31 Javascript Initialize 2d Array
Oct 17, 2017 - Instead, I would recommend that ... an array with zeros function calls (the inductive case). Though the resulting code will be more verbose, it's much less confusing. – Xavi Sep 11 '10 at 6:19 ... Late to the Party, but this Post is still high up in the Google search results. To create an empty 2D-Array with ... JavaScript has a buit in array constructor new Array (). But you can safely use [] instead. These two different statements both create a new empty array named points: const points = new Array ();
Top 50 Array Interview Questions Amp Answers 2021 Update
Example 1: js initialize 2d array let data = []; for (let row=0; row
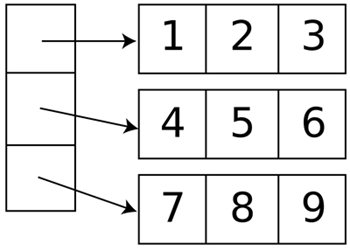
Javascript initialize 2d array. 17/7/2021 · JavaScript fundamental (ES6 Syntax): Exercise-53 with Solution. Write a JavaScript program to Initialize a two dimension array of given width and height and value. Use Array.from() and Array.prototype.map() to generate h rows where each is a new array of size w. Use Array.prototype.fill() to initialize all items with value val. you call the Array () constructor with two or more arguments, the arguments will create the array elements. If you only invoke one argument, the argument initializes the length of the new array; the new array's elements are not initialized. Apr 28, 2021 - Using the array constructor and the for-loop, creating a two-dimensional array in JavaScript is as simple as: ... The Array.from() method creates a new Array instance from the specified array and optionally map each array element to a new value. To create a 2D array, the idea is to map each ...
Mar 27, 2019 - Quora is a place to gain and share knowledge. It's a platform to ask questions and connect with people who contribute unique insights and quality answers. It's usually a good practice to start with an empty array, rather than filling w random values. (You normally declare array as const x = [] in 1D, so better to start w empty in 2D.) ... You can create a 2 Dimensional array m x n with initial value m and n can be any numbers v can be any value ... Now to initialize that array object, JavaScript provides three ways: First, Array declaration and initialization using JavaScript Array Literals. Second, it's declaration and initialization using JavaScript Array directly (new keyword). Third, it's declaration and initialization using JavaScript Array constructor (new keyword).
Now, let's create a couple of single-element arrays. Open up your JavaScript editor or IDE and type in the commands shown below: const fruits = ['apple' , 'orange' , 'banana']; simple array of fruits in JavaScript. Arrays are usually used when a particular category or variable will have a high number of different values. When we initialize an empty array using this notion var arr = [,,]; It initializes array with the number of elements equal to the number of comma used inside square brackets []. So in above case, length of the array will be 2 instead of 3. Feb 09, 2020 - Some languages, C#, Java and even Visual Basic, to name a few, let one declare multi-dimensional arrays. But not JavaScript. In JavaScript you have to build them. A two-dimensional array is a…
Aug 05, 2019 - Sometimes, you need to create and manipulate a two-dimensional (2D) array or a matrix. In JavaScript, an array of arrays can be used as a 2D array. 1234567const mat = [ [0, 1, 2, 3], [4, 5, 6, 7] Arrays too, like variables, should be declared before they are used. Array initialization refers to populating the array elements. Array element values can be updated or modified but cannot be deleted. Declaring and Initializing Arrays. To declare and initialize an array in JavaScript use the following syntax − If the Array () constructor is called with only a single parameter, and that parameter is an integer, JavaScript will initialize an array with the specified number of empty slots. Assuming you'd like to fill those slots with some default content, you can use the Array.fill () function to do so.
JavaScript program that uses array literal. // Initialize array with 3 elements. var values = [10, 20, 30] ; console.log ( "ARRAY: " + values); ARRAY: 10,20,30. Constructor. We can use the Array constructor with the new keyword in JavaScript. This is more complex. With one argument, we have a specified number of empty elements in our array. Sep 11, 2018 - Not the answer you're looking for? Browse other questions tagged javascript arrays dynamic 2d push or ask your own question. The two-dimensional array is a collection of items which share a common name and they are organized as a matrix in the form of rows and columns.The two-dimensional array is an array of arrays, so we create an array of one-dimensional array objects. The following program shows how to create an 2D array :
19/4/2015 · To create a 2D array in javaScript we can create an Array first and then add Arrays as it's elements. This method will return a 2D array with the given number of rows and columns. function Create2DArray(rows,columns) { var x = new Array(rows); for (var i = 0; i < rows; i++) { x[i] = new Array(columns); } return x; } In JavaScript, array is a single variable that is used to store different elements. It is often used when we want to store list of elements and access them by a single variable. Unlike most languages where array is a reference to the multiple variable, in JavaScript array is a single variable that stores multiple elements. Declaration of an Array Arrays in JavaScript can be created by using an array literal and Array constructor. Recommended Articles. This is a guide to Dynamic Array in JavaScript. Here we discuss how Array elements literate, how to declare, functions, a constructor with examples to implement. You can also go through our other related articles to learn more -
JavaScript program that uses array literal // Initialize array with 3 elements. var values = [10, 20, 30] ; console.log ( "ARRAY: " + values); Output ARRAY: 10,20,30. Constructor. We can use the Array constructor with the new keyword in JavaScript. This is more complex. With one argument, we have a specified number of empty elements in our array. Apr 28, 2021 - This post will discuss how to create a 2D array pre-filled with a specified value in JavaScript. To create a 2D array of fixed dimensions initialized with a specified value, you can use any of the following methods: A JavaScript array is initialized with the given elements, except in the case where a single argument is passed to the Array constructor and that argument is a number (see the arrayLength parameter below).
To append a new property in a 2D array, we used JavaScript array forEach() method to iterate the inner array one by one and add the percentage to the array. When we see in the table, it will become a column of the table. Removing elements from the JavaScript 2D array. To remove an item from an array, you use the array pop() or array splice ... May 24, 2020 - Get code examples like "javascript initialize array of arrays" instantly right from your google search results with the Grepper Chrome Extension. JavaScript Arrays - Arrays are used to store multiple elements in a single variable. Examples : prime numbers, names of students in a class, etc. Array also provides methods that help in accessing or transforming or applying functions on elements of array. Syntax to initialize array and examples are provided.
An array in JavaScript can be defined and initialized in two ways, array literal and Array constructor syntax. However, we can create a multidimensional array in JavaScript by making an array of arrays i.e. the array will be consisting of other arrays as elements. The easiest way to define a Multi-Dimensional Array in JavaScript is to use the array literal notation. Below examples will create a 2-dimensional array person. var Employee = [ Array.filter () The filter () method creates a new array with array elements that passes a test. This example creates a new array from elements with a value larger than 18: Example. const numbers = [45, 4, 9, 16, 25]; const over18 = numbers.filter(myFunction); function myFunction (value, index, array) {.
The first parameter (2) defines the position where new elements should be added (spliced in). The second parameter (0) defines how many elements should be removed. The rest of the parameters ("Lemon" , "Kiwi") define the new elements to be added. The splice () method returns an array with the deleted items: Jul 28, 2021 - (let [rows (Integer/parseInt ... (to-array-2d (repeat rows (repeat cols nil)))] (aset a 0 0 12) (println "Element at 0,0:" (aget a 0 0))) ... (let ((d1 (read)) (d2 (read))) (assert (and (typep d1 '(integer 1)) (typep d2 '(integer 1))) (d1 d2)) (let ((array (make-array (list d1 d2) :initial-element nil)) ... Initialization. 1. Sized Array. C++ gives us the opportunity to initialize array at the time of declaration. For the arrays with specified size we initialize values as follows. For a 2-Dimensional integer array, initialization can be done by putting values in curly braces " {" and "}".
How to Create a Two Dimensional Array in JavaScript The two-dimensional array is a set of items sharing the same name. The two-dimensional array is an array of arrays, that is to say, to create an array of one-dimensional array objects. They are arranged as a matrix in the form of rows and columns. Creating and Initializing Arrays In Javascript Multidimensional Arrays in Javascript Iterating Through Arrays in Javascript Javascript Array Sort: Sorting Arrays in Javascript Pop and Shift: Removing Elements From Arrays in Javascript Javascript Slice: Selecting Part of an Array Javascript Array Concat: Adding One Array To Another Javascript Join: Joining Elements of an Array You can find the definition of the Array constructor here - Array (MDN). If you need 2D arrays often, you can write a function that initializes them for you: var init2DArray = function (xlen, ylen, factoryFn) { var ret = []
Click here to learn how to create two dimensional arrays in JavaScript. Jul 06, 2016 - I assumed that var row_major = ... needed a 2D array today and found that it doesn't. Damn. – Mark K Cowan Jun 11 '14 at 18:51 ... Yes you can create an empty array and then push data into it. There is no need to define the length first in JavaScript.... Get code examples like "javascript initialize 2d array " instantly right from your google search results with the Grepper Chrome Extension.
Cloning Arrays: The Challenge. In JavaScript, arrays and objects are reference types. This means that when a variable is assigned an array or object, what gets assigned to the variable is a reference to the location in memory where the array or object was stored. Arrays, just like every other object in JavaScript, are reference types. Multidimensional arrays are not directly provided in JavaScript. If we want to use anything which acts as a multidimensional array then we need to create a multidimensional array by using another one-dimensional array. So multidimensional arrays in JavaScript is known as arrays inside another array. Introduction to JavaScript multidimensional array. JavaScript does not provide the multidimensional array natively. However, you can create a multidimensional array by defining an array of elements, where each element is also another array. For this reason, we can say that a JavaScript multidimensional array is an array of arrays.
24/4/2018 · I would like to create a two-dimensional array that gets initialized with booleans which are set to false. Currently I'm using this method of array creation: const rows = 3 const cols = 5 const nestedArray = new Array (rows).fill ( new Array (cols).fill (false) ) The nestedArray looks fine, but as soon as I change the value of nestedArray [0] ... JavaScript arrays are zero-indexed. The first element of an array is at index 0, and the last element is at the index value equal to the value of the array's length property minus 1. Using an invalid index number returns undefined. Technically, there is no two-dimensional array in JavaScript. JavaScript supports 2D arrays through jagged arrays - array of arrays. Jagged arrays are essentially multiple arrays jagged together to form a multidimensional array. A two-dimensional array in JavaScript is a jagged array of multiple one-dimensional arrays.
Given a string S and a character C, return an array of integers representing the shortest distance from the character C in the string. javascript ... Write a recursive function flattenRecursively that flattens a nested array. Your function should be able to handle varying levels of nesting.
Create 2d Array Javascript Code Example
Different Ways To Declare And Initialize 2 D Array In Java
Two Dimensional Array In C Programming
Two Dimensional Arrays Processing Org
2d Arrays In Javascript Learn How To Create 2d Arrays In
Python Using 2d Arrays Lists The Right Way Geeksforgeeks
Javascript Pitfalls Amp Tips 2d Array Matrix Sanori S Blog
2d Arrays In C How To Declare Initialize And Access
How Can I Create A Two Dimensional Array In Javascript
Javascript Two Dimensional Array Code Example
Filter 2d Array Javascript Code Example
Convert Object To 2d Array In Javascript Stack Overflow
How To Return Multidimensional Array Using Two Parameters In
Two Dimensional Arrays Processing Org
Javascript Push And Read From Multidimensional Array Stack
A Newb S Request For Help 2d Array Ask For Help
Two Dimensional Array Matrix Svet Programiranja
Convert 2d Array To 1d Array In C
2d Array Initialization Help Uipath Community Forum
Multi Dimensional Arrays In C C 2d Amp 3d Arrays Unveil
Declare And Initialize 2d Array In Java Devcubicle
C Multidimensional Arrays 2nd And 3d Arrays
Two Dimensional Array Javascript Code Example
Java67 How To Declare And Initialize Two Dimensional Array
Two Dimensional Array Javascript Example
C Pointers And Two Dimensional Array C Programming
Learning Multidimensional Array Addition In Javascript By
0 Response to "31 Javascript Initialize 2d Array"
Post a Comment