35 How To User Input In Javascript
Apr 28, 2017 - Not the answer you're looking for? Browse other questions tagged javascript html input user-input or ask your own question. ... To subscribe to this RSS feed, copy and paste this URL into your RSS reader. It is a scripting language basically built for the web. It doesn't support standard input output stream, but using methods we can implement this functionality. In JavaScript, we have a prompt () method which takes the user input through a popup and return the user entered data. Here is an example,
Dynamically Change Background Color Based On User Input
To access all the values entered in input fields use the following method: var input = document.getElementsByName ('array []'); The document.getElementsByName () method is used to return all the values stored under a particular name and thus making input variable an array indexed from 0 to number of inputs.
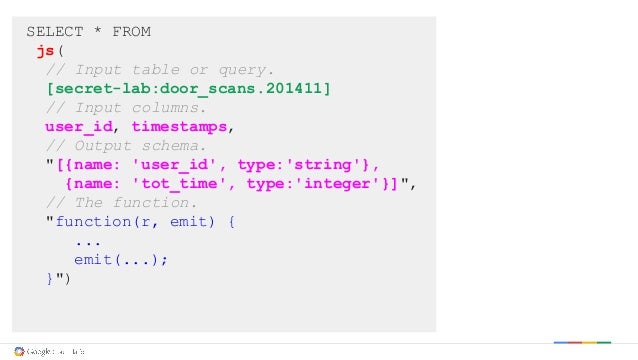
How to user input in javascript. Jan 24, 2017 - I want to get user inputs using the tag in HTML and not the prompt function in JavaScript. I also don't want to have to use another language on top of this like php. Edit Something like this wou... formname.getElementsByTagName('input') is an array containing all of the input fields in the form. When you get the length of an array it tells you how many entries are in the array. There are several methods are used to get an input textbox value without wrapping the input element inside a form element. Let's show you each of them separately and point the differences. The first method uses document.getElementById ('textboxId').value to get the value of the box:
When the user clicks on the button, the onclick event has been fired and the JavaScript message listed in the value of the attribute are executed. ... Your Name is ____. The city in which you live is ____. <!DOCTYPE html> <html> <head> <title>User Input</title> </head> <body> <button ... You can simply use the value property of the DOM input element to get the value of text input field. The following example will display the entered text in the input field on button click using JavaScript. 9/6/2021 · There are two ways of getting user input from JavaScript depending on whether you want to get the input from the browser or NodeJS. This tutorial will help you to learn both. Getting user input from the browser console. To ask for user input from the browser, you need to use the prompt() method provided by the browser.
In this video tutorial we will learn how to take input from user from a HTML textbox using JavaScript.We will be using the document object & its getElementBy... Giraffe Academy is rebranding! I've decided to re-focus the brand of this channel to highlight myself as a developer and teacher! The newly minted Mike Dane ... Contact forms in JavaScript look very similar, most of the time there is an input field for name, surname, address, email and of course for a question or a message. Making a contact form from the image above is our goal. Before we start creating our contact form in JavaScript, we need to cover some ground.
var name = prompt("Please enter your name", "Harry Potter"); Most often, the purpose of data validation is to ensure correct user input. Validation can be defined by many different methods, and deployed in many different ways. Server side validation is performed by a web server, after input has been sent to the server. Client side validation is performed by a web browser, before input is sent to a web server. This tutorial provides an introduction to working with JSON in JavaScript. Some general use cases of JSON include: storing data, generating data from user input, transferring data from server to client and vice versa, configuring and verifying data.
Mar 31, 2015 - In the JavaScript code we have a function called say_hi. It used the getElementById we have already seen to locate the DOM element representing the input element with the id first_name. The object returned has a method value that will return the text the user has typed in that field. This looks like code for SpiderMonkey, the Command Line JavaScript runtime environment from Mozilla. readline () is their method to read stdin and print () is the stdout method. If you come from web, you could almost compare readline to prompt (), the script simply pauses and waits until something comes from user input. Functions are one of the fundamental building blocks in JavaScript. A function in JavaScript is similar to a procedure—a set of statements that performs a task or calculates a value, but for a procedure to qualify as a function, it should take some input and return an output where there is some obvious relationship between the input and the output. To use a function, you must define it ...
JavaScript HTML Input Examples Previous Next Examples of using JavaScript to access and manipulate HTML input objects. Button Object. Disable a button Find the name of a button Find the type of a button Find the value of a button Find the text displayed on a button Find the id of the form a button belongs to. To achieve all of these objectives and more, JavaScript comes with the built in Date object and related methods. This tutorial will go over how to format and use date and time in JavaScript. The Date Object. The Date object is a built-in object in JavaScript that stores the date and time. It provides a number of built-in methods for formatting ... If there are multiple Radio buttons in a webpage, first, all the input tags are fetched and then the values of all the tags that have type as 'radio' and are selected are displayed. Example 1: The following program displays the value of selected radio button when user clicks on Submit button.
However, we can use JavaScript to do exactly that: enter data into form fields. Let's view an example straight away to illustrate how this works. ... And in the third, we captured the user input ... Sometimes the programs also need to collect user input. For example, a map program can ask you which city you are interested in. There are several different ways to collect user input. In JavaScript, prompt() is a user-interface function, it allows us to get a piece of text from user. JavaScript Message Boxes: alert (), confirm (), prompt () JavaScript provides built-in global functions to display messages to users for different purposes, e.g., displaying a simple message or displaying a message and take the user's confirmation or displaying a popup to take the user's input value.
If you don’t know your input is going to be a string value than I’d suggest to convert it into a string with a template literal such as backtick quotes. const age = 20; console.log(typeof `$ {age}`); If you don’t support ES6 features, than try this method: const age = 20; console.log( age + ''); One way to ask a user for data is by using the JavaScript prompt command. To try out the prompt command, open the JavaScript console and type the following: prompt("What is your name?"); After you press Return or Enter, a pop‐up window appears in your browser window with a text field, as ... In this lesson we'll take a look at how to write programs in JavaScript which accept user input. ... Programs which get input from the user need to be written in a specific way. The program will essentially prompt the user for information, and then when the user hits enter, will take whatever ...
The Scanner class is used to get user input, and it is found in the java.util package. To use the Scanner class, create an object of the class and use any of the available methods found in the Scanner class documentation. In our example, we will use the nextLine () method, which is used to read Strings: I want to get user inputs using the tag in HTML and not the prompt function in JavaScript. I also don't want to have to use another language on top of this like php. Edit Something like this wou... Jul 16, 2020 - You can use # to get the value when user clicks the button using document.querySelector(“”); Following is the JavaScript code − ... <!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>Document</title> ...
Sep 06, 2019 - Now that you can capture user input, you are able to take that and do all kinds of things with it like form validation, mathematical calculations, etc. If you’d like to learn more be sure to check out our JavaScript Training. Have an amazing day! JavaScript is especially useful when you want to take user information and process it without sending the data back to the server. JavaScript is much faster than sending everything to the server to process, but you must be able to read user input and use the right syntax to work with that input. Oct 24, 2016 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers.
After input text and click: Using jquery val() method: The val() method is used to return or set the value attribute of the selected elements.In default mode this method returns the value of the value attribute of the FIRST matched element & sets the value of the value attribute for ALL matched elements. I'm new to JavaScript and have run into a challenge (for me). I've looked online for a solution, but to my surprise I couldn't find it. This must have a very basic solution! The problem: I want JavaScript to execute an if statement based on user input from a text field. Learn how to handle user input synchronously in Node.js. The prompt() function returns the user feedback, so simply store that return value to a variable to use it later. In the example above, the name variable stores the value, and it is then repeated to the user on the next line.. Letting Users Exit. By default, most terminal programs will exit with Ctrl + C (This sends a SIGINT, or ...
Write a javascript to prompt for user name and display a welcome message and username. ... You work on a JavaScript project. How do you prompt users with messages and at the same requesting user inputs? ... Create a prompt pop-up box by using prompt() method. Add a default text //in the prompt ... JavaScript lets you perform client-side dynamic calls to forms and the input typed by your users. For instance, suppose you want to ensure that the user has entered a value into a "first_name" text box before you send the input to the server. Definition and Usage The prompt () method displays a dialog box that prompts the visitor for input. A prompt box is often used if you want the user to input a value before entering a page. Note: When a prompt box pops up, the user will have to click either "OK" or "Cancel" to proceed after entering an input value.
how do you get input from a user js? ... use the window.prompt() method to ask the user for a label for the heading. ... You work on a JavaScript project. How do ... Make JavaScript take HTML input from user, parse and display? Javascript Web Development Front End Technology Object Oriented Programming. The HTML input value is a string. To convert the string to integer, use parseInt (). var messageBox = document.getElementById ("display"); The insert () function uses the references to each input field to get their value. It then uses the push () method on the respective arrays to put the current value into the array.
The <input> tag specifies an input field where the user can enter data. The <input> element is the most important form element. The <input> element can be displayed in several ways, depending on the type attribute. The different input types are as follows: 2/1/2018 · All you need is a button and an onclick event. The onclick event calls a function that checks input and writes to the <div>. function wawa () { var variable = document.getElementById ('input_id').value; document.getElementById ('alert').innerHTML = 'The user input is: ' + variable; } Sometimes we need to set a default value of the <input> element, This example explains methods to do so. Text Value Property This property set/return the value of value attribute of a text field. The value property contains the default value, the value a user types or a value set by a script. Syntax: Return the value property: textObject.value
70 480 Exam Dumps Hotspot You Are Validating User Input By
Bigquery Javascript User Defined Functions By Thomas Park And
Github Any Code Input Validation Validate User Input Using
Javascript Input Value From The User Using Prompt
Azure Stream Analytics Javascript User Defined Functions
Javascript Prompt How Does Prompt Pop Up Work In Javascript
Validate User Input With Javascript
Executing Javascript Inside Css Another Reason To Whitelist
On Twitter Javascript Has A 20y Old Built In Function
Process Number Input In Javascript Form Stack Overflow
Create Pie Chart From User Input In Articulate Storyline
Todo Application With Html And Javascript By Kishore Ravi
Accepting User Input Javascript For Beginners Learn With 6
Node Js Ask For User Input Code Example
Custom Input Formatting In Pega Saltech Consulting
Put Labels In Your Html Input Text With Javascript Jquery
Mobile Web App Html5 Canvas Javascript Interactive User
How To Filter User Input An Overview Infosec Resources
Javascript Basics Tutorial 3 Simple User Input And Output
Pass The Message User Input And Output Using Html Css
How Do I Get Console Input In Spidermonkey Javascript
Evaluate A User Input String As An Expression Axure Rp 8
Features Of Javascript 13 Vital Javascript Features You
Week 3 User Input Validation With Java Script
Javascript Tutorial Working With User Input Lynda Com
Javascript Text Input Javascript Text Box Taking Input
User Input Introduction To Computer Science
Xss Bypass Filters Amp Sanitization
Storing User Input In A Variable General Discussions
Take User Input Form Console Node Js Code Example
User Inputs To Dynamic Table Using Javascript Stack Overflow
How To Make My Js Button Accept User Input I Want To Submit
0 Response to "35 How To User Input In Javascript"
Post a Comment