25 Javascript Array Insert Element
Nov 02, 2019 - Also there isn't any insert method in JavaScript, but we have a method which is a built-in Array method which does the job for you. It's called splice... Let's see what's splice()... The splice() method changes the contents of an array by removing existing elements and/or adding new elements. Adding an element at a given position of the array. Sometimes you need to add an element to a given position in an array. JavaScript doesn't support it out of the box. So we need to create a function to be able to do that. We can add it to the Array prototype so that we can use it directly on the object.
How To Add Object In Array Using Javascript Javatpoint
Insert One or Multiple Elements at a Specific Index Using Array.splice () The JavaScript Array.splice () method is used to change the contents of an array by removing existing elements and optionally adding new elements. This method accepts three parameters.
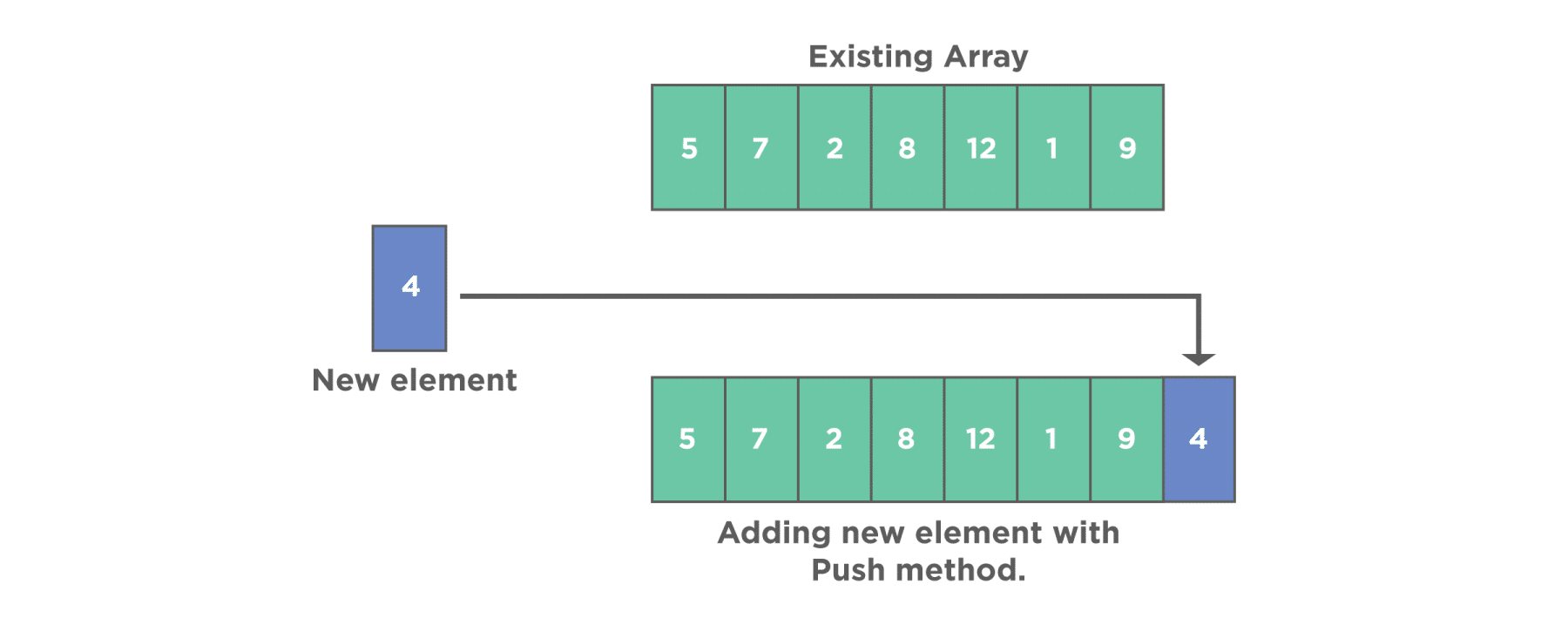
Javascript array insert element. If you aren't adverse to extending natives in JavaScript, you could add this method to the Array prototype: Array. prototype. insert = function ( index, item) { this.splice( index, 0, item); }; I've tinkered around quite a bit with arrays, as you may have noticed: Remove an Item From an Array. Clone Arrays. Inserting an item into an existing array. Inserting an item into an existing array is a daily common task. You can add elements to the end of an array using push, to the beginning using unshift, or to the middle using splice. Those are known methods, but it doesn't mean there isn't a more performant way. Here we go: Adding an element at the end May 06, 2021 - When you already have an existing array and need to make some edits, the Array.splice() method comes in handy. The splice() method allows you to INSERT, REMOVE, and REPLACE elements from a javascript array.
Jul 21, 2019 - A tutorial on how to use `splice()` to add and remove elements from JavaScript arrays You can use the splice() method to insert a value or an item into an array at a specific index in JavaScript. This is a very powerful and versatile method for performing array manipulations. DOM Element. accessKey ... Previous JavaScript Array Reference Next ... Definition and Usage. The push() method adds new items to the end of an array. push() changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift(). Browser Support.
Arrays in JavaScript can work both as a queue and as a stack. They allow you to add/remove elements both to/from the beginning or the end. In computer science the data structure that allows this, is called deque. Methods that work with the end of the array: pop. Extracts the last element of the array and returns it: 5 ways to add an item to the end of an array. Push, Splice, and Length will mutate the original array. Concat and Spread won't and will return a new array... JavaScript | Add new elements at the beginning of an array. Adding new elements at the beginning of existing array can be done by using Array unshift () method. This method is similar to push () method but it adds element at the beginning of the array.
May 04, 2020 - Get code examples like "insert element in array position 0" instantly right from your google search results with the Grepper Chrome Extension. See the Pen JavaScript: Add items in a blank array and display the items - array-ex-13 by w3resource (@w3resource) on CodePen. Improve this sample solution and post your code through Disqus Previous: Write a JavaScript program to compute the sum and product of an array of integers. push (value) → Add an element to the end of the array. unshift (value) → Add an element to the beginning of an array. To add an element to the specific index there is no method available in Array object. But we can use already available splice method in Array object to achieve this.
Array.prototype.splice () The splice () method changes the contents of an array by removing or replacing existing elements and/or adding new elements in place. To access part of an array without modifying it, see slice (). To add an element to the end of an array, we can use the fact that the length of an array is always one less than the index. Say, the length of an array is 5, then the last index at which the value will be 4. So, we can directly add the element at the last+1 index. Let us take a look: Jul 20, 2021 - The unshift() method is used to add one or multiple elements to the beginning of an array. It returns the length of the new array formed. An object can be inserted by passing the object as a parameter to this method. The object is hence added to the beginning of the array.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Using Array.unshift () The easiest way to add elements at the beginning of an array is to use unshift () method. Jul 22, 2019 - insert an element at specific position in array javascript ... JS insert into array at position...
JavaScript Array type provides a very powerful splice() method that allows you to insert new elements into the middle of an array. However, you can use this method to delete and replace existing elements as well. push () ¶. The push () method is an in-built JavaScript method that is used to add a number, string, object, array, or any value to the Array. You can use the push () function that adds new items to the end of an array and returns the new length. The new item (s) will be added only at the end of the Array. You can also add multiple elements to ... JavaScript arrays have 3 methods for adding an element to an array: push () adds to the end of the array. unshift () adds to the beginning of the array. splice () adds to the middle of the array. Below are examples of using push (), unshift (), and splice ().
Here are the different JavaScript functions you can use to add elements to an array: # 1 push - Add an element to the end of the array #2 unshift - Insert an element at the beginning of the array #3 spread operator - Adding elements to an array using the new ES6 spread operator In the above program, the splice () method is used to add a new element to an array. In the splice () method, The first argument is the index of an array where you want to add an element. The second argument is the number of elements that you want to remove from the index element. javascript has two inbuilt array methods name unshift() and push(), which is used to add elements or items at first or beginning and last or ending of an array in javascript. This tutorial has the purpose to explain how to add an element at the beginning and ending of an array in javascript using these unshift and push js methods.
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: You want to explicitly add it at a particular place of the array. That place is called the index. Array indexes start from 0, so if you want to add the item first, you'll use index 0, in the second place the index is 1, and so on. To perform this operation you will use the splice () method of an array. Nov 11, 2019 - Learn how to insert an element in specific index in array in JavaScript.
In this snippet, we are going to discuss a question concerning JavaScript array. Today's topic covers the insertion of an item into the existing array at a specific index. However, there is no inbuilt method that directly allows inserting an element at any arbitrary index of the array. One of the methods is the splice function. The first and probably the most common JavaScript array method you will encounter is push (). The push () method is used for adding an element to the end of an array. Let's say you have an array of elements, each element being a string representing a task you need to accomplish. There are a couple of different ways to add elements to an array in Javascript: ARRAY.push ("ELEMENT") will append to the end of the array. ARRAY.unshift ("ELEMENT") will append to the start of the array. ARRAY [ARRAY.length] = "ELEMENT" acts just like push, and will append to the end. ARRAYA.concat (ARRAYB) will join two arrays together.
In the above program, the splice () method is used to insert an item with a specific index into an array. The splice () method adds and/or removes an item. In the splice () method, The first argument specifies the index where you want to insert an item. The second argument (here 0) specifies the number of items to remove. You can use this to functionally push a single element onto the front or back of an existing array; to do so, you need to turn the new element into a single element array: const array = [3, 2, 1] const newFirstElement = 4 const newArray = [newFirstElement].concat(array) // [ 4, 3, 2, 1 ] Removes the first element from an array and returns that element. Array.prototype.slice() Extracts a section of the calling array and returns a new array. Array.prototype.some() Returns true if at least one element in this array satisfies the provided testing function. Array.prototype.sort() Sorts the elements of an array in place and returns ...
How to insert an element to the DOM in JavaScript March 11, 2020 • Atta In my previous articles, we looked at how to add markup as well as plain-text to HTML elements by using vanilla JavaScript. The indexes of elements after the inserted ones are updated to reflect their new positions. The splice method returns an empty array when no elements are removed; otherwise it returns an array containing the removed elements. ... And More ... © 2001-2021 Dynamic Web Coding Tutorials | JavaScript | ...
Javascript How To Insert Elements Into A Specific Index Of
Python List Append How To Add An Element To An Array
Javascript Array A Complete Guide For Beginners Dataflair
Insert An Element At A Particular Index In An Array Time
Javascript Array Push How To Add Element In Array
C Program To Insert An Element In An Array
Javascript Array Splice Delete Insert And Replace
Java67 How To Add Elements Of Two Arrays In Java Example
Java67 How To Remove A Number From An Integer Array In Java
How To Find Even Numbers In An Array Using Javascript
Python List Append How To Add An Element To An Array
Insert An Element At A Particular Index In An Array Time
How To Remove And Add Elements To A Javascript Array
Javascript Array Remove Element From Array Tuts Make
5 Way To Append Item To Array In Javascript Samanthaming Com
Javascript Array Methods Cheat Sheet Part 1 Therichpost
C Java Php Programming Source Code Javascript Add
Tools Qa Array In Javascript And Common Operations On
Javascript Tutorial 7 Changing And Adding Array Elements
Add Or Remove Array Value With Javascript And Jquery
Program To Insert An Element In An Array C Programs
How To Add Array Element At Specific Index In Javascript
0 Response to "25 Javascript Array Insert Element"
Post a Comment