27 Email Validation In Javascript Using Regular Expression W3schools
Possible duplicate of How to validate an email address in JavaScript? - jayarjo Apr 5 '19 at 9:41 Possible duplicate of JavaScript Regular Expression Email Validation - Shibon Apr 5 '19 at 9:46 Note, the the aforementioned referenced topics do not take into account unicode characters in the local part. An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. A "Unique_personal_id" and a domain. It can easily validate by using regex JavaScript. reg ex - Regular + expression First part can contains the following ASCII characters. Uppercase (A-Z) and lowercase (a-z) English letters. Digits (0-9). Characters ! Read More...
Http Www W3schools Com Js Default Asp Javascript Form
Using String Methods. In JavaScript, regular expressions are often used with the two string methods: search() and replace(). The search() method uses an expression to search for a match, and returns the position of the match. The replace() method returns a modified string where the pattern is replaced.
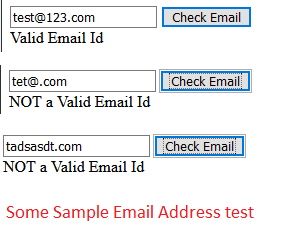
Email validation in javascript using regular expression w3schools. Javascript (JS) Email Validation without Regex. In this version of email validation we will be validating Email without using Regex In javascript, we can do this by using HTML5 Email attribute and by creating custom JavaScript, considering some points: Email Address string must contain "@" sign. "." (dot) must be last character in string to test. 1/6/2009 · Sometimes most of the registration and login page need to validate email. In this example you will learn simple email validation. First take a text input in html and a button input like this. <input type='text' id='txtEmail'/><input type='submit' name='submit' onclick='checkEmail();'/><script> function checkEmail() { var email = ... Mar 12, 2021 - An email id data will be sent to the server where it will check with the database. But you can do HTML input validation of email id text format are Right or wrong using Regular expression and JavaScript. You can validate the HTML form on the client-side so data processing will be faster than ...
15 Answers15. JavaScript to validate the phone number: The above script matches: This regular expression /^ (\ ( [0-9] {3}\)\s*| [0-9] {3}\-) [0-9] {3}- [0-9] {4}$/ validates all of the following: To break down what's happening: The group in the beginning validates two ways, either (XXX) or XXX-, with optionally spaces after the closing ... 4/7/2012 · Simple regular expression to validate email address. Below is simple regular expression to validate an email address. It will accept email address in upper case also. <script type="text/javascript"> function validateEmail(email) { var reg = /^\w+([-+.']\w+)*@\w+([-.]\w+)*\.\w+([-.]\w+)*$/ if (reg.test(email)){ return true; } else{ return false; } } </script> Regular expression to validate case-sensitive email address. Below is regular expression to validate an email address … Methods to validate Email in JavaScript. There are many ways to validate email address, the three most popular ways are: Using the regex expression. Using HTML in-built email validation. Using a third party library. As using regex expression is most popular, we will take a look at how we can validate an email address with a regex expression.
This is a very important step while validating an HTML form. In this post, we will discuss the process of email validation using JavaScript. Now we will look at the three ways to validate an email address. They are -. Using regular expression. Simple email validation by checking '@'and '. Email input field validation is mandatory before submitting the HTML form. Email validation helps to check if a user submitted a valid email address or not. Using regular expression in JavaScript, you can easily validate the email address before submitting the form. We'll use the regular expression for validate an email address in JavaScript. Using regular expressions is one of the best ways of email validation in JavaScript. It utilizes regular expressions for defining the character's pattern. Some of the examples of valid email id: Own.minesite@myuniverse . Siteowner@up.down . Siteofmine@myuniverse .
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. /w3schools/i is a regular expression. w3schools is a pattern (to be used in a search). i is a modifier (modifies the search to be case-insensitive). For a tutorial about Regular Expressions, read our JavaScript RegExp Tutorial. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
regular-expression. Stats. 600.8K views. 10 bookmarked. Email Validation in JavaScript. ... While it's fine to check the format of an email address client-side using a regex, the only way to really, totally, be sure that an email address exists is to confirm that you can send email to it, and that somebody can receive it. ... please give me a ... 38 Email Validation In Javascript Using Regular Expression W3schools. Written By Roger B Welker Friday, July 16, 2021 Add Comment. Edit. Email validation in javascript using regular expression w3schools. Github Thekondrashov Stuff My A Curated List Of Goodies. Laravel 5 8 Ajax Form Submit With Validation W3adda. Validation in JavaScript for Registration form Example. In the example we have 5 input fields:-. Name. Email id. Username. Passwords. Confirm password. These all fields are created with basic HTML code. Now coming to form validation in JavaScript using a regular expression, We will create JavaScript functions (one for each input field) that ...
One of the most popular ways of validating email in JavaScript is by using regular expressions. JavaScript uses regular expressions to describe a pattern of characters. email.js. This file contains the JavaScript code that we need for email validation. We are using regular expressions to validate the email at the client-side of a web application. 3/8/2021 · Email validation using Regular Expressions. The most common way to validate an email with JavaScript is to use a regular expression (RegEx). Regular expressions will help you to define rules to validate a string. If we summarize, an email is a string following this format: 1. First part of an email. uppercase and lowercase letters: a-z and A-Z; digits: 0-9 Mar 04, 2020 - Get code examples like "how to validate email response in javascript w3schools" instantly right from your google search results with the Grepper Chrome Extension.
After reading this article you'll be able to do advanced javascript validation using regular expressions a.k.a. RegExp for basic javascript validation read Javascript Validation - Textbox Combobox Radiobutton Checkbox. Using regular expressions the entered content can be validated with advanced conditions like the string entered shouldn't ... JavaScript email validation: A email is tricky because of its format. Some of basic checks are as follows: Presence of @ and . character. Presence of at least one character before and after the @. Presence of at least two characters after . (dot). JavaScript Form Validation: Removing Spaces and Dashes. Some undesired spaces and dashes from the user input can be removed by using the string object replace() method. The regular expression is used to find the characters and then replace them with empty spaces. Example: JavaScript Form Validation: Removing Spaces
Jun 04, 2021 - Email Validation in PHP Regular ... of email validation, and one of the most popular ways is to achieve this task by using a regular expression. https://developer.mozilla /en-US/docs/Web/HTML/Element/input/email https://stackoverflow /questions/21537690/javascript-email-val... JavaScript email validation. We can validate the email by the help of JavaScript. There are many criteria that need to be follow to validate the email id such as: email id must contain the @ and . character; There must be at least one character before and after the @. There must be at least two characters after . (dot). Email validation in javascript. JavaScript : email validation, Using regular expressions is probably the best way. You can see a bunch of tests here (taken from chromium) function validateEmail(email) { const re Email validation.
To validate the said format we use the regular expression ^[A-Za-z]\w{7,15}$, where \w matches any word character (alphanumeric) including the underscore (equivalent to [A-Za-z0-9_]). Next the match() method of string object is used to match the said regular expression against the input value. Here is the complete web document. HTML Code: Jul 15, 2012 - I was looking through some ways to validate email. I saw the one on w3schools, but that is a basic one, so I started to code something more complicated adding all those if, else if, else statements, etc. Then I came across this... function validateEmail(email){var allowed=/^([a-zA-Z0-9._-]+@[a-zA... The pattern attribute specifies a regular expression that the <input> element's value is checked against on form submission. Note: The pattern attribute works with the following input types: text, date, search, url, tel, email, and password. Tip: Use the global title attribute to describe the pattern to help the user.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. This article contains the JavaScript code examples to Validate Email in JavaScript by using different type of Regular Expression filters in client side. Sep 20, 2019 - Given an email id and the task is to validate the email id is valid or not. The validation of email is done with the help of Regular Expressions. Approach 1: RegExp – It checks for the valid characters in the Email-Id (like, numbers, alphabets, few special characters.)
Email Validation in PHP Regular Expression. PHP has different ways of email validation, and one of the most popular ways is to achieve this task by using a regular expression. This tutorial contains a PHP function to validate an email address. The function checks the input matching with patterns using regular expressions. PHP preg_match ... Valid email address in javascript - In forms when using email ID fields it is a good idea to use client side validation along with your programming language validation. The following example shows how you can validate an email address for a form. 29/7/2021 · Validating email is a very important point while validating an HTML form. In this page we have discussed how to validate an email using JavaScript : An email is a string (a subset of ASCII characters) separated into two parts by @ symbol. a "personal_info" and a domain, that is [email protected] The length of the personal_info part may be up to 64 characters long and domain name …
The pattern property sets or returns the value of the pattern attribute of an email field. The pattern attribute specifies a regular expression that the email field's value is checked against. Tip: Use the global HTML title attribute or the DOM title property to describe the pattern to help the user. Tip: Learn more about Regular Expressions in our ... Validate Zip code using JavaScript Regular expression February 20, 2008 @ 10:04 pm […] to validate zip code using regular expression in JavaScript. ... Hi , email validation code present in this site is very nice and it is very useful to validate email address using regular expressions. My sincere thanks to the programmer who posted this ...
Web Scraper Tool Screaming Frog
How To Use Regular Expressions Regex In Testim
70 480 Exam Free Actual Q Amp As Page 1 Examtopics
35 Javascript Regular Expression W3schools Javascript Overflow
Javascript Email Address Validation Using Regular Expression
The Essentials Of Regular Expressions By Sgwethan Towards
Javascript Email Validation Using Javascript With Or
Perfect Javascript Form Validation Using Regular Expressions
Email Regex Javascript Validation Regular Expression
Email Validation In Javascript In Hindi 2018 With Source
39 Email Validation Using Javascript Regular Expression
Form Validation Using Ajax Formget
How To Creating A Custom Email Validation Attribute In Mvc
Javascript Email Validation W3resource
Regular Expression Html Tag Login Information Account Loginask
Javascript Validation Stack Overflow
Jquery Email Validation Using Jquery Various Methods Qa
Email Validation In Php Regular Expression
How To Validate Form Fields Using Jquery Formden Com
Jquery Form Validation Tutorial W3schools
How To Validate An Email Address In Javascript Stack Overflow
Javascript Email Validation W3resource
Are Web Development Search Results Being Manipulated
39 Email Validation Using Javascript Regular Expression
0 Response to "27 Email Validation In Javascript Using Regular Expression W3schools"
Post a Comment