29 Javascript Iterate Json Object
Right now what I do with a normalized JSON object is to simply pass it in lodash's _.values (obj) when I need to iterate with array methods. Short snippet of one of my mapStateToProps method: const mapStateToProps = (state) => { return { data: _.values(state.data) } } How to dynamically create and iterate Json Object inside a Json Array using Postman - Javascript. General Discussion. request. security-explorer-25 15 August 2021 17:28 #1. ... **I want to increment the json objects dynamically(it can be a duplicate as well) ...
Iterate Json Array Java Javatpoint
Aug 17, 2020 - Complete a function that takes in one parameter, an object. Your function should iterate over the object, and log the values to the console. Your function need not return anything. NOTE: DO NOT USE Object.keys, or Object.values in your solution.
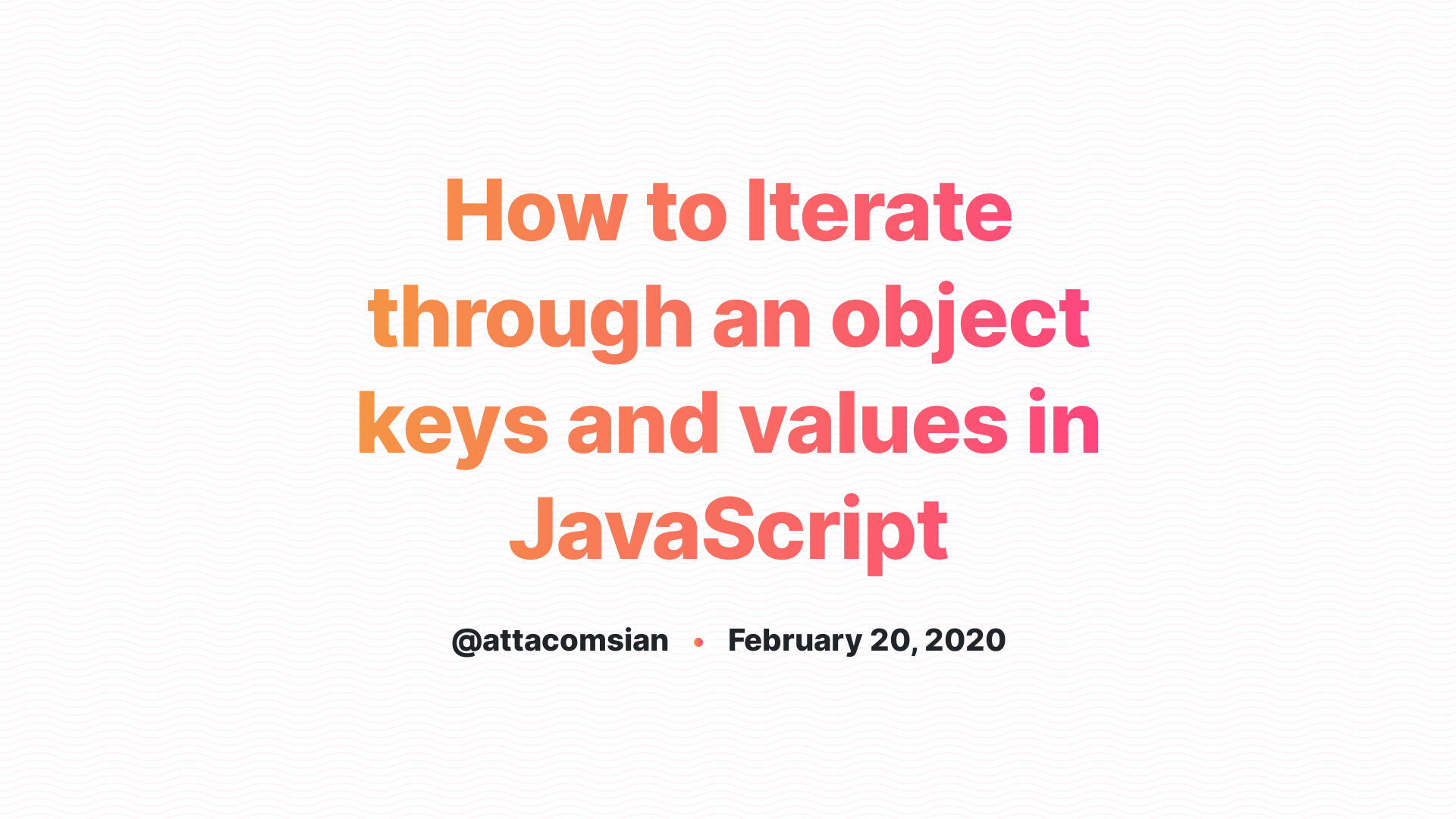
Javascript iterate json object. Convert JSON String to PHP Array or Object. PHP >= 5.2.0 features a function, json_decode, that decodes a JSON string into a PHP variable. By default it returns an object. The second parameter accepts a boolean that when set as true, tells it to return the objects as associative arrays. You can learn more about the json_decode function from PHP ... 1 day ago - JavaScript has multiple iteration methods, starting with a traditional for loop and not the forEach method. Helper libraries like Lodash (forEach) and jQuery (each) can make it easier. Oct 30, 2018 - This tutorials help to process json objects using javascript. I have also provided example to type of json objects and how to iterate on json.
Get code examples like"javascript iterate over json". Write more code and save time using our ready-made code examples. Aug 10, 2020 - Complete a function that takes in one parameter, an object. Your function should iterate over the object, and log the values to the console. Your function need not return anything. NOTE: DO NOT USE Object.keys, or Object.values in your solution. May 16, 2020 - This is the most common problem when we are working with the server-side development and we have to traverse JSON string dynamically as we often don’t know how deep our JSON string would be! There…
JSON stands for J ava S cript O bject N otation. JSON is a lightweight data interchange format. JSON is language independent *. JSON is "self-describing" and easy to understand. * The JSON syntax is derived from JavaScript object notation syntax, but the JSON format is text only. Code for reading and generating JSON data can be written in any ... Apr 09, 2020 - Complete a function that takes in one parameter, an object. Your function should iterate over the object, and log the values to the console. Your function need not return anything. NOTE: DO NOT USE Object.keys, or Object.values in your solution. Here's a very common task: iterating over an object properties, in JavaScript Published Nov 02, 2019 , Last Updated Apr 05, 2020 If you have an object, you can't just iterate it using map() , forEach() or a for..of loop.
Well according to the JSON standards . 4. Objects. An object structure is represented as a pair of curly brackets surrounding zero or more name/value pairs (or members). A name is a string. A single colon comes after each name, separating the name from the value. A single comma separates a value from a following name. JSON.parse () The JSON.parse () method parses a JSON string, constructing the JavaScript value or object described by the string. An optional reviver function can be provided to perform a transformation on the resulting object before it is returned. FYI: You do not have a "JSON object." There's no such thing as a "JSON object." It's a widespread misnomer. JSON is a text format. What you have is an array of objects. Not JSON, just objects. - Mike Cluck Aug 26 '16 at 16:13
JSON stands for JavaScript Object Notation. It's a light format for storing and transferring data from one place to another. So in looping, it is one of the most commonly used techniques for transporting data that is the array format or in attribute values. Some of my Favorite JavaScript Tips and Tricks Tutorials ; How to use Gson -> fromJson() to convert the specified JSON into an Object of the Specified Class ; How to iterate through Java List? Seven (7) ways to Iterate Through Loop in Java 20/2/2020 · To iterate over the array returned by Object.entries(), you can either use the for...of loop or the forEach() method as shown below: // `for...of` loop for ( const [ key , value ] of Object . entries ( animals ) ) { console . log ( ` ${ key } : ${ value } ` ) ; } // `forEach()` method Object . entries ( animals ) . forEach ( ( [ key , value ] ) => { console . log ( ` ${ key } : ${ value } ` ) } ) ;
// Example object let obj = { key1: "val1", key2: "val2", key3: "val3" } We'll go over a few ways JavaScript allows us to "iterate" through JSON objects. Method 1: .entries() We can use Object.entries() to convert a JSON array to an iterable array of keys and values. Object.entries(obj) will return an iterable multidimensional array. A common use of JSON is to exchange data to/from a web server. When receiving data from a web server, the data is always a string. Parse the data with JSON.parse(), and the data becomes a JavaScript object. Introduction. An object in JavaScript is a data type that is composed of a collection of names or keys and values, represented in name:value pairs.The name:value pairs can consist of properties that may contain any data type — including strings, numbers, and Booleans — as well as methods, which are functions contained within an object.. Objects in JavaScript are standalone entities that ...
Comparison to JavaScript Object. It is worth keeping in mind that JSON was developed to be used by any programming language, while JavaScript objects can only be worked with directly through the JavaScript programming language. In terms of syntax, JavaScript objects are similar to JSON, but the keys in JavaScript objects are not strings in quotes. When targeting an ECMAScipt ... the built-in iterator implementation in the engine. The TypeScript docs are an open source project. Help us improve these pages by sending a Pull Request ❤ ... See how TypeScript improves day to day working with JavaScript with minimal ... 1 week ago - The Object.entries() method returns an array of a given object's own enumerable string-keyed property [key, value] pairs. This is the same as iterating with a for...in loop, except that a for...in loop enumerates properties in the prototype chain as well).
iterate over json object js; javascript loop through json object get value; javascript loop through each element of a json object; loop through json file javascript; js loop through json; loop json in javascript; loop through json object javascript. for loop json object; how to loop through json object in javascript; loop json data javascript ... Complete a function that takes in one parameter, an object. Your function should iterate over the object, and log the values to the console. Your function need not return anything. NOTE: DO NOT USE Object.keys, or Object.values in your solution. May 07, 2021 - Once we have our response as a JavaScript object, there are a number of methods we can use to loop through it. Use a for…in Loop A for…in loop iterates over all enumerable properties of an object:
4 weeks ago - A protip by steveniseki about jquery and javascript. JSON forEach tutorial shows how to loop over a JSON array in JavaScript. In this tutorial we use JSON server to handle test data. The json-server is a JavaScript library to create testing REST API. First, we create a project directory an install the json-server module. $ mkdir jsonforeach $ cd jsonforeach $ npm init -y $ npm i -g json-server. JSON was inspired by the JavaScript Object Literal notation, but there are differences between the two. ... We'll need to parse this into a JavaScript object before we can loop through its ...
Objects created from built-in constructors like Array and Object have inherited non-enumerable properties from Object.prototype and String.prototype, such as String's indexOf() method or Object's toString() method. The loop will iterate over all enumerable properties of the object itself and those the object inherits from its prototype ... JavaScript iterate key & value from json? [duplicate] Ask Question Asked 5 years, 2 months ago. Active 6 months ago. ... How do I loop through or enumerate a JavaScript object? 3468. Checking if a key exists in a JavaScript object? 9750. How can I remove a specific item from an array? Apr 10, 2021 - You might work with JSON, In case if you don’t know about JSON then here is a nice explanation on JSON. JSON stands for JavaScript Object Notation. There are two ways data can be stored in JSON: You…
JSON is a file format widely used for static storage and app config management with any of the frameworks and data servers. Any JSON file contains the key-value pair separated by the comma operator. JavaScript objects are an integral part of the React app, so they need to get accessed from JSON files/data to be uses in components. All I am trying to do is loop through a JSON Object and publish relevant Object items onto my MVC web page. Here is my code below (I've kept this very basic just so I can get the basics correct first time round) However, I keep getting the following error: Newtonsoft.Json.Linq.JProperty' does not contain a definition for 'alertName''. JSON Object Literals. JSON object literals are surrounded by curly braces {}. JSON object literals contains key/value pairs. Keys and values are separated by a colon. Each key/value pair is separated by a comma. It is a common mistake to call a JSON object literal "a JSON object". JSON cannot be an object.
2/7/2009 · Your jsonObject is not a real JSON object. It is a javascript object. That is why this works. However if anybody have a JSON object he can convert it to a JS object and then use your method. To convert a JSON object to JS object use jsObject = JSON.parse(jsonObject); – prageeth Oct 31 … Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. you need to iterate over the array inside the data not the data object. data.geonames.map ( () => .... ) The data you are getting after .json () is still an object. It is the geonames key, which is actually an array.
Array.filter () The filter () method creates a new array with array elements that passes a test. This example creates a new array from elements with a value larger than 18: Example. const numbers = [45, 4, 9, 16, 25]; const over18 = numbers.filter(myFunction); function myFunction (value, index, array) {. 23/3/2020 · We first want to parse the JSON to make it iterable as a normal Object: const parsedJSON = JSON.parse(json); If we want the values of each property we can do the following: for( let prop in parsedJSON ){ console.log( json[parsedJSON] ); } We cycle through each property and output the value by referencing the current prop in our object. Possible to iterate through a JSON object and return values of each property? JavaScript. njanne19. October 28, 2020, 4:30pm #1. Hey all, I'm trying to add a shopping list to my Recipe box project as an extra personalization, and when researching on how to do this, one camper recommended that in order for me to render my Shopping list in a ...
Oct 13, 2019 - The question won't be complete if we don't mention about alternative methods for looping through objects. Nowadays many well known JavaScript libraries provide their own methods for iterating over collections, i.e. over arrays, objects, and array-like objects.
Parse Json String Into Javascript Object Prototype Or
How To Iterate Through An Object Keys And Values In Javascript
Parse Json And Store Json Data Using Node Js Codez Up
Json Handling With Php How To Encode Write Parse Decode
Search Through A Json Object Using Javascript Dev Community
How To Flatten Deeply Nested Json Objects In Non Recursive
Postman Extract Value From The Json Object Array By Knoldus
Nested Arrays In Json Object Json Tutorial
Json Object Parsing With Nested Array Help Uipath
Understanding Json In Javascript Json Javascript Object
Javascript Iterate Through Object Properties Code Example
How To Work With Json In Javascript
How Do I Loop Through Or Enumerate A Javascript Object
Parse Multiple Objects From Json String Service Integration
Json Object Parsing With Nested Array Help Uipath
How To Iterate Through A Json File In Javascript Code Example
How To Dynamically Create And Iterate Json Object Inside A
How To Convert Js Object To Json String In Jquery Javascript
Speedy Tip How To Loop Through A Json Response In Javascript
How To Retrieve Value From Complex Json Object And Array In Power Automate Using Expression
Javascript How To Add An Element To A Json Object
How To Iterate Over A Javascript Object Geeksforgeeks
Javascript How To Receive Data From Json Array Stack Overflow
How To Loop Through An Array Of Objects In Javascript Pakainfo
Speedy Tip How To Loop Through A Json Response In Javascript
Python Iterate Over Json Keys Code Example
Iterate Json Array In Node Js Code Example
How To Fetch Data From Json File And Display In Html Table
0 Response to "29 Javascript Iterate Json Object"
Post a Comment