29 Javascript Create Empty Array
May 21, 2021 - It would be silly and slow to create an empty array and grow it using .push(). While it's technically true that setting values outside the array length also cause it to grow, the VMs are able to often optimize the backing store to the array and speed things up when you declare up front how ... Jul 20, 2021 - If your code needs to create arrays with single elements of an arbitrary data type, it is safer to use array literals. Alternatively, create an empty array first before adding the single element to it. In ES2015, you can use the Array.of static method to create arrays with single element.
Check If An Array Is Empty Or Exists Stack Overflow
May 13, 2021 - Asks the user for values using prompt and stores the values in the array. Finishes asking when the user enters a non-numeric value, an empty string, or presses “Cancel”.
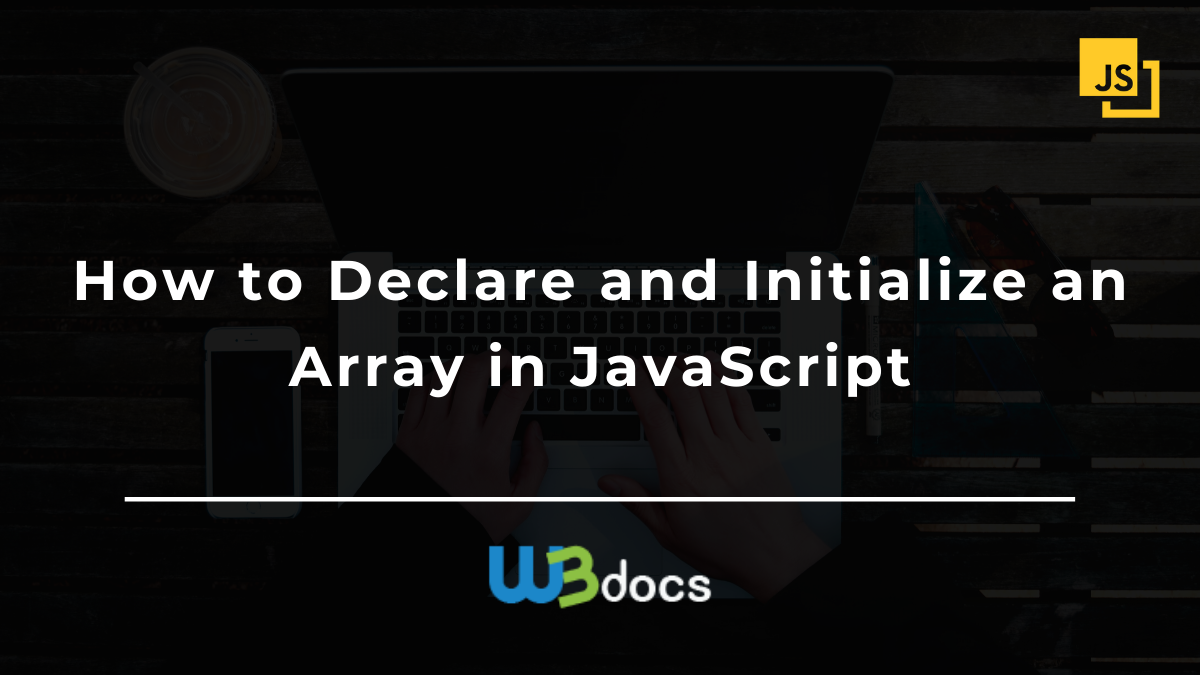
Javascript create empty array. Jul 20, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. There are many ways to empty an array in JavaScript some of them are discussed below: Method 1: Setting to new Array: This method sets the array variable to new array of size zero, then it will work perfectly. The Array () constructor creates Array objects. You can declare an array with the "new" keyword to instantiate the array in memory. Here's how you can declare new Array () constructor: let x = new Array (); - an empty array
The most popular method for creating arrays is using the array literal syntax, which is very straightforward. However, when you want to dynamically create arrays, the array literal syntax may not always be the best method. An alternative method is using the Array constructor. Here is a simple code snippet showing the use of the Array constructor. Jul 20, 2021 - The length property of an object which is an instance of type Array sets or returns the number of elements in that array. The value is an unsigned, 32-bit integer that is always numerically greater than the highest index in the array. javascript create variable containing an object that will contain three properties that store the length of each side of the box · convert the following 2 d array into 1 d array in javascript
Creating an empty array is super easy: · If you want to create an array pre-filled with some content use the ES6 Array.prototype.fill: The two-dimensional array is an array of arrays, that is to say, to create an array of one-dimensional array objects. They are arranged as a matrix in the form of rows and columns. JavaScript suggests some methods of creating two-dimensional arrays. Sep 03, 2020 - No. of ways to empty an array in JavaScript · Python program to reverse an array in groups of given size? Java program to reverse an array in groups of given size · How to create permutation of array with the given number of elements in JavaScript
When you set a value to an element in an array that exceeds the length of the array, JavaScript creates something called "empty slots". These actually have the value of undefined, but you will see something like: [1, 2, 3, 7 x empty, 11] depending on where you run it (it's different for every browser, node, etc.). In Javascript how to empty an array. Javascript Front End Technology Object Oriented Programming. There are multiple ways to clear/empty an array in JavaScript. You need to use them based on the context. Let us look at each of them. Assume we have an array defined as −. let arr = [1, 'test', {}, 123.43]; Jun 10, 2020 - Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript
In the above example first, we create an empty array in javascript or we can also empty an array in javascript. Then using Array.isArray() method first we check that value is an array or not. After it confirms that value is array then we check it's length. If array length is zero then it means the array is empty. Nov 05, 2020 - The ! operator negates an expression. That is, we can use it to return true if an array is empty. For this example, let's open up our JavaScript console. To open up your console in Chrome, you can click Inpsect -> Console. First, create an array with no items in it. Array.length is also a way to empty an array in javascript. I now you are confused that Array.length is used to check the array length but if you assign an Array.length to zero it also creates an empty array in javascript. Now see it in action.
JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array (); Jul 20, 2021 - Note that this special case only ... literals created with the bracket syntax. ... If the only argument passed to the Array constructor is an integer between 0 and 2^32 - 1 (inclusive), this returns a new JavaScript array with its length property set to that number (Note: this implies an array of arrayLength empty slots, not ... Jan 31, 2020 - This tutorial shows you various ways to empty an array in JavaScript.
1 week ago - The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Jul 21, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions. Definition and Usage. The push () method adds new items to the end of an array. push () changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift ().
If start is negative, it is treated as array.length + start.; If end is negative, it is treated as array.length + end.; fill is intentionally generic: it does not require that its this value be an Array object.; fill is a mutator method: it will change the array itself and return it, not a copy of it.; If the first parameter is an object, each slot in the array will reference that object. 31/5/2021 · There are three ways you can create an empty array using JavaScript. This tutorial will help you to learn them all. The first way is that you can get an empty array by assigning the array literal notation to a variable as follows: let firstArr = []; let secondArr = ["Nathan", "Jack"]; Jul 31, 2021 - A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
In Introduction to Objects I, i saw that in the lesson no. 26.Arrays of Objects the empty array was declared as: And you should take note that it ... as javascript manages strings differently than other primitive types, not to mention arrays of objects. The type may affect what happens. ... Then you are assigning new array reference to a, if reference in a is already assigned to any other variable, then it will not empty that array ... The OP asked in javascript how would I create an empty array of a given size. This solves that problem. - mariocatch Jan 22 '16 at 1:21. 16. The OP has provided an example of "empty". - RobG Jan 22 '16 at 1:22. 9. Well this is another option to the question.
This returns an Array Iterator containing the keys of all the indexes in the array. Even though the array is empty, the empty slots still have an internal key (0, 1, 2… etc.). This key is used when the array needs to be iterated over, for instance using for…of. Array (1000). keys So now we have an array iterator containing the keys 0 to 999. list = [] assigns a reference to a new array to a variable, while any other references are unaffected. which means that references to the contents of the previous array are still kept in memory, leading to memory leaks. list.length = 0 deletes everything in the array, which does hit other references.. In other words, if you have two references to the same array (a = [1,2,3]; a2 = a;), and you ... Jul 20, 2021 - The Array.of() method creates a new Array instance from a variable number of arguments, regardless of number or type of the arguments.
30/10/2008 · Similarly it is also possible to create an empty array using different syntax: var arrayA = [] var arrayB = new Array() javascript arrays object javascript-objects new-operator This is how we can create empty array in python. Create empty 2D arrays in Python. In python, we can create 2D arrays in python by using a list. The 2D array is an array within an array. In 2D array, the position of the element is referred by two and it is represented by rows and columns. In this tutorial, we will learn how to create arrays; how they are indexed; how to add, modify, remove, or access items in an array; and how to loop through arrays. Creating an Array. There are two ways to create an array in JavaScript: The array literal, which uses square brackets. The array constructor, which uses the new keyword.
If you know the expected length of the array you’re creating, you can also create an empty array of that length where all the elements are undefined, like this: var arrayName = new Array (expectedLength); Here, if you check the length of the array, the output will be ‘expectedLength’ while in the first implementation it would be 0. var arr = [,,]; It initializes array with the number of elements equal to the number of comma used inside square brackets []. So in above case, length of the array will be 2 instead of 3. var arr1 = [,,]; var arr2 = [,,,]; console.log (arr1.length); console.log (arr2.length); And in the above example, as we made use of length property to ... If you know the expected length of the array you're creating, you can also create an empty array of that length where all the elements are undefined, like this: var arrayName = new Array (expectedLength); Here, if you check the length of the array, the output will be 'expectedLength' while in the first implementation it would be 0.
validation if array empty javascript; javascirpt check if array is emtpy before includes or not; how to check if result body array is empty javascript; if not amprty array; javascript array is null or empty; array.isarray. jsonarray.length. empty check nodejs; js empty array null; how to cehck is somthing is a empty array; check if an object is ... Jul 20, 2021 - The JavaScript exception "reduce of empty array with no initial value" occurs when a reduce function is used. In this case, we are creating an empty object with a length property set to 10 Using the "spread" syntax The spread syntax is simply ".." i.e. two dots. What it does is, as the name suggests, expands the entered iterables. Please refer to this article to understand how spread syntax works. Let us now use it to fill an array.
In Example # 2, we create an array literal, but it is empty. (var arr = []; works just fine, but it is an empty array.) When we check the length property and try to inspect the object with console.dir (arr), we can clearly see that it is empty. Then we add elements to the array, and add named properties (e.g. arr ["drink"] = "beer"). Given a JavaScript array, see how to clear it and empty all its elements. 🏠 Go back to the homepage How to empty a JavaScript array Given a JavaScript array, see how to clear it and empty all its elements. Published Dec 03, 2018. There are various ways to empty a JavaScript array. The easiest one is to set its length to 0: const list = ['a ... JavaScript has different ways to declare an empty array. One way is to declare the array with the square brackets, like below. var array1 = []; The other way is to use the constructor method by leaving the parameter empty.
How Much Memory Do Javascript Arrays Take Up In Chrome
Hacks For Creating Javascript Arrays
Module 3 Program Flow And Data Collections D
Powershell Array Guide How To Use And Create
Javascript Array Methods How To Use Map And Reduce
React Reactjs Update An Array State
List Control Methods Part 2 Insert Sample Data At Current
Java67 How To Remove A Number From An Integer Array In Java
Javascript Clear Array How To Empty Array In Javascript
Javascript Array Splice Delete Insert And Replace
How To Empty An Array In Javascript
Dynamic Array In Javascript Using An Array Literal And
How To Remove Commas From Array In Javascript
Check For Empty Array In Javascript
4 Ways To Convert String To Character Array In Javascript
Insert Values To Empty Array Javascript Code Example
Better Array Check With Array Isarray By Samantha Ming
Need Help Creating A Proper Structure For The Chegg Com
Javarevisited Jsp How To Check If Arraylist Is Empty Using
Using A For Each Loop On An Empty Array In Javascript Stack
How To Deep Clone An Array In Javascript Dev Community
How To Declare And Initialize An Array In Javascript
Note It Is Required To Use The Es6 Style Of Keywords Chegg Com
Reactjs Check Empty Array Or Object Example
Let S Get Those Javascript Arrays To Work Fast Gamealchemist
Javascript Getting Good With Loop And Array
How To Check If A Javascript Array Is Empty Or Not With Length
A 6 Minute Guide To 24 Javascript Array Methods By Mohit
0 Response to "29 Javascript Create Empty Array"
Post a Comment