33 Javascript Array Find Example
The array on which find() operated. thisArg - It is optional. The value to use as this while executing callback. Return. The value of first element of the array that satisfies the function condition. JavaScript Array find() method example. Let's see some examples of find() method. Example 1. Let's see a simple example of find() method. Internally, the Array.prototype.includes() uses the same value zero algorithm to search for the presence of the element value in the array. Examples of JavaScript Array Contain. Let us consider a few examples of how the includes() method can be used to determine the presence of particular value in the array that is specified.
Javascript Get Array First Element Example Mywebtuts Com
Return value:It returns the array element index if any of the elements in the array pass the test, otherwise it returns -1. Below examples illustrate the Array findIndex() function in JavaScript: Example 1: In this example the method findIndex() finds all the indices that contain odd numbers.
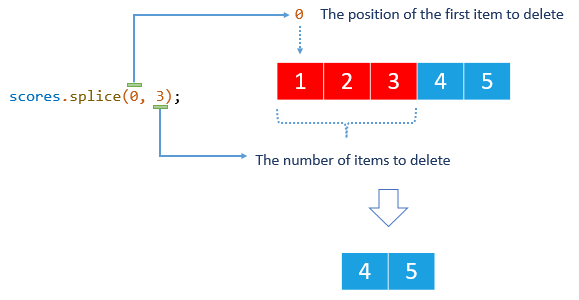
Javascript array find example. The .find () method is an easier way to find and return the first element of a provided array, under a defined testing function. However, .find () only return a single element and if nothing is found it returns a value of undefined. So if the goal is to the return a single value, use .find (). If the callback returns a truthy value, the find() immediately returns the element and stop searching. Otherwise, it returns undefined. If you want to find the index of the found element, you can use the findIndex() method. JavaScript find() examples. The following example uses the find() method to search for the first even number in an array ... Summary: in this tutorial, you will learn how to use the Array findIndex() method to find the first element that satisfies a given test.. ES6 added a new method called findIndex() to the Array.prototype, which allows you to find the first element in an array that satisfies a provided testing function.. The findIndex() method returns the index of the element that satisfies a testing function or ...
Array.find() and Array.findIndex() are two new methods in JavaScript ES6. They make searching arrays more easier than before without writing cumbersome code and loops. Let's see them in more details with simple examples. The Array.find() Method by Example. The Array.find() method is used to search for the first element in the array that ... The find() method searches through an array and gives you 1 item based on your provided test. The find() method returns a single item. Jul 26, 2021 - Below examples illustrate the JavaScript Array find() method in JavaScript: Example 1: Here the arr.find() method in JavaScript returns the value of the first element in the array that satisfies the provided testing method.
Definition and Usage. The find() method returns the value of the array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values) The reduce() method executes a user-supplied “reducer” callback function on each element of the array, passing in the return value from the calculation on the preceding element. The final result of running the reducer across all elements of the array is a single value. JavaScript find min value in Array of objects | Example code Posted December 18, 2020 July 6, 2021 by Rohit There are many ways to get the min value in an array of objects in JavaScript.
1 week ago - An array is an object that can store a collection of items. Arrays become really useful when you need to store large amounts of data of the same type. Suppose you want to store details of 500 employe In JavaScript, find () is an Array method that is used to return the value of the first element in the array that meets a specific criteria. Because the find () method is a method of the Array object, it must be invoked through a particular instance of the Array class. The following example finds an element in the array that is a prime number (or returns undefined if there is no prime number): function isPrime ( element , index , array ) { let start = 2 ; while ( start <= Math . sqrt ( element ) ) { if ( element % start ++ < 1 ) { return false ; } } return element > 1 ; } console . log ( [ 4 , 6 , 8 , 12 ] . find ( isPrime ) ) ; // undefined, not found console . log ( [ 4 , 5 , 8 , 12 ] . find ( isPrime ) ) ; // 5
Example 1: Using find () method. function isEven(element) { return element % 2 == 0; } let randomArray = [1, 45, 8, 98, 7]; let firstEven = randomArray.find (isEven); console.log (firstEven); // 8 // using arrow operator. let firstOdd = randomArray.find ( (element) => element % 2 == 1 ); console.log (firstOdd); // 1. I know similar questions have been asked before, but this one is a little different. I have an array of unnamed objects, which contain an array of named objects, and I need to get the object where "name" is "string 1". Examples of JavaScript array find method let's see example of Array find () function in JS. The find () method executes the function once for each element present in the array: If it finds an array element then it will return the value of that element and doesn't t check the remaining values. Otherwise, it returns the undefined output.
The find () method searches through an array and gives you 1 item based on your provided test. Array find () only returns one item. If you want to get more than one item, look at filter (). Here we'll find one person based on their name: How to add JavaScript to html How ... How to find factorial of a number in JavaScript How to get the value of PI using JavaScript How to make a text italic using JavaScript What are the uses of JavaScript How to get all checked checkbox value in JavaScript How to open JSON file Random image generator in JavaScript How to add object in array using JavaScript ... The JavaScript Array.find method is a convenient way to find and return the first occurence of an element in an array, under a defined testing function. When you want a single needle from the haystack, reach for find()!. When to Use Array.find. The function and syntax of find() is very much like the Array.filter method, except it only returns a single element.
Find duplicates in an array using javaScript In this article we shall look at the different methods of finding duplicates in an array. Some of these methods only count the number of duplicate elements while the others also tell us which element is repeating and some do both. There is nothing special about JavaScript arrays and the properties that cause this. JavaScript properties that begin with a digit cannot be referenced with dot notation and must be accessed using bracket notation. For example, if you had an object with a property named 3d, it can only be ... JavaScript provides many functions that can solve your problem without actually implementing the logic in a general cycle. Let's take a look. Find an object in an array by its values - Array.find. Let's say we want to find a car that is red. We can use the function Array.find. let car = cars.find(car => car.color === "red");
Definition and Usage. The findIndex() method returns the index of the first array element that passes a test (provided by a function).. The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, findIndex() returns the index of that array element (and does not check the remaining values) find () method executes a callback function once for each element in the array until it finds a value that returns true. If nothing passes, undefined is returned. find () does not mutate or change the original Array. Example #1 JavaScript find() method. find() method is used to get the first element from an array which passes the given test (condition). Syntax: array.find(function, [value]); Parameters: A function name and an optional value to be tested with all elements. Ref: JS Array find() function. Return value: A first element which passes the test. Example:
JavaScript Array. JavaScript array is an object that represents a collection of similar type of elements. There are 3 ways to construct array in JavaScript. By array literal; By creating instance of Array directly (using new keyword) By using an Array constructor (using new keyword) 1) JavaScript array literal Working of JavaScript Arrays. In JavaScript, an array is an object. And, the indices of arrays are objects keys. Since arrays are objects, the array elements are stored by reference. Hence, when an array value is copied, any change in the copied array will also reflect in the original array. For example, The JavaScript Array find() method returns the value of the first element in the array that satisfies the provided testing function. Otherwise undefined is returned.
Array.from() Creates a new Array instance from an array-like or iterable object.. Array.isArray() Returns true if the argument is an array, or false otherwise.. Array.of() Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments. in the example above, Array.find () will return only the first even number in the array. working with nested arrays: In order to work with nested arrays we have to use another array method called flat () Array.flat () takes a number which represent the level of the flatten from SO: Array.prototype.find() is undefined Yes, in IE11 it returns the expected result of 5 instead of TypeError: undefined is not a function, as SO: Array.prototype.find() is undefined in 2014 stated.. So... Am I missing something and IE11 really doesn't work properly with Array.prototype.find , or the last updates of IE11 that were made a while ago (but later than the SO question above was ...
There are multiple methods to find the smallest and largest numbers in a JavaScript array, and the performance of these methods varies based on the number of elements in the array. Let's discuss each of them separately and give the testing results in the end.
Checking If An Array Contains A Value In Javascript
Javascript Object Array Find Item
Javascript Find An Object In Array Based On Object S
Find The Length Of A Javascript Object Geeksforgeeks
Javascript Array Find How To Find Element In Javascript
Four Different Ways To Search An Array In Javascript
Javascript Array Map Method Geeksforgeeks
Javascript Array Distinct Ever Wanted To Get Distinct
Javascript Array Indexof And Lastindexof Locating An Element
Javascript Find Min Value In Array Of Objects Example Code
Tools Qa Array In Javascript And Common Operations On
Javascript Algorithms What Is Binary Search A Detailed Step
Arrays In Python What Are Python Arrays Amp How To Use Them
Javascript Array Splice Delete Insert And Replace
How To Append Element To Array In Javascript Tecadmin
Javascript Array Find Function With Example Json World
Filter Array In Javascript Json World
Javascript Find Minimum In Rotated Sorted Array Stack
Find The Smallest And Second Smallest Elements In An Array
Indexed Collections Javascript Mdn
All About Javascript Arrays In 1 Article By Rajesh Pillai
Javascript Array Contains A Step By Step Guide Career Karma
Javascript Array Helper Find Steven Stromick
Learn Javascript Es6 Array Find Amp Array Findindex By
Find Equal Elements In Array Javascript Code Example
Javascript Array Find Find First Occurrence Of Array Tuts
How To Search In An Array Of Objects With Javascript
How To Find Array Length In Java Javatpoint
5 Way To Append Item To Array In Javascript Samanthaming Com
Hacks For Creating Javascript Arrays
0 Response to "33 Javascript Array Find Example"
Post a Comment