30 Check Number In Array Javascript
The indexof () method in Javascript is one of the most convenient ways to find out whether a value exists in an array or not. The indexof () method works on the phenomenon of index numbers. This method returns the index of the array if found and returns -1 otherwise. Let's consider the below code: javascript: count number of array element occurrences in another array find frequency of elements in an array in n time and 1 space in js stackoverflow occurrence findd in number array
Should You Use Includes Or Filter To Check If An Array
We have various ways to check if a value is a number. The first is isNaN (), a global variable, assigned to the window object in the browser: const value = 2 isNaN(value) //false isNaN('test') //true isNaN({}) //true isNaN(1.2) //false If isNaN () returns false, the value is a number.
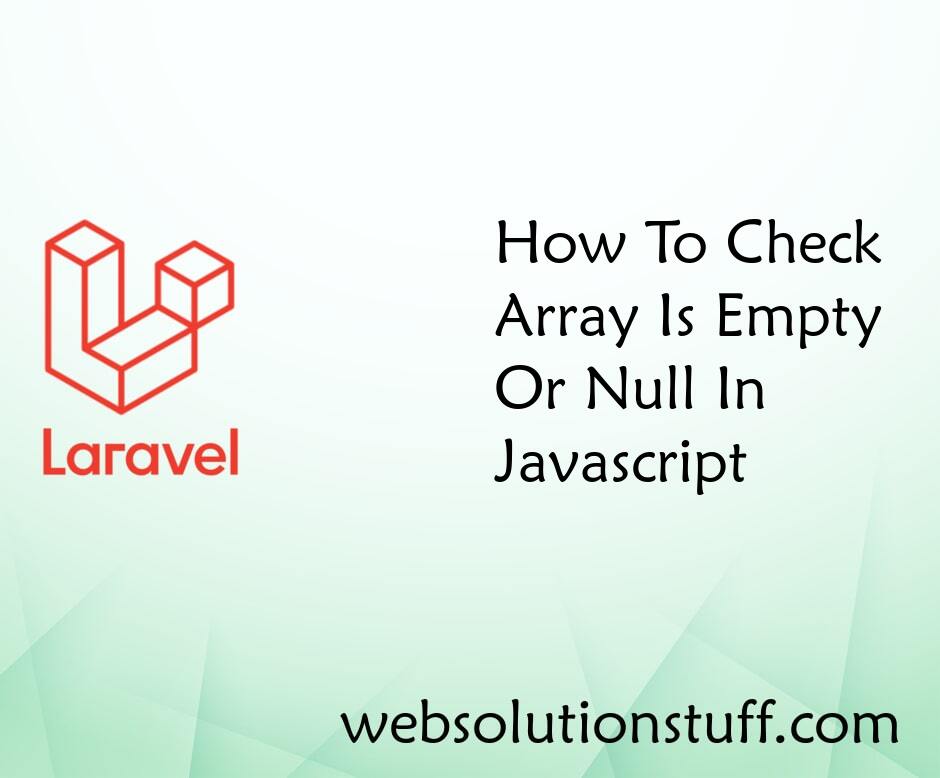
Check number in array javascript. The syntax for the array.find () is let element = array.find (callback); The callback is the function that is executed on each value in the array and takes three arguments: element - the element being iterated on (required) Jul 24, 2021 - The Math.max() function returns the largest of the zero or more numbers given as input parameters, or NaN if any parameter isn't a number and can't be converted into one. get prime numbers from array using js, fetch prime numbers in array by javascript, extract prime numbers from array using javascript. We will take a variable of javascript for examples. And we will take the integer value from 2 to leke 20 in the array in that variable.
JavaScript: Check If Array Elements (Numbers) Are in Order. This is a snippet to check whether array elements (which all are numbers) are ordered (ascending / descending). In ascending order, for instance, we have these arrays to test: In descending order, we have these arrays to test: So then, let's go type a JavaScript function. How to check if a value exists in an array using Javascript? We continue with Flexiple's tutorial series to explain the code and concept behind common use cases. In this article, we will solve for a specific case: To check if a value exists in an array. We then also look at its implementation in Javascript and jQuery. Where can we use this? Dec 19, 2019 - Check if a value exists in a JavaScript array. This is a tutorial on how to check if a given value exists inside a JavaScript array. This can be useful if you want to avoid adding duplicate values to an array. It can also be used for client side searches and whatnot.
The Array.isArray() method determines whether the passed value is an Array. Aug 21, 2020 - JavaScript offers a bunch of useful array methods to check whether an array contains a particular value. While searching for primitive value like number or string is relatively easy, searching for objects is slightly more complicated. In this post, you will read about how to determine if an ... By using Javascript Array Length property can get the numbers of elements in an Array. A Lenght property returns the number of elements in that array. Length of Array can be stored in JavaScript variable. Now, you might be thinking what is use of Array Length?
The simplest and fastest way to check if an item is present in an array is by using the Array.indexOf () method. This method searches the array for the given item and returns its index. If no item is found, it returns -1. You can use the indexOf () method to check whether a given value or element exists in an array or not. The indexOf () method returns the index of the element inside the array if it is found, and returns -1 if it not found. Let's take a look at the following example: Aug 20, 2016 - Edit: Note that this returns false if the item in the array is an object. This is because similar objects are two different objects in JavaScript. ... @nirvanaswap A polyfill is a script you can use to ensure that any browser will have an implementation of something you're using. In this case, you'd add a script that checks ...
2 weeks ago - I think that the simple calculation ... index in an array is so simple that it's two times faster than doing an actual loop iteration. Here is a complex example doing three checks per iteration, but this is only possible with a longer calculation which causes the slowdown of the code. ... If you are using JavaScript 1.6 or later ... Method 2: Create an empty object and loop through first array. Check if the elements from the first array exist in the object or not. If it doesn't exist then assign properties === elements in the array. Loop through second array and check if elements in the second array exists on created object. Jun 04, 2021 - In this tutorial, I show How you can check whether an Array already contains a specific value or not. This requires within the program in some cases like - Stop new value from insert if it already exists in an Array, execute script when the Array contains the particular value, etc..
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. In javascript we can check whether a variable is array or not by using three methods. 1) isArray() method. The Array.isArray() method checks whether the passed variable is array or not. If the variable is an array it displays true else displays false.
Make a program that filters a list of strings and returns a list with only your friends name in it.javascript ... Write a function called lucky_sevens which takes an array of integers and returns true if any three consecutive elements sum to 7. by js Sep 15, 2020 - The includes() method determines whether an array includes a certain value among its entries, returning true or false as appropriate. Array.from() Creates a new Array instance from an array-like or iterable object.. Array.isArray() Returns true if the argument is an array, or false otherwise.. Array.of() Creates a new Array instance with a variable number of arguments, regardless of number or type of the arguments.
Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more. Jul 20, 2021 - Ways of iterating over a array in JavaScript. How to ignore loop in else condition using JavaScript ? ... In JavaScript, we can check if a variable is an array by using 3 methods, using the isArray method, using the instanceof operator and using checking the constructor type if it matches an ... The indexOf () method searches an array for a specified item and returns its position. The search will start at the specified position (at 0 if no start position is specified), and end the search at the end of the array. indexOf () returns -1 if the item is not found.
Checking for Array of Objects using some () For a more versatile solution that works on other data types, you may want to use some instead. ".some ()": tests whether at least one element in the array passes the test implemented by the provided function. It returns a Boolean value. Nearest number in an array : Our problem is to find the nearest number in an array specific to a given number. For example, if our array is [1,5,10,15,20] and if the given number is 18, the output of the program should be 20 as this is the nearest number to 18 in this array. Again, if the number is 16, it will print 15.Note that we are checking in both ways to find the nearest number. Jan 09, 2021 - With ES6 JavaScript added the includes method natively to both the Array and String natives. The method returns true or false to indicate if the sub-string or element exists. While true or false may provide the answer you need, regular expressions can check if a sub-string exists with a more ...
11 ways to check for palindromes in JavaScript. Simon Høiberg. Follow. ... 'b' becomes 2, etc.), and then subtracting the position in the array from that number. The reason that I'm subtracting 96 is, that 'a' is the 97th character in the ASCII Table. There are multiple methods available to check if an array contains duplicate values in JavaScript. You can use the indexOf () method, the Set object, or iteration to identify repeated items in an array. Aug 26, 2020 - Summary: in this tutorial, you ... the JavaScript Array every() method. ... Sometimes, you need to test whether every element of an array satisfies a specified condition. Typically, you use a for loop to iterate all elements and check each individual element against the condition. Suppose that you have an array numbers with three ...
1) Using Array.isArray (variableName) method to check if a variable is an array The Array.isArray (variableName) returns true if the variableName is an array. Otherwise, it returns false. The Array.isArray () method is a recommended way to check if a variable is an array because it has good browser support. Dec 10, 2020 - This tutorial shows you how to determine whether a JavaScript array includes a given value. ... A typical setup in your app is a list of objects. Here, we’re using an array of users. The task is to check if a user with a given name exists in the list of users. JavaScript: Tips of the Day. empty slots. const numbers = [1, 2, 3]; numbers[10] = 11; console.log(numbers); When you set a value to an element in an array that exceeds the length of the array, JavaScript creates something called "empty slots". These actually have the value of undefined, but you will see something like: [1, 2, 3, 7 x empty, 11]
To check if an array contains a primitive value, you can use the array method like array.includes() The following example uses the array.includes()method to check if the colorsarray contains 'red': constcolors = ['red', 'green', 'blue']; Approach #1: Return the Largest Numbers in a Array With a For Loop. Here's my solution, with embedded comments to help you understand it: function largestOfFour (arr) { // Step 1. Create an array that will host the result of the 4 sub-arrays var largestNumber = [0,0,0,0]; // Step 2. Create the first FOR loop that will iterate through the ... The arr.find () method is used to get the value of the first element in the array that satisfies the provided condition. It checks all the elements of the array and whichever the first element satisfies the condition is going to print.
Maximum Size of the Array¶. The apply and spread methods had a limitation of 65536 which came from the limit of the maximum number of arguments. In 2019, the limit is the maximum size of the call stack, meaning that the maximum size for the numbers in case of apply and spread solutions is approximately 120000. You can use the reduce () method to find the sum of an array of numbers. The reduce () method executes the specified reducer function on each member of the array resulting in a single output value as in the following example: The 0 in is the default value. If default value is not supplied, the first element in the array will be used. Dec 07, 2020 - In this tutorial, we'll go over examples of how to check if an array includes/contains an element or value in JavaScript.
How To Check Array Is Empty Or Null In Javascript
Find Max And Min Value From An Array In Javascript Codekila
How To Check If Array Is Empty In Javascript Codekila
Array Check If Value Exists Javascript Code Example
Javascript Array Indexof And Lastindexof Locating An Element
Indexed Collections Javascript Mdn
Js Check If Value Exist In Array Code Example
How To Remove Commas From Array In Javascript
How To Get Last Element Of An Array In Javascript
The Fastest Way To Find Minimum And Maximum Values In An
Javascript Quiz Can In Operator Check Value Or Index In An
Check If An Array Is A Subset Of Another Array In Javascript
Better Array Check With Array Isarray
How To Filter Out Only Numbers In An Array Using Javascript
How To Check All Values Of An Array Are Equal Or Not In
How To Check If A Javascript Array Contains A Specific Value
How To Play With Arrays In Javascript Like A Pro Positronx Io
Javascript Basic Check Whether Two Arrays Of Integers Of
Javascript Array Splice Delete Insert And Replace
How To Check If An Object Is An Array In Javascript Stackhowto
How To Check If An Array Is Empty Or Not In Javascript
Check If An Array Contains Any Element Of Another Array In
Check If Value Exists Jquery In Array Jquery Inarray
Javascript Check Index Of Element In Array
Check If Javascript Array Is Empty Null Or Undefined In 4 Ways
How To Check If A Variable Is An Array In Javascript
3 How To Check Javascript Arrays Javascript Tutorial
Check And Fill In Vacancies Javascript Array Method
Check If A Value Exists In Array In Javascript Learn Simpli
0 Response to "30 Check Number In Array Javascript"
Post a Comment