35 Get Set In Javascript Class
All you need to do is add the class to each element and then use a single line of jQuery inside a javascript function to either hide or show those classes. HTML: <div class="example"><p>Hide me</p></div> <button ... onclick="exampleClick()">Click to Hide</button> JavaScript: function exampleClick() { jQuery(".example").hide(); } Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Object Prototypes Learn Web Development Mdn
Aug 26, 2018 - Normally methods are defined on the instance, not on the class. ... JavaScript does not have a built-in way to define private or protected methods. There are workarounds, but I won’t describe them here. ... You can add methods prefixed with get or set to create a getter and setter, which ...
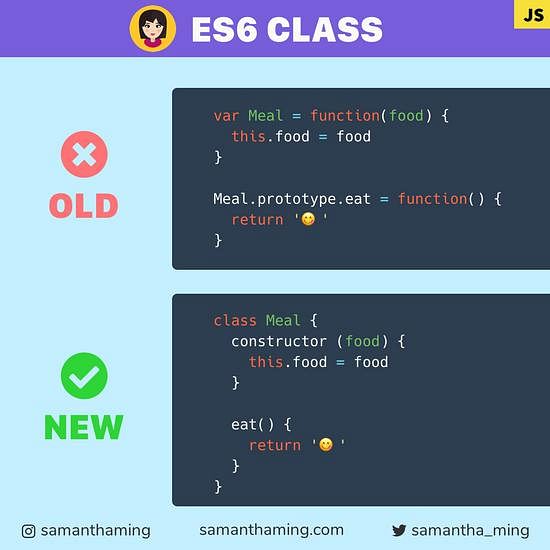
Get set in javascript class. A Set is a special type collection - "set of values" (without keys), where each value may occur only once. Its main methods are: new Set (iterable) - creates the set, and if an iterable object is provided (usually an array), copies values from it into the set. set.add (value) - adds a value, returns the set itself. Class Declarations Example: Hoisting. Unlike function declaration, the class declaration is not a part of JavaScript hoisting. So, it is required to declare the class before invoking it. Let's see an example. <script>. //Here, we are invoking the class before declaring it. var e1=new Employee (101,"Martin Roy"); var e2=new Employee (102,"Duke ... In JavaScript, you use the getElementsByClassName () method to select elements based on their classes. The getElementsByClassName () method is available on the document object and any HTML element. The getElementsByClassName () method accepts a single argument which is a string that contains one or more class names: let elements = document ...
A helper class can invoke a .NET instance method as an Action. Helper classes are useful in the following scenarios: When several components of the same type are rendered on the same page. In a Blazor Server apps, where multiple users concurrently use the same component. In the following example: Let's say v1 of an object or class (rightly or wrongly) allowed users to get / set attributes directly using dot notation. Later you realize more logic is needed — some of the attributes now need setting and/or getting logic. You could change the calling convention to disallow dot notation and require function calls — yuck. Nov 21, 2018 - To be fair, just in general I don't believe in pretending Javascript is Java and trying to encapsulate stuff (whether with "private variables" created using inner function scopes or with getter/setters). So if making Javascript act like Java floats your boat, you'll probably feel differently.
A class in JavaScript is created with the special word: function , using this syntax: className = function () { // code of the className class } A class can contain public and private variables (called also properties) and functions (also called methods). The private variables, and functions are defined with the keyword " var ". JavaScript Accessors (Getters and Setters) ECMAScript 5 (ES5 2009) introduced Getter and Setters. Getters and setters allow you to define Object Accessors (Computed Properties). JavaScript Getter (The get Keyword) In JavaScript, you can use "getters" and "setters" within your class definitions to bind a function to a property. Both "getters" and "setters" behave like r...
The following table defines the first browser version with full support for Classes in JavaScript: Chrome 49. Edge 12. Firefox 45. Safari 9. Opera 36. Mar, 2016. Classes JavaScript is different from other object-oriented languages. It is based on constructors and prototypes rather than on classes. For a long time classes were not used in JavaScript. They were introduced in ECMAScript 2015. However, they did not bring a new object oriented model based on classes. Classes just make the code easier to […] Javascript access the dom elements by id, class, name, tag, attribute and it's valued. Here you will learn how to get HTML elements values, attributes by getElementById(), getElementsByClassName(), getElementByName(), getElementsByTagName(). Selecting Elements in Document. The following javascript dom method help to select the elements in ...
The new ES6 class allows set to accept only distinct objects, to remove an element from a set, delete method can be used whereas, in the array, we have to iterate using a loop and then remove using splice and also can convert set to an array using the spread operator. Apr 04, 2017 - Good article explaining just this can be found at: coryrylan /blog/javascript-es6-class-syntax "In our class above we have a getter and setter for our name property. We use ‘_’ convention to create a backing field to store our name property. With out this every time get or set is called ... In JavaScript, this can be accomplished with the use of a getter. It is not possible to simultaneously have a getter bound to a property and have that property actually hold a value, although it is possible to use a getter and a setter in conjunction to create a type of pseudo-property. Note the following when working with the get syntax:
Set.prototype.values() - It returns all the values from the Set in the same insertion order. Syntax: set1.values(); Parameter: No parameters Returns: An iterator object that contains all the values of the set in the same order as they are inserted. Set.prototype.keys() - It also returns all the values from the Set in the insertion order. Note: - It is similar to the values() in case of Sets In JavaScript, accessor properties are methods that get or set the value of an object. For that, we use these two keywords: get - to define a getter method to get the property value set - to define a setter method to set the property value 2/8/2019 · Javascript Object Oriented Programming Front End Technology Classes allow using getters and setters. It is smart to use getters and setters for the properties, especially if you want to do something special with the value before returning them, or before you set them. To add getters and setters in the class, use the get and set keywords.
Getters and setters in JavaScript are used for defining computed properties, or accessors. A computed property is one that uses a function to get or set an object value. The basic theory is doing something like this: Apr 18, 2018 - i mean if i had to possibility to define the setters and getters in my constructor for all my fields that the form contains , guess it would be fine. I could call new Form(fields). The only thing is it should by dynamic. ... Not the answer you're looking for? Browse other questions tagged javascript ... May 11, 2021 - Technically, such class declaration works by creating getters and setters in User.prototype. ... Such features are easy to remember, as they resemble that of literal objects. ... Class fields are a recent addition to the language.
A getter returns the current value of the variable and its corresponding setter changes the value of the variable to the one it defines. Let's create a User Javascript class and define few below properties. firstName. lastName. emailId. age. We also create getter and setter methods for all the above properties. Description In JavaScript, a setter can be used to execute a function whenever a specified property is attempted to be changed. Setters are most often used in conjunction with getters to create a type of pseudo-property. It is not possible to simultaneously have a setter on a property that holds an actual value. Class can also have getter/setters to get the property value and or to set the property values. Something like below. ... Subclassing is a way you can implement inheritance in Javascript classes, ...
Define Class in JavaScript. JavaScript ECMAScript 5, does not have class type. So it does not support full object oriented programming concept as other languages like Java or C#. However, you can create a function in such a way so that it will act as a class. The following example demonstrates how a function can be used like a class in JavaScript. 15/2/2015 · In our class above we have a getter and setter for our name property. We use _ convention to create a backing field to store our name property. Without this every time get or set is called it would cause a stack overflow. The get would be called and which would cause the get to be called again over and over creating an infinite loop. Showing results for div id javascript id selector combine with class Search instead for div id javascript id selector cobine with class How to combine class and ID in JQerry selector
How to Get the Value of Text Input Field Using JavaScript In this tutorial, you will learn about getting the value of the text input field using JavaScript. There are several methods are used to get an input textbox value without wrapping the input element inside a form element. JavaScript setInterval () method. The setInterval () method in JavaScript is used to repeat a specified function at every given time-interval. It evaluates an expression or calls a function at given intervals. This method continues the calling of function until the window is closed or the clearInterval () method is called. Here Employee object name is set as private, Private modifier properties allow to access it inside the class only and will not be accessible outside. To make it simple, provided getter/setter methods for name and added public access modifier to access/manipulate outside class. This is a set and get accessor method example
To bring the traditional classes to JavaScript, ES2015 standard introduces the class syntax: a syntactic sugar over the prototypal inheritance. This post familiarizes you with JavaScript classes: how to define a class, initialize the instance, define fields and methods, understand the private and public fields, grasp the static fields and methods. Accessor properties are represented by "getter" and "setter" methods. In an object literal they are denoted by get and set: let obj = { get propName() { }, set propName(value) { } }; The getter works when obj.propName is read, the setter - when it is assigned. Jun 09, 2020 - In this tutorial, you learn how to use the TypeScript getters and setters to control access to properties of a class.
Dec 23, 2013 - When the property is accessed, the return value from the getter is used. When a value is set, the setter is called and passed the value that was set. It's up to you what you do with that value, but what is returned from the setter is the value that was passed in – so you don't need to return ... A JavaScript class is a type of function. Classes are declared with the class keyword. We will use function expression syntax to initialize a function and class expression syntax to initialize a class. const x = function() {} Copy. const y = class {} Aug 01, 2019 - In this blog post, we talk about the utility of getters and setters in modern web development. Are they useless? When does it make sense to use them?
In JavaScript, the standard way of selecting an element is to use the document.getElementById ("Id"). Of course, it is possible to obtain elements in other ways, as well, and in some circumstances, use this. For replacing all the existing classes with a single or more classes, you should set the className attribute, as follows: The class name attribute can be used by CSS and JavaScript to perform certain tasks for elements with the specified class name. Adding the class name by using JavaScript can be done in many ways. Using .className property: This property is used to add a class name to the selected element. Syntax: element.className += "newClass"; Is there any way to access controller variable set in a Method in Javascript. In the example above the value is set in constructor. But when I set it in a method it is showing as NULL in Javascript In controller: public class abc{public string varA{get;set;} public void fn(){ varA='hello';}} In VFPage: function hello(){var bool = '{!varA}';
Dart queries related to “get set javascript class” ... The getter method method must contain both database method name and an object method before you can add it to an object method. ... Learn how Grepper helps you improve as a Developer! ... 'protoc-gen-dart' is not recognized as an internal ... Dec 11, 2020 - Classes are a template for creating objects. They encapsulate data with code to work on that data. Classes in JS are built on prototypes but also have some syntax and semantics that are not shared with ES5 class-like semantics.
Making Sense Of Es6 Class Confusion Toptal
Es6 Class Constructor Super Inheritance Amp Get Set Stuffs
How To Create A Javascript Uml Class Diagram Dhtmlx Diagram
Javascript Classes Under The Hood By Majid Tajawal Medium
Javascript Es6 Classes Objects In Programming Languages
Retain The Selected List Group Item Using Vue Js Stack Overflow
How To Check If Object Is Empty In Javascript Samanthaming Com
Instantiate An Object From Javascript To Call A Method
Oop In Javascript Javascript The Freecodecamp Forum
Es6 Javasript Es6 Class Explanation With Example Qa With
Javascript Adding A Class Name To The Element Geeksforgeeks
Vro Development With Typescript Vmware Cloud Management
Javascript Classes Under The Hood By Majid Tajawal Medium
Pass Model Object From Razor View To Javascript File
Classes In Javascript Samanthaming Com
Oo Javascript Cheat Sheet By Theese Download Free From
Javascript Es6 Write Less Do More
Object Oriented Javascript For Beginners Learn Web
C Class And Object With Example
Understand Javascript Classes With Practical Examples
Javascript Es6 Classes Objects In Programming Languages
How To Create Custom Html Elements Html Javascript
Typescript Programming With Visual Studio Code
Indefinite Call To Set Function In Javascript Es6 Class
Javascript Classes An In Depth Look Part 2 By Gravity
Javascript Class Find Out How Classes Works Dataflair
Object Oriented Javascript For Beginners Learn Web
Javascript Classes Under The Hood By Majid Tajawal Medium
Refactoring Javascript Phpstorm
0 Response to "35 Get Set In Javascript Class"
Post a Comment