22 Remove In Array Javascript
Example 2: Using Array.splice () // program to remove item from an array function removeItemFromArray(array, n) { const index = array.indexOf (n); // if the element is in the array, remove it if(index > -1) { // remove item array.splice (index, 1); } return array; } const result = removeItemFromArray ( [1, 2, 3 , 4, 5], 2); console.log (result); Remove elements from array using JavaScript filter - JavaScript. Javascript Web Development Front End Technology Object Oriented Programming. Suppose, we have two arrays of literals like these − ...
How To Remove First Element From Array In Javascript
If deleteCount is omitted, or if its value is equal to or larger than array.length - start (that is, if it is equal to or greater than the number of elements left in the array, starting at start), then all the elements from start to the end of the array will be deleted. If deleteCount is 0 or negative, no elements are removed.
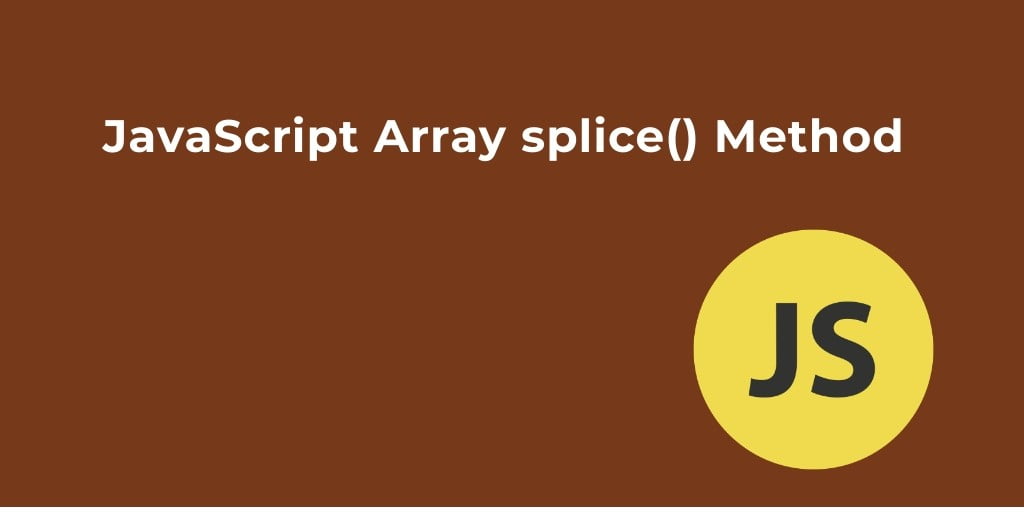
Remove in array javascript. The JavaScript Array class is a global object that is used in the construction of arrays; which are high-level, list-like objects. Description Arrays are list-like objects whose prototype has methods to perform traversal and mutation operations. To remove the first element of an array, we can use the built-in shift () method in JavaScript. Here is an example: const fruits = ["apple", "banana", "grapes"]; fruits.shift(); console.log(fruits); Note: The shift () method also returns the removed element. Similarly, we can also remove the first element of an array by using the splice ... There are many cases when it needs to remove some unused values from the Array which no longer needed within the program. Inbuilt functions are available in JavaScript to do this. Some functions auto-arrange the Array index after deletion and some just delete the value and not rearrange the index.
25/7/2018 · How to Remove an Element from an Array in JavaScript JavaScript suggests several methods to remove elements from existing Array . You can delete items from the end of an array using pop() , from the beginning using shift() , or from the middle using splice() functions. Use the splice () Method to Remove an Object From an Array in JavaScript The method splice () might be the best method out there that we can use to remove the object from an array. It changes the content of an array by removing or replacing existing elements or adding new elements in place. The syntax for the splice () method is shown below. JavaScript remove object from array. To remove an object from the array in Javascript, use one of the following methods. array.pop () - The pop () method removes from the end of an Array. array.splice () - The splice () method deletes from a specific Array index.
8 Ways to Remove Duplicate Array Values in JavaScript. 1. De-Dupe Arrays with Set Objects. Set objects objects are my go-to when de-duplicating arrays. Try out this example in your browser console, spoiler - Set objects work great for primitive types, but may not support use cases requiring the de-duplication of objects in an array. 1/1/2014 · JavaScript Array elements can be removed from the end of an array by setting the length property to a value less than the current value. Any element whose index is greater than or equal to the new length will be removed. var ar = [1, 2, 3, 4, 5, 6]; ar.length = 4; console.log( ar ); To remove duplicates from an array: First, convert an array of duplicates to a Set. The new Set will implicitly remove duplicate elements. Then, convert the set back to an array.
For code that would work in all browsers, you would have to manually find each element from b in a and remove it. var a = [1, 2, 3, 4, 5]; var b = [2, 3]; var result = [], found; for (var i = 0; i < a.length; i++) { found = false; // find a [i] in b for (var j = 0; j < b.length; j++) { if (a [i] == b [j]) { found = true; break; } } if ... Add and remove elements in arrays in Javascript - seems like a very easy topic and so it is but what I've always felt is that all the methods and things that JS provides us to accomplish this go under-appreciated at times. For that purpose, I decided to write this article about some of the best methods we can use to add and remove elements ... The splice () method enables us to delete the element from the given array. We can perform several other operations using this function which are deleting existing elements, insert new elements, and replace elements within an array. Using this method, we can delete and replace existing elements within the array.
Remove elements from array in JavaScript. An array is a variable used to store one or more elements of the same data type. Basically, it stores multiple elements of the same type. Sometimes we need to remove these elements from an array. JavaScript offers several built-in array methods to add or remove the elements from an array easily. Our goal is to remove all the falsy values from the array then return the array. The good people at freeCodeCamp have told us that falsy values in JavaScript are false, null, 0, "", undefined, and NaN. They have also dropped a major hint for us! They suggest converting each value of the array into a boolean in order to accomplish this challenge. We can remove an object with a specific key using the built-in filter method. arr = arr.filter((elem) => elem.id !== 0); This will remove all objects with an id of 0. It also creates a copy of the array and does not modify the original array.
The array.splice () method is used to add or remove items from an array. This method takes in 3 parameters, the index where the element's id is to be inserted or removed, the number of items to be deleted and the new items which are to be inserted. This method actually deletes the element at index and shifts the remaining elements leaving no ... Here are a few ways to remove an item from an array using JavaScript. All the method described do not mutate the original array, and instead create a new one. If you know the index of an item. Suppose you have an array, and you want to remove an item in position i. One method is to use slice(): The Array pop() method is used to delete the last element from the array and returns that element. The pop method changes the array on which it is requested, This means unlike using remove the last element is removed effectively and the array length reduced.
Use Array.filter () to Remove a Specific Element From JavaScript Array The filter methods loop through the array and filter out elements satisfying a specific given condition. We can use it to remove the target element and keep the rest of them. It helps us to remove multiple elements at the same time. Javascript remove a specific element from an array using splice Method In this example, we are taking a simple array as an example and the indexOf () and splice () method helps to remove specific element values in an array. so you can see the below example. There are different methods and techniques you can use to remove elements from JavaScript arrays: pop — Removes from the End of an Array shift — Removes from the beginning of an Array splice —...
20/5/2020 · JavaScript provides many ways to remove elements from an array. You can remove an item: By its numeric index. By its value. From the beginning and end of the array. Removing an element by index. If you already know the array element index, just use the Array.splice() method to remove it from the array. This method modifies the original array by removing or replacing existing elements and returns the removed elements … In the above program, Set is used to remove duplicate items from an array. A Set is a collection of unique values.. Here, The array is converted to Set and all the duplicate elements are automatically removed.; The spread syntax ... is used to include all the elements of the Set to a new array. To remove a particular element from an array in JavaScript we'll want to first find the location of the element and then remove it. Finding the location by value can be done with the indexOf() method, which returns the index for the first occurrence of the given value, or -1 if it is not in the array.
When you work with arrays, it is easy to remove elements and add new elements. This is what popping and pushing is: Popping items out of an array, or pushing items into an array. ... Since JavaScript arrays are objects, elements can be deleted by using the JavaScript operator delete: Example. Trong javascript có nhiều cách javascript remove element from array. Có nhiều cách ta đã biết từ lâu, nhưng cũng nên học thêm những cách khác như, remove item in array javascript es6, hay remove object in array javascript, hoặc có thể remove element from array by index or value. Nếu chúng ta biết trước index or value.
Arrays are Objects. Arrays are a special type of objects. The typeof operator in JavaScript returns "object" for arrays. But, JavaScript arrays are best described as arrays. Arrays use numbers to access its "elements". In this example, person [0] returns John:
How To Remove Duplicates From An Array Of Objects Using
How To Remove Element From Array Javascript Tech Dev Pillar
How To Add Remove And Replace Items Using Array Splice In
5 Easy Ways To Remove An Element From Javascript Array
9 Ways To Remove Elements From A Javascript Array
Javascript Remove The Last Item From An Array Geeksforgeeks
How To Remove A Specific Element From An Array In Javascript
Javascript Array Remove Duplicate Items From An Array
How To Remove An Element From A Javascript Array By Eniola
Remove Elements From A Javascript Array Geeksforgeeks
Javascript Array Remove Null 0 Blank False Undefined And
Remove Duplicate Id From Array Javascript Code Example
Remove First And Last Element From Array Javascript
Removing Empty Or Undefined Elements From An Array Stack
Manipulating Javascript Arrays Removing Keys By Adrian
How Can I Remove A Specific Item From An Array Stack Overflow
How To Remove Duplicates From Javascript Array Codekila
The Fastest Way To Remove A Specific Item From An Array In
Javascript Array Splice Remove Add And Replace Tuts Make
Remove Duplicate Objects From Array Javascript Code Example
Javascript Tutorial Removing A Specific Element From An
0 Response to "22 Remove In Array Javascript"
Post a Comment