle with TypeScript / Javascript to make it hierarchical, in order to later build a questionnaire. Every entry of the json has a Id (unique), ParentId (0 I
Dec 07, 2019 - Convert a plain array of nodes (with pointers to parent nodes) to a tree A JavaScript repl by kkneville Build tree array from JSON in JavaScript. We are required to write a JavaScript function that takes in one such array. The function should build a tree structure from this array based on the "name" property of objects. Therefore, for the above array, the output should look something like this −.
How would I convert it back to a flat array? javascript arrays algorithm tree hierarchy. Share. ... 3,156 3 3 gold badges 26 26 silver badges 43 43 bronze badges. 1. I found plenty of questions asking how to create a tree from an array, but none converting back in the other direction, so I've posted the solution I came up with. ... Browse other ... Convert a plain array of nodes (with pointers to parent nodes) to a nested data structure - GitHub - alferov/array-to-tree: Convert a plain array of nodes (with pointers to parent nodes) to a neste... Home » JavaScript » Create nested tree Object from a flat string Array. Search for: Search for: JavaScript August 23, 2021. Create nested tree Object from a flat string Array. Given the following Array of strings: ['A','AA','AAA','AAAA']
Construct a complete binary tree from given array in level order fashion. Given an array of elements, our task is to construct a complete binary tree from this array in level order fashion. That is, elements from left in the array will be filled in the tree level wise starting from level 0. Recommended: Please try your approach on {IDE} first ... I have a complex json file that I have to handle with javascript to make it hierarchical, in order to later build a tree. Every entry of the json has : id : a unique id, parentId : the id of the pa Feb 25, 2015 - With the meteoric rise of JavaScript MVC frameworks, data structures and algorithms long handled server-side are now managed on the client. JavaScript references objects thru memory addresses, making linked lists, trees, even graphs all possible... | Dan Martensen | Software Engineering
Here, the answer isn't so obvious. JavaScript lets us create tree-like structures easily enough. We can stick objects inside arrays, and arrays inside objects. But there's no real concept of a Tree type provided by JavaScript. So, there's no Tree.prototype.map() or Tree.prototoype.reduce(). We're on our own. In vanilla JavaScript, you can use the Array.concat () method to flatten a multi-dimensional array. This method works in all modern browsers, and IE6 and above. The apply () method is a part of concat () function's prototype that calls the function with a given this value, and arguments provided as an array. Alternatively, you could use the ES6 ... how - Build tree array from flat array in javascript . lodash array to tree ... in order to later build a tree. Every entry of the json has : id : a unique id, parentId : the id of the parent node (which is 0 if the node is a root of the tree) level : the level of depth in the tree ...
We can use the array flat() method; We can use the array reduce() method; We can use the array map() method; Solution 1: The best solution is we can use the flat() method to flatten nested array in Javascript. And when a flat method is been used it is necessary to pass the Infinity as the argument. May 01, 2020 - I have a complex json file that I have to handle with javascript to make it hierarchical, in order to later build a tree. Every entry of the json has : id : a unique id, parentId : the id of the parent node (which is 0 if the node is a root of the tree) level : the level of depth in the tree Change Fiddle listing shows latest version. The fiddle listings (Public, Private, Titled, etc) will now display latest versions instead of the ones saved as Base versions - this was causing more confusion than good, so we decided to change this long-standing behavior. Previous update Got it. Settings.
Flat array of objects to tree in JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have an array of objects like this − ... Build tree array from flat array in JavaScript; Flat a JavaScript array of objects into an object; I really need your help to create a JSX tree from a flat array in react using recursive function. For the moment only the items 1 to 6 are correctly displayed and the recursion is stopped after. 1) my flat JSON data is : (but it could have more than 1000 items) I have a complex json file that I have to handle with javascript to make it hierarchical, in order to later build a tree. Every entry of the json has : id : a unique id, parentId : the id of the p...
Answer 1. One approach would be to construct the tree with two phases of processing where you first: Sort the input items in data by level, with an ascending sort order. This pre-process simplfies tree contruction in the next step, allowing nodes to be inserted into the tree in order starting from root. "Reduce" the sorted array items, building ... Then we will use this props array to dynamically set the arguments of setNestedValue() method. It's a very simple workflow; We map each object in the item to it's nested form. It's the data.map(d => props line. props array contains 3 arrays one for each id,name and amt. We take them one by one in .reduce((n,p) => p line. To build our tree, we're going to want to: Iterate through the data array. Find the parent element of the current element. In the parent element's object, add a reference to the child. If there is no parent for an element, we know that will be our tree's "root" element. We must realize that references will be maintained down the ...
The algorithm doesn't use any helper methods, just plain JavaScript. The algorithm builds the arrays as it traverses the tree from the top. So here is an example data: var node = { Lets discuss the functionality of getRecursive method step by step,. We call the method getFromServer with the unique identifier of the record and further pipe it with following operators map, flatMap, forkJoin, tap; map converts the data of type FlatTreeData into {parent: {name: string; id: number;children:Array<number>}} we reset the children as empty array since future operators in the pipe ... "Convert nested array of values to a JSON object" "JSON object" is more meaningfully as tree "Convert nested array into a tree" Style. The variables data and parent should be declared as constants const as the references they hold do not change. The JavaScript naming convention is camelCase try to avoid using snake_case.
How would I convert it back to a flat array? javascript arrays algorithm tree hierarchy · How to flatten an array in JavaScript. reduce () Method. flat () & flatMap () Methods. In vanilla JavaScript, you can use the Array.concat () method to flatten a multi-dimensional array. Hey, it's pure gold - exactly what I was Looking for. I am using it to build tree structure based on array of URL's. Of course, firstly I've split every url into an array of paths. Then to achieve fullUrl of current path I had to change one thing, link so:
An Easy Way To Build A Tree In Javascript Using Object
Deep Dive Array Access In Lightning Web Components Codescience
A Hierarchical Approach To Removal Of Unwanted Variation For
Life Cycle Impact Assessment Of Semiconductor Packaging
Find Parent And Child From Array Javascript Code Example
Javascript Trees The Tree Is One Of The Most Common Data
Array Databases Concepts Standards Implementations
How Database B Tree Indexing Works Dzone Database
Create Jsx Tree From Flat Array With Recursive Function In
10 1 Binaryheap An Implicit Binary Tree
How To Flatten An Array In Javascript
Js Tree Structure And Flat Array Conversion Programmer Sought
Convert Nested Array To Single Array Javascript Code Example
Jquery Tree View Plugins Jquery Script
Data Structures In Javascript Arrays Hashmaps And Lists
Javascript Array Flat How To Flat Array In Javascript
Group By In Javascript Flat Array To Nested Json Stack
How To Flatten An Array In Javascript
Convert Sorted Array To Binary Search Tree Leetcode
Create Jsx Tree From Flat Array With Recursive Function In
How To Flatten A Nested Javascript Array By Cynthia
Jquery Tree View Plugins Jquery Script
Github Mrpeak Flatten Tree Transforms A Nested Tree
Functional Javascript Traversing Trees With A Recursive Reduce
Source Maps From Top To Bottom Javascript Indepth
How To Save A Binary Tree To An Array Of In Order Traversal
Electronics Free Full Text A Compressive Sensing Inspired
Understanding Nested Arrays In Javascript Dev Community
11 Ways To Iterate An Array In Javascript Dev Community
Array Databases Concepts Standards Implementations
What Are Data Structures Definition From Whatis Com
0 Response to "34 Build Tree Array From Flat Array In Javascript"
Post a Comment