33 Find Item In Array Javascript
The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating no element passed the test. index will be -1 which means the item was not found. Because objects are compared by reference, not by their values (differently for primitive types). The object passed to indexOf is a completely different object than the second item in the array.. You can use the findIndex value like this, which runs a function for each item in the array, which is passed the element, and its index.
Find Equal Elements In Array Javascript Code Example
16/9/2012 · var array = [ { name:"string 1", value:"this", other: "that" }, { name:"string 2", value:"this", other: "that" } ]; You can use the following function to search for items. const search = what => array.find(element => element.name === what); And you can check whether the item was found or not.
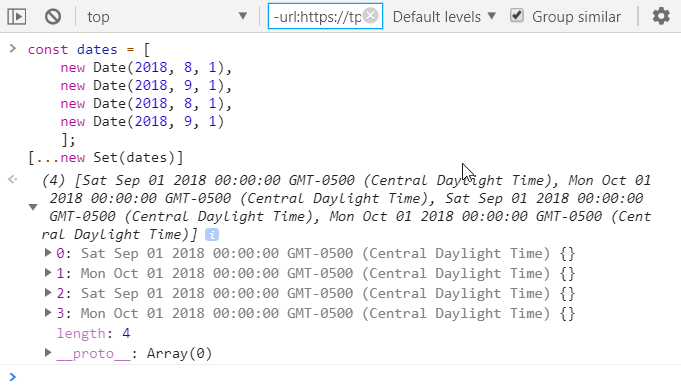
Find item in array javascript. Array.find () method runs the callback function on every element present in the array and returns the first matching element. Otherwise, it returns undefined. Find the object from an array by using property The findIndex () method returns the index of the first array element that passes a test (provided by a function). The method executes the function once for each element present in the array: Javascript: Find Object in Array. To find Object in Array in JavaScript, use array.find () method. javascript array find () function returns the value of the first item in the provided array that satisfies the provided testing function.The find () function returns the value of the first item in an array that pass the test (provided as a function).
The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex (). If you need to find the index of a value, use Array.prototype.indexOf (). If you want to get an array of foo properties, you can do this with the map() method: myArray.filter(x => x.id === '45').map(x => x.foo); Side note: methods like find() or filter() , and arrow functions are not supported by older browsers (like IE), so if you want to support these browsers, you should transpile your code using Babel (with the ... Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
Definition and Usage. The includes () method returns true if an array contains a specified element, otherwise false. includes () is case sensitive. In JavaScript, there are multiple ways to check if an array includes an item. You can always use the for loop or Array.indexOf () method, but ES6 has added plenty of more useful methods to search through an array and find what you are looking for with ease. 24/6/2020 · For all these use cases, JavaScript's Array.prototype methods have you covered. In this article, we will discuss four methods we can use to search for an item in an array. These methods are: Filter; Find; Includes; IndexOf; Let's discuss each of them. Array.filter() We can use the Array.filter() method to find elements in an array that meet a certain condition.
The Array.prototype.findIndex() method returns an index in the array if an element in the array satisfies the provided testing function; otherwise, it will return -1, which indicates that no element passed the test. It executes the callback function once for every index in the array until it finds the one where callback returns true. Method 2: Create an empty object and loop through first array. Check if the elements from the first array exist in the object or not. If it doesn't exist then assign properties === elements in the array. Loop through second array and check if elements in the second array exists on created object. Method 2 — Using Array.find() The Array.find() method takes a callback function as parameter and executes that function once for each element present in the array, until it finds one where the function returns a true value. If the element is found it returns the value of the element, otherwise undefined is returned.
The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found. Read the tutorial and learn the methods of getting the last item in the array. Get to know the methods and their peculiarities to find the best solution. ... JavaScript arrays are zero-indexed, where the first element is at index 0, and the last element is at the index equivalent to the value of the length property minus 1. ... I suggest using JavaScript's Array method filter () to identify an element by value. It filters data by using a "function to test each element of the array. Return true to keep the element, false otherwise.."
findIndex() method is used to find the index of first element in the array based on a function parameter. If the element is not found, it returns -1 . const givenArr = [ { id : 0 , name : "Alex" } , { id : 2 , name : "Bob" } , { id : 2 , name : "Charlie" } ] ; const obj = { id : 2 , name : "Charlie" } ; if ( givenArr . findIndex ( e => ( e . id === obj . id && e . name === obj . name ) ) === - 1 ) { console . log ( "Not found.." Array.prototype.findIndex () The findIndex () method returns the index of the first element in the array that satisfies the provided testing function. Otherwise, it returns -1, indicating that no element passed the test. See also the find () method, which returns the value of an array element, instead of its index. The arr.find () method is used to get the value of the first element in the array that satisfies the provided condition. It checks all the elements of the array and whichever the first element satisfies the condition is going to print.
Here are a few ways to remove an item from an array using JavaScript. All the method described do not mutate the original array, and instead create a new one. If you know the index of an item. Suppose you have an array, and you want to remove an item in position i. 9/9/2020 · The JavaScript Array.find method is a convenient way to find and return the first occurence of an element in an array, under a defined testing function. When you want a single needle from the haystack, reach for find ()! When to Use Array.find The find() method returns the value of the array element that passes a test (provided by a function). The method executes the function once for each element present in the array: If it finds an array element where the function returns a true value, find() returns the value of that array element (and does not check the remaining values)
The $.inArray () method is similar to JavaScript's native .indexOf () method in that it returns -1 when it doesn't find a match. If the first element within the array matches value, $.inArray () returns 0. Because JavaScript treats 0 as loosely equal to false (i.e. 0 == false, but 0 !== false), to check for the presence of value within array ... JavaScript Array find() In this tutorial, we will learn about the JavaScript Array find() method with the help of examples. The find() method returns the value of the first array element that satisfies the provided test function. 23/9/2020 · The findIndex() method. The Array findIndex() method returns the index of first matched element in array that satisfies a condition. This method also takes a function argument which returns true or false. The findIndex() method works the same way as find() method except find() returns the first matched element and findIndex() returns the index of the first matched element.
Find Object In Array With Certain Property Value In JavaScript July 7, 2020 by Andreas Wik If you have an array of objects and want to extract a single object with a certain property value, e.g. id should be 12811, then find () has got you covered. 10/5/2020 · Today, you'll learn a useful trick to find all matching items in an array by using the Array.filter () method. The Array.filter () method creates a new array by iterating over all elements of an array and returns those that pass a certain condition as an array. The callback function passed as an argument takes in up to three optional parameters. 6/7/2020 · This method can take an additional argument which defines the index from where we want to start looking, leave empty if you want to check the whole Array. Let's continue with more methods: const arr = [1,2,3,4,5]; !!arr.find( (a) => a === 2); // true !!arr.find( (a) => a === 6); // false. Enter fullscreen mode.
Javascript array find. Javascript Array find () is a built-in function used to get a value of the first element in the Array that meets the provided condition. The find () function accepts function and thisValue as arguments. The function takes element, index, and array. If you need an index of the found item in the Array, use the findIndex () .
Javascript Array Find The Most Frequent Item Of An Array
Javascript Replace Value In Array Inside Object Code Example
C Program To Find Largest Number In An Array
How To Move An Array Element From One Array Position To
Javascript Array Find How To Find Element In Javascript
How To Find An Item In A Javascript Array Performance Tests
Javascript Array Find Duplicate Values In A Array W3resource
Javascript Array Distinct Ever Wanted To Get Distinct
3 Ways To Detect An Array In Javascript
Array Find Javascript Code Example
How To Search In An Array Of Objects With Javascript
Find The Index Number Of A Value In A Powershell Array
Javascript Array Find Method Dev Community
Javascript Array And Object Lookups Have About The Same
Find Object By Id In An Array Of Javascript Objects
Find The Index Of An Item In A Javascript Array The
5 Ways To Check If An Array Contains A Value In Javascript
How To Find An Item In A Javascript Array Performance Tests
C Java Php Programming Source Code Javascript Find
How To Remove Array Duplicates In Es6 By Samantha Ming
Javascript Array Find How To Find Element In Javascript
Removing Items From An Array In Javascript Ultimate Courses
Javascript Find An Object In Array Based On Object S
How To Get The Length Of An Object In Javascript
5 Way To Append Item To Array In Javascript Samanthaming Com
Find The Min Max Element Of An Array In Javascript Stack
Find An Item By Property In An Array Without Using Es6 Methods
Javascript Array A Complete Guide For Beginners Dataflair
How To Get The Index Of An Item In An Array In Javascript
9 Ways To Remove Elements From A Javascript Array
Should You Use Includes Or Filter To Check If An Array
0 Response to "33 Find Item In Array Javascript"
Post a Comment