26 How To Draw Shapes In Javascript
Afterwards we add these shape objects to the stage and call stage.update() which will do the actual drawing in the canvas. Below you can see JavaScript code for creating and drawing circle shape which is then added to the stage. For code used to draw other shapes, please refer to the codepen for this post. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Drawing Shapes With Canvas Web Apis Mdn
1/2/2015 · How to make arbitrary D3.js-based SVG shapes in JavaScript. Examples of lines, circle, rectangle, and path.
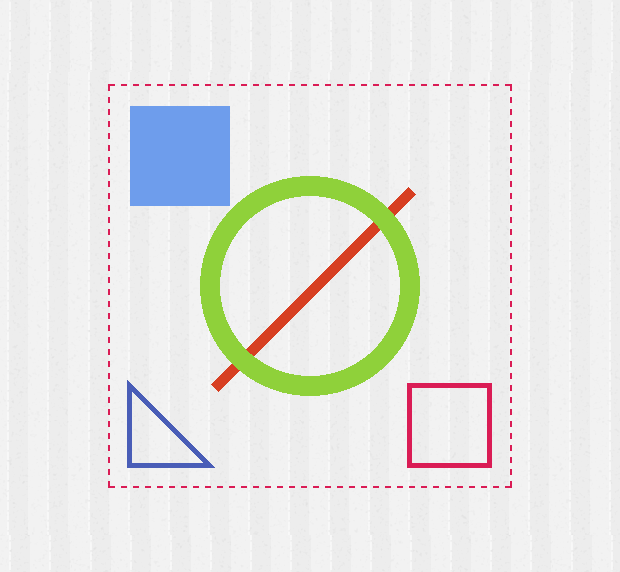
How to draw shapes in javascript. You can add various shapes to your map. A shape is an object on the map, tied to a latitude/longitude coordinate. The following shapes are available: lines, polygons, circles and rectangles. You can also configure your shapes so that users can edit or drag them. Polylines. To draw a line on your map, use a polyline. See the Pen javascript-drawing-exercise-2 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus. Previous: Write a JavaScript program to draw the following rectangular shape. Next: Write a JavaScript program to draw two intersecting rectangles, one of which has alpha transparency. Let's learn how to draw shapes with JavaScript and create fabulous Fibonacci flowers all from scratch with just plain vanilla JavaScript. In this HTML5 Canva...
Drawing Shapes with the JavaScript Canvas API JavaScript. By Joshua Hall. Published on August 5, 2019; While this tutorial has content that we believe is of great benefit to our community, we have not yet tested or edited it to ensure you have an error-free learning experience. It's on our list, and we're working on it! DOCTYPE HTML > < html > < head > < script > window.onload = function(){!--w w w. j a v a 2 s. c o m--> var canvas = document.getElementById("myCanvas"); var context = canvas.getContext("2d"); // draw rectangle context.beginPath(); context.rect(240, 30, 130, 130); context.fillStyle = "blue"; context.fill(); // draw circle context.globalAlpha = 0.5; // set global alpha context.beginPath(); context.arc(359, 150, 70, 0, 2 * Math.PI, … Function to create diamond shape given a value in JavaScript? Javascript Web Development Object Oriented Programming. You can create your own function to create diamond shapes for a given value. Following is the code −
That said, when I made the shift from Flash and ActionScript to HTML5 and JavaScript, I found the Canvas API to be very low-level in that it took many lines of code to simply draw a basic shape. To make the process more efficient, I have been creating custom libraries that take care of the rendering details and free me up to focus on the ... Collision detection and physics. Perform collision detection and react with physics, using JavaScript. Check for overlap between shapes, apply hitboxes and calculate new velocities. Make it more natural with object mass, gravity and restitution. By the end of this tutorial, you'll have a basic physics simulation running in your game. In JavaScript we can access it by id and draw it as shown below. ... For a complete tutorial about drawing basic shapes you can refer to the Mozilla Developer Network documentation.
4/9/2015 · You can use the Math.Floor function to generate a random number, then with that random number you can use it to draw a specific shape from your array. As you haven't provided your array I have created a very simple jsFiddle showing you an example https://jsfiddle /kny74wve/ Just keep pressing the run button in the top left to generate a new random number Learn how to draw rectangles and lines with code (JavaScript and ProcessingJS). If you're seeing this message, it means we're having trouble loading external resources on our website. If you're behind a web filter, please make sure that the domains *.kastatic and *.kasandbox are unblocked. On JavaScript code, implement the getContext ('2d') method to draw shapes in a context. To draw a circle, add the beginPath () method along with the arc () method. And, to fill the circle add the fillStyle () method along with the fill () method. In the arc () method, define the center point position and the radius.
To draw lines and shapes using HTML5 canvas, the path API must be used. The path API stores a collection of subpaths formed by various shape functions. Here are the various shape functions used in JavaScript code which can be used to draw lines, triangle, rectangles and circles. context.beginPath () - This function is used to begin a path. Canvas How to - Draw Spade, Heart, Club, Diamond. Back to Shape ↑ Question. We would like to know how to draw Spade, Heart, Club, Diamond. Answer I want to draw an ellipse shape in canvas.js or any other javascript. Is it possible to draw in canvas.js or not? Please help me draw the ellipse shape and program the code. Thank you.
Draws a solid shape by filling the path's content area. The first step to create a path is to call the beginPath(). Internally, paths are stored as a list of sub-paths (lines, arcs, etc) which together form a shape. Every time this method is called, the list is reset and we can start drawing new shapes. fillText () — draws filled text. strokeText () — draws outline (stroke) text. Both of these take three properties in their basic usage: the text string to draw and the X and Y coordinates of the point to start drawing the text at. In this video, I start writing code and cover the basics of coordinates systems, shapes, and drawing in p5.js.💻 Code: https://thecodingtrain /beginners/p...
Javascript Draw two semicircle arcs on a canvas. Javascript Extend a line before and after original endpoints. Javascript Fill a path. Javascript Fill rectangle. Javascript Fill Triangle. Javascript Get random points on circle. Javascript Link lines to create shape. Javascript Link mouse clicked points with lines. Drawing complex shapes. Basic shapes are great. You can definitely draw anything by carefully constructing polylines and polygons with lots of points. But, SVG has a better solution for drawing complex shapes. The <path> element is the most powerful shape construct. It is a little programming language of its own. 26/2/2020 · Write a JavaScript program to draw the following rectangular shape. Sample output: Sample Solution: HTML Code: <!DOCTYPE html> <html> <head> <meta charset=utf-8 /> <title> Draw a rectangular shape </title> </head> <body onload="draw ();"> <canvas id="canvas" width="150" height="150"></canvas> </body> </html>. Copy.
Courtesy of Walter Zorn, you can now use JavaScript to draw objects on your web pages. Fire up your JavaScript Editor, follow the instructions below and you will be drawing shapes on your web pages in no time. This library is a great choice for creating games, or just to spruce up your web pages. Inside of our init function, we use context2D.beginPath to tell the canvas we want to start a new path/shape. On the next line, we create and draw a rectangle (a square in this case). There are two different methods we use to draw the path to the screen: ctx.strokeRect(x, y, width, height) — this creates a "stroked" rectangle. Stroke is ... Now, getting back to drawing the cube. First, create an html file with any name you like, say "cube.html" and put the html template I've provided above in it. Since most of the code we'll use to draw the cube and other shapes is based on javascript, our code will go into the script tags. I shall avoid multiple files for the sake of ...
A few primitive shapes can be drawn directly onto the graphics context in JavaScript. The most common shapes for HTML5 and CSS3 programming that most web developers use are rectangles and text. Rectangle functions You can draw three different types of rectangles: clearRect(x, y, w, h): Erases a rectangle with the upper-left corner (x,y) and […] Displaying images and other flat shapes in web pages is pretty easy. However, when it comes to displaying 3D shapes things become less easy, as 3D geometry is more complex than 2D geometry. How to draw shapes in javascript. Currently Enrolling S Example: Python3 Don't press . Markers are placed at each waypoint along the route widgets import DrawingWidget import hyperbolic We can manually draw shapes by consecutive commands giving coordinates. Rectangle functions You can draw three different types of rectangles: clearRect(x, y, w ...
Summary: in this tutorial, you'll learn how to use the JavaScript rotate() method to rotate drawing objects. Introduction to JavaScript rotate() canvas API. The rotate() is a method of the 2D drawing context. The rotate() method allows you to rotate a drawing object on the canvas. Here is the syntax of the rotate() method: Drawing Shapes. In this lesson, we will learn how to draw simple shapes using HTML5 canvas. We are going to draw circles, rectangles and polygons in different colours. Setting up. First of all, create a new folder where you will store all your code. If you have trouble coming up with a name, I named mine LunarLander.
How To Draw Geometrical Shapes In Html Canvas Via Javascript
Drawing Sketchy Shapes In Javascript Using Roughjs Dev
Mindfusion Javascript Diagram Library V2 6 Mindfusion
A Basic Introduction To Fabric Js To Draw 2d Graphics On Web
Create Line Segment In Three Js With 2d Shapes Stack Overflow
Drawing Shapes With Canvas Web Apis Mdn
Beginner P5 Js Lesson 2 Drawing Shapes Ellipse Triangle Rectangle Lines And Changing Colors
Graphpad Prism 9 User Guide Drawing Lines Arrows And Shapes
How To Draw Shapes With Javascript Html5 Canvas Tutorial For
How To Compose Canvas Animations In Typescript
How To Use Quick Shape In Procreate 4 2 Cate Shaner Blog
How To Draw And Use Freeform Shapes In Microsoft Word
Drawing With P5 Js Coding Projects With P5 Js
Creating 2d Texture On A 3d Shape In P5 Js By Nazia
Drawing Shapes With The Pen Tool Chapter 7 Wielding The
Working With Shapes In Web Design Css Tricks
Creating And Drawing On An Html5 Canvas Using Javascript By
Draw Shapes And Fill With Color In Javascript Typescript
How To Draw Animals That Are Cute Using Mostly Rectangles
Getting Started With Pixi Js Ihatetomatoes
Computer Programming Intro To Js Drawing Amp Animation Drawing
How To Draw Rectangle Circle And Basic Shape On Pdf Page
10 Javascript Libraries To Draw Your Own Diagrams 2020 Edition
Draw And Edit Freeform Shapes In Activepresenter 8
Working With Canvas Javafx 2 Tutorials And Documentation
0 Response to "26 How To Draw Shapes In Javascript"
Post a Comment