29 Remove All Children Javascript
The delete operator is designed to remove properties from JavaScript objects, which arrays are objects. The reason the element is not actually removed from the array is the delete operator is more about freeing memory than deleting an element. The memory is freed when there are no more references to the value. JavaScript DOM — Get the children of an element. To get all child nodes of an element, you can use the childNodes property. This property returns a collection of a node's child nodes, as a NodeList object. By default, the nodes in the collection are sorted by their appearance in the source code. You can use a numerical index (start from 0) to ...
How To Remove Only The Parent Element And Not Its Child
while (myNode.firstChild) { myNode.removeChild(myNode.lastChild); }
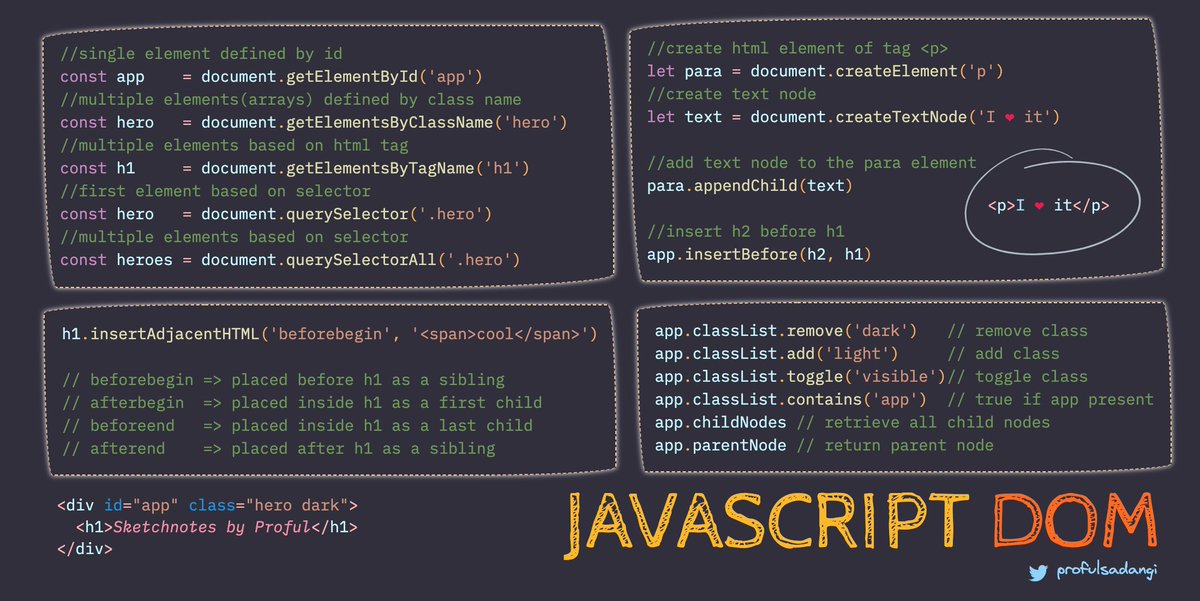
Remove all children javascript. 18/7/2019 · Second way is to remove firstChild of the parent node until the node has a children , var node= document.getElementById("parent"); while (node.firstChild) { node.removeChild(myNode.firstChild); } 3. Jul 26, 2019 - Access to XMLHttpRequest at ... policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. ... Check your Homestead.yaml (or Homestead.json) file, the path to your private key does not exist. ... using javascript when i ' m iterate localstorage ... textContent¶. The second approach is clearing textContent.It is similar to the above method. This method is much faster than innerHTML. Using textContent browsers won't invoke HTML parsers and immediately replace all children of the element with a single #text node:
Related Searches to javascript tutorial - Remove element by id javascript remove element by class jquery remove element by id javascript remove html element javascript removechild javascript remove all child nodes javascript remove array remove parent element javascript javascript remove() javascript delete all elements with class how to remove ... Definition and Usage The removeChild () method removes a specified child node of the specified element. Returns the removed node as a Node object, or null if the node does not exist. Note: The removed child node is no longer part of the DOM. Dec 26, 2017 - In this tutorial, we will go over how to create new nodes and insert them into the DOM, replace existing nodes, and remove nodes.
Assume that we have a DOM element (for example, div or span) whose name is elm, the most trivial and intuitive way to remove all its child nodes is as follows: elm.innerHTML = ''; It looks perfectly fine, and should works without any problem. In fact, I use this method to empty all contents of a DOM element in the beginning and it works as ... Removing an element with the plain JavaScript remove () method. As you might know, the DOM does not support removing an element directly. When removing an element with JavaScript, you must go to its parent first instead. This was always odd and not so straightforward. According to DOM level 4 specs, which is the current version in development ... Remove all child elements of a DOM node in JavaScript? How can I remove a child node in HTML using JavaScript? Remove the child node of a specific element in JavaScript? How to remove all child nodes from a parent node using jQuery? First and last child node of a specific node in JavaScript? Replace a child node with a new node in JavaScript?
Remove all children from a node // This is one way to remove all children from a node // box is an object reference to an element while ( box . firstChild ) { //The list is LIVE so it will re-index each call box . removeChild ( box . firstChild ) ; } Say I have a ul element with 100 children and I want to remove all children besides 10, 20, 30, 40, 50 (or all besides these with a certain class). How could I remove all children besides the 5 I ... Description: Remove all child nodes of the set of matched elements from the DOM. version added: 1.0.empty() This method does not accept any arguments. This method removes not only child (and other descendant) elements, but also any text within the set of matched elements. This is because, according to the DOM specification, any string of text ...
First, select the first child node (firstChild) and remove it using the removeChild() method. Once the first child node is removed, the next child node will automatically become the first child node. Second, repeat the first steps until there is no remaining child node. The following removeAllChildNodes() function removes all the child nodes of a node: 16/4/2019 · Remove all the child elements of a DOM node in JavaScript. Last Updated : 16 Apr, 2019. Child nodes can be removed from a parent with removeChild (), and a node itself can be removed with remove (). Another method to remove all child of a node is to set it’s innerHTML=”” property, it is an empty string which produces the same output. Aug 25, 2020 - Access to XMLHttpRequest at ... policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. ... Check your Homestead.yaml (or Homestead.json) file, the path to your private key does not exist. ... using javascript when i ' m iterate localstorage ...
Sep 04, 2020 - while (myNode.firstChild) { myNode.removeChild(myNode.lastChild); } The querySelectorAll() method returns a static / non-live NodeList collection of elements when there's at least one match, and an empty NodeList if there are no matches. With that information, we can simply loop over the NodeList collection returned by querySelectorAll() and remove the DOM nodes linearly.. We can use the following loops to loop over the NodeList object (which is iterable ... 15/3/2020 · Removing all Child elements. First, we are accessing the parent element by using its id attribute. const div = document.getElementById('container'); Next, we are removing all child elements by assigning an empty string to the parent element innerHTML property. div.innerHTML = " ";
String replacements in JavaScript are a common task. It still can be tricky to replace all appearances using the string.replace() function. Removing all whitespace can be cumbersome when it comes to tabs and line breaks. Luckily, JavaScript's string.replace() method supports regular expressions. This tutorial shows you how to remove all ... Aug 25, 2020 - Access to XMLHttpRequest at ... policy: No 'Access-Control-Allow-Origin' header is present on the requested resource. ... Check your Homestead.yaml (or Homestead.json) file, the path to your private key does not exist. ... using javascript when i ' m iterate localstorage ... How to js remove all children (Javascript Scripting Language) By: xtotiza Amit. editAnswer. call_missed_outgoingFollow. report Report. Answer(s) available: 1. Anju rrnwopyn, BLANCHING MACHINE OPERATOR, Digital Media, Operational Excellence. 1.
Code language: JavaScript (javascript) How it works: First, get the ul element with the id menu by using the getElementById() method. Then, remove the last element of the ul element by using the removeChild() method. The menu.lastElementChild property returns the last child element of the menu. Put it all together. Remove its child node until it doesn't have any children. Option 2 B: Looping to remove every lastElementChild:. This approach preserves all non-Element (namely #text nodes and <!-- comments -->) children of the parent (but not their descendants) - and this may be desirable in your application (e.g. some templating systems that use inline HTML comments to store template instructions).This approach wasn't used until recent years as Internet Explorer ...
Remove an Element Node The removeChild () method removes a specified node. When a node is removed, all its child nodes are also removed. This code will remove the first <book> element from the loaded xml: Removing HTML Element children with JavaScript. Tuesday, January 24, 2006. Just a quick fyi, but if you are doing work in JavaScript and need to dynamically remove all of the childNodes from a DOM element, make sure to do it with a while loop, and not a for loop. For example, this is bad: To remove all child nodes of an element, you can use the element's removeChild () method along with the lastChild property. The removeChild () method removes the given node from the specified element. It returns the removed node as a Node object, or null if the node is no longer available. Here is an example code snippet:
Sep 21, 2020 - I have the following HTML: hello world And ... all of the child elements of a DOM node in JavaScript? Oct 27, 2020 - To remove child elements, set the innerHTML to ‘’.ExampleFollowing is the code − while (myNode.firstChild) { myNode.removeChild(myNode.lastChild); }
Jun 14, 2021 - The Node.removeChild() method removes a child node from the DOM and returns the removed node · The removed child node still exists in memory, but is no longer part of the DOM. With the first syntax form shown, you may reuse the removed node later in your code, via the oldChild object reference Javascript Dom Remove All Children Of An Element. The Document Object Model Eloquent Javascript. Create Insert Replace And Delete Dom Nodes With Javascript. How To Add An Element To The Dom. 7 Javascript Data Structures You Must Know Dev Community ... Jul 26, 2019 - while (myNode.firstChild) { myNode.removeChild(myNode.lastChild); }
Program to remove all nodes with only one child from a binary tree in Python? How to select ALL children (in any level) from a parent in jQuery? How to expand JTree row to display all the nodes and child nodes in Java; Remove all child elements of a DOM node in JavaScript? How to remove all style attributes using jQuery? Javascript removes all descendants of a div / node I've been working on some memory leaks in Javascript for some time now. I've come to a point where I feel the only solution for at least some of the leaks is to remove a given div and then ALL of it's descendants. Meaning children, grandchildren, etc Aug 28, 2019 - The loop ends when all children are removed. The first, in most performance benchmarks I checked, looks like being the fastest solution. Download my free JavaScript Beginner's Handbook and check out my JavaScript Masterclass!
Given an HTML element and the task is to remove the HTML element from the document using JavaScript. Approach: Select the HTML element which need to remove. Use JavaScript remove() and removeChild() method to remove the element from the HTML document. Example 1: This example uses removeChild() method to remove the HTML element.
Javascript Reference Cheat Sheet
Delete All Child Elements Jquery Code Example
Enable Or Disable Inherited Permissions For Objects In
Remove All Elements From Parent Remove All Elements From
Enable Or Disable Inherited Permissions For Objects In
Javascript Memory Management Masterclass Speaker Deck
Dom Manipulation With Javascript Engineering Education
Puppeteer Documentation Devdocs
How To Completely Remove Uninstall Drivers On Windows 10
Anakin Using Javascript Selfremoveallchildren Mister
9 Ways To Turn Off Parental Controls Wikihow
Protect Your Family From Sources Of Lead Us Epa
Proful Sadangi On Twitter Javascript Dom Cheatsheet
Systems Serology Detects Functionally Distinct Coronavirus
Removing A Child Node From Javascript Modern Web Design
How To Remove Parent Element Except Its Child Element Using
Delete A Node With Two Children In A Binary Search Tree Test
Javascript Remove Removes Element But Still Can Find And
Wisdom Teeth Removal What To Expect Before During And
Create Add And Delete Elements Of The Web Page Javascript
Demystifying A Common Bug Encountered By Developers New To
Css Selector Guide For Google Tag Manager Simo Ahava S Blog
Javascript Dom Get The First Child Last Child And All
How To Remove All Children From A Dom Element
How To Find Event Listeners On A Dom Node When Debugging Or
I M Confused About A Javascript Problem Please Help Chegg Com
Remove All Children Of The Node In Javascript By
0 Response to "29 Remove All Children Javascript"
Post a Comment