22 Push Notifications Javascript Example
To make push notifications work, we are going to create: A JavaScript file that asks the user permission. A service worker that manages the notifications. Let's create a file called push-notifications.js that: understand if the push notifications are supported by the browser. Asks user consent. Progressive Web Apps (PWAs) enables a website to make make a lot of interactions that are app-like. Among these are Push Notifications. This is a functionality that enables you to make native notifications for many different devices and to invoke these notifications even when the browser is not active.
Integrating Push Notifications In Your Node React Web App
1 week ago - The Push API gives web applications the ability to receive messages pushed to them from a server, whether or not the web app is in the foreground, or even currently loaded, on a user agent. This lets developers deliver asynchronous notifications and updates to users that opt in, resulting in ...
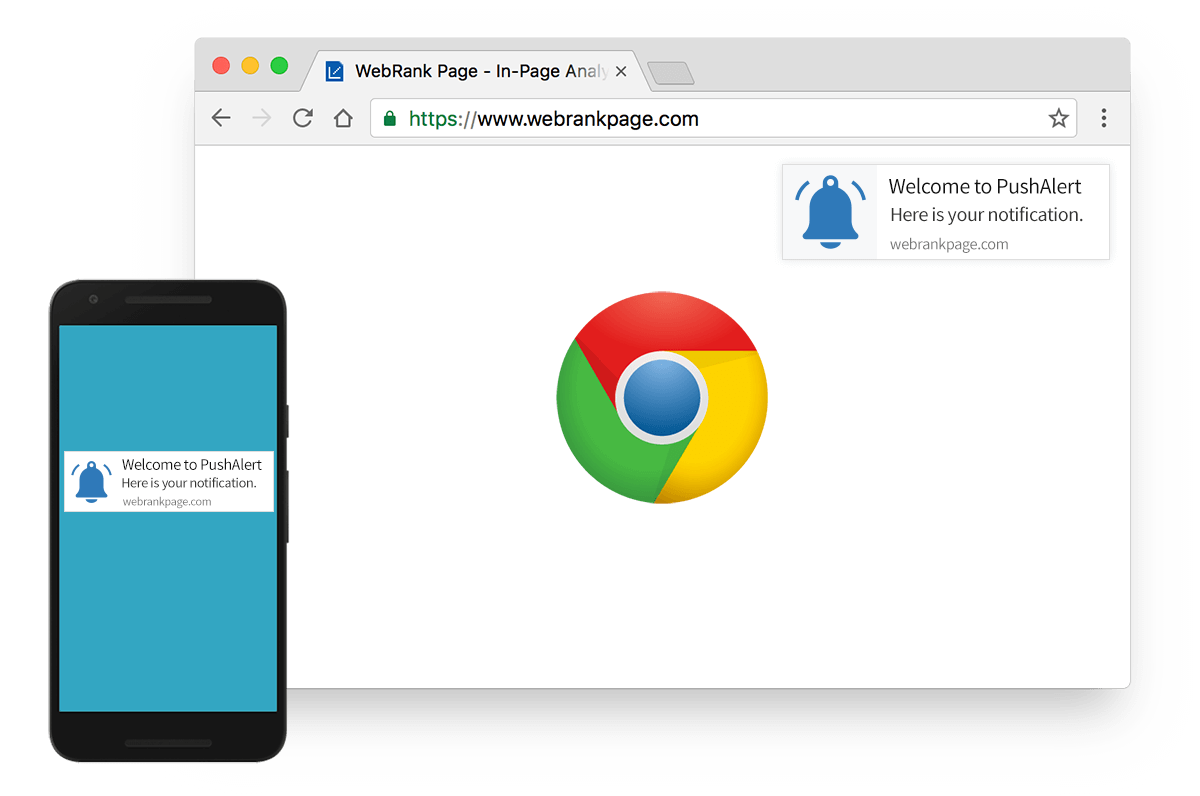
Push notifications javascript example. const myNoti = new Notification('Notification Title', { body: 'This is my notification', icon: 'ICON_URL', image: 'IMAGE_URL' }); Now let's see the live example. JavaScript Desktop Notification Example. Below is an example for showing desktop/browser notification using JavaScript. Push messaging provides a simple and effective way to re-engage with your users and in this code lab you'll learn how to add push notifications to your web app. What you'll learn. How to subscribe and unsubscribe a user for push messaging; How to handle incoming push messages; How to display a notification; How to respond to notification clicks Firebase Push Notification Message with JavaScript. ... In this tutorial, I will show you how to use firebase using JavaScript client to send token and notification messages to the FCM Legacy server.
The Notifications API lets a web page or app send notifications that are displayed outside the page at the system level; this lets web apps send information to a user even if the application is idle or in the background. This article looks at the basics of using this API in your own apps. Typically, system notifications refer to the operating system's standard notification mechanism: think for ... For example, you could do this beforehand with a command-line tool and then hardcode the public key into the JavaScript application and the private key into the application that sends the push notifications. A push notification server consists of two parts: storing deviceIds and sending push notifications. To achieve this we'll create two endpoints: register and send. Register will utilize the mongodb node module mongoose and Send will leverage two platform-specific node modules: apns and node-gcm. We'll also be using restify to set up our ...
The excellent web-push npm module lets you send push notifications without going through an intermediary service like PubNub. This article will walk you through setting up a "Hello, World" example of web push notifications using a vanilla JavaScript frontend and Express on the backend. The final result will look like what you see below. Aug 16, 2020 - This API is only available through ... up a service worker for push notifications in the tutorial, but in case you want to learn more about them, I wrote a few articles on that topic. I recommend that you start with the introduction to the JavaScript API of service work... Push notifications can be a great way of getting your users' attention whenever there is urgent, important and time-sensitive information that you'd like to share with them. We at SessionStack for example, plan to utilize push notifications to let our users know when there is a crash, issue or anomaly in their product.
Dec 21, 2018 - The FCM JavaScript API lets you receive notification messages in web apps running in browsers that support the Push API. This includes the browser versions listed in this support matrix and Chrome extensions via the Push API. Lets start the tutorial How to make web push notifications in PHP, JQuery , AJAX And Mysql. 1. Create a new database with the name " notifikasi ". 2. Create 2 table with name user and notif like the following image : or please execute the following SQL code. One new(er) feature, web push notifications, allows users to receive and display real-time notifications within their web browsers with a little bit of JavaScript code and the Push API. This article will go over what they are and how to set them up within a website.
Note: In the above example we spawn notifications in response to a user gesture (clicking a button).This is not only best practice — you should not be spamming users with notifications they didn't agree to — but going forward browsers will explicitly disallow notifications not triggered in response to a user gesture. Dec 22, 2018 - Using Push.js to Display Web Browser Notifications ... The Notifications API for the web gives us the ability to send custom notifications to our users that we can control using JavaScript. As you might already know, notifications are used to alert the user about important information, and ... Sending Push Notifications from the server. In this article, to send push notifications, we use a Node.js server. An essential thing to keep in mind is that the data needs to be encrypted. An excellent tool for that is the web-push library. To authenticate we need a public and a private VAPID key: the web-push library can generate it for us ...
For example, a push notification could let a user know when a new mail message is available even when the local mail app isn't running. Windows handles a toast notification by displaying a message, such as the first line of a text message. Use this example to send push notifications by using the AWS SDK for JavaScript in Node.js. This example assumes that you've already installed and configured the SDK for JavaScript in Node.js. This example also assumes that you're using a shared credentials file to specify the Access Key and Secret Access Key for an existing IAM user. Guide: Getting Started with Push Notifications API ... For further reading there is also this 5 minute guide. ... To get the information on how to connect to the QuickBlox library, please, refer to the Connect Javascript SDK to Web and Node.js applications page.
How Location-based Push Notifications & Geofencing Work. There two main methods brands can use to send push notifications based on location: Location-based push notifications: These pushes are sent to app users based on user data you've already collected, like your customer's IP address, or information they've provided on order forms like their city, region, and country. Answer: Yes, push notification can be sent from our own back-end. Support for the same has come to all major browsers. Check this codelab from Google to better understand the implementation. Some Tutorials: Implementing push notification in Django Here. Using flask to send push notification Here & Here. Sending push notifcaiton from Nodejs Here This is a set of example notifications that are referenced throughout the web push book content.
Push notifications! First of all here is the git repository, you can simply clone it and run:. npm install npm start. We are going to use inexedDB to store information from push notifications on ... As part of the tutorial, we'll write a full-stack application with a simple JavaScript client that can subscribe or unsubscribe from push notifications. The frontend will also be able to trigger a notification that will be sent to all subscribed clients. Mar 30, 2021 - Learn what web push notifications are and how they work on different browsers, devices, and operating systems. See examples of push notifications.
In this video, we'll talk about Push.js - A javascript library to send desktop notification. Learn how to setup, create a notification and customize the opti... Understanding Push Notifications on the web. Push notifications let your app extend beyond the browser, and are an incredibly powerful way to engage with the user. They can do simple things, such as alert the user to an important event, display an icon and a small piece of text that the user can then click to open up your site. Jun 10, 2019 - Now that you've got a manifest set up you can go back into your sites JavaScript. To subscribe, you have to call the subscribe() method on the PushManager object, which you access through the ServiceWorkerRegistration. This will ask the user to give your origin permission to send push notifications.
Send push notifications using Firebase Cloud Messaging. Using a Web browser, go to https://console.firebase.google and sign in using your Google account. Click the + icon to add a new project and give a name to the project. Click CREATE PROJECT (see Figure 4 ). Take note of the Project ID. Creating Scheduled Push Notifications. Scheduled is the key word there — that's a fairly new thing! When a push notification is scheduled (i.e. "Take your pill" or "You've got a flight in 3 hours") that means it can be shown to the user even if they've gone offline. That's an improvement from the past where push notification ... Aug 01, 2019 - In the above example we call the subscribe method on the pushManager and log the subscription object to the console. Notice we are passing a flag named userVisibleOnly to the subscribe method. By setting this to true, the browser ensures that every incoming message has a matching (and visible) notification...
Step5: Handle Push Notifications. We will create a JavaScript file notification.js and implement function getNotification() to get notification details by making Ajax request to notification.php file. The function will also handle push notification functionality by checking permissions and display it. The JavaScript quickstart sample demonstrates all code required to receive messages. ... In this example, we create a notification with title, body and icon fields. ... Best practices for notifications. If you're familiar with push messaging for web, ... In this tutorial, you will be writing an iOS app to register a device to obtain an unique token ID, so your JavaScript web app can send it push notifications from desktop. When you created a new project template, you should already have an /www/index.js with a few functions that PhoneGap uses have already filled in.
Push Notifications provide a way to deliver some information to a user while they are not using your app actively. The following use cases can be covered by push notifications: Offline messages. Send a chat message when a recipient is offline. In this case, a push notification will be sent automatically if the user is offline. Offline calls. Examples of notifications on macOS and Android. ... When you subscribe a client to push notifications from your JavaScript code, you provide your public key. When the push service generates an endpoint for the device, it associates the provided public key with the endpoint. Sep 26, 2018 - Service Worker thread: This is an independent javascript thread which runs on the background and can run even when the page has been closed. If you notice we haven’t used our service worker till now for any purpose other than just registering it. With respect to Push Notifications, the JS ...
Adding Push Notifications In React Native With Custom Sounds
Implementing Push Notifications In Progressive Web Apps Pwas
Which Push Notification Tool Should You Use Segment Blog
Push Notifications Using Node Js Amp Service Worker
Push Notifications For Chrome Pushalert
Javascript Desktop Browser Push Notification Example
Push Notifications In Javascript Yes You Can By Lorenzo
Push Notifications Demo Vue Js Feed
Push Notifications In Javascript Yes You Can By Lorenzo
What Are Push Notifications An In Depth Guide For 2021
Web Push Notifications Timely Relevant And Precise
Apple Push Notifications With React Native And Node Js By
10 Useful Javascript Notification Libraries Bashooka
Github Invokemedia Vue Push Notification Example An
How To Create Web Push Notifications Full Tutorial
Adding Push Notifications To A Web App Web Fundamentals
Push Js Tutorial Javascript Desktop Notification Laptrinhx
Using The Notifications Api Web Apis Mdn
Web Push Notification Full Stack Application With Node Js
0 Response to "22 Push Notifications Javascript Example"
Post a Comment