29 Encryption Algorithms In Javascript
A javascript library for asymmetric data encryption A javascript library for symmetric data encryption (in case our message/data is quite long) The crypto packages in the go standard library... javascript At both ends, we have to take same encryption and decryption algorithm. Here we are using AES (Advanced encryption standard) algorithm. When we required plain text send as encrypt text with a secret key. sometimes it becomes challenging to decrypt an encrypted text to the cross-platform environment.
How Javascript Works Cryptography How To Deal With Man In
Before starting this article you can also visit the previous article Encrypt in JavaScript and Decrypt in C# With AES Algorithm in ASP.Net MVC. What is AES Algorithm ? Advanced Encryption Standard (AES) is a symmetric encryption algorithm.The algorithm was developed by two Belgian cryptographers, Joan Daemen and Vincent Rijmen.
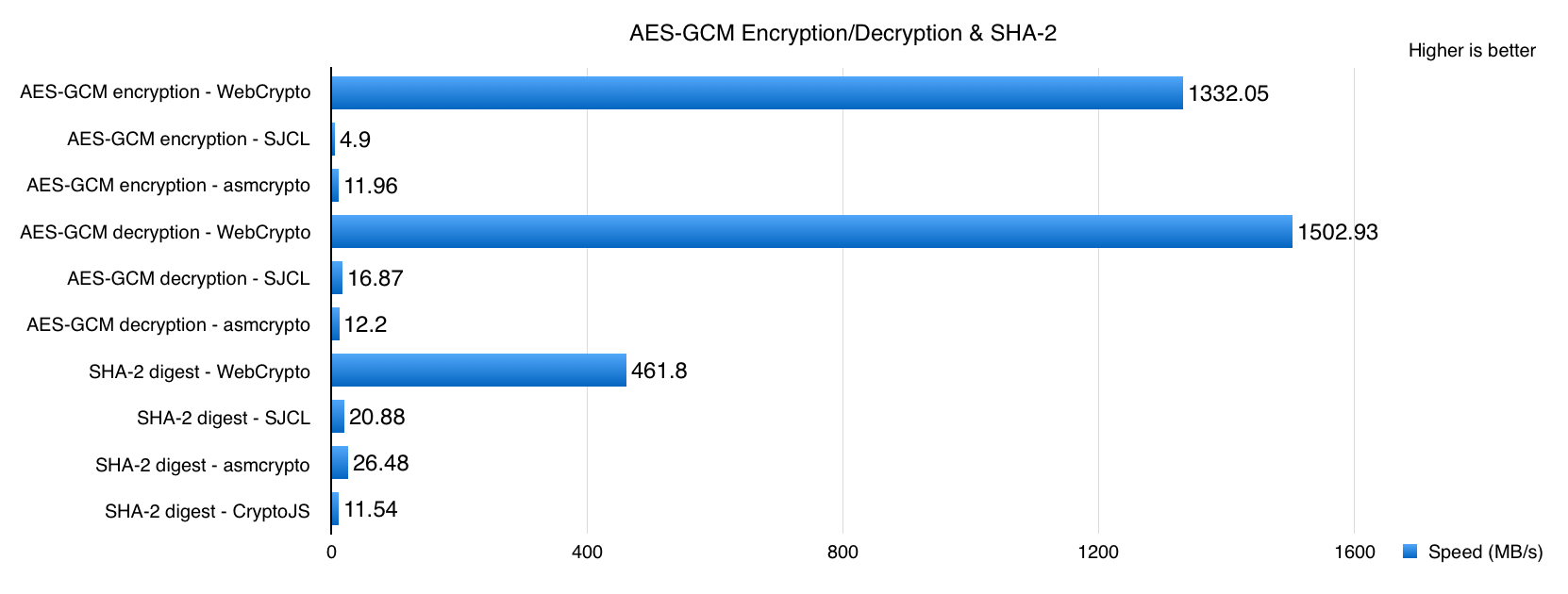
Encryption algorithms in javascript. For AES encryption in javascript we have imported two js files - crypto.js and pbkdf2.js.We have AesUtil.js that has common codes to perform encryption and decryption. Here this.keySize is the size of the key in 4-byte blocks.Hence, to use a 128-bit key, we have divided the number of bits by 32 to get the key size used for CryptoJS. Having not used an AES JavaScript implementation before I will provide Javascript AES encryption. I felt, 'JSAES is a powerful implementation of AES in JavaScript' seemed like a simple option. However, this will need to be your decision. A customizable encryption scheme for transmitting confidential information via a non-secure channel. It uses Steganography techniques to encode the secret data into an image or video file and can be used multiple times to make it a layered encryption and decrease the risk of being discovered. python encryption steganography encryption-algorithm ...
We are required to write a JavaScript function that takes in a string and encrypts it based on the following algorithm − The string contains only space separated words. We need to encrypt each word in the string using the following rules − The first letter needs to be converted to its ASCII code. Moreover I need an encryption system that can prevent anyone (WAF and admins) from reading the content of the requests and responses I send to the malware. That's why it led me to use Javascript encryption. In this article I do not describe the malware itself. This article sections are : The encryption algorithm I chose The cryptographic functions provided by the Web Crypto API can be performed by one or more different cryptographic algorithms: the algorithm argument to the function indicates which algorithm to use. Some algorithms need extra parameters: in these cases the algorithm argument is a dictionary object that includes the extra parameters.. The table below summarizes which algorithms are suitable ...
A lightweight 128-bit encryption algorithm implemented in C++ and JavaScript - GitHub - hutorny/chaskey: A lightweight 128-bit encryption algorithm implemented in C++ and JavaScript Client-server encryption-decryption using Advanced Encryption Algorithm (AES) in client and server is complicated because exactly the same algorithm must be implemented twice: once for client side in JavaScript and once for server side in PHP,C# etc. Named after its creators, the Rivest-Sharmir-Adleman (RSA) is established as the standard public-key encryption algorithm. It is asymmetric because it has a public and a private key that encrypts data being sent and received. Its scrambling level takes far too much time for any attackers to break and keeps communication quite secure.
JavaScript is fast-growing scripting language in browsers, servers (node.js), and databases. So encryption of data using JavaScript is important. Crypto-JS supports AES-128, AES-192, and AES-256. It will pick the variant by the size of the key you pass in. Crypto-JS also support other Hasher Algorithms MD, SHA-1, and SHA-2. AES Algorithm. The Advanced Encryption Standard (AES) is a symmetric encryption algorithm. The algorithm was developed by the two Belgian cryptographers Joan Daemen and Vincent Rijmen. AES was designed to be efficient in both hardware and software and supports a block length of 128 bits and key lengths of 128, 192 and 256 bits. The Stanford Javascript Crypto Library (hosted here on GitHub) is a project by the Stanford Computer Security Lab to build a secure, powerful, fast, small, easy-to-use, cross-browser library for cryptography in Javascript.. SJCL is easy to use: simply run sjcl.encrypt("password", "data") to encrypt data, or sjcl.decrypt("password", "encrypted-data") to decrypt it.
The Advanced Encryption Standard (AES, Rijndael) is a block cipher encryption and decryption algorithm, the most used encryption algorithm in the worldwide. The AES processes block of 128 bits using a secret key of 128, 192, or 256 bits. This article shows you a few of Java AES encryption and decryption examples: Interoperable AES encryption with Java and JavaScript. AES implementations are available in many languages, including Java and JavaScript. In Java, the javax.crypto.* packages are part of the standard, and in JavaScript, the excellent CryptoJS provides an implementation for many cryptographic algorithms. However, due to different default settings and various implementation details, it is not ... The System.Security.Cryptography has a bunch of symetric (and asymetric) encrytion algorithms ready to use. (For something super secure use aes) You should be able to find matching Javascript implementation for most (here are a few aes implementations in JS)
JSON Web Encryption is a Foot-Gun. Encryption leaves a lot of room for potential implementation errors, especially when asymmetric (a.k.a. public-key) encryption is involved. The encryption algorithms permitted by JWE are spelled out in RFC 7518, which comes in two sections: Key encryption, which gives you options such as: RSA with PKCS #1v1.5 ... algorithm is an object specifying the algorithm to be used, and any extra parameters as required. The values given for the extra parameters must match those passed into the corresponding encrypt() call. To use RSA-OAEP, pass an RsaOaepParams object. To use AES-CTR, pass an AesCtrParams object. To use AES-CBC, pass an AesCbcParams object. The Web Crypto API provides four algorithms that support the encrypt() and decrypt() operations.. One of these algorithms — RSA-OAEP — is a public-key cryptosystem.. The other three encryption algorithms here are all symmetric algorithms, and they're all based on the same underlying cipher, AES (Advanced Encryption Standard).The difference between them is the mode.
I would like to know if it is possible to encrypt and decrypt a text, using pure JavaScript. I don't want to use a key. It may be an entry lever solution. But I simply want to encode a text "my-name-1" into some text format and want to retrieve the text from it. Is this possible, without using any js libraries? A simple algorithm works with & play with an ASCII value of characters. Algorithm works within the following six different steps. Step 1: Convert input string characters in respected ASCII codes & store it in an array like the following example of JavaScript code. Using encrypt() and decrypt() To use SimpleCrypto, first create a SimpleCrypto instance with a secret key (password). Secret key parameter MUST be defined when creating a SimpleCrypto instance. To encrypt and decrypt data, simply use encrypt() and decrypt() function from an instance. This will use AES-CBC encryption algorithm.
Crypto-JS is a growing collection of standard and secure cryptographic algorithms implemented in JavaScript using best practices and patterns. They are fast, and they have a consistent and simple interface. Algorithms: MD5, SHA-1, SHA-256, AES, DES, Rabbit, MARC4, HMAC (HMAC-MD5, HMAC-SHA1, HMAC-SHA256) PBKDF2. Stanford - info. A pure JavaScript implementation of the AES block cipher algorithm and all common modes of operation (CBC, CFB, CTR, ECB and OFB). Features. Pure JavaScript (with no dependencies) Supports all key sizes (128-bit, 192-bit and 256-bit) Supports all common modes of operation (CBC, CFB, CTR, ECB and OFB) Works in either node.js or web browsers AES Implementations have been validated as conforming to the Advanced Encryption Standard (AES) Algorithm, as specified in Federal Information Processing Standard Publication 197, Advanced Encryption Standard, using the tests found in the Advanced Encryption Standard Algorithm Validation Suite (AESAVS). CCM Implementations have been validated as conforming to the Counter with Cipher Block ...
Encryption in cryptography is a process by which a plain text or a piece of information is converted into cipher text or a text which can only be decoded by the receiver for whom the information was intended. The algorithm that is used for the process of encryption is known as cipher. It helps in protecting consumer information, emails and other sensitive data from unauthorized access to it as ... AES Encryption in JavaScript Nodejs provides a crypto module for working with cryptographic functionalities like OpenSSL's hash, cipher, decipher, sign, verify and HMAC functions. There are many...
Hashing In Action Understanding Bcrypt
Encrypt In Javascript And Decrypt In C With Aes Algorithm
Symmetry Free Full Text A Secure And Efficient
How To Encrypt And Decrypt Files Using The Aes Encryption
How Javascript Works Cryptography How To Deal With Man In
Share Encryption And Decryption Methods And Examples Of Md5
Encryption And Hashing Loginradius Engineering
Types Of Encryption 5 Encryption Algorithms Amp How To Choose
Create Your Own Cipher Using Javascript By Nitin Manocha
Encryption Decryption Using Nodejs Node Js Nodejsera
Encrypt In Javascript And Decrypt In C With Aes Algorithm
Object Oriented Javascript Class Library In C Net Style
Implementing Rsa Encryption And Signing In Node Js With
An Introduction To Utilizing Public Key Cryptography In
Encrypt In Javascript And Decrypt In C With Aes Algorithm In
Javascript Object Signing Amp Encryption
Update On Web Cryptography Webkit
Client Side Field Level Encryption Mongodb Manual
Net Tripledes Encryption Not Matching In Javascript Cryptojs
How To Encrypt Decrypt With Crypto Js Stack Overflow
How To Encrypt Strings Amp Files In Your Source Code Dev
Implementing Rsa Encryption And Signing In Node Js With
Encrypt In Javascript And Decrypt In C With Aes Algorithm In
Github Paragonie Ciphersweet Js Searchable Encryption For
0 Response to "29 Encryption Algorithms In Javascript"
Post a Comment