35 Javascript Map Reduce Filter
Nov 17, 2019 - Filter, map, and reduce are methods available to use to manipulate data in an array. Since JS introduced these methods in 2011, they’ve become some of the most common methods used for traversing… Jun 21, 2021 - In JavaScript we have These three methods as parts of Array.prototype methods, but what are the differences between them and what exactly they do?
Comparing Js Iteration Methods Map Filter Foreach Reduce
JavaScript map(), reduce(), and filter() are pure functions that return a new array and does not modify the original array. These functions are array methods. Each one will iterate over the array and perform some kind of transformation or computation. The map() function will return a new array based on the result of the function.
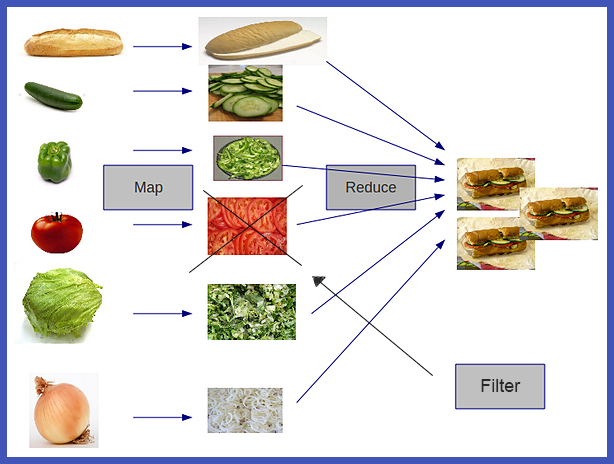
Javascript map reduce filter. This tutorial explains how to use map (), reduce (), and filter () function in javascript programming language. Nowadays these function are widely used during react and react native application development. These are 3 really powerful array functions: map returns an array with the same length, Just like map () and filter (), reduce () also executes the callback for each element of the array. To better understand reduce, we first need to understand two terms: " accumulator" and "reducer". The accumulator is the value that we end up with, and the reducer is what action we will perform in order to get to one value. Jun 08, 2020 - When working with arrays in JavaScript, there are a number of methods at our disposal. I always think...
There are many contexts in which this is useful, such as mapping AJAX results to React components with map, removing extraneous data with filter, and using reduce. These functions, called "Array Extras", are abstractions over for loops. There is nothing you can do with these functions that you can't achieve with for, and vice versa. JavaScript: Conhecendo map (), filter () e reduce () Dando continuidade ao meu artigo anterior, onde demonstrei a diferença entre Map & forEach, vejamos agora um outro exemplo com map (), filter () e reduce (). Para os nossos exemplos, iremos utilizar o seguinte array de objetos contendo: nome, idade e tipo. Jun 14, 2021 - In the previous post we talked about First Class Functions and how JavaScript treat functions as a...
Cara menggunakan Map, Filter, dan Reduce di JavaScript. Indonesian (Bahasa Indonesia) translation by Andy Nur (you can also view the original English article) Pemrograman fungsional telah membuat cukup banyak percikan di dunia pengembangan akhir-akhir ini. Dan untuk alasan yang baik: Teknik fungsional dapat membantumu menulis lebih banyak kode ... 20/3/2016 · The syntax for the reduce array method in JavaScript is: let newArray = arr.filter(callback(currentValue, accumulatedValue) { // return the accumulated value, given the current and previous accumulated value }, initialValue[, thisArg]); map creates a new array by transforming every element in an array, individually. In this article, I am going to explain some functions like .map(), .reduce(), filter(), find(), etc. which play an important role when one wants to transform the array of play with JSON responses.
The cause of the lack of map/reduce/filter on Map/Set collections seem to be mainly conceptual concerns. Should each collection type in Javascript actually specify its own iterative methods only to allow this Higher order functions can help you to step up your JavaScript game by making your code more declarative. That is, short, simple, and readable. A Higher Order Function is any function that returns a function when executed, takes a function as one or more of its arguments, or both. If you have used any of the Array methods like map or filter, or ... 13/4/2018 · map, filter, reduce, find. Those are 3 really powerful array functions: map returns an array with the same length, filter as the name implies, it returns an array with less items than the original array; reduce returns a single value (or object) find returns the first items in an array that satisfies a condition
The video explains how javascript .filter(), map() and .reduce() methods work.=========================================================------------------AUDI... Reduce works on an array but can return anything you want it to return. As the name speaks for itself, it can be reduced to anything and can behave like map, find, filter or any other JavaScript array function. The above code snippet works on an array and reduces to compute the total value of items in the array. Unlike the map and filter methods, the reduce method expects two arguments: an identity element, and a lambda expression. You can think of the identity element as an element which does not alter ...
Feb 03, 2020 - Prerequisites This post assumes that you understand the basics of JavaScript and are comfo... Just like.map (),.reduce () also runs a callback for each element of an array. What's different here is that reduce passes the result of this callback (the accumulator) from one array element to... 8/5/2020 · The map (), reduce () and filter () are array functions that transform the array according to the applied function and return the updated array. They are used to write simple, short and clean codes for modifying an array instead of using the loops.
To solve this problem, we're going to utilize map (), reduce (), and filter () to accomplish the same goal. The first thing we need to do with our data is filter out the cats. We can use filter () to accomplish this. Our filter method takes the pet as input and will return true on a an animal if its type is equal to dog: Jan 25, 2021 - JavaScript has a lot of kinds of methods when it comes to the operations performed on Arrays. Over time, many redundant and repeated operations are made easy through the construction of built-in… Apr 28, 2021 - Map, reduce, and filter are all array methods in JavaScript. Each one will iterate over an array and perform a transformation or computation. Each will return a new array based on the result of the function. In this article, you will learn why and how to use each one. Here
Apr 02, 2019 - Map, reduce, and filter are three very useful array methods in JavaScript that give developers a ton of power in a short amount of space. Let’s jump right JavaScript Functional Programming — map, filter and reduce Published: 24 Oct 2017 Even if you don't know what functional programming is you've probably been using map, filter and reduce just because they're so incredibly useful and make your code stink less by allowing you to write cleaner logic. Jul 29, 2019 - A basic definition of an Array goes like, An array is a special variable, which can hold more than one value at a time. Arrays in JavaScript, are single variables used to store different kind of elements. One of the primary needs in dealing with a...
Dec 03, 2019 - A higher-order function is a function that takes one or more functions as arguments or returns a function as its result. map, filter, andreduce are all higher order functions, which take a function… .map(), .filter() และ .reduce() สามทหารเสือในการจัดการกับ Array[…] หมดยุคของ for loop Win Eiwwongcharoen Map/Reduce/Filter/Find are slow because of many reason, some of them are. They have a call back to execute so that act as a overhead . There are lot of corner cases that javascript function consider like getters, sparse array and checking arguments that are passed is array or not which adds up to overhead. I found a lib. that reimplement ...
Map, Filter, Reduce. Map, Filter, and Reduce are paradigms of functional programming. They allow the programmer (you) to write simpler, shorter code, without neccessarily needing to bother about intricacies like loops and branching. Essentially, these three functions allow you to apply a function across a number of iterables, in one fell swoop. The reduce method. The method reduce () allows for more general forms of array processing, and it's possible to show that both filter and map can be derived as special applications of reduce. It iterates over each item in an array and returns a single value. This is achieved with a callback that it takes as an argument. 28/11/2020 · Use Javascript -.map ().find ().reduce () and.filter () | Anansewaa Javascript comes with a couple of functions that make things easy. You probably have heard of.map (),.find (),.reduce () and.filter (). But, how and when can we use these functions to simplify our code.
May 24, 2020 - In this article, I have explained the implementation of JavaScript’s Map, Filter, and Reduce method. 2 weeks ago - The reduce() method executes a user-supplied “reducer” callback function on each element of the array, passing in the return value from the calculation on the preceding element. The final result of running the reducer across all elements of the array is a single value. 15 Useful JavaScript Examples of .map(), .reduce() and .filter() When you read about Array.reduce and how cool is that, the following and sometimes the only thing you see, is an… medium
Map, reduce, and filter are all array methods in JavaScript. Each one will iterate over an array and perform a transformation or computation. Each will return a new array based on the result of the function. 2 weeks ago - The filter() method creates a new array with all elements that pass the test implemented by the provided function. reduce () is written like map () and filter (), but the inner function will take two parameters. It also has an extra spot at the end where we'll insert an empty object {}. This could also be an empty array [], but in our example it will be an object. This extra parameter is the intial value, which is 0 by default.
The method filter () will test every element of the array against our test in the callback function returning a new array with the values that return true in the callback. Like forEach () and map (), we are going to have three arguments in our callback function and an optional parameter to assign the value of this inside our callback function ...
Map Filter Reduce And More In Swift Explained With Emoji By
Writing Your Own Filter Map And Reduce Functions In
Javascript Array And Its Functions Map Reduce And
Don T Object Ify Me An Introduction To Functional
Traverse Array Of Objects Using Map Filter Reduce Or Object
An Illustrated And Musical Guide To Map Reduce And Filter
Learn To Chain Map Filter And Reduce Method In Javascript
Functional Programming In Js Map Filter Reduce Pt 5
Simplify Your Javascript With Map Reduce And Filter
Simplify Your Javascript Use Map Reduce And Filter
Functional Programming In Js Map Filter Reduce Pt 5
68 Javascript Difference Between Sort Filter Map
Understanding Map Filter And Reduce In Javascript
How To Use Map Filter And Reduce In Javascript
Functional Programming In Js Map Filter Reduce Pt 5
How To Write Your Own Map Filter And Reduce Functions In
Javascript Map Filter Reduce Es6 By Pradeep
Explain It To Me Like I M Five Map Reduce Amp Filter
Javascript Kingdom On Twitter Map Filter Reduce As Simple
An Illustrated And Musical Guide To Map Reduce And Filter
Javascript Map Reduce And Filter Js Array Functions
What Are Map Filter And Reduce Quora
Javascript Filter Map And Reduce On Real World Examples
Javascript Map Amp Filter Dev Community
Javascript Array For Interviews Filter Map Reduce And Isarray
4 Javascript Array Methods You Must Know 30 Seconds Of Code
A Definitive Guide To Map Filter And Reduce In
How To Explain Javascript Filter Map And Reduce To
Joel Thoms Javascript On Twitter Tip Filter And Then
Javascript Map Filter Techyowls
Map Filter And Reduce In Javascript By Nikhil K
How To Simplify Your Codebase With Map Reduce And
Functional Programming For Oo Programmers Part 2
0 Response to "35 Javascript Map Reduce Filter"
Post a Comment