30 Javascript Regex Insert Character
Characters Meaning; x|y: Matches either "x" or "y". For example, /green|red/ matches "green" in "green apple" and "red" in "red apple". [xyz] [a-c] A character class. Matches any one of the enclosed characters. You can specify a range of characters by using a hyphen, but if the hyphen appears as the first or last character enclosed in the square brackets it is taken as a literal hyphen to be ... A "wordly" character: either a letter of Latin alphabet or a digit or an underscore _. Non-Latin letters (like cyrillic or hindi) do not belong to \w. For instance, \d\s\w means a "digit" followed by a "space character" followed by a "wordly character", such as 1 a. A regexp may contain both regular symbols and character classes.
A Guide To Javascript Regular Expressions
19/8/2021 · You need to add the above Javascript code in your JS file or in HTML things you need to note here is. Add ID to the form in your HTML. Add ID to the input in your HTML. This is an example of the input and form tags. <form id="form"> <input type="text" id="text" required> </form>.
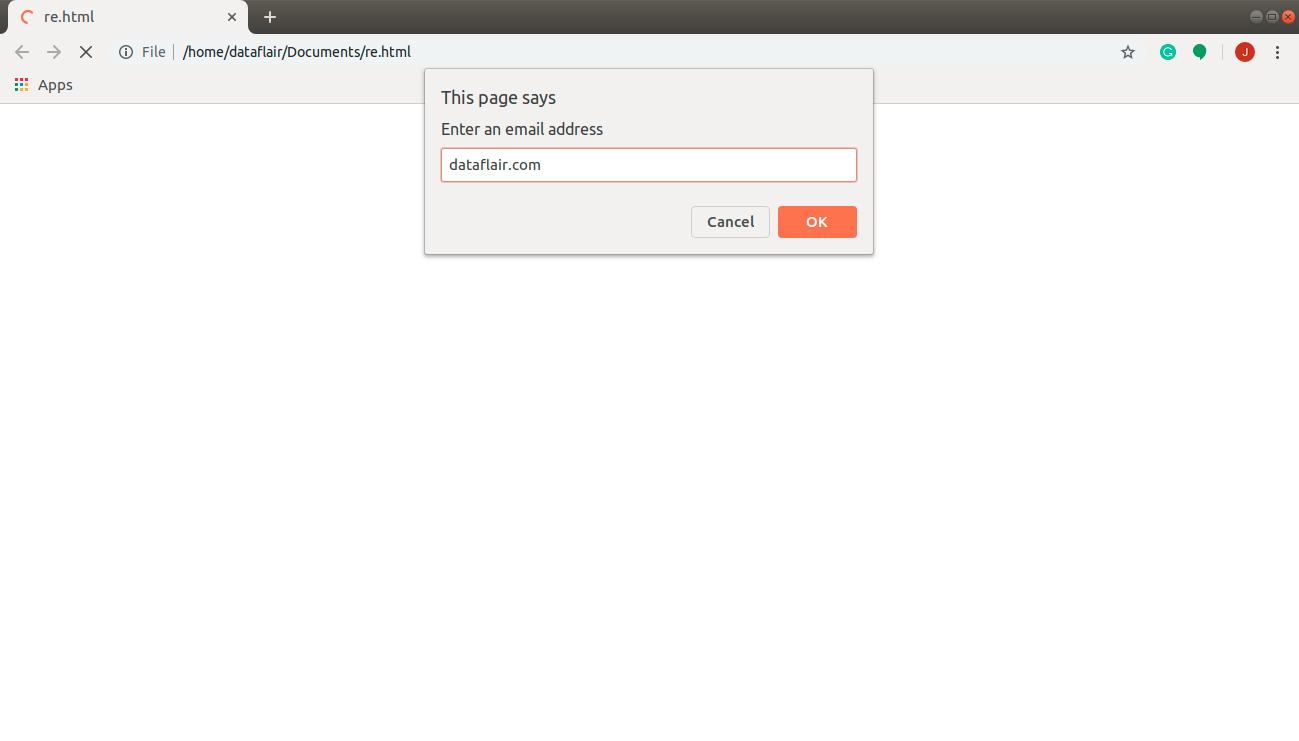
Javascript regex insert character. Regex (a.k.a. Regular Expressions) is a tool for finding words or patterns of text within strings. It is also used to validate text, for example to check that you have entered a valid email address. It's a swiss army knife that you will use again and again in both web and back-end programming using Javascript. Here [0-9A-F] has two ranges: it searches for a character that is either a digit from 0 to 9 or a letter from A to F.. If we'd like to look for lowercase letters as well, we can add the range a-f: [0-9A-Fa-f].Or add the flag i.. We can also use character classes inside […].. For instance, if we'd like to look for a wordly character \w or a hyphen -, then the set is [\w-]. I have a list of numbers between square brackets, and I need to add words before and after the exact numbers (i.e. keep the same numbers). I use notepad++ to replace, but if you have a solution with other program please advise.
The \s metacharacter is used to find a whitespace character. A whitespace character can be: A space character. A tab character. A carriage return character. A new line character. A vertical tab character. A form feed character. 2.20. Insert the Regex Match into the Replacement Text Problem Perform a search-and-replace that converts URLs into HTML links that point to the URL, and use the URL as the … - Selection from Regular Expressions Cookbook, 2nd Edition [Book] Regular expressions allow you to check a string of characters like an e-mail address or password for patterns, to see so if they match the pattern defined by that regular expression and produce actionable information. Creating a Regular Expression. There are two ways to create a regular expression in Javascript.
The simplest way is to convert ... use the javascript indexOf, includes methods as shown below. charAt (x) Returns the character at the “x” position within the string. The following table illustrates the valid numeric format strings: In this post we will learn how to check string contains special characters using regex (regular ... In JavaScript, a regular expression is simply a type of object that is used to match character combinations in strings. Creating Regex in JS. There are two ways to create a regular expression: Regular Expression Literal — This method uses slashes ( / ) to enclose the Regex pattern: var regexLiteral = /cat/; Find a whitespace character \S: Find a non-whitespace character \b: Find a match at the beginning/end of a word, beginning like this: \bHI, end like this: HI\b \B: Find a match, but not at the beginning/end of a word \0: Find a NULL character \n: Find a new line character \f: Find a form feed character \r: Find a carriage return character \t: Find a tab character \v
Regular Expressions to insert "\r" every n characters in a line and , I don't think that a regex will do this for you. I would google for javascript wordwrap, I'm sure that someone has written a library to do this for you. Stack Overflow for Teams is a private, secure spot for you and your ... Sep 10, 2020 - Get code examples like "javascript add character to string at position" instantly right from your google search results with the Grepper Chrome Extension. The constructor of the regular expression object—for example, new RegExp('ab+c')—results in runtime compilation of the regular expression. Use the constructor function when you know the regular expression pattern will be changing, or you don't know the pattern and obtain it from another source, such as user input.
Relevent modifiers are i (ignore case) and x (extended regex). Regular Expressions (also called RegEx or RegExp) are a powerful way to analyze text. With RegEx, you can match strings at points that match specific characters (for example, JavaScript) or patterns (for example, NumberStringSymbol - 3a&). The .replace method is used on strings in JavaScript to replace parts of Defining Regular Expressions. In JavaScript, regular expressions are represented by RegExp object, which is a native JavaScript object like String, Array, and so on. There are two ways of creating a new RegExp object — one is using the literal syntax, and the other is using the RegExp () constructor.
Add a Dash or Hyphen every 3rd Character using JavaScript I am using an input box where I̢۪ll enter the numbers or a text string. The onkeyup event of the input box will call a function, which will automatically add or insert a dash (-) or a hyphen to the string, using Regex. The Markup and the Script Nov 29, 2019 - Learn how to change a String by inserting a new character at an arbitrary position. Both of those regular expression objects represent the same pattern: an a character followed by a b followed by a c. When using the RegExp constructor, the pattern is written as a normal string, so the usual rules apply for backslashes.
There is no direct method for this, you can write your own Method like InsertAt(char,pos) using Prototype object [References from here] String.prototype.InsertAt=function(CharToInsert,Position){ return this.slice(0,Position) + CharToInsert … I have a string: "The quick brown fox jumps over the lazy dogs." I want to use JavaScript (possibly with jQuery) to insert a character every n characters. For example I want to call: var... Stack Overflow. About ... check every n characters and add delimiter at that location. ... JavaScript regex pattern concatenate with variable. 15. Definition and Usage. The \r metacharacter is used to find a carriage return character. \r returns the position where the carriage return character was found. If no match is found, it returns -1.
why is there a need to capture the entire word to add space? if you take only the 2 characters and add space between them it works the same way ... The /g suffix on the regular expression means "repeat this over the entire string until all matches have been found". We think of the word "global" to mean the same thing. ... Continue browsing in r ... 5/8/2015 · If you want to add a character after the sixth character, simple use the search ^(.{6}) and the replacement. $1, (Example inserts a ,) technically spoken this will replace the first 6 characters of every line with MatchGroup 1 (backreference $1) followed by a comma. I want to insert a slash after every two characters, but just for the first two instances. The following regex inserts after every occurrence. Does anyone know how to limit it to two occurrences? The amount will be entered by the user through an input element. So if a user entered 30032017 it would be something like the following.
A regular expression can be a single character or a more complicated pattern. We can use regex to perform any type of text search and text replace operations. There are two different ways of using a regular expression in JavaScript, they are: Using RegExp object's constructor function. Using Regular Expression Literal But sometimes a regular expression is the only sane way to perform some string manipulation, so it's a very valuable tool in your pocket. This tutorial aims to introduce you to JavaScript Regular Expressions in a simple way, and give you all the information to read and create regular expressions. 1) . — Match Any Character. Let's start simple. The dot symbol . matches any character: b.t. Above RegEx matches "bot", "bat" and any other word of three characters which starts with b and ends in t. But if you want to search for the dot symbol, you need to escape it with \, so this RegEx will only match the exact text "b.t": b\.t. 2) .*.
Regular Expressions to insert "\r" every n characters in a line and , I don't think that a regex will do this for you. I would google for javascript wordwrap, I'm sure that someone has written a library to do this for you. I have a list of numbers between square brackets, and I need to add ... Jul 03, 2019 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. JavaScript Regex Cheatsheet. Regular Expression Basics. Any character except newline ... Regular Expression Special Characters \\n: Newline \\r: Carriage return \\t: Tab \\0: Null character ... \\cY: Control character Y: Regular Expression Posix Classes; Regular Expression Replacement $$ Inserts $ $& Insert entire match $` Insert preceding ...
Capturing groups. A part of a pattern can be enclosed in parentheses (...). This is called a "capturing group". That has two effects: It allows to get a part of the match as a separate item in the result array. If we put a quantifier after the parentheses, it applies to the parentheses as a whole. The full stop, or any special character in a Character Class, is no longer a special character. So in the example, the dot in [.] matches a dot only, whereas, the dot in ".+" matches any character (and is a special character here. To continue with beginning and ending... Jul 09, 2020 - Regular Expression to Insert Character after every X Characters - gist:3941158
5 days ago - Insert text at the beginning of a character vector using the '^' operator, which returns a zero-length match, and the 'emptymatch' keyword. str = 'abc'; expression = '^'; replace = '__'; newStr = regexprep(str,expression,replace,'emptymatch') In JavaScript, the RegExp object is a regular expression object with predefined properties and methods. ... The test() method is a RegExp expression method. It searches a string for a pattern, and returns true or false, depending on the result. The following example searches a string for the character ... A regular expression pattern is composed of simple characters, such as /abc/, or a combination of simple and special characters, such as /ab*c/ or /Chapter (\d+)\.\d*/. The last example includes parentheses, which are used as a memory device. The match made with this part of the pattern is remembered for later use, as described in Using groups.
JavaScript | RegExp \s Metacharacter. Difficulty Level : Medium. Last Updated : 06 Apr, 2021. The RegExp \s Metacharacter in JavaScript is used to find the whitespace characters. The whitespace character can be a space/tab/new line/vertical character. It is same as [ \t\n\r]. Syntax: /\s/. or. In this article we'll cover various methods that work with regexps in-depth. str.match(regexp) The method str.match(regexp) finds matches for regexp in the string str.. It has 3 modes: If the regexp doesn't have flag g, then it returns the first match as an array with capturing groups and properties index (position of the match), input (input string, equals str): Nov 16, 2011 - 10 chars ending in a space), then ... than 10 characters could be found), it matches one word (no matter how long it is) plus a space, then inserts \r after this 3. it matches the end of the string and inserts \r ... I don't think that a regex will do this for you. I would google for javascript wordwrap, ...
As we may recall, regular strings have their own special characters, such as \n, and a backslash is used for escaping. String quotes "consume" backslashes and interpret them on their own, for instance: …And when there's no special meaning: like \d or \z, then the backslash is simply removed. So new RegExp gets a string without backslashes. Insert a character at nth position in string in JavaScript Javascript Web Development Front End Technology Object Oriented Programming We are required to write a JavaScript function that takes in a string as the first argument and a number as the second argument and a single character as the third argument, let's call this argument char.
How Do I Add Brackets To The Beginning Of Every Line Super
Regex Ignore Case Sensitivity Stack Overflow
How To Restrict Special Characters From A Form Field
Full Documentation To The World S Most Comprehensive Regex Editor
Full Documentation To The World S Most Comprehensive Regex Editor
Insert Character Into String Before End Javascript Code Example
Insert Special Character Into A String In Javascript
Full Documentation To The World S Most Comprehensive Regex Editor
Using Regex To Find And Replace Text In Notepad Technical
How To Insert Text Into The Second Line With Regex In Notepad
Insert Special Character Into A String In Javascript
Regex For Horizontal Whitespace S H T Blank Etc
Regular Expression Code Example
Automationanywhere Javascript Regular Expression Stack
How To Use Regex For Google Tag Manager The Ultimate Guide
Using Regex To Find And Replace Text In Notepad Technical
How Javascript Works Regular Expressions Regexp By
Javascript Fundamentals Understanding Regex By Timothy
Javascript Regular Expression How To Create Amp Write Them In
Javascript Replace With Reference To Matched Group Stack
Javascript Regular Expressions And Their Weird Behavior Dev
Introduction To The Use Of 10 Regular Expressions In
T Sql Regex Commands In Sql Server
Master Javascript Form Validation By Building A Form From Scratch
Tips For Using Regex In Data Studio Clickinsight
Regular Expressions In Php Iyappan
Working With Regular Expressions In Postgresql
Add Text At Start And End Of Each Line Notepad Code2care
0 Response to "30 Javascript Regex Insert Character"
Post a Comment