27 Javascript Array Pop First
Definition and Usage. The push() method adds new items to the end of an array.. push() changes the length of the array and returns the new length. Tip: To add items at the beginning of an array, use unshift(). Apr 28, 2021 - No votes so far! Be the first to rate this post. We are sorry that this post was not useful for you! ... Thanks for reading. Please use our online compiler to post code in comments using C, C++, Java, Python, JavaScript, C#, PHP, and many more popular programming languages. Like us?
Remove Element From Array Javascript First Last Value
Array.prototype.find () The find () method returns the value of the first element in the provided array that satisfies the provided testing function. If no values satisfy the testing function, undefined is returned. If you need the index of the found element in the array, use findIndex () . If you need to find the index of a value, use Array ...
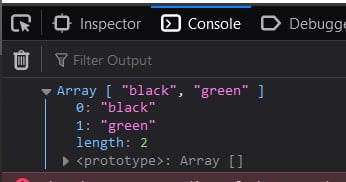
Javascript array pop first. JavaScript Array pop () Method. This method deletes the last element of an array and returns the element. Syntax: array.pop () Return value: It returns the removed array item. An array item can be a string, a number, an array, a boolean, or any other object types that are applicable in an array. May 31, 2020 - var list = ["bar", "baz", "foo", "qux"]; list.shift()//["baz", "foo", "qux"] Code language: JavaScript (javascript) The figure below illustrates each step in the script. Initially, the stack has 5 elements. The pop () method removes the element at the end of the array i.e., at the top of the stack one at a time.
Removing Elements from Beginning of a JavaScript Array. How do you remove the first element of a JavaScript array? The shift method works much like the pop method except it removes the first element of a JavaScript array instead of the last. There are no parameters since the shift method only removed the first array element. Array indexing in JavaScript Note: Array's index starts with 0, not 1. Add an Element to an Array You can use the built-in method push () and unshift () to add elements to an array. The shift() method removes the first item of an array. Note: This method changes the length of the array. Note: The return value of the shift method is the removed item. Tip: To remove the last item of an array, use the pop() method.
Removing the first element. To remove the first element of an array, we can use the built-in shift () method in JavaScript. Here is an example: const fruits = ["apple", "banana", "grapes"]; fruits.shift(); console.log(fruits); Note: The shift () method also returns the removed element. Similarly, we can also remove the first element of an array ... Definition and Usage. The shift () method removes the first item of an array. shift () returns the element it removes. shift () changes the original array. Tip: To remove the last item of an array, use pop (). pop () is a widely used method in Javascript which follows the principle of "Last come First Out" which means the element which was inserted at the end of the array is the one which will be removed first by it. We have seen the same in all of the above examples as well.
The first is an expression and the second is the radix to the callback function, Array.prototype.map passes 3 arguments: the element; the index; the array; The third argument is ignored by parseInt—but not the second one! This is the source of possible confusion. Here is a concise example of the iteration steps: In this case, you can use shift()method to get the first element, but be cautious that this method modifies the original array (removes the first item and returns it). Therefore the length of an array is reduced by one. This method can be used in inline cases where you just need to get the first element, but you dont care about the original array. var arr = [1, 2, 3, 4]; var theRemovedElement = arr.shift(); // theRemovedElement == 1 console.log(arr); // [2, 3, 4]
Shifting is equivalent to popping, working on the first element instead of the last. The shift () method removes the first array element and "shifts" all other elements to a lower index. 15 Common Operations on Arrays in JavaScript (Cheatsheet) The array is a widely used data structure in JavaScript. The number of operations you can perform on arrays (iteration, inserting items, removing items, etc) is big. The array object provides a decent number of useful methods like array.forEach (), array.map () and more. pop(): Remove an item from the end of an array. push(): Add items to the end of an array. shift(): Remove an item from the beginning of an array. unshift(): Add items to the beginning of an array. ... const array1 = [1, 2, 3]; const firstElement = array1.shift(); console.log(array1); // expected ...
Which method will you use to remove the first element from an array · How to check whether a checkbox is checked in jQuery · Access to XMLHttpRequest at 'http://localhost:5000/mlphoto' from origin 'http://localhost:3000' has been blocked by CORS policy: No 'Access-Control-Allow-Origin' header ... The more elements in the array, the more time to move them, more in-memory operations. The similar thing happens with unshift: to add an element to the beginning of the array, we need first to move existing elements to the right, increasing their indexes.. And what's with push/pop?They do not need to move anything. How to use length property, delete operator, and pop, shift, and splice methods to remove elements from the end, beginning, and middle of arrays in JavaScript.
Example 1: javascript pop first element pop(): Remove an item from the end of an array. push(): Add items to the end of an array. shift(): Remove an item from the be javascript by Sleepy Sardine on Jan 16 2021 Comment 6 pop(): Remove an item from the end of an array. push(): Add items to the end of an array. shift(): Remove an item from the beginning of an array. unshift(): Add items to the beginning of an array. JavaScript Array Management with Push (), Pop (), Shift () and Unshift () When you need to work with the first or last elements in a JavaScript array, the push (), pop (), shift () and unshift () methods are usually the best way to go. Programmers who are new to JavaScript or come to it via languages such as PHP may find arrays a bit limiting.
To remove the first item of an array in javascript (it will also work if you're using jquery) use data.urls.shift(). This will also return the first item, but you can ignore the return value if you don't want to use it. For more info, see http://www.w3schools /jsref/jsref_shift.asp. Example 1: javascript pop first element pop(): Remove an item from the end of an array. push(): Add items to the end of an array. shift(): Remove an item from the be The pop method removes the last element from an array and returns that value to the caller. pop is intentionally generic; this method can be called or applied to objects resembling arrays. Objects which do not contain a length property reflecting the last in a series of consecutive, zero-based numerical properties may not behave in any meaningful manner.
Mar 29, 2019 - The key difference between the two methods lies in Steps 1 and 2. splice() creates a new array and populates it with the first item. This instantiation and definition of a previously non-existent property 0 is relatively computationally expensive. 1 week ago - The shift() method removes the first element from an array and returns that removed element. This method changes the length of the array. Javascript Array: Shift () Method The shift () method is like the pop () method, only it works at the beginning of the array. The shift () method pulls the first element off of the given array and returns it. This alters the array on which the method was called.
Top 8 Methods in Multi-Dimensional Array in JavaScript. Below are the methods used in Multi-Dimensional Array in JavaScript: 1. Pop( ) This method is used to remove the element at the last index of the array. This will eventually result in the array length decreased by 1. Code: var … Don't confuse this with its similar cousin slice() that is used to extract a section of an array. ... If you're always interested in removing the first or the last item, then you have an alternative solution. The methods shift() and pop() exist precisely for this purpose. The pop() method removes the last element of an array and returns that element. ... Try a Top Quality Javascript Developer for 7 Days. Pay Only If Satisfied.
Returns a new array containing the results of calling a function on every element in this array. Array.prototype.pop() Removes the last element from an array and returns that element. Array.prototype.push() Adds one or more elements to the end of an array, and returns the new length of the array. Array.prototype.reduce() Sep 08, 2017 - I have list of items created via ng-repeat. I also have Delete button. Clicking delete button removes last item of the array one by one. Plunker But I want to remove items one by one starting from... Feb 26, 2019 - In JavaScript, and just like many other languages out there, at some point you'll likely need to remove an element from an array. Depending on your use-case this could be as easy as using the built-in shift() or pop() commands, but that only works if the element is at the beginning or end of ...
Note that slice(1) doesn't "remove the first element" from the array. Instead, it creates a new array with shallow copies of all of the values from the array except the first. - T.J. Crowder Jul 1 '16 at 17:29 26/5/2021 · In this example: arr [0] is an array. So “as usual”, arr [0] [0] refers to the first element, arr [0] [1] refers to the second element. arr [1] is an object. So “as usual”, use arr [1] ["PROPERTY"] to access it. arr [2] is a function. So “as usual”, we call the function using arr [2] (). If it is too confusing, think this way ... 7/1/2020 · shift () removes the first element. unshift () adds an element to the beginning of the array. Let’s look at the methods in full. Array pop () The pop () method is a method applied on arrays which removes the last element of the array. i.e it removes the element at the highest index value – array [array.length - 1] .
JavaScript gives us four methods to add or remove items from the beginning or end of arrays: pop(): Remove an item from the end of an array let cats = ['Bob', 'Willy', 'Mini']; cats.pop(); // ['Bob', 'Willy'] pop() returns the removed item. push(): Add items to the end of an array Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John: The "shift method" works much like the pop method except it removes the first element of a JavaScript array instead of the last. There are no parameters since the shift method only removed the ...
Javascript array pop() method removes the last element from an array and returns that element. Syntax. Its syntax is as follows −. array.pop(); Return Value. Returns the removed element from the array. Example. Try the following example.
Unshift Javascript Unshift Array Methods In Javascript
Remove Element From Array Using Slice Stack Overflow
Best Way To Remove Or Delete A Specific Element From An Array
Remove Element From Array Javascript First Last Value
Implementing A Javascript Stack Using Push Amp Pop Methods Of
Pop Push Shift And Unshift Array Methods In Javascript
Array Shift Codesnippet Io Javascript Tips Amp Tricks
Ravin On Twitter Javascript Array Methods Cheat Sheet Part
Remove Element From Array Javascript First Last Value
How To Find Even Numbers In An Array Using Javascript
Javascript Array Methods Shift Unshift Push Pop
Javascript Array Methods How To Use Map And Reduce
Javascript Interview Questions Arrays Kevin Chisholm Blog
The Best Way To Remove The First Element Of An Array In
Working With Arrays In Javascript Learnbatta
Javascript Remove The Last Item From An Array Geeksforgeeks
Hacks For Creating Javascript Arrays
4 Ways To Convert String To Character Array In Javascript
Javascript Array Push Adding Elements In Array With
Push And Pop Array Methods In Javascript Tutorial First
How Can I Remove A Specific Item From An Array Stack Overflow
Javascript Array Methods How To Use Map And Reduce
Algorithms 101 Rotate Array In Javascript Three Solutions
Javascript Pop First Element From Array
Remove First N Elements From Array Javascript Example Code
Manipulating Javascript Arrays Removing Keys By Adrian
0 Response to "27 Javascript Array Pop First"
Post a Comment