32 How To Change String To Int In Javascript
string The value to parse. If this argument is not a string, then it is converted to one using the ToString abstract operation. Leading whitespace in this argument is ignored. radix Optional. An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) of the string.Be careful—this does not default to 10!If the radix value is not of the Number type it will ... 14/7/2009 · There are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. All of the tricks in the other answers (e.g. unary plus) involve implicitly coercing the type of the string to a number. You can also do the same thing explicitly with the Number function. Parsing
How To Convert String To Number In Javascript
Need of Convert string to int javascript. At starting we see 2 types of data declaration in Javascript. Now see what are the problem and where we needed to convert a string to int:-If you have to the comparison of the two variables, it would fail because they're two different types of objects. Let's see the problem in the example code.
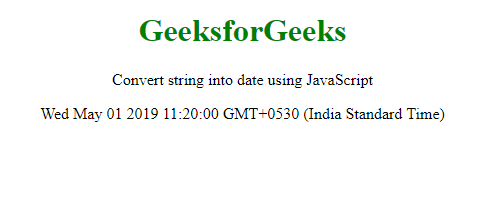
How to change string to int in javascript. Nov 03, 2019 - Managing data is one of the fundamental concepts of programming. Converting a Number to a String is a common and simple operation. The same goes for the other... Nov 03, 2019 - Managing data is one of the fundamental concepts of programming. Because of this, JavaScript offers plenty of tools to parse various data types, allowing you t... The toString() method is a built-in method of the JavaScript Number object that allows you to convert any number type value into its string type representation.. How to Use the toString Method in JavaScript. To use the toString() method, you simply need to call the method on a number value. The following example shows how to convert the number value 24 into its string representation.
The parseInt () function parses a string and returns an integer. The radix parameter is used to specify which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that the number in the string should be parsed from a hexadecimal number to a decimal number. In an earlier article, we looked at how to convert an array to string in vanilla JavaScript. Today, let us look at how to do the opposite: convert a string back to an array.. String.split() Method The String.split() method converts a string into an array of substrings by using a separator and returns a new array. It splits the string every time it matches against the separator you pass in as ... Convert string to integer in javascript javascript 1min read In this tutorial, we are going to learn how to convert a numeric string to integer/number in javascript.
You can convert string to number in a number of ways. But there are two main ways to convert a string to a number in javascript. One way is to parse it and the other way is to change its type to a Number. ... The parseInt() function parses a string and returns an integer. 6 days ago - The value to parse. If this argument is not a string, then it is converted to one using the ToString abstract operation. Leading whitespace in this argument is ignored. ... An integer between 2 and 36 that represents the radix (the base in mathematical numeral systems) of the string. Learn how to convert a string to a number using JavaScript. This takes care of the decimals as well. Number is a wrapper object that can perform many operations. If we use the constructor (new Number("1234")) it returns us a Number object instead of a number value, so pay attention.Watch out for separators between digits:
Jul 17, 2014 - Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Sep 08, 2017 - JavaScript convert string to number method: ways to easily apply JavaScript string to number in your code, and master javascript convert string to number. By concatenating with empty string "" This the most simplest method which can be used to convert a int to string in javascript. As javascript is loosely typed language when we concatenate a number with string it converts the number to string. 15 + '' = "15"; 15.55 + '' = "15.55"; 15e10 + '' = "150000000000"
Convert a String to Number Using the + Unary Operator. You can also make use of the unary operator or + before a string to convert it to a number but the string should only contain number characters. For example: let result = +"2020"; typeof result "number". As you see when using the unary plus operator with a JS string, the result is a number. Return Value: The num.toString () method returns a string representing the specified number object. Below are some examples to illustrate the working of toString () method in JavaScript: Converting a number to a string with base 2: To convert a number to a string with base 2, we will have to call the toString () method by passing 2 as a parameter. The difference between the String() method and the String method is that the latter does not do any base conversions. How to Convert a Number to a String in JavaScript¶ The template strings can put a number inside a String which is a valid way of parsing an Integer or Float data type:
How To Add Value To Parse String To Number Using Javascript - parseInt()Source Code: http://1bestcsharp.blogspot /2017/01/javascript-convert-string-to-int... 2 weeks ago - The Number constructor contains constants and methods for working with numbers. Values of other types can be converted to numbers using the Number() function. Convert String Into Integer Using parseInt () parseInt is most popular method to convert string into integer.The parseInt () takes two parameters, The first parameter is the string value and second one the radix. The radix parameter is used to specify which numeral system to be used. var x = parseInt ("1000", 10);
Jul 24, 2020 - The parseInt() function takes a ... into an integer. The radix parameter is used to define which numeral system to be used, for example, a radix of 16 (hexadecimal) indicates that a number in the string should be parsed from the hexadecimal number to the decimal number. If the radix parameter is not passed, then JavaScript assumes the ... To convert String into Integer, we can use Integer.valueOf () method which returns instance of Integer class. We can use tyhe Number function to convert an integer string into an integer. For instance, we can write: const num = Number ("1000") console.log (num) Then num is 1000.
10/4/2019 · Last Updated : 26 Jul, 2021 In JavaScript parseInt () function is used to convert the string to an integer. This function returns an integer of base which is specified in second argument of parseInt () function. parseInt () function returns Nan (not a number) when the string doesn’t contain number. Dec 02, 2015 - Browse other questions tagged javascript string performance coding-style numbers or ask your own question. ... The full data set for the 2021 Developer Survey now available! Podcast 371: Exploring the magic of instant python refactoring with Sourcery ... How to deal with a boss who keeps changing ... 23/8/2019 · Front End Technology Javascript Object Oriented Programming To convert a string to an integer parseInt () function is used in javascript. parseInt () function returns Nan (not a number) when the string doesn’t contain number. If a string with a number is sent then only that number will be returned as the output.
2. How to Convert a String into an Integer¶. Use parseInt() function, which parses a string and returns an integer.. The first argument of parseInt must be a string.parseInt will return an integer found in the beginning of the string. Remember, that only the first number in the string will be returned. Nov 09, 2019 - Just like the previous two examples, the parseFloat() function takes a string of "25" and converts it into a number value of 25. And here are some additional examples using the same string variations as the other methods: Convert the string of any base to integer in JavaScript. The parseInt function available in JavaScript has the following signature −. Where, the paramters are the following −. String − The value to parse. If this argument is not a string, then it is converted to one using the ToString method. Leading whitespace in this argument is ignored.
Nov 06, 2017 - Today, let’s look at three different ways to convert a string into a number. ... The parseInt() method converts a string into an integer (a whole number). Convert Hexadecimal Number in a String to Number. A hex number is a base 16 number, to convert it into an integer with the data type of number in JavaScript supply the radix as the second argument like this: var output = parseInt("0xF", 16); console.log(output); console.log(typeof(output)); 15 number. Jul 20, 2021 - The toString() method returns a string representing the specified Number object.
While answers such as Kyra Frost show multiple methods for converting a number into a string in JavaScript without explicitly calling toString(), it's important to understand that all these methods invoke toString() implicitly. While you can't ove... convert array of string to array of int javascript; convert string array to integer array in javascript; how to make a string a number in an array; how to convert array of string into array of int in javascript; convert char into ascii binary value c#; convert char into ascii value c#; how to add a int to a string in c++; convert string to ... string Use the String() Function to Convert an Integer to a String in JavaScript. We have used String() to convert the value of a variable to a string. This method is also called typecasting. Typecasting means changing the data type of one value to another data type. Check the code below. var a = 45; var b = String(a); console.log(typeof(b ...
This method is used to parse a string and convert it into an integer. You can use this method according to the following syntax: parseInt (string, radix); In the above syntax: string refers to the value to be converted and is a required parameter. radix refers to the number system to which the value is to be converted i.e. decimal, octal, etc. How to convert string to number JavaScript IvanLomakin - Aug 4: How to convert int to string in C++? Lalit Kumar - Jul 13, 2020: Convert string array to int array java Hasnain_khan - Oct 10, 2020: Converting String to different DataTypes in JAVA Takele - Aug 13: How to convert int to boolean in java kesav.eee - Jun 14 Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Convert Float to Int Using the parseInt () Function in JavaScript parseInt () is widely used in JavaScript. With this function, we can convert the values of different data types to the integer type. In this section, we will see the conversion of a float number to an integer number.
Dart Flutter Convert String To Double Int And Vice Versa
Sql Cast And Sql Convert Function Overview
How To Convert Integer Variable To String In React Native
C Convert Int To String Javatpoint
Kotlin Android Convert String To Int Long Float Double
Java Convert String To Double Javatpoint
How To Convert String To Float Number In Javascript
Java Convert Int To String Javatpoint
Java Convert String To Int Examples
Solved Convert Text To Number Power Platform Community
Java String String Functions In Java With Examples Edureka
Javascript Convert String To Number
String Concatenation And Format String Vulnerabilities
Es6 Convert String To Number Code Example
How To Convert A String To A Number In Javascript
Excel Formula Convert Text To Numbers Exceljet
Java Convert String To Int Javatpoint
How To Convert A String To An Integer In Javascript Stack
What S The Best Way To Convert A Number To A String In
Hex To String Converter Convert Your Hexadecimal To Text
Convert String To Different Case Styles Snake Kebab Camel
Convert String Into Date Using Javascript Geeksforgeeks
Converting Python 3 Data Types Numbers Strings Lists
2 Ways To Convert Values To Boolean In Javascript
How To Convert Strings To Numbers Using Javascript
String To Number General Node Red Forum
In Java How To Convert String To Char Array Two Ways
Convert String To Int And Back In Swift Learnappmaking
How To Easily Convert String To Integer In Java
Crypt Array By Converting The String Into Int And Int Into String
Beginner Goodies Convert A Javascript Object To Json String
0 Response to "32 How To Change String To Int In Javascript"
Post a Comment