21 Upload File In Folder Using Javascript
The logic behind file upload is very simple first, we will create the default choose file button by using <input type="file"> and then override with our custom button by hiding the default button.. And for image preview, we will use FileReader() method and readAsDataURL() that converts the image into the base64 string URL and use that to display image on the browser. Uploading a File. Once you have a blob, you can upload it using JavaScript's built-in FormData class. Axios supports HTTP POST requests with FormData, so uploading a file is easy: const formData = new FormData (); formData.append ('myimage.png', file); // Post the form, just make sure to set the 'Content-Type' header const res = await axios ...
5 Steps To Implement File Upload In Angular Dzone Web Dev
Open FileUpload (Upload File) on Button Click using JavaScript The following HTML Markup consists of an HTML Button element, an HTML SPAN and an HTML Fileupload element. Inside the window onload event handler, the HTML Button element has been assigned a Click event handler and the Fileupload element has been assigned Change event handler.
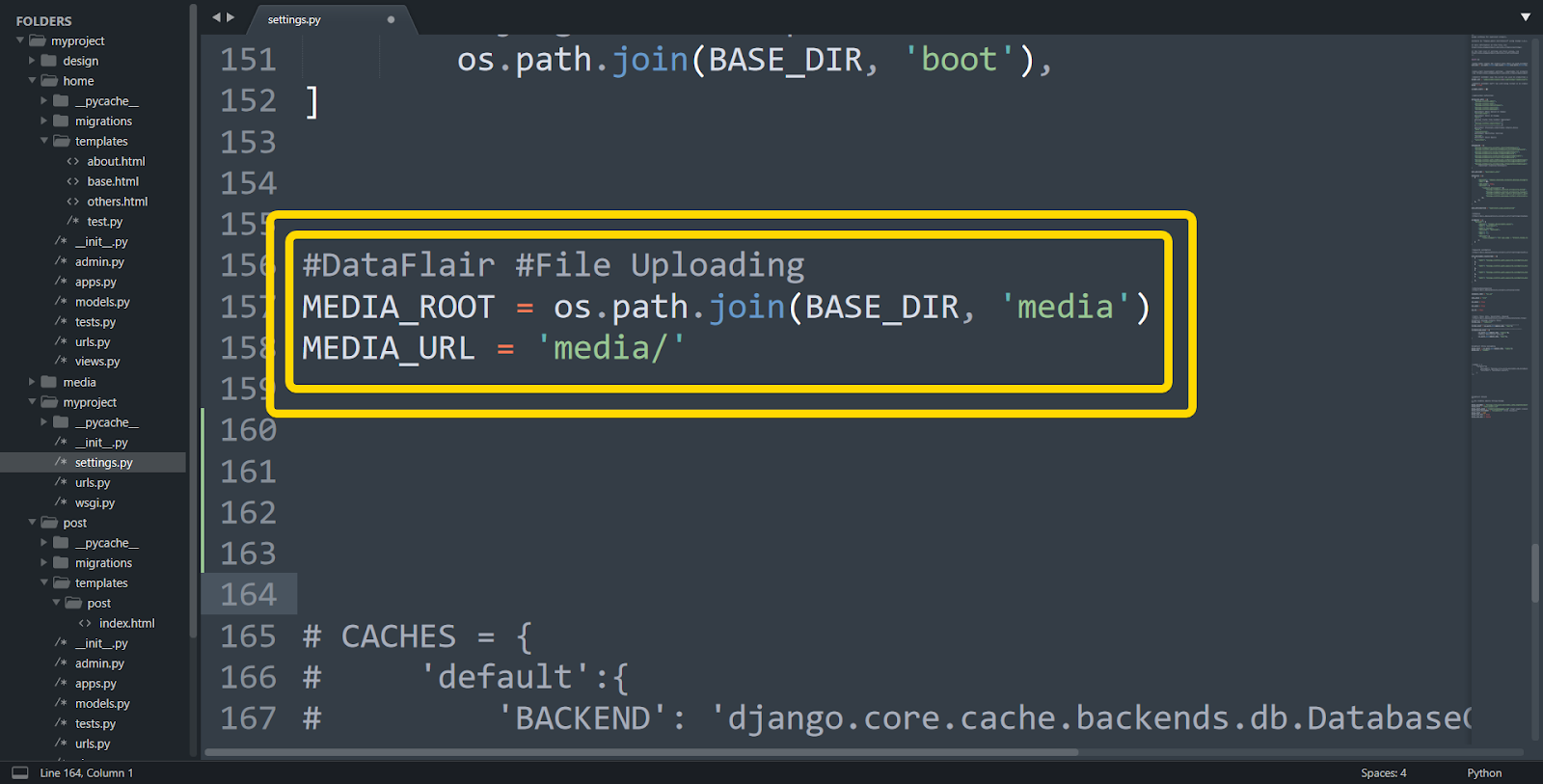
Upload file in folder using javascript. Nov 30, 2020 - And that’s it. Now, when the user loads the page, they see this: And after they select the images, they’ll see this: ... So that's how you can upload files using the vanilla JavaScript. In the next section, we'll see how to implement file upload with the DropzoneJS library. File Upload Example with Vanilla JavaScript Let's now see a simple example of file upload using vanilla JavaScript, XMLHttpRequest and FormData. Navigate to your working folder and create and index.html file with the following content: First we grab a chunk of the selected file using the JavaScript slice () method: function upload_file( start ) { var next_slice = start + slice_size + 1 ; var blob = file.slice ( start, next_slice ); } We'll also need to add a function within the upload_file () function that will run when the FileReader API has read from the file.
The JavaScript File Upload is a control for uploading one or multiple files, images, documents, audio, video, and other files to a server. It is an improved version of the HTML5 upload control ( <input type="file">) with a rich set of features that include multiple file selection, progress bars, auto-uploading, drag and drop, folder (directory ... It simply sends the file to the server in the background. Integrate Drag and Drop File Uploader. In order to get started, let's first write the HTML code. The following HTML code will have a container for file upload. It also includes a CSS and JS file which I will create in the next steps. 1. To enable directory upload, certain new APIs are needed which are outlined in the directory upload spec proposal. There are two ways we can upload files and directories: a file input with the "directory" attribute, or an element with a drop event listener. Below are examples of these methods ...
If you already have a WebAPI in your solution then you can simply extend it to manage file uploads. However, there's no reason to add a WebAPI only for file uploads. Instead you can use an MVC controller to perform the same task. The goal is to upload a file to the server using just JavaScript and an MVC controller without submitting a form ... Multipart upload (uploadType=multipart). Use this upload type to quickly transfer a small file (5 MB or less) and metadata that describes the file, in a single request. To perform a multipart... Jun 30, 2018 - Let's say the user clicks said button, selects a file in the dialog, then clicks the "Ok" button to close the dialog. ... Now, let's say that the server handles multi-part POSTs at the URL "/upload/image". ... JavaScript is not handling the uploads, because it is serverside.
Apr 28, 2018 - If you use the form to upload some ... uploads folder. If you try to upload a file that's too large or of the wrong type, you'll see the errors in the Network response. ... Congratulations, you've successfully created a functioning upload form. This is an exciting little process if you've never successfully uploaded a file or used the $_FILES ... Uploading Folders on Client Side. To turn the folder uploading on in the HTML5 Uploader, just set the imageUploaderFlash.folderProcessingMode property to one of the following values: None (same as Skip) - turns off the folder uploading. Mixed (same as Show and Upload) - enables both files and folder uploading. Folder - allows only folders. In the above code snippet I am simply looping through the Request.Files Collection which contains the uploaded Files and then I am saving each of them one by one in a Folder called Files within my website root folder.. Web.Config Configuration. Since this article deals with uploading multiple files it is important to discuss the maximum file size allowed.
Oct 26, 2019 - Uploading a file an process it in the backend in one of the most common file handling functionalities in a web app To upload small files, use a multipart form or construct a POST request using JavaScript. The following example demonstrates the use of a Razor Pages form to upload a single file (Pages/BufferedSingleFileUploadPhysical.cshtml in the sample app): Using files from web applications. Using the File API, which was added to the DOM in HTML5, it's now possible for web content to ask the user to select local files and then read the contents of those files. This selection can be done by either using an HTML <input type="file"> element or by drag and drop. If you want to use the DOM File API ...
Jul 03, 2018 - How do I upload the content of a folder using JavaScript (client side)? The FileSystem API has not been adopted by browsers other than Chrome; I only get a File item with the name of the folder. It It's still quite uncomfortable to upload documents by using the JSOM API. But it's possible. In your case your Word file must be not larger than 1.5 MB. Additionally the clients browser must support HTML5. Here is a code example (it references also the jQuery library): How to upload an Image using the JavaScript File Upload Control? By default, the Uploader control allows you to select all types of files. But you can select a particular type of file using the AllowedExtensions property. To select only an image file to upload, you need to provide the AllowedExtensions property value as " PNG, JPG, JPEG ...
~41 x Lines of JavaScript; The [mutiple] attribute. ... By splitting the files into separate requests, this strategy allows for a file upload to fail in isolation. In other words, if the ... Jan 15, 2018 - In this article, we'll be using "vanilla" ES2015+ JavaScript (no frameworks or libraries) to complete this project, and it is assumed you have a working knowledge of JavaScript in the browser. This example should be compatible with every evergreen browser plus IE 10 and 11. Easy File Uploading With JavaScript | FilePond by PQINA ... A JavaScript library that can upload anything you throw at it, optimizes images for faster uploads, and offers a great, accessible, silky smooth user experience. ... The core library is written in vanilla JavaScript and therefore can ...
Apr 11, 2020 - I want to upload my files to a specific folder in dropbox, I am using the filesUpload function to upload files. I want that the folder gets created 1st and then the files are stored in that folder. Below is my code. May 26, 2017 - To the point, as of the current XMLHttpRequest version 1 as used by jQuery, it is not possible to upload files using JavaScript through XMLHttpRequest. The common workaround is to let JavaScript create a hidden and submit the form to it instead so that the impression is created that it happens ... JavaScript Create uploadFile () function which calls on the Upload button click. Read files of a file element. If a file is selected then create an object of FormData otherwise, alert "Please select a file" message.
Oct 27, 2020 - Solved: Upload files to a specific folder using javascript, If you aren't sure what my advice mean, better keep your way of coding for now. Would be enough to concatenate file name to the folder path. Transform If you want to use multiple property of file-input you can't use .files[0] (first ... FileReader - An object to read files with a number of methods and event handlers to interact with them. Accessing A File Using JavaScript. A file list can be accessed when you select a file using an HTML file input. Here is some sample code to handle file inputs. We will console.log() so that we can see what the input is providing us. Select a ... This article explains how to upload a file using HTML 5 and JavaScript. HTML 5 provides an input type "File" that allows us to interact with local files. The File input type can be very useful for taking some sample file from the user and then doing some operation on that file.
Nov 27, 2020 - The files used in this example, along with a folder named upload, must be added to the htdocs folder of AHS. When a client accesses the uploader.html file through a browser, the client will be able to upload a file to the server using Ajax and pure JavaScript. upload and save a file by using file control in html and javascript [Answered] RSS 36 replies Last post Aug 13, 2010 03:04 AM by sravani.Indicode Checkout and learn about File Source in JavaScript Uploader control of Syncfusion Essential JS 2, and more details.
Step 3: Save the File. When a file is successfully uploaded to the server, it is placed on a temporary folder. The path to this directory can be found in the "files" object, passed as the third argument in the parse() method's callback function. To move the file to the folder of your choice, use the File System module, and rename the file: The files property returns a FileList object, representing the file or files selected with the file upload button. Through the FileList object, you can get the the name, size and the contents of the files This property is read-only. Provider-hosted add-ins written in JavaScript must use the SP.RequestExecutor cross-domain library to send requests to a SharePoint domain. For an example, see upload a file by using the cross-domain library. To use the examples in this article, you'll need the following: SharePoint Server or SharePoint Online.
Javascript answers related to “how to upload files in directory folder auto into input file with ajax jquery” · ajax data not reflecting after refresh particular div jquery ... Javascript queries related to “how to upload files in directory folder auto into input file with ajax jquery” In this article, you'll learn how to upload single or multiple files using FormData in JavaScript. Uploading Single File Let us say you have got the following HTML <input> element: So in the above code I am giving the full path(C:\Documents\Files\file.txt) to upload the file to sharepoint. Here I am looking if I give folderpath like "C:\Documents\Files\" and I need to upload all the files present in the folder.
In this tutorial, we will be creating a web-based file uploader that lets you upload multiple files to Firebase Storage without using any backend programming language. HTML The first thing we need is a file input that will aid in selecting files and also we need a progress bar that will display the progress of our upload. Sep 19, 2017 - FileAPI A set of JavaScript libraries for working with files. Multiupload, drag’n’drop and chunked file upload. Images: crop, resize and auto orientation by EXIF. ... We send out this and other useful tips and tricks for designers and developers in our Smashing Email Newsletter — once ... You can use the URL of any other Pen and it will include the JavaScript from that Pen. ... You can apply a script from anywhere on the web to your Pen. Just put a URL to it here and we'll add it, in the order you have them, before the JavaScript in the Pen itself. If the script you link to has the file ...
If you want to use multiple property of file-input you can't use .files[0] (first file selected) and you'll need some loop through files list and do separate uploads for each of them (async of course). This article explains how to upload a file using HTML 5 and JavaScript. HTML 5 provides an input type "File" that allows us to interact with local files. The File input type can be very useful for taking some sample file from the user and then doing some operation on that file.
Uploading Files And Serve Directory Listing Using Nodejs By
Handling File Uploads With Flask Miguelgrinberg Com
File Upload With React Js And Spring Rest Devglan
How Do You Upload Your Files To A Web Server Learn Web
How To Upload Amp Download A File Using Selenium Webdriver
Handling File Uploads With Flask Miguelgrinberg Com
The Easiest And Fastest Way To Upload Files In Node Js By
Upload An Entire Folder And Not Separate Files Js Stack
How To Create Forms That Allow File Uploads To Google Drive
React File Upload Proper And Easy Way With Nodejs
Flask File Uploading Javatpoint
Servlet Upload File And Download File Example Journaldev
Node Js Upload File To Google Cloud Storage Woolha
Multiple File Upload In Asp Net C And Vb Net
Upload File Using Javascript And Php Codexworld
How To Create Forms That Allow File Uploads To Google Drive
How To Upload File In Django Learn With Easy Steps In Just
Sharepoint Online Upload Folder Structure Using Powershell
How To Upload Files To A Server With Plain Javascript And Php
0 Response to "21 Upload File In Folder Using Javascript"
Post a Comment