25 Create Node Html Javascript
I've touched on DocumentFragment to create and store DOM nodes for performance in the past, but that post illustrated element creation via document.createElement: // Use a DocumentFragment to store and then mass inject a list of DOM nodes var frag = document.createDocumentFragment(); for(var x = 0; x 10; x++) { var li = document.createElement ... To add text to the <p> element, you must create a text node first. This code creates a text node: const node = document.createTextNode("This is a new paragraph.");
Node Js Tutorial For Beginners 17 Serving Html Pages
11/5/2018 · Now that we have a server up and running, let’s see how to render html with Node.js. To do this, we of course need an html file to serve so we can create and populate an index.html file now. index.html <!doctype html> <html lang="en"> <head> <title>Hello, world!</title> </head> <body> <h1>Hello, world!</h1> </body> </html>
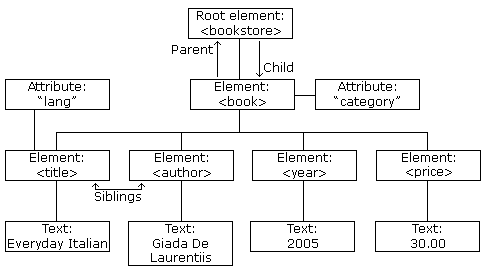
Create node html javascript. In this tutorial you'll learn how to create HTML elements using JavaScript. In other words, we'll dynamically create HTML elements with JavaScript code. Get... The document.createElement () is used to dynamically create an HTML element node with the specified name via JavaScript. This method takes the name of the element as the parameter and creates that element node. In an HTML document, the document.createElement () method creates the HTML element specified by tagName, or an HTMLUnknownElement if tagName isn't recognized.
Code language: HTML, XML (xml) 3) Creating a script element example. Sometimes, you may want to load a JavaScript file dynamically. To do this, you can use the document.createElement() to create the script element and add it to the document. The following example illustrates how to create a new script element and loads the /lib.js file to the ... For creating server on Node JS we have used "http" module. For using any module in Node JS we have to use "require" module. So fist we import the "http" module. var http=require ("http"); Now we are creating a server. For creating the server we have to use 'createServer 'method of http module and this method takes 2 ... DOCTYPE html > < html lang = " en " > < head > < title > createTextNode example </ title > < script > function addTextNode (text) {var newtext = document. createTextNode (text), p1 = document. getElementById ("p1"); p1. appendChild (newtext);} </ script > </ head > < body > < button onclick = " addTextNode ('YES! '); " > YES! </ button > < button onclick = " addTextNode ('NO! '); " > NO! </ button > < button onclick = " addTextNode …
Template element. A built-in <template> element serves as a storage for HTML markup templates. The browser ignores it contents, only checks for syntax validity, but we can access and use it in JavaScript, to create other elements. In theory, we could create any invisible element somewhere in HTML for HTML markup storage purposes. These days you can do pretty much anything with JavaScript and HTML. Thanks to Node-WebKit, we can even create desktop applications that feel native, and have full access to every part of the operating system. JavaScript Create Element. Creating DOM elements using js, adding text to the new element and appending the child to the Html body is not a hard task, it can be done in just a couple of lines of code. Let's see in the next example: var div = document.createElement ('div'); //creating element div.textContent = "Hello, World"; //adding text on ...
Definition and Usage The createElement () method creates an Element Node with the specified name. Tip: After the element is created, use the element.appendChild () or element.insertBefore () method to insert it to the document. New elements can be dynamically created in JavaScript with the help of createElement () method. The attributes of the created element can be set using the setAttribute () method. The examples given below would demonstrate this approach. Example 1: In this example, a newly created element is added as a child to the parent element. But before we have a look at them, let's first create a sample JavaScript (Node) project that we want to provide to our clients as a binary executable so that they do not need to install Node.js ...
Node.nodeValue Returns / Sets the value of the current node. Node.ownerDocument Read only Returns the Document that this node belongs to. If the node is itself a document, returns null. Node.parentNode Read only Returns a Node that is the parent of this node. If there is no such node, like if this node is the top of the tree or if doesn't participate in a tree, this property returns null. Create a text node with some text which will display as a link. Append the text node to the anchor <a> element. Set the title and href property of the <a> element. Append <a> element in the body. 16/8/2020 · I had the need to serve an HTML page from a Node.js server, and this is the simplest code that does the trick: const http = require ( 'http' ) const fs = require ( 'fs' ) const server = http . createServer (( req , res ) => { res . writeHead ( 200 , { 'content-type' : 'text/html' }) fs . createReadStream ( 'index.html' ). pipe ( res ) }) server . listen ( process . env .
The HTML DOM allows you to add and remove nodes through JavaScript. JavaScript create element action can be done when you add an element to the HTML DOM. You will have to create element JavaScript (element node) first, then append it to an element that already exists. There are specific methods used to create, remove, and replace node elements. Need to add some content, or update a certain part of the page using Javascript? There are 2 common ways to create and insert HTML elements in Javascript. By directly changing the inner HTML of an element - document.getElementById ("ID").innerHTML = "<p>Text</p>"; By creating and appending a new element. var para = document.createElement ("p"); In this example, we are going to create a list of bookmarks, append bookmarks to parent element and then present it in HTML using plain JavaScript. The starting point is an index.html file that consist of a div element with an ID of bookmarks. The ID is an important and necessary factor in order to locate the element so that we can manipulate it.
CSS file. Now we have the CSS, in other words, the style of our page. To modify each element in our HTML file, we can select them using ids, classes or the tag names themselves, though the last ... You can create an element using document.createElement. After creation you can add attributes. If you want the element to show up in your document, you have to insert in into the DOM-tree of the document. Try playing around with this code: 6/10/2019 · 3 Answers3. const fs = require ('fs'); const parse = require ('node-html-parser').parse; fs.readFile ('index.html', 'utf8', (err,html)=> { if (err) { throw err; } const root = parse (html); const body = root.querySelector ('body'); //body.set_content ('<div id = "asdf"></div>'); body.appendChild ('<div id = "asdf"></div>'); console.log ...
The createTextNode() method creates a Text Node with the specified text. Tip: Use the createElement() method to create an Element Node with the specified name. Tip: After the Text Node is created, use the element.appendChild() or element.insertBefore() method to append it to an element. Understanding NodeJS: A brief introduction. Javascript is a simple and modern language that was initially created to add dynamic behavior to websites inside the browser. When a website is loaded, Javascript is run by the browser's Javascript Engine and converted into a bunch of code that the computer can understand. 25/6/2019 · The file should be saved in your default node.js project directory. Run the code by initiating the file at the command prompt like a regular node js file, and then open the port in a browser. Take some time now and study the syntax properly. You’ll notice that I used html+= frequently. So you can play around with it to get your desired style.
The following snippet inserts a new node after the first list item: First, select the ul element by its id ( menu) using the getElementById () method. Second, create a new list item using the createElement () method. Third, use the insertBefore () method to insert the list item element before the next sibling of the first list item element. Other DOM methods we need to mention. appendChild is a method of a Node, it appends an element to a given element. This is an essential method for actually adding elements to the HTML document. We call it after the createElement method and finalize the creation of the element. We can add the new element. In Node.js, a module is a collection of JavaScript functions and objects that can be used by external applications. In this tutorial, you will create a Node.js module organized with npm that suggests what color web developers should use in their desig
12/5/2020 · function htmlToElem (html) { let temp = document.createElement('template'); html = html.trim(); // Never return a space text node as a result temp.innerHTML = html; return temp.content.firstChild; } let td = htmlToElem('<td>foo</td>'), div = htmlToElem('<div><span>nested</span> <span>stuff</span></div>'); alert(td); alert(div); /* @param {String} HTML representing any number of sibling elements @return {NodeList} */ function htmlToElems (html… Browsers parse the HTML document and construct the nodes based on the contents of the HTML file. All nodes are created by the browser for the initial load. However, it is possible to dynamically inject new nodes using the document.createElement() method. Create element dynamically inside document body Create a new file called html.js. This file will be used later to return HTML text in an HTTP response. We'll put the template code here and copy it to the other servers that return various types. In the terminal, enter the following:
That was the HTML example. Now let's create the same div with JavaScript (assuming that the styles are in the HTML/CSS already). Creating an element. To create DOM nodes, there are two methods: document.createElement(tag) Creates a new element node with the given tag:
How To Build A Node Js Application With Docker Digitalocean
What Is A Node In Javascript Stack Overflow
How To Load Html In Node Js Code Example
Why Use Node Js A Comprehensive Tutorial With Examples Toptal
Node Fs Nodejs Create File Read File Write To File
How To Create Html Object In Javascript Code Example
Build Node Js Apps With Visual Studio Code
How To Create And Save An Image With Node Js And Canvas
Using Node Js To Read Html File And Send Html Response
Github Kussberg Node Html To Pdf Node Js Create A Pdf From
Practical Html Page Builder Creating Cli Using Javascript
Esp8266 Web Server Fast Development Of Html Js With Node
Html Server On Heroku With A Node Js Static Web Server By
Read Html Form Data Using Get And Post Method In Node Js By
Puppeteer Html To Pdf Generation With Node Js Risingstack
Create A Simple Http Server With Node Js Ilovecoding
Create Pdfs With Node Js And Puppeteer Reverentgeek
Creating A Dynamic Html Table With Javascript By Daniel
Can T Load Javascript To Html Page In Node Js With Netbeans
Rendering Html Pages As An Http Server Response Using Node Js
Render Html In Node Js Vegibit
0 Response to "25 Create Node Html Javascript"
Post a Comment