35 How To Get Parent Element In Javascript
Get the parent of an object. To go further, we need to get the parent of the targeted object. In JavaScript, there's no clean and easy way to get the parent of a js nested object. When you are working with the DOM or an xml, you can target the parent node ; but if you try that with a Javascript object, this won't do anything. All HTML form element objects accessed via JavaScript (such as HTMLInputElement, HTMLTextAreaElement, HTMLButtonElement, etc.) have the property "form" which refers to their parent form element. To demonstrate this, let's assume we have the following HTML form:
Req Coding Page Transitions In Vanilla Javascript
The following code gets the parent node of the element with the id main: const current = document. querySelector ( '#main'); const parent = current. parentNode; How it works: First, select the element with the CSS selector #main. Then, use the parentNode property to get the parent element of the selected element. Source.
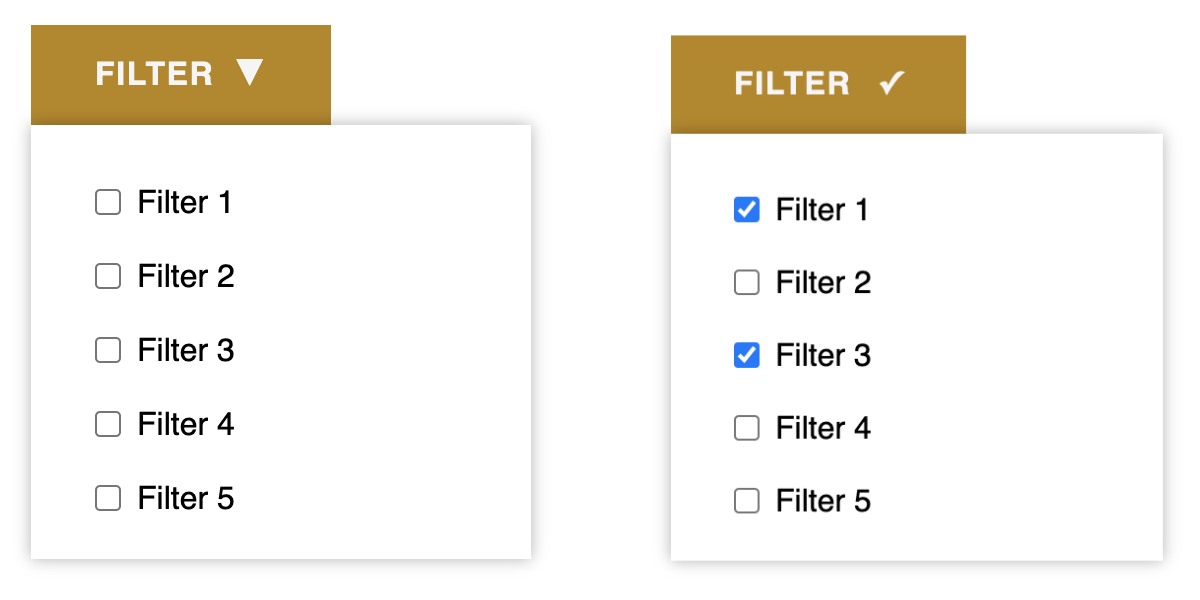
How to get parent element in javascript. We can use JavaScript to get parent element or jQuery to get parent element but which one would be best for you is up to your need and familiarity with JavaScript and jQuery. In this article we will take different conditions and examples to show how jquery finds parent element and same case with JavaScript. Knowing the parent of an element is useful when you are trying to position them out the "real-flow" of elements. Below given code will output the id of parent of element whose id is provided. Can be used for misalignment diagnosis. The parentElement property returns the parent element of the specified element. The difference between parentElement and parentNode, is that parentElement returns null if the parent node is not an element node: In most cases, it does not matter which property you use, however, parentNode is probably the most popular. This property is read-only.
Select an element whose child element is going to be selected. Use .children property to get access of all the children of element. Select the particular child based on index. Example 1: This example implements the above approach. Finding child element of parent with pure JavaScript. up.innerHTML = "Click on the button select the child node."; To get the parent node of an HTML element, you can use the parentNode property. This property returns the parent node of the specified element as a Node object.. Let us say you have the following HTML code: To get the parent of an element, you use the parentNode property of the element. The following code gets the parent node of the element with the id main: const current = document .querySelector ( '#main' ); const parent = current.parentNode; Code language: JavaScript (javascript) How it works: First, select the element with the CSS selector # ...
How to get the child element of a parent using JavaScript? Javascript Web Development Object Oriented Programming. Following is the code for getting the child element of a parent using JavaScript −. 1.1 - Get parent element AKA DOM NODE if there is one. Once a reference to an element is gained by use of a method like getElementById or querySelector, there is the parentElement property of the element reference if it has one. This property is there to get a parent DOM element rather than a node in general, as there are some kinds of nodes ... Often you'll want your JavaScript functions to access parent elements in the DOM. To accomplish this in JavaScript, try element.parentNode . To do the same in jQuery, try element.parent() .
Element.closest () The closest () method traverses the Element and its parents (heading toward the document root) until it finds a node that matches the provided selector string. Will return itself or the matching ancestor. If no such element exists, it returns null. closest. Ancestors of an element are: parent, the parent of parent, its parent and so on. The ancestors together form the chain of parents from the element to the top. The method elem.closest(css) looks for the nearest ancestor that matches the CSS-selector. The elem itself is also included in the search.. In other words, the method closest goes up from the element and checks each of parents. The task is to get the index of child element among other children. Here are few techniques discussed. Approach 1: Select the child element of parent element. Select the parent by .parentNode property. Use Array.prototype.indexOf.call(Children_of_parent, current_child) to get the index. Example 1: This example using the approach discussed above.
Get the current computed width for the first element in the set of matched elements or set the width of every matched element..width() Description: Get the current computed width for the first element in the set of matched elements. The difference between .css( "width" ) and .width() is that the latter returns a unit-less pixel value (for example, 400) while the former returns a value with ... 10/8/2021 · Note that parent element of cartError is p tag not cart-item-details. Actually you need to use cartError.parentElement.parentElement to access the cart-item-details and then use … Solution 1. Accept Solution Reject Solution. Try parentNode instead of parent. JavaScript HTML DOM Elements (Nodes) [ ^] Here is a common workaround: Find the child you want to remove, and use its parentNode property to find the parent: JavaScript. Copy Code. var child= document .getElementById ( "p1" ); child. parentNode .removeChild (child);
javascript get parent element; javascript object length; add style javascript; hide div in javascript; javascript list length; How to uninstall npm modules in node js? npm react router dom; javascript date today dd mm yyyy; javascript get random array value; window.href; javascript get attribute; javascript remove event listener; string to ... Keep the script simple, and just get every parent element every time. Provide an option to filter parent elements by a selector (only returning parent elements that have a class of .pick-me, for example. 1. Get all parents. If you go this route, we can remove the selector argument from the function, and pull out the matches() polyfill. How to call a parent window function from an iframe using JavaScript? Search Element in a Dictionary using Javascript; Last element of vector in C++ (Accessing and updating) Accessing an array returned by a function in JavaScript; Fixing the Collapsed Parent using CSS; Place an element in the middle of the parent element in Bootstrap 4 ...
Using the jQuery Parent Selector. No single CSS solution can fix our issues here, so let's see what JavaScript can do for us instead. In this example we are going to use jQuery for its robust APIs, convenience, and browser compatibility. At the time of writing, jQuery provides three methods to select parent elements. First, Let's get the reference to our h1 tag. // reference to h1 tag const h1 = document.querySelector("#main-heading"); Now let's use the closest() DOM element method and pass the selector string for the parent tag that is the .article class name as an argument to it. To get the parent node of a specified node in the DOM tree, you use the parentNode property: let parent = node.parentNode; Code language: JavaScript (javascript) The parentNode is read-only. The Document and DocumentFragment nodes do not have a parent, therefore the parentNode will always be null. If you create a new node but haven't attached ...
In this example, when someone clicks an .accordion-toggle link, I want to get the .accordion element, but I don't neccessarily know how many nested div's will be in the markup.. Creating a helper function. To do this, we can setup a for loop to climb up the DOM. On each loop, we'll grab the element's .parentNode and see if it has our selector. If it doesn't, we'll jump to the next ... In the above HTML, the div element with ID "parentDiv" is the parent element and the div element inside it with the ID "childDiv" is the child element of its parent div. Now we want to get the child element of the parent div element in JavaScript. Given a jQuery object that represents a set of DOM elements, the parent() method traverses to the immediate parent of each of these elements in the DOM tree and constructs a new jQuery object from the matching elements.. This method is similar to .parents(), except .parent() only travels a single level up the DOM tree. Also, $( "html" ).parent() method returns a set containing document whereas ...
Now we have to get the parent ID of the child element with the ID "childID". We can do it very easily using just one line of JS code. Below is our JavaScript code to get the parent ID of our element: alert (document.getElementById("childID").parentElement.id); Using the above code, we are showing the ID of the parent element as an alert text. JavaScript DOM — Get the children of an element. To get all child nodes of an element, you can use the childNodes property. This property returns a collection of a node's child nodes, as a NodeList object. By default, the nodes in the collection are sorted by their appearance in the source code. You can use a numerical index (start from 0) to ... Definition and Usage. The parentNode property returns the parent node of the specified node, as a Node object. Note: In HTML, the document itself is the parent node of the HTML element, HEAD and BODY are child nodes of the HTML element. This property is read-only.
Get The Id Of The Parent Tag In Js Programmer Sought
A Simple Explanation Of Event Delegation In Javascript
Get Parent Node With Getelementsbyclassname Stack Overflow
The Difference Between Parentnode Parentelement Childnodes
Javascript Fundamental Es6 Syntax Return True If The
Css4 Today Address Parent Elements Using Cssparentselector
How To Get The Child Element Of A Parent Using Javascript
Jquery Find Nested Parent Child Elements Html Dom Pakainfo
Child And Sibling Selectors Css Tricks
Jquery Is It Possible In Js Jquery To Access The Parent
Javascript Parent Node How Does Parent Node Done In Javascript
How To Call A Block Action In A Mobile Screen Outsystems
Vuejs Parent Child Communication Vegibit
Get Closest Element Vanilla Js Tutorial
Meet Has A Native Css Parent Selector And More Smashing
Javascript Dom Nodes Tutorial Republic
Challenge Remove The Removeme Element From The Parent
How To Get The Last Child Of A Parent Dom Element In
Building Interactive Websites With Javascript Javascript And
Javascript Dom Get The Parent Of An Element
Position A Child Div Relative To Parent Container In Css
Xpath Contains Following Sibling Ancestor Amp Selenium And Or
Check If The Element Is The First Child In Jquery
Event Bubbling And Event Capturing In Javascript By Vaibhav
Chapter 10 Document Object Model Dom Objects And
How Does Js Get The Value Of The Parent Element Of The
How To Create Element In Javascript With Examples
How To Get The Parent Name Of A Clicked Element In Gtm Tag
How To Get Key Attribute Of Parent Div When Button Is Clicked
How To Find The First Scrollable Parent Element With
Javascript 親要素 親ノードを取得する Parentelement
0 Response to "35 How To Get Parent Element In Javascript"
Post a Comment