25 How To Define A Function In Javascript
Functions don't need to be declared directly inside a tag. You can also put the code: function functionname() { Alert('hello world') } anywhere between the two script tags. As for your question on having the code run after the user clicks the table cell, you can do this. One small thing that confused me as I was learning JavaScript was the many different kinds of ways to define a JavaScript function. I could never seem to find one place that showed all of them, and explained why you might use each one. That is the aim of this post. So without further ado, here are ALL the ways to define a JavaScript function.
Var Functionname Function Vs Function Functionname
How to Create a Function in JavaScript. Use the keyword function followed by the name of the function. After the function name, open and close parentheses. After parenthesis, open and close curly braces. Within curly braces, write your lines of code. This code is editable. Click Run to Execute.
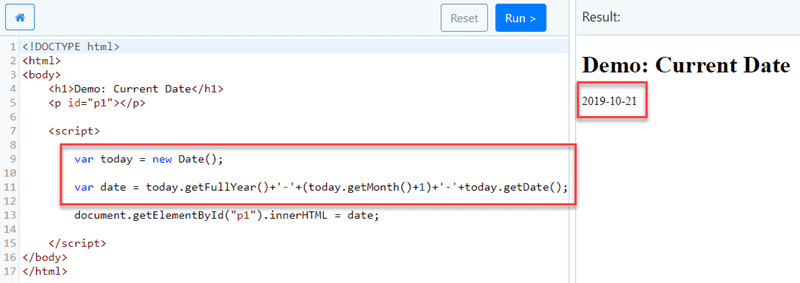
How to define a function in javascript. JavaScript Functions JavaScript provides functions similar to most of the scripting and programming languages. In JavaScript, a function allows you to define a block of code, give it a name and then execute it as many times as you want. A JavaScript function can be defined using function keyword. Nov 02, 2019 - A function is a parametric block of code defined once and called multiple times later. In JavaScript a function is composed and influenced by many components: ... This post teaches you six approaches to declare JavaScript functions: the syntax, examples and common pitfalls. Before we use a function, we need to define it. The most common way to define a function in JavaScript is by using the function keyword, followed by a unique function name, a list of parameters (that might be empty), and a statement block surrounded by curly braces.
A JavaScript function is defined with the function keyword, followed by a name, followed by parentheses (). Function names can contain letters, digits, underscores, and dollar signs (same rules as variables). The parentheses may include parameter names separated by commas: (parameter1, parameter2,...) The function is a way to create a block of code that can be reused in JavaScript programs again and again by simply calling the function. Function in JavaScript may take one or more parameters and may also return value. A JS function code will execute only when it is called. JavaScript functions are basically objects. Defining and Calling a Function Function starts with the keyword function, followed by the name of the function you want to create in JavaScript.
JavaScript Function: Definition. Now that you have an idea, what a function is, let's see its definition, and how we can define a function, the basic function structure, and using a function in JavaScript. JavaScript function is a set of statements that are used to perform a specific task. It can take one or more input and can return output as ... A JavaScript function is a block of code that consists of a set of instructions to perform a specific task. A function can also be considered as a piece of code that can be used over again and again in the whole program, and it also avoids rewriting the same code. We define a function in Javascript with the help of the ' function' keyword, followed by the function name, followed by parenthesis ' () ' (will discuss more on this), followed by opening curly...
Function Basics. Level Set! If you are coming from a programming background, you all know what declaring a function means, right? It looks something like this in JavaScript. A function is a set of statements that takes input, do some specific computation and produce output. Basically, a function is a set of statements that performs some specific task or does some computation and then return the result to the user. async function. An async function is a function declared with the async keyword, and the await keyword is permitted within them. The async and await keywords enable asynchronous, promise-based behavior to be written in a cleaner style, avoiding the need to explicitly configure promise chains. Async functions may also be defined as expressions.
set1.forEach (callback [,thisargument]); Parameter: callback - It is a function which is to be executed for each element of the Set. thisargument - Value to be used as this when executing the callback. In JavaScript, functions are called Function Objects because they are objects. Just like objects, functions have properties and methods, they can be stored in a variable or an array, and be passed as arguments to other functions. Functions are First-Class Objects Jul 20, 2021 - Each must be a string that conforms to the rules for a valid JavaScript identifier or a list of such strings separated with a comma; for example "x", "theValue", or ";a,b";. ... A string containing the JavaScript statements comprising the function definition. Invoking the GeneratorFunction ...
In JavaScript functions are defined with the function keyword, followed by the name of the function, a pair of parentheses (opening and closing), and a code block. Let's take a look at the following example to understand how to define a function in JS. Using the dollar sign is not very common in JavaScript, but professional programmers often use it as an alias for the main function in a JavaScript library. In the JavaScript library jQuery, for instance, the main function $ is used to select HTML elements. In jQuery $("p"); means "select all p elements". Local variables can be accessed only within the specified function. That is why, you can't reach them from any other function in the document. It is recommended to use local variables in JavaScript functions because it allows using variables with the same name, as they are local variables that stay within separate functions.
HTML,CSS,JavaScript,DHTML,XML,XHTML,ASP,ADO and VBScript tutorial from W3Schools. JavaScript functions are defined with the function keyword. You can use a function declaration or a function expression. ... Declared functions are not executed immediately. They are "saved for later use", and will be executed later, when they are invoked (called upon). ... Semicolons are used to ... This is rather close to the way mathematicians define exponentiation and arguably describes the concept more clearly than the looping variant. The function calls itself multiple times with ever smaller exponents to achieve the repeated multiplication. But this implementation has one problem: in typical JavaScript ...
Jul 26, 2021 - There are a few different ways in which a function can be defined in JavaScript, and the way it is defined affects function behavior. Let’s explore each way one by one. ... This might be the most familiar way to define a function. A function declaration consists of a name preceded by the ... In JavaScript, a constructor function is used to create objects. For example, // constructor function function Person () { this.name = 'John', this.age = 23 } // create an object const person = new Person (); In the above example, function Person () is an object constructor function. To create an object from a constructor function, we use the ... Function Declaration is made up of the keyword, function, followed by the mandatory name of the function, and then the parameter within a pair of parenthesis.(You can also define a function ...
In a function definition, this refers to the "owner" of the function. In the example above, this is the person object that "owns" the fullName function. In other words, this.firstName means the firstName property of this object. Read more about the this keyword at JS this Keyword. ... JavaScript ... A JavaScript function is a group of reusable all the source code which can be called any where in your web page or script. This terminate the required of writing the same source code repete and repete. It useful programmers in writing modular or MVC based source codes. Functions allow a devloper to divide a big script project into a number of ... If I had 3 variables needed by 2 functions: 1. (best) pass the 3 variables into both functions -- the functions can be defined once and only once. 2. (good) create the 2 functions where the variables are outside the scope of both. This is a closure. (Basically the answer here.) Sadly, the functions are redefined for each new use of the variables. 3.
Dec 02, 2018 - Functions always return a value. In JavaScript, if no return value is specified, the function will return undefined. Functions are objects. Define a Function. There are a few different ways to define a function in JavaScript: Read our JavaScript Tutorial to learn all you need to know about functions. Start with the introduction chapter about JavaScript Functions and JavaScript Scope. For more detailed information, see our Function Section on Function Definitions, Parameters, Invocation and Closures. JavaScript Functions. In this tutorial you will learn how to define and call a function in JavaScript. What is Function? A function is a group of statements that perform specific tasks and can be kept and maintained separately form main program. Functions provide a way to create reusable code packages which are more portable and easier to debug.
May 11, 2021 - So, there are indeed reasons why class can be considered a syntactic sugar to define a constructor together with its prototype methods. Still, there are important differences. First, a function created by class is labelled by a special internal property [[IsClassConstructor]]: true. Functions are defined, or declared, with the function keyword. Below is the syntax for a function in JavaScript. function nameOfFunction() { } The declaration begins with the function keyword, followed by the name of the function.
A function defined like this is accessible from anywhere within its context by its name. But sometimes it can be useful to treat function references like object references. For example, you can assign an object to a variable based on some set of conditions and then later retrieve a property from one or the other object: Javascript Web Development Front End Technology Object Oriented Programming To define custom sort function, you need to compare first value with second value. If first value is greater than the second value, return -1. If first value is less than the second value, return 1 otherwise return 0. Example 3: Add Two Numbers. // program to add two numbers using a function // declaring a function function add(a, b) { console.log (a + b); } // calling functions add (3,4); add (2,9); Output. 7 11. In the above program, the add function is used to find the sum of two numbers. The function is declared with two parameters a and b.
Jun 21, 2021 - For example, the jQuery framework defines a function with $. The Lodash library has its core function named _. These are exceptions. Generally function names should be concise and descriptive. ... Functions should be short and do exactly one thing. If that thing is big, maybe it’s worth it to split the function into ... Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. Jun 24, 2011 - In JavaScript, there’s many different ways of doing something. This is both a good thing and a bad thing. To the newcomer this is definitely a bad thing, as it means not only more things to learn, but more little caveats and more places to go wrong. And so it is with declaring functions!
Jul 20, 2021 - You can also define functions using the Function constructor and a function expression. ... The function name. ... The name of an argument to be passed to the function. Maximum number of arguments varies in different engines.
We Need To Minimize The Use Of Global Variables In Javascript
Javascript Fixing Function Is Not Defined Error
5 Differences Between Arrow And Regular Functions
Define And Call A Function In Javascript With Example
Arrow Functions Vs Regular Functions In Javascript Level Up
Python Functions Examples Call Indentation Arguments
How To Define A Javascript Function In Html 6 Steps
Var Functionname Function Vs Function Functionname
How To Define A Javascript Function In Html 6 Steps
Javascript Immediately Invoked Function Expressions Iife
Chapter 2 Variables Functions Objects And Events
Learn How To Get Current Date Amp Time In Javascript
All The Javascript You Need To Know Before Starting With React
Xpages And More Bootstrap Plugins In Xpages Part V Jquery
Practical Javascript Programming Session 3 8
Function Bind Scopes Closures In Javascript Codeburst
Mastering This In Javascript Callbacks And Bind Apply
Run Javascript In The Console Chrome Developers
Map In Javascript Geeksforgeeks
5 Javascript Terms Beginners Need To Know Course Report
Python Vs Javascript What Are The Key Differences Between
Javascript Es6 Write Less Do More
Instantiation Patterns In Javascript By Jennifer Bland
Three Ways To Create A Javascript Class Learn Web Tutorials
0 Response to "25 How To Define A Function In Javascript"
Post a Comment