35 Map To An Object Javascript
Aug 17, 2015 - When you have key-value data whose keys you don’t know in advance, it’s generally better to store it in an ES6 Map than in an object. But how do you convert Maps to and from JSON? This blog post tells you. Doing a spread operator in the loop {...main, [key]: value} is as inefficient as Object.assign() in the OP. In contrast; obj[key] = value is simply modifying the reducer sum value, rather than recreating it on every loop. The ES6 spread operator is indeed faster than Array.from(), but is still slower than the for of loop.. TL:DR; crude results below at 100,000 iterations of a 15 element map.
Json Generated By Javascript Code Returns Arrays As Objects
Map vs Object in JavaScript. Map is a data structure which helps in storing the data in the form of pairs. The pair consists of a unique key and a value mapped to the key. It helps prevent duplicity. Object follows the same concept as that of map i.e. using key-value pair for storing data. But there are slight differences which makes map a ...
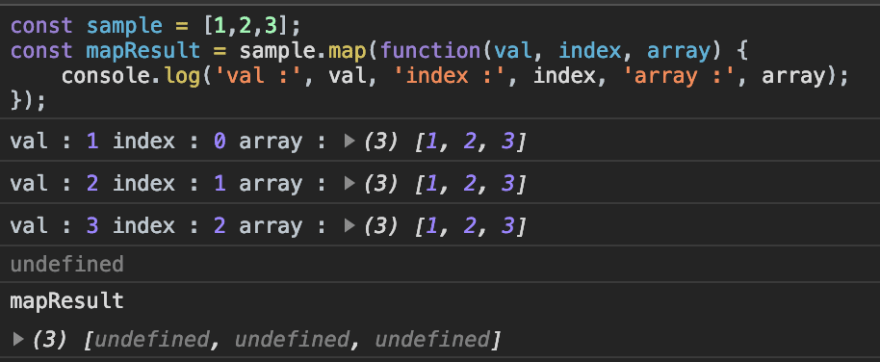
Map to an object javascript. Introduction to JavaScript Map Object. The JavaScript map object is a type of collection that allows storing values based on key-value pair terminology. The map object is introduced in the ES6 specification of JavaScript and it allows storing the pairs of keys and respective values in it. i'm trying to convert a Map into array of object Lets say I have the following map: let myMap = new Map().set('a', 1).set('b', 2); And I want to convert the above map into the following: [ {... Stack Overflow. ... Find object by id in an array of JavaScript objects. 5111. For-each over an array in JavaScript. Your post isn't clear, but I think you're trying to just merge two objects into one. This can be done with the es6 spread operator.Here is the MDN docs for the spread operator.. Here is an example I wrote to show how you can use the spread operator to merge two objects and then log the key/values of the merged object:. let object1 = { firstKey: 'firstValue', secondKey: 'secondValue' }; let ...
Apr 28, 2019 - Here's a function that converts object to map data type. There is one important thing to note about using an Object or Array as a key: the Map is using the reference to the Object to compare equality, not the literal value of the Object. In JavaScript {} === {} returns false, because the two Objects are not the same two Objects, despite having the same (empty) value. Map is a collection of elements where each element is stored as a Key, value pair. Map object can hold both objects and primitive values as either key or value. When we iterate over the map object it returns the key,value pair in the same order as inserted.
In JavaScript, objects are used to store multiple values as a complex data structure. An object is created with curly braces {…} and a list of properties. A property is a key-value pair where the key must be a string and the value can be of any type.. On the other hand, arrays are an ordered collection that can hold data of any type. In JavaScript, arrays are created with square brackets ... Map is a data structure in JavaScript which allows storing of [key, value] pairs where any value can be either used as a key or value. The keys and values in the map collection may be of any type and if a value is added to the map collection using a key which already exists in the collection, then the new value replaces the old value. Introduction to JavaScript Map object Prior to ES6, when you need to map keys to values, you often use an object, because an object allows you to map a key to a value of any type. However, using an object as a map has some side effects: An object always has a default key like the prototype.
Sep 15, 2020 - We are required to write a JavaScript function that takes in such an object with key value pairs and converts it into a Map. Dec 02, 2020 - On the other hand, Objects and Arrays have been traditionally used for data storage and manipulation in JavaScript, and have direct compatibility with JSON, which continues to make them the most essential data structures, especially for working with REST APIs. Maps and Sets are primarily useful ... Jan 30, 2020 - Summary: in this tutorial, you will learn about the JavaScript Map object that maps a key to a value. ... Prior to ES6, when you need to map keys to values, you often use an object, because an object allows you to map a key to a value of any type.
In order to add a new property to every object from the array of objects, the transformed object with a new property value has to be returned in the Array.map () callback function. We use the JavaScript spread operator to include all existing object property values along with the new properties that need to be added to object entries. JavaScript map with an array of objects JavaScript map method is used to call a function on each element of an array to create a different array based on the outputs of the function. It creates a new array without modifying the elements of the original array. So Ive got the following javascript which contains a key/value pair to map a nested path to a directory. function createPaths(aliases, propName, path) { aliases.set(propName, path); } map = n...
JavaScript Map Objects. A Map holds key-value pairs where the keys can be any datatype. A Map remembers the original insertion order of the keys. A Map has a property that represents the size of the map. Being able to use objects as keys is an important Map feature. Map vs regular Objects. Earlier I mentioned that Map are a key/value pair data structure. The same can be said for regular objects in JavaScript, but there are some differences between the two ...
If you want to alter the original objects, then a simple Array#forEach will do: rockets.forEach(function(rocket) { rocket.launches += 10; }); If you want to keep the original objects unaltered, then use Array#map and copy the objects using Object#assign: Map The Map object holds key-value pairs and remembers the original insertion order of the keys. Any value (both objects and primitive values) may be used as either a key or a value. Definition and Usage. The map() method creates a new array with the results of calling a function for every array element.. The map() method calls the provided function once for each element in an array, in order.. map() does not execute the function for empty elements. map() does not change the original array.
Create a JavaScript Object From a Map Modern JavaScript engines ship with the Object.fromEntries () method. Creating an object from entries is what you're looking for when creating a plain object from a map instance. Here's an example transforming a map of users to an object: Nov 09, 2019 - This post describes the use cases when it’s better to use maps instead of plain objects. ... As presented above, if the object’s key is not a string or symbol, JavaScript implicitly transforms it into a string. Contrary, the map accepts keys of any type: strings, numbers, boolean, symbols. Everything in JavaScript is an object, and methods are functions attached to these objects..call () allows you to use the context of one object on another. Therefore, you would be copying the context of.map () in an array over to a string.
The map () method creates a new array populated with the results of calling a provided function on every element in the calling array. map [key] isn't the right way to use a Map Although map [key] also works, e.g. we can set map [key] = 2, this is treating map as a plain JavaScript object, so it implies all corresponding limitations (only string/symbol keys and so on). So we should use map methods: set, get and so on. Map can also use objects as keys. JSON is a javascript object notation for the format of key and values enclosed in braces. This code works in Javascript as this uses Latest Es6 classes and syntax. How to Convert Map to JSON object examples There are multiple ways we can do this. Convert Map into JSON object using Iterate and Stringify method. First
The Object.fromEntries() method takes a list of key-value pairs and returns a new object whose properties are given by those entries. The iterable argument is expected to be an object that implements an @@iterator method, that returns an iterator object, that produces a two element array-like object, whose first element is a value that will be used as a property key, and whose second element ... JavaScript Map Object Map in JavaScript is a collection of keyed data elements, just like an Object. But the main difference is that the Map allows keys of any type. A Map object iterates its items in insertion order and using the for…of loop, and it returns an array of [key, value] for each iteration. Jul 27, 2021 - Object.entries(obj) – returns an array of [key, value] pairs. Please note the distinctions (compared to map for example):
String Maps as JSON via objects # Whenever a Map only has strings as keys, you can convert it to JSON by encoding it as an object. Converting a string Map to and from an object # The following two function convert string Maps to and from objects: function strMapToObj (strMap) { let obj = Object.create(null); for (let [k,v] of strMap) { // We ... Jun 09, 2021 - Javascript - Different ways to convert Object to Map · In this blog post, We are going to learn How to convert an object to Map with examples in javascript . 1 week ago - The new Map() constructor accepts an iterable of entries. With Object.entries, you can easily convert from Object to Map:
Map Vs Set Vs Object In Javascript By Osgood Gunawan Medium
Useful Javascript Array And Object Methods By Robert Cooper
Array Vs Set Vs Map Vs Object Real Time Use Cases In
Master Modern Javascript Array Includes Array Reduce Map
Implementing Our Own Array Map Method In Javascript Dev
Javascript Map Object Examples Of Javascript Map Object
Javascript Tracking Key Value Pairs Using Hashmaps By
How To Use The Ruby Map Method With Examples Rubyguides
Javascript Map Data Structure With Examples Dot Net Tutorials
Tomek Sulkowski On Twitter Javascript Tip For Today When
Javascript Map Distinct Value From Object Code Example
Remove Object From An Array Of Objects In Javascript
Understanding Maps In Javascript Codeproject
Javascript Map Object Examples Of Javascript Map Object
The Map Guy De Making Immutable Js Objects Easier To Work
Map In Javascript Geeksforgeeks
Javascript Array Map Method Geeksforgeeks
Javascript Quiz Find Out The Size Of A Javascript Map
Script Based Object Map Feature For Squish Gui Tester Froglogic
Javascript Array Map Return Object Code Example
Deep Dive Into Javascript Map Object By Eric Sarpong
Es6 Map Vs Object What And When By Maya Shavin
How To Use Javascript Collections Map And Set
What Is The Difference Between Javascript Map And Object
Github Thinkloop Map Or Similar A Javascript Js Map Or
Map Dictionaries In Javascript When To Use Map Vs Object
Javascript Map Data Structure With Examples Dot Net Tutorials
Introduction To Javascript Source Maps Html5 Rocks
Javascript Engine Fundamentals Shapes And Inline Caches
Joel Thoms Javascript On Twitter Property Keys Vs
A Map Of Every Object In Our Solar System Visual Capitalist
Javascript Tracking Key Value Pairs Using Hashmaps By
0 Response to "35 Map To An Object Javascript"
Post a Comment