32 Concatenation Of String In Javascript
In JavaScript, concat () is a string method that is used to concatenate strings together. The concat () method appends one or more string values to the calling string and then returns the concatenated result as a new string. In JavaScript, concatenation is most commonly used to join variable values together, or strings with other strings, to form longer constructions. Somewhat confusingly, the most common JavaScript concatenation operator is +, the same as the addition operator.
Javascript Multiline String Using The Concatenation Of
JavaScript's join () method is handy for turning elements in an array into a string. JavaScript arrays can contain values of different types. If you only want to concatenate strings, you can filter out non-string values using filter () and typeof as shown below.
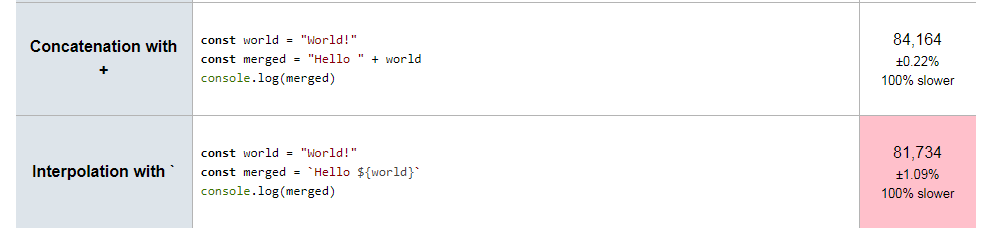
Concatenation of string in javascript. String Interpolation using Backticks. We can use the backtick `` (found next to Z or 1) on your keyboard instead of quotes to concatenate strings as seen here: const firstName = "Paul" const lastName = "Halliday" const greeting = `Hello, my name is $ {firstName} $ {lastName}`. Inside of our backticks we're using the $ {expression} syntax, where ... JavaScript String Literals, Objects, Concatenation, Comparison. Here you will learn what string is, how to create, compare and concatenate strings in JavaScript. String is a primitive data type in JavaScript. A string is textual content. It must be enclosed in single or double quotation marks. The concat () method joins two or more strings. concat () does not change the existing strings, but returns a new string.
Extracting String Parts. The methods listed below are used for extracting a piece of a string: slice (start, end) substring (start, end) substr (start, length) JavaScript slice () is used to extract a piece of string and return it as a new string. There are two parameters: a start position index and an end one. I showed a few examples of using the different ways of concatenating strings. Which way is better all depends on the situation. When it comes to stylistic preference, I like to follow Airbnb Style guide. When programmatically building up strings, use template strings instead of concatenation. eslint: prefer-template template-curly-spacing JavaScript String concat() Method. The JavaScript concat() method combines two or more strings. concat() accepts as many parameters as you want, each one representing a string to merge. The concat() method is an alternative to the concatenation operator. Here's the syntax for the JavaScript concat() string function:
Other ways to concatenate string values in JavaScript include: Using the + operator. Using the ES6 template literal. According to the performance test between the assignment operators and the string concat () method, it is strongly recommended that the assignment operators (+, +=) are used instead of the concat () method. Conclusion. The concat ... Dec 11, 2019 · 2 min read In JavaScript, we can assign strings to a variable and use concatenation to combine the variable to another string. To concatenate a string, you add a plus sign+ between... Concatenate strings in Javascript with a separator. Earlier, when we used the join() and concat() methods, we found out that the resulting string has a separator with every value. The separator was a comma that is the default separator of these two methods. But while concatenating strings using the (+) sign, we should use some kind of ...
Plan A for string concatenation: using the + operator. string1 + string2. If you ask a Javascript programmer how to concatenate a string, this is likely the method he’ll respond with. It simply uses the + operator to concatenate the right operand with the left operand. This method used to be fairly slow, but has recently been optimized enough ... Being able to concatenate(join) two or more strings together is pretty useful - you may be combining user input for storage in a single databasecolumn or doing the reverse - combining data from multiple database columns into a single string for display or output. JavaScript String object has a built-in concat () method. As the name suggests, this method joins or merges two or more strings. The concat () method doesn't modify the string in place. Instead, it creates and returns a new string containing the text of the joined strings.
Concatenation, or joining strings, is an important facility within any programming language. It's especially vital within web applications, since strings are regularly used to generate HTML output.... I know that I've had to look this up in the past because every language is a little different with its built-in functions. concat () is basically an included function with JavaScript strings that takes in as many arguments as you want and appends — or concatenates — them. The official syntax, as described by MDN Web Docs, is as follows: str.concat() function is used to join two or more strings together in JavaScript. Syntax: str.concat(string2, string3, string4,....., stringN) Arguments: The arguments to this function are the strings that need to be joined together. The number of arguments to this function is equal to the number of strings to be joined together. Return value:
Concatenation is the operation that forms the basis of joining two strings. Merging strings is an inevitable aspect of programming. Before getting into "String Concatenation in JavaScript", we need to clear out the basics first. When an interpreter executes the operation, a new string is created. Code language: JavaScript (javascript) The concat () accepts a varied number of string arguments and returns a new string containing the combined string arguments. If you pass non-string arguments into the concat (), it will convert these arguments to a string before concatenating. javascript string concatenation . javascript by Exuberant Elk on May 14 2020 Comment . 1. Source: www.techonthenet . Add a Grepper Answer . Javascript answers related to "how to concatenate string and variable in javascript" javascript concat; string concatenation js ...
In JavaScript, there are three ways you can create multi-line strings: Using new line characters (\n). Using string concatenation. Using template string literals. In this guide, we're going to talk about how to use each of these three approaches to write a multi-line string. We'll walk through examples of each approach so you can easily get ... 15/10/2020 · We are required to write a JavaScript function that takes in two strings and concatenates the second string to the first string. If the last character of the first string and the first character of the second string are the same then we have to omit one of those characters. It can be used to concatenate strings too in JavaScript. Syntax: let str = 'Hello' ; str += ' ' ; str += 'World' ; //Output: Hello World. One can also concat strings using the + operator using the shorthand += operator. let str = 'Hello' ; str += ' ' ; str += 'World' ; //Output: Hello World.
The concat () function concatenates the string arguments to the calling string and returns a new string. Changes to the original string or the returned string don't affect the other. If the arguments are not of the type string, they are converted to string values before concatenating. Next, we'll turn our attention to strings — this is what pieces of text are called in programming. In this article, we'll look at all the common things that you really ought to know about strings when learning JavaScript, such as creating strings, escaping quotes in strings, and joining strings together. Introduction to the JavaScript String concat () method. The String.prototype.concat () method accepts a list of strings and returns a new string that contains the combined strings: If the arguments are not strings, the concat () converts them to strings before carrying the concatenation. It's recommended that you use the + or += operator for ...
19/4/2019 · String concatenation “+” operator. In Javascript, you can do that in a multitude of ways, and one of them is by using the String concatenation operator, or the “+” operator. Now, this “+” operator, if used with numbers, like this: var a = 1 + 2; that would just add these two numbers and give out 3 as the output. JavaScript String concat() Method. The JavaScript string concat() method combines two or more strings and returns a new string. This method doesn't make any change in the original string. Syntax. The concat() method is represented by the following syntax: 11/10/2013 · 1. Build up the entire document into an array, then join with a "\n"at the end. (The rationale for this is of course to not have lots of new lines scattered all about! And if you are on IE7 or less, Array#joinis considerably faster than repeated string concatenation.) Code here: http://jsfiddle /ZCbCZ/.
29/7/2019 · String#concat () JavaScript strings have a built-in concat () method. The concat () function takes one or more parameters, and returns the modified string. Strings in JavaScript are immutable, so concat () doesn't modify the string in place. const str1 = 'Hello'; const str2 = …
Why Should We Use Concatenation Instead Of Just The Print
Just A Few Things 100daysofcode D29 Basic Javascript
Javascript Performance Tips Amp Tricks Modus Create
Java Exercises Append Two Given Strings Such That If The
Javascript String Concatenation Qandeel Academy
C Program To Concatenate Two Strings Without Using Strcat
How To Concatenate Two Strings Using Concat Method In Java
Strings In Powershell Replace Compare Concatenate Split
Javascript Operators Top 7 Types That You Can T Omit While
Javascript Concat String How Does Concat Function Work In
Java Quiz On String Concatenation Top Java Tutorial
Are Backticks Slower Than Other Strings In Javascript
4 Ways To Concatenate Strings In Javascript
Concatenating Joining Strings In Javascript 3 Methods
Javascript Chapter 10 Strings And Arrays
C Program For String Concatenation Studytonight
How To Do Text Concatenation In Storyline Javascript Light
Javascript Lesson 3 Strings In Javascript Geeksread
The Optimum Method To Concatenate Strings In Java Java Code
How To Create Multi Line Strings In Javascript Geeksforgeeks
String Concat Inefficient Code Stack Overflow
Java String Concatenation Which Way Is Best By Nicholas
Jquery Html Javascript String Concatenation Onclick Event In
5 Ways To Convert A Value To String In Javascript By
C Program To Concatenate Two Strings Without Using Strcat
Php Echo Concatenate String And Variable Code Example
0 Response to "32 Concatenation Of String In Javascript"
Post a Comment