21 How To Create Javascript Module
MANY WAYS TO CREATE REUSABLE COMPONENTS. There are probably endless ways to create reusable components in Javascript. But let me do a quick compilation and generalization: The "traditional way" - Grouping functions and objects together. Javascript Modules - Importing and exporting, or require in the "NodeJS way". Since our index.js file contains references to the Node built-in process and Buffer modules, both Browserify and Webpack will automatically include the polyfills for those entire modules in the bundle. From this simple 9-line module, I calculated that Browserify and Webpack will create a bundle weighing 24.7KB minified (7.6KB min+gz).
Javascript Module In 5 Minutes
A JavaScript module is created by a variable. Inside that we put a "Self-invoking-function-expression". This function is called automatically. Enough talking, just dive into the code! JavaScript Module Let's create a module called MrFrontendModule.
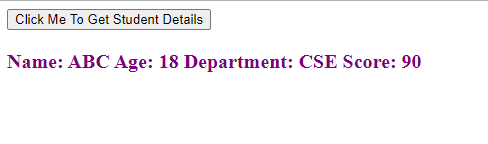
How to create javascript module. Creating an ES6 module may be accomplished by adding an export statement to any JavaScript script file: This will make the objects being exported available for all scripts to import. Public variables, functions and classes are exposed using an export statement. By default, all declared objects within an ES6 module are private. Modules (sometimes referred to as ECMAScript modules or ES Modules) are now available natively in JavaScript, and in the rest of this tutorial you will explore how to use and implement them in your code. Native JavaScript Modules. Modules in JavaScript use the import and export keywords: import: Used to read code exported from another module. To create a package.json file, on the command line, in the root directory of your Node.js module, run npm init: For scoped modules, run npm init --scope=@scope-name; For unscoped modules, run npm init; Provide responses for the required fields (name and version), as well as the main field: name: The name of your module. version: The initial module version.
Use type="module" statement in the script tag and then import the function using import statement as shown above. 2. Assign the function to window object. Any method in a javascript module is available only within the scope of the module. So to increase its scope outside of the module you can assign it to window object. Snippet 1.68: Importing a module. To use an import in the browser, we must use the script tag. The module import can be done inline or via a source file. To import a module, we need to create a script tag and set the type property to module. If we are importing via a source file, we must set the src property to the file path. This is shown in ... 22/1/2016 · With CommonJS, each JavaScript file stores modules in its own unique module context (just like wrapping it in a closure). In this scope, we use the module.exports object to expose modules, and require to import them. When you’re defining a CommonJS module, it might look something like this:
Step 1 — Creating a Module. This step will guide you through creating your first Node.js module. Your module will contain a collection of colors in an array and provide a function to get one at random. You will use the Node.js built-in exports property to make the function and array available to external programs. The common pattern for nodejs modules is to create a file (e.g. mymodule.js) so: var myFunc = function() { ... }; exports.myFunc = myFunc; If you store this in the directory node_modules it can be imported thus: var mymodule = require('mymodule'); mymodule.myFunc(args...); So, in your case, your module server.js could look like this: The second method of creating a JavaScript object is using a constructor function. As opposed to object literals, here, you define an object type without any specific values. Then, you create new object instances and populate each of them with different values. ... Before the flexbox layout module was introduced, it had been a challenge to ...
Real example how to create a universal open-source javascript module that will work in both browser and NodeJS environments and publish to the NPM registry There are a lot of inconsistency in the… Editing Monitors :https://amzn.to/2RfKWgLhttps://amzn.to/2Q665JWhttps://amzn.to/2OUP21a.Check out our website: http://www.telusko Follow Telusko on Twitte... This post presents 4 best practices on how to organize better your JavaScript modules. 1. Prefer named exports. When I started using JavaScript modules, I had used the default syntax to export the single piece that my module defines, either a class or a function. For example, here's a module greeter that exports the class Greeter as a default ...
Create a new module. Create a requirejs-config.js and a JavaScript module file. Create a layout update to add a template that will enable the JavaScript module. Create a template file. JavaScript modules are an implementation of closures, thus, I strongly suggest that you go through DataFlair's JavaScript tutorial on Closures before you continue further with this topic. Moving on, JavaScript modules (also called "ES modules" or "ECMAScript modules") contain a class or a library of functions. For the list of Node.js modules, you can go here. There are 3 module type in Node.js: - Built-in modules (provided by Node.js installation) - User-defined modules (created by the user) - Third party modules (generally available on the Internet) Here are the steps for creating a User-defined module: 1) Create a .js file which contains the Custom ...
19/10/2011 · Module : {}; Module.Utils = (function() { var settings = { x : 1, y : 2 }; function C(){ console.log("Module.Utils: C"); }; function D(){ console.log("Module.Utils: D"); }; return { C:C, D:D } }()); javascript design-patterns module Module is self-contained and reusable chunk of JavaScript code written in a modular way. Until ES6 JavaScript did not define any language construct for working with modules. So writing modular JavaScript involves the use of some coding convention. With ES6 JavaScript changed from a programming language that many people dreaded using to one of the most popular and loved languages. Of the new changes in ...
You can also embed the module's script directly into the HTML file by placing the JavaScript code within the body of the <script> element: < script type ="module"> /* JavaScript module code here */ </ script > The script into which you import the module features basically acts as the top-level module. Notice that we use ./ to locate the module, that means that the module is located in the same folder as the Node.js file. Save the code above in a file called "demo_module.js", and initiate the file: Initiate demo_module.js: C:\Users\ Your Name >node demo_module.js. If you have followed the same steps on your computer, you will see the same ... In the browser, we can make a variable window-level global by explicitly assigning it to a window property, e.g. window.user = "John". Then all scripts will see it, both with type="module" and without it. That said, making such global variables is frowned upon. Please try to avoid them.
Tree shaking is a term commonly used in the context of JavaScript for dead-code elimination. It relies on the static structure of ES2015 module syntax, that is, import and export. The name and concept have been popularized by the ES2015 module bundler rollup. Webpack and Rollup both support Tree Shaking, meaning we need to keep certain things ... The export statement is used when creating JavaScript modules to export live bindings to functions, objects, or primitive values from the module so they can be used by other programs with the import statement. The value of an imported binding is subject to change in the module that exports it. When a module updates the value of a binding that it exports, the update will be visible in its ... Exporting a library: There is a special object in JavaScript called module.exports. When some program include or import this module (program), this object will be exposed. Therefore, all those functions that need to be exposed or need to be available so that it can used in some other file, defined in module.exports.
javascript programming. The Module Pattern is one of the most common design patterns used in JavaScript and for good reason. The module pattern is easy to use and creates encapsulation of our code. Modules are commonly used as singleton style objects where only one instance exists. The Module Pattern is great for services and testing/TDD. 3. Write your module. There should now be a package.json file inside your project directory. We need to write our code to upload it as a module. Note: In this example, we are simply writing a function to print some text to the console. Create a file and name it index.js in the project directory. Copy and paste the following code to index.js: Creating modules using IIFE is the oldest way of creating JavaScript modules. Here is example code on how to create modules using IIFE. You can place the below code in an separate file to make it an distributable module or place it directly in your main program for your own use. //Module Starts. (function( window){.
I'm trying to take an existing object in Javascript and rewrite it as a module. Below is the code I'm trying to rewrite as a module: var Queue = {}; Queue.prototype = { add: function(x) { ... A common question we get is around techniques for code re-use between projects that use different types of JavaScript evaluation mechanisms, like the CommonJS module spec, Ti.include, or just in the browser. I thought I'd share a quick technique for creating a JavaScript module that will work in all three of these environments.
How To Access Variables From Another File Using Javascript
Learn The Basics Of The Javascript Module System And Build
4 Best Practices To Write Quality Javascript Modules
Node Js Creating And Using Modules Parallelcodes
Create And Publish A Universal Javascript Module By
How To Add A Javascript Module Adobe Commerce Developer Guide
Writing And Publishing A Simple Javascript Module To Npm
Titanium Module Concepts Documentation Amp Guides 2 0
Javascript Modules A Shot To Figure Out How The Most By
Javascript Modules Explained With Examples
How To Resolve Cannot Use Import Statement Outside A Module
Build Javascript Actions For Native Mobile Studio Pro 9 How
How To Create And Delete Folders And Files In Javascript Nodejs
Server Side Javascript Create Module 1
Using Method Chaining With The Revealing Module Pattern In
Javascript Modules A Shot To Figure Out How The Most By
Learn The Basics Of The Javascript Module System And Build
Chapter 5 Creating A Webassembly Module That Calls Into
How To Create Your Own Node Js Module
0 Response to "21 How To Create Javascript Module"
Post a Comment